.NET实现应用程序登录Web页
一、VB.NET Code
Imports System.Text Imports System.Net Imports System.IO Public Class UwantsLogin Private loginID As String = "spang" Private loginPass As String = "123456" Public Sub New() Dim encoding As New UTF8Encoding Dim postData As String = "username=" & loginID & "&password=" & loginPass Dim data() As Byte = encoding.GetBytes(postData) 'Prepare web request Dim request As HttpWebRequest = CType(WebRequest.Create("http://www5.uwants.com/logging.php?action=login"), HttpWebRequest) request.Method = "POST" request.ContentType = "application/x-www-form-urlencoded" request.ContentLength = data.Length Dim newStream As Stream = request.GetRequestStream() 'Send the data newStream.Write(data, 0, data.Length) newStream.Close() 'Get response Dim response As HttpWebResponse = CType(request.GetResponse(), HttpWebResponse) Dim reader As StreamReader = New StreamReader(response.GetResponseStream(), System.Text.Encoding.GetEncoding("big5")) Dim content As String = reader.ReadToEnd() End Sub End Class
二、Visual C# Code
using Microsoft.VisualBasic; using System; using System.Collections; using System.Collections.Generic; using System.Data; using System.Diagnostics; using System.Text; using System.Net; using System.IO; public class UwantsLogin { private string loginID = "spang"; private string loginPass = "123456"; public UwantsLogin() { UTF8Encoding encoding = new UTF8Encoding(); string postData = "username=" + loginID + "&password=" + loginPass; byte[] data = encoding.GetBytes(postData); //Prepare web request HttpWebRequest request = (HttpWebRequest)WebRequest.Create("http://www5.uwants.com/logging.php?action=login"); request.Method = "POST"; request.ContentType = "application/x-www-form-urlencoded"; request.ContentLength = data.Length; Stream newStream = request.GetRequestStream(); //Send the data newStream.Write(data, 0, data.Length); newStream.Close(); //Get response HttpWebResponse response = (HttpWebResponse)request.GetResponse(); StreamReader reader = new StreamReader(response.GetResponseStream(), System.Text.Encoding.GetEncoding("big5")); string content = reader.ReadToEnd(); } }
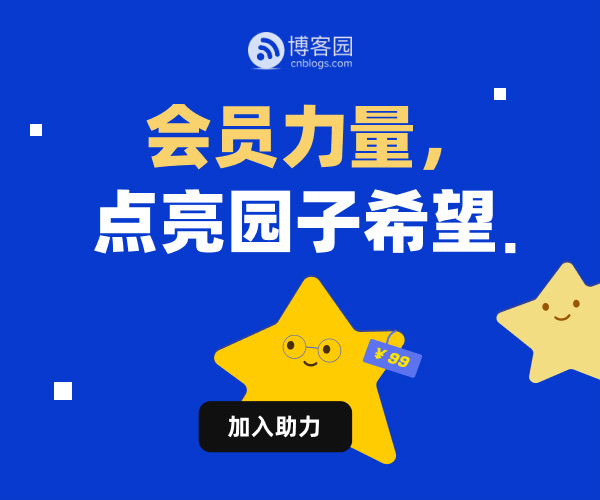