1、布局
<div class="wrapper">
<!-- 按钮组 -->
<div class="btn-box">
<div class="btn"><el-button @click="onAdd">新增</el-button></div>
<div class="btn"><el-button @click="onEdit">修改</el-button></div>
<div class="btn">
<el-button @click="onDel" type="danger">删除</el-button>
</div>
<div class="btn search">
<el-input placeholder="请输入名称过滤查询" v-model="searchValue">
</el-input>
</div>
</div>
<!-- 表格 -->
<div class="table-box">
<el-table
ref="grid"
:data="tableDataSearch"
style="width: 100%"
@selection-change="onSelectChange"
@row-click="onRowClick"
>>
<el-table-column type="selection" width="55"> </el-table-column>
<el-table-column
v-for="(item, index) in fieldConfig"
:key="index"
:prop="item.field"
:label="item.value"
>
</el-table-column>
<el-table-column fixed="right" label="操作" width="180">
<template slot-scope="scope">
<el-button type="text" size="small" @click="onLookUp(scope)"
>查看</el-button
>
<el-button type="text" size="small" @click="onUp(scope)"
>上移</el-button
>
<el-button type="text" size="small" @click="onDown(scope)"
>下移</el-button
>
</template>
</el-table-column>
</el-table>
</div>
<!-- 弹窗 -->
<el-dialog
:title="dialogConfig.title"
:visible.sync="dialogConfig.show"
width="50%"
>
<div class="form-box">
<el-form
ref="form"
:model="formData"
label-width="80px"
:rules="formRules"
>
<el-row>
<el-col :span="12">
<el-form-item label="名称" prop="name">
<el-input v-model="formData.name" :disabled="state"></el-input>
</el-form-item>
</el-col>
<el-col :span="12">
<el-form-item label="地址" prop="address">
<el-input
v-model="formData.address"
:disabled="state"
></el-input>
</el-form-item>
</el-col>
</el-row>
<el-row>
<el-col :span="12">
<el-form-item label="爱好" prop="hobby">
<el-input v-model="formData.hobby" :disabled="state"></el-input>
</el-form-item>
</el-col>
<el-col :span="12">
<el-form-item label="学历" prop="education">
<el-select
v-model="formData.education"
placeholder="请选择爱好"
:disabled="state"
>
<el-option
v-for="(item, index) in educationData"
:key="index"
:label="item.name"
:value="item.key"
></el-option>
</el-select>
</el-form-item>
</el-col>
</el-row>
</el-form>
</div>
<span slot="footer" class="dialog-footer" v-if="!state">
<el-button @click="dialogConfig.show = false">取 消</el-button>
<el-button type="primary" @click="onSave">确 定</el-button>
</span>
</el-dialog>
</div>
2、定义数据
name: "grid",
data() {
return {
// 搜索值
searchValue: "",
// 表头字段
fieldConfig: [
{
field: "name",
value: "姓名"
},
{
field: "address",
value: "地址"
},
{
field: "hobby",
value: "爱好"
},
{
field: "education",
value: "学历"
}
],
// 表格数据
tableData: [
{
uuid: "1",
name: "1",
address: "1",
hobby: "1",
education: "1"
},
{
uuid: "2",
name: "2",
address: "2",
hobby: "2",
education: "2"
},
{
uuid: "3",
name: "3",
address: "3",
hobby: "3",
education: "3"
}
],
// 弹窗配置
dialogConfig: {
show: false,
type: "",
title: ""
},
// 表单数据
formData: {
uuid: "",
name: "",
address: "",
hobby: "",
education: ""
},
// 爱好数据
educationData: [
{
key: "01",
name: "幼本"
},
{
key: "02",
name: "大专"
},
{
key: "03",
name: "本科"
},
{
key: "04",
name: "重本"
}
],
// 表单验证
formRules: {
name: [{ required: true, message: "请输入名称", trigger: "blur" }],
address: [{ required: true, message: "请输入名称", trigger: "blur" }],
hobby: [{ required: true, message: "请输入名称", trigger: "blur" }],
education: [
{ required: true, message: "请输入名称", trigger: "change" }
]
},
// 表格选中数据
tableSelectData: [],
// 表格选中拷贝数据
tableSelectDataCopy: [],
// 弹窗页脚显示
state: false
};
},
3、计算属性
computed: {
// 查询
tableDataSearch() {
return this.tableData.filter(o => {
return o.name.indexOf(this.searchValue) > -1;
});
}
},
4、方法
methods: {
// 新增
onAdd() {
this.dialogConfig = {
show: true,
title: "添加",
type: "add"
};
this.state = false;
this.$nextTick(() => {
this.resetForm();
});
},
// 修改
onEdit() {
if (this.tableSelectData.length === 1) {
this.dialogConfig = {
show: true,
title: "修改",
type: "edit"
};
this.state = false;
this.tableSelectDataCopy = JSON.parse(
JSON.stringify(this.tableSelectData)
); // 深拷贝
this.formData = this.tableSelectDataCopy[0];
} else {
this.$message.error("请选择一条数据");
}
},
// 删除
onDel() {
const _this = this;
if (_this.tableSelectData.length > 0) {
this.$confirm("是否要删除数据, 是否继续?", "提示", {
confirmButtonText: "确定",
cancelButtonText: "取消",
type: "warning"
})
.then(() => {
// 表格数据
_this.tableData.forEach((item, index) => {
// 选中数据
_this.tableSelectData.forEach(item2 => {
if (item.uuid === item2.uuid) {
_this.tableData.splice(index, _this.tableSelectData.length);
}
});
});
_this.$message({
type: "success",
message: "删除成功!"
});
})
.catch(() => {
_this.$message({
type: "info",
message: "已取消删除"
});
});
} else {
_this.$message.error("请选择要操作的数据");
}
},
// 查看
onLookUp(rowData) {
this.dialogConfig = {
show: true,
title: "查看",
type: "Look"
};
this.formData = rowData.row;
this.state = true;
},
// 重置表单
resetForm() {
(this.formData = {
uuid: "",
name: "",
address: "",
hobby: "",
education: ""
}),
this.$refs.form.resetFields();
},
// 保存
onSave() {
const _this = this;
if (this.dialogConfig.type === "add") {
this.$refs.form.validate(valid => {
if (valid) {
_this.formData["uuid"] = (_this.tableData.length + 1).toString();
_this.tableData.push(_this.formData);
_this.tableData = JSON.parse(JSON.stringify(_this.tableData)); // 深拷贝
_this.dialogConfig.show = false;
} else {
_this.$message.error("请输入相关信息");
}
});
} else if (_this.dialogConfig.type === "edit") {
_this.tableData.forEach(item => {
if (item.uuid === _this.formData.uuid) {
item.address = _this.formData.address;
item.education = _this.formData.education;
item.hobby = _this.formData.hobby;
item.name = _this.formData.name;
}
});
_this.dialogConfig.show = false;
}
},
// 点击表格行
onRowClick(row) {
this.$refs.grid.toggleRowSelection(row);
},
// 表格多选
onSelectChange(row) {
this.tableSelectData = row;
},
// 上移
onUp(row) {
const index = row.$index;
if (index > 0) {
const length = this.tableData[index - 1];
this.$set(this.tableData, index - 1, this.tableData[index]);
this.$set(this.tableData, index, length);
}
},
// 上移
onDown(row) {
const index = row.$index;
if (index < this.tableData.length - 1) {
const length = this.tableData[index + 1];
this.$set(this.tableData, index + 1, this.tableData[index]);
this.$set(this.tableData, index, length);
}
}
}
5、样式
<style lang="less" scoped>
.wrapper {
max-width: 1200px;
height: 100%;
margin: 0 auto;
.btn-box {
border: 1px solid #ddd;
border-bottom: none;
display: flex;
padding: 20px;
.btn {
margin-right: 10px;
}
}
.table-box {
border: 1px solid #ddd;
}
.el-select {
width: 100%;
}
}
</style>
6、效果
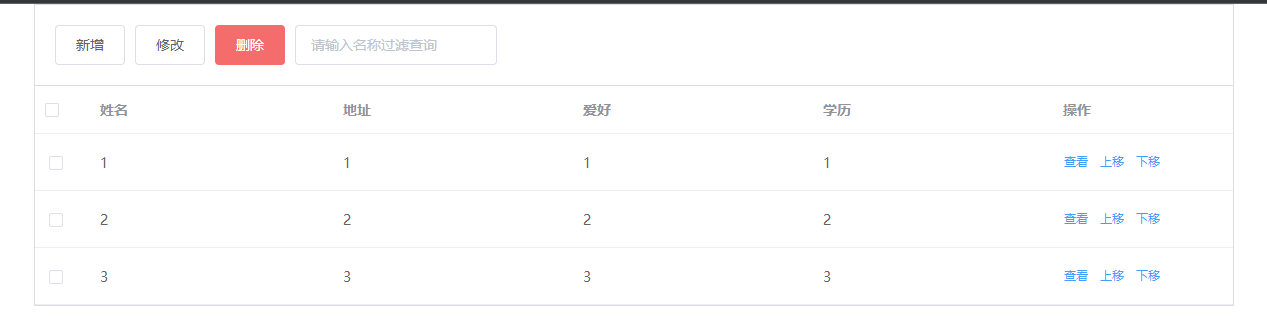