C++对象所有属性(包括指针属性)内存连续分配*
#include <string.h>
#include <iostream>
using namespace std;
struct TestStruct {
char *a{nullptr};
char *b{nullptr};
char *c{nullptr};
int id;
};
int main(int argc, const char *argv[]) {
// sizeof(TestStruct): 实际长度为:3个指针占有的长度 + 一个int的字节数=28,但是输出值为32,因为对齐规则有一条:
// "结构总尺寸也要对齐. 要为最大尺寸的成员的整数倍",所以结果为32
cout << "TestStruct sizeof: " << sizeof(TestStruct) << endl;
cout << endl;
int size = sizeof(TestStruct) + 6 + 3 + 2;
auto st = (TestStruct *)malloc(size);
st->id = 100;
// reinterpret_cast<char *>(st) + sizeof(TestStruct)的逻辑容易错误的写成:st + sizeof(TestStruct)
char *d = reinterpret_cast<char *>(reinterpret_cast<char *>(st) + sizeof(TestStruct));
const char *c = "qiumc";
memcpy(d, c, 6);
st->a = d;
c = "11";
d = reinterpret_cast<char *>(reinterpret_cast<char *>(st) + sizeof(TestStruct) + 6);
memcpy(d, c, 3);
st->b = d;
c = "x";
d = reinterpret_cast<char *>(reinterpret_cast<char *>(st) + sizeof(TestStruct) + 6 + 3);
memcpy(d, c, 2);
st->c = d;
cout << st->a << endl;
cout << st->b << endl;
cout << st->c << endl;
cout << st->id << endl;
}
内存分析如下:这样可以使得结构体的所有属性都在同一段内存中连续分配(1~8, 包括结构体本身,内存连续分配)
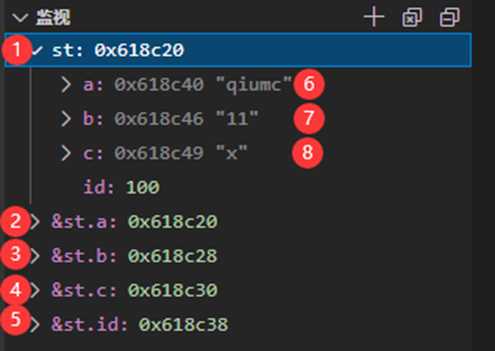