Springboot Thymeleaf 发邮件 将html内容展示在邮件内容中
需要的依赖主要如下:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
代码如下:
主要文件有interface Mailserver 和 MailServerImpl
代码:
package com.example.demo.email;
public interface MailService {
void sendSimpleMail(String to, String subject, String content);
void sendHtmlMail(String to, String cc,String subject, String content);
void sendAttachmentsMail(String to, String subject, String content, String filePath);
}
package com.example.demo.email;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.FileSystemResource;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Component;
import org.springframework.stereotype.Service;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import java.io.File;
@Component
//@Service
public class MailServiceImpl implements MailService {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
@Autowired
private JavaMailSender javaMailSender;
@Value("${spring.mail.username}")
private String from;
@Value("${spring.mail.bcc}")
private String bcc;
@Override
public void sendSimpleMail(String to, String subject, String content) {
SimpleMailMessage message = new SimpleMailMessage();
message.setFrom(from);
message.setTo(to);
message.setSubject(subject);
message.setText(content);
try {
javaMailSender.send(message);
logger.info("发送简单邮件成功!");
} catch (Exception e) {
logger.error("发送简单邮件时发生异常!", e);
}
}
@Override
public void sendHtmlMail(String to, String cc,String subject, String content) {
MimeMessage message = javaMailSender.createMimeMessage();
try {
//true表示需要创建一个multipart message
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setFrom(from);
helper.setTo(to);//邮件接收者
helper.setCc(cc);
helper.setBcc(bcc);
helper.setSubject(subject);//邮件主题
helper.setText(content, true);//邮件内容
FileSystemResource avatar = new FileSystemResource("image/Jasmine.png");
helper.addInline("avatar",avatar);
javaMailSender.send(message);
logger.info("发送HTML邮件成功!");
} catch (MessagingException e) {
logger.error("发送HTML邮件时发生异常!", e);
}
}
@Override
public void sendAttachmentsMail(String to, String subject, String content, String filePath) {
MimeMessage message = javaMailSender.createMimeMessage();
try {
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setFrom(from);
helper.setTo(to);
helper.setSubject(subject);
helper.setText(content, true);
FileSystemResource file = new FileSystemResource(new File(filePath));
String fileName = filePath.substring(filePath.lastIndexOf(File.separator));
helper.addAttachment(fileName, file);
javaMailSender.send(message);
logger.info("发送带附件的邮件成功!");
} catch (MessagingException e) {
logger.error("发送带附件的邮件时发生异常!", e);
}
}
}
如何调用
Context context = new Context();
context.setVariable("bugnum", "bugnum#"+bugNotifyBean.getId());
context.setVariable("pro_img", "avatar");
context.setVariable("bugdes", bugNotifyBean.getDescription());
context.setVariable("bugstatus", bugNotifyBean.getBugStatus());
context.setVariable("crname", bugNotifyBean.getCrname());
context.setVariable("crnum", bugNotifyBean.getCrnum());
context.setVariable("oname", bugNotifyBean.getOname());
context.setVariable("pname", bugNotifyBean.getPname());
context.setVariable("rca", bugNotifyBean.getRca());
context.setVariable("tasknum", bugNotifyBean.getTasknum());
context.setVariable("solution", bugNotifyBean.getSolution());
String emailContent = templateEngine.process("emailTemplate", context);
mailService.sendHtmlMail(developerEmail, testerEmail,"您好,有一个Bug要关注,谢谢!!", emailContent);
1. 买坑之旅
org.springframework.mail.MailSendException: Failed messages: javax.mail.MessagingException: IOException while sending message;
nested exception is:
java.io.FileNotFoundException: image\Jasmine.png
; message exception details (1) are:
2. html中带有图片,即
<html><body><img src="cid:avatar"/></body></html>
@Override
public void sendHtmlMailwithImage(String to, String cc, String subject, String content) {
MimeMessage message = javaMailSender.createMimeMessage();
try {
//true表示需要创建一个multipart message
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setFrom(from);
helper.setTo(to);//邮件接收者
helper.setCc(cc);
helper.setSubject(subject);//邮件主题
helper.setText(content, true);//邮件内容
String imagePath="src/main/resources/proba.jpg";
FileSystemResource fileSystemResource = new FileSystemResource(imagePath);
//FileSystemResource avatar = new FileSystemResource("image/Jasmine.png");
System.out.println(fileSystemResource);
helper.addInline("avatar",fileSystemResource);
javaMailSender.send(message);
System.out.println("发送HTML邮件成功!");
} catch (MessagingException e) {
System.out.println("发送HTML邮件时发生异常!"+ e);
}
}
更新于20190701 读取图片,加载到邮件内容中
利用springboot-mail除了发送上面三种邮件格式
简单邮件内容
html邮件内容
带有附件的邮件内容,还可以读取pic,组装到对应的html中
String imagePath="src/main/resources/proba.jpg";
FileSystemResource fileSystemResource = new FileSystemResource(imagePath);
//FileSystemResource avatar = new FileSystemResource("image/Jasmine.png");
System.out.println(fileSystemResource);
helper.addInline("avatar",fileSystemResource);
获取pic的路径,如果是在程序中执行,可以给出相对路径
如果部署到服务上去,我给出server的详细路径也可
html中获取用格式:
<html><body><img src="cid:avatar"/></body></html>
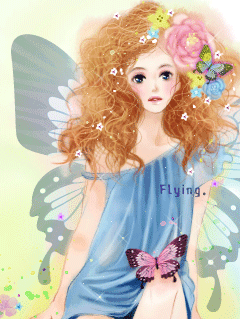