TestMap HashMap的常见操作
public class TestMap { public static void main(String[] args) { Map map=new HashMap(); //在此映射中关联指定值与指定键。 map.put("key1", "value1"); map.put("key2", "value2"); map.put("key3", "value2"); map.put("key4", "value4"); map.put("key5", "value5"); map.put("key6",null); map.put(null,null); System.out.println(map.size()); System.out.println(map.get(null)); for(int i=1;i<map.size();i++){ System.out.println(map.get(i)); } Iterator it=map.keySet().iterator(); while(it.hasNext()){ Object key=it.next(); Object value=map.get(key); System.out.println("key="+key+"-->"+"value="+value); } Iterator itt=map.entrySet().iterator(); while(itt.hasNext()){ System.out.println(itt.next()); } Iterator it2=map.entrySet().iterator(); while(it2.hasNext()){ Map.Entry key=(Map.Entry) it2.next(); System.out.println("key="+key.getKey()+"-->"+"value="+key.getValue()); } } }
HashMap
package com.example.demo; import org.testng.annotations.Test; import java.util.HashMap; public class TestHashMap { @Test(description = "Hashmap的存值") public void TestPut() { ///*Integer*/map.put("1", 1); // 向map中添加值(返回这个key以前的值,如果没有返回null) HashMap<String, Integer> map=new HashMap<>(); Integer flag_a = map.put("1", 1); Integer flag_b = map.put("1", 2); System.out.println(flag_a);//null System.out.println(flag_b);//1 } @Test(description = "Hashmap的取值") public void TestGet() { HashMap<String, Integer> map=new HashMap<>(); map.put("key", 1); /*Value的类型*///得到map中key相对应的value的值 System.out.println(map.get("1"));//null System.out.println(map.get("key"));//1 } @Test(description = "Hashmap的判断为空") public void TestCheckEmpty() { HashMap<String, Integer> map=new HashMap<>(); /*boolean*///判断map是否为空 System.out.println(map.isEmpty());//true map.put("key", 1); System.out.println(map.isEmpty());//false } @Test(description = "Hashmap判断是否含有key") public void TestCheckKey() { HashMap<String, Integer> map=new HashMap<>(); /*boolean*///判断map中是否存在这个key System.out.println(map.containsKey("key"));//false map.put("key", 1); System.out.println(map.containsKey("key"));//true } @Test(description = "Hashmap判断是否含有value") public void TestCheckValue() { HashMap<String, Integer> map=new HashMap<>(); /*boolean*///判断map中是否存在这个value boolean flag_a = map.containsValue(1); System.out.println(flag_a);//false map.put("key", 1); boolean flag_b = map.containsValue(1); System.out.println(flag_b);//true } @Test(description = "Hashmap删除这个key值下的value") public void TestDelValue() { HashMap<String, Integer> map=new HashMap<>(); /*Integer*///删除key值下的value Integer result =map.remove("key1"); System.out.println(result);//null map.put("key1", 2); System.out.println(map.remove("key1"));//2(删除的值) } @Test(description = "Hashmap显示所有的value值") public void TestGetAllValue() { HashMap<String, Integer> map=new HashMap<>(); /*Collection<Integer>*///显示所有的value值 System.out.println(map.values());//[] map.put("key1", 1); map.put("key2", 2); System.out.println(map.values());//[1, 2] } @Test(description = "Hashmap的元素个数") public void TestGetNumber() { HashMap<String, Integer> map=new HashMap<>(); /*int*///显示map里的值得数量 System.out.println(map.size());//0 map.put("key1", 1); System.out.println(map.size());//1 map.put("key2", 2); System.out.println(map.size());//2 } @Test(description = "Hashmap删除这个key值下的value") public void TestDelKeysValue() { HashMap<String, Integer> map=new HashMap<>(); /*SET<String>*///显示map所有的key System.out.println(map.keySet());//[] map.put("key1", 1); System.out.println(map.keySet());//[key1] map.put("key2", 2); System.out.println(map.keySet());//[key1, key2] } @Test(description = "Hashmap删除这个key值下的value") public void TestShow() { HashMap<String, Integer> map=new HashMap<>(); /*SET<map<String,Integer>>*///显示所有的key和value System.out.println(map.entrySet());//[] map.put("key1", 1); System.out.println(map.entrySet());//[key1=1] map.put("key2", 2); System.out.println(map.entrySet());//[key1=1, key2=2] } @Test(description = "Hashmap添加另一个同一类型的map下的所有内容") public void TestContainShow() { HashMap<String, Integer> map=new HashMap<>(); HashMap<String, Integer> map1=new HashMap<>(); /*void*///将同一类型的map添加到另一个map中 map1.put("key11", 1); map.put("key", 2); System.out.println(map);//{key=2} map.putAll(map1); System.out.println(map);//{key11=1, key11=2} } @Test(description = "Hashmap删除这个key和value") public void TestDelKeyandValue() { HashMap<String, Integer> map=new HashMap<>(); /*boolean*///删除这个键值对 map.put("TestDelKeyandValue1", 1); map.put("TestDelKeyandValue2", 2); System.out.println(map);//{DEMO1=1, DEMO2=2} System.out.println(map.remove("TestDelKeyandValue2", 1));//false System.out.println(map.remove("TestDelKeyandValue2", 2));//true System.out.println(map);//{TestDelKeyandValue1=1} } @Test(description = "Hashmap替换这个key的value:(java8)") public void TestReplaceValue() { HashMap<String, Integer> map=new HashMap<>(); /*value*///判断map中是否存在这个key map.put("key1", 1); map.put("key2", 2); map.put("key3", 3); System.out.println(map);//{key1=1, key2=2} System.out.println(map.replace("key2", 22));//2 System.out.println(map.replace("key3", 33));//3 System.out.println(map);//{key1=1, key2=22} } @Test(description = "清空这个hashmap") public void TestClear() { HashMap<String, Integer> map=new HashMap<>(); /*void*///清空map map.put("key1", 1); map.put("key2", 2); System.out.println(map);//{key1=1, key2=2} map.clear();//2 System.out.println(map);//{} } @Test(description = "Hashmap的克隆") public void TestClone() { HashMap<String, Integer> map=new HashMap<>(); /*object*///克隆这个map map.put("DEMO1", 1); map.put("DEMO2", 2); System.out.println(map.clone());//{DEMO1=1, DEMO2=2} Object clone = map.clone(); System.out.println(clone);//{DEMO1=1, DEMO2=2} } }
(java8新增方法)
如果当前 Map
不存在键 key 或者该 key 关联的值为 null
,那么就执行 put(key, value)
;否则,便不执行 put
操作
public static void main(String[] args) { HashMap<String, Integer> map=new HashMap<>(); /*boolean*///判断map中是否存在这个key map.put("DEMO1", 1); map.put("DEMO2", 2); System.out.println(map);//{DEMO1=1, DEMO2=2} System.out.println(map.putIfAbsent("DEMO1", 12222));//1 System.out.println(map.putIfAbsent("DEMO3", 12222));//null System.out.println(map);//{DEMO1=1, DEMO2=2} }
如果当前 Map
的value为xx时则值为xx否则为xx:(java8新增方法)compute
方法更适用于更新 key 关联的 value 时,新值依赖于旧值的情况
public static void main(String[] args) { HashMap<String, Integer> map=new HashMap<>(); /*boolean*///当这个value为null时为1,否则为3 map.put("DEMO1", 1); map.put("DEMO2", 2); System.out.println(map);//{DEMO1=1, DEMO2=2} map.compute("DEMO2", (k,v)->v==null?1:3); System.out.println(map);//{DEMO1=1, DEMO2=3} }
我们提一个需求:给定一个 List<String>,统计每个元素出现的所有位置。
比如,给定 list:["a", "b", "b", "c", "c", "c", "d", "d", "d", "f", "f", "g"] ,那么应该返回:
a : [0]
b : [1, 2]
c : [3, 4, 5]
d : [6, 7, 8]
f : [9, 10]
g : [11]
很明显,我们很适合使用 Map 来完成这件事情:
package com.example.demo; import java.util.*; public class TestSort { public static Map<String, List<Integer>> getElementPositions(List<String> list) { Map<String, List<Integer>> positionsMap = new HashMap<>(); for (int i = 0; i < list.size(); i++) { String str = list.get(i); List<Integer> positions = positionsMap.get(str); if (positions == null) { // 如果 positionsMap 还不存在 str 这个键及其对应的 List<Integer> positions = new ArrayList<>(1); positionsMap.put(str, positions); // 将 str 及其对应的 positions 放入 positionsMap } positions.add(i); // 将索引加入 str 相关联的 List<Integer> 中 } return positionsMap; } public static void main(String[] args) throws Exception { List<String> list = Arrays.asList("a", "b", "b", "c", "c", "c", "d", "d", "d", "f", "f", "g"); System.out.println("使用 Java8 之前的 API:"); Map<String, List<Integer>> elementPositions = getElementPositions(list); System.out.println(elementPositions); } }
Java8 时,Map<K, V> 接口添加了一个新的方法,putIfAbsent(K key, V value),功能是:
如果当前 Map 不存在键 key 或者该 key 关联的值为 null,那么就执行 put(key, value);否则,便不执行 put 操作
package com.example.demo; import java.util.*; public class TeatSort2 { public static Map<String, List<Integer>> getElementPositions(List<String> list) { Map<String, List<Integer>> positionsMap = new HashMap<>(); for (int i = 0; i < list.size(); i++) { String str = list.get(i); positionsMap.putIfAbsent(str, new ArrayList<>(1)); // 如果 positionsMap 不存在键 str 或者 str 关联的 List<Integer> 为 null,那么就会进行 put;否则不执行 put positionsMap.get(str).add(i); } return positionsMap; } public static void main(String[] args) throws Exception { List<String> list = Arrays.asList("a", "b", "b", "c", "c", "c", "d", "d", "d", "f", "f", "g"); System.out.println("使用 putIfAbsent:"); Map<String, List<Integer>> elementPositions = getElementPositions(list); System.out.println(elementPositions); } }
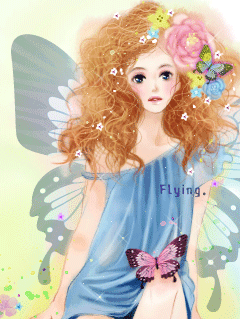