⑩ 添加筛选条件
1 实现效果

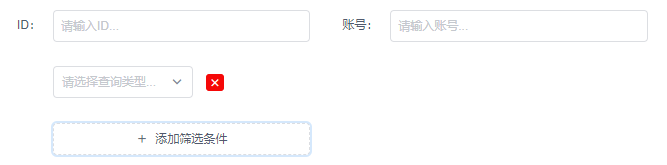
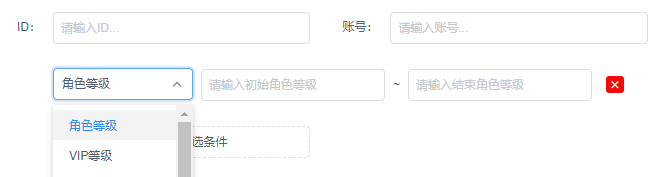
2 应用
2.1 父组件调用模板
<template>
<Row>
<Form label-position="right" :label-width="80">
<Col span="24" v-for="(conditionItem, key, index) in otherCondition.conditionItems" :key="index">
<FormItem>
<Select
v-model="conditionItem.id"
placeholder="请选择查询类型..."
@on-change="val=>{ conditionItemChange(val, conditionItem, otherCondition.otherConditionselect) }"
style="width:140px; float:left; margin-right:8px"
>
<Option
v-for="optionItem in otherCondition.otherConditionselect"
:value="optionItem.id"
:key="optionItem.id"
>{{ optionItem.name }}</Option>
</Select>
<div class="conditionItemDetails" style="width:400px; float:left;">
<form-type :conditionItem="conditionItem"></form-type>
</div>
<span class="j-sm-close-btn ml-5" @click.stop.prevent="deletecondition(key)">
<Icon type="md-close"></Icon>
</span>
<div class="clearfix"></div>
</FormItem>
</Col>
<Col :xs="24" :sm="12" :md="8" :lg="5">
<FormItem>
<Button type="dashed" long icon="md-add" @click="addOtherCondition">添加筛选条件</Button>
</FormItem>
</Col>
</Form>
</Row>
</template>
2.2 父组件相关js
属性名 | 内容 | 可选项 |
---|---|---|
id | 字段名 | |
name | 昵称 | |
type | 类型 | text - 单选文本框; texts - 多选文本框; textarea - 多行文本框;select - 单选下拉框; selects - 多选下拉框;time - 时间节点; times - 时间段 |
value | 改字段的值 | 数字-null; 字符串-''; 数组-[] |
options | 下拉选项 |
1. 查询条件
otherCondition: {
conditionItems: [],
otherConditionselect: [
{ id: 'level', name: '角色等级', type: 'texts' },
{ id: 'create_dt', name: '注册时间', type: 'times' },
{ id: 'online', name: '是否在线', type: 'select', options: [{value: 0, label: '否'}, {value: 1, label: '是'}]},
{ id: 'login_ip', name: '登录IP', type: 'text', value: '' },
{ id: 'platform', name: '登录渠道商', type: 'cascader', options: []}
]
}
2. 获取下拉数据
async getConditionPlatformMap() {
try {
let { data: result } = await this.$axios.get(`${this.url}?id=${this.id}`)
if(result.code === 1) {
let data = result.data
data.data_list.forEach(item => {
item.children.unshift({ value: 'all', label: '全部' })
})
let otherSelect = this.otherCondition.otherConditionselect
otherSelect.forEach(item => {
if(item.id === 'platform') {
item.options = data.data_list
}
})
otherSelect = null
} else {
this.$Message.warning(result.msg)
}
} catch(e) {
this.$Message.warning('操作失败')
}
}
tip:
forEach
用来修改原数组;map
会返回一个新数组
3. 其他相关方法
- 添加条件
addOtherCondition () {
this.otherCondition.conditionItems.push({ id: '' });
}
- 删除条件
deletecondition(key) {
this.otherCondition.conditionItems.splice(key, 1);
}
- 选择条件
conditionItemChange (val, item, otherConditionselect) {
for (var i = 0; i < otherConditionselect.length; i++) {
if (val === otherConditionselect[i].id) {
if (['texts', 'selects', 'times', 'cascader'].indexOf(otherConditionselect[i].type) !== -1) {
this.$set(item, 'value', []);
} else {
this.$set(item, 'value', null);
}
item['formType'] = otherConditionselect[i];
}
}
}
- 初始化其他查询条件的标识key
setOtherConditionName (datas) {
let conditionSelect = this.otherCondition.otherConditionselect;
for (var i = 0; i < conditionSelect.length; i++) {
datas[conditionSelect[i].id] = [];
}
conditionSelect = null;
}
- 获取其他查询条件
getOtherCondition (datas) {
let conditionItems = this.otherCondition.conditionItems
for(var i = 0; i < conditionItems.length; i++) {
let value = conditionItems[i].value
let type = conditionItems[i].formType.type
let itemId = conditionItems[i]['id']
if(
String(value) === 'undefined' ||
String(value) === 'null' ||
String(value) === '' ||
String(value) === '[]'
) {
// 选了查询类型但是没选值,不做处理
continue
}
if(['texts', 'selects', 'times', 'cascader'].indexOf(type) !== -1 && value.length <= 1) {
// 选了 'texts', 'selects', 'times', 'cascader' 但是没选值或只选一个值,不做处理
continue
}
if(['texts', 'selects', 'cascader'].indexOf(type) !== -1) {
let newdata = value[1] == 'all' ? value[0] + ',' : value[0] + ',' + value[1]
datas[itemId].push(newdata)
newdata = null
} else if(type === 'times') {
datas[itemId].push(
this.formatDate(new Date(value[0]), 'yyyy-MM-dd hh:mm:ss') +
',' +
this.formatDate(new Date(value[1]), 'yyyy-MM-dd hh:mm:ss')
);
} else if(type === 'time') {
datas[itemId].push(this.formatDate(value, 'yyyy-MM-dd hh:mm:ss'))
} else {
datas[itemId].push(value)
}
}
conditionItems = null
}
3 组件代码
3.1 模板代码
<template>
<div class="temp">
<div class="form-type" v-if="conditionItem.id !== ''">
<Input
type="text"
:placeholder="'请输入'+conditionItem.formType.name"
:name="conditionItem.formType.id"
v-model="conditionItem.value"
v-if="conditionItem.formType.type == 'text'"
style="width:100%;"
></Input>
<div
class="texts"
v-if="conditionItem.formType.type == 'texts'"
:name="conditionItem.formType.id"
>
<Input type="text" :placeholder="'请输入初始'+conditionItem.formType.name" v-model="conditionItem.value[0]" class="texts-options"></Input>
<span class="texts-interval">~</span>
<Input type="text" :placeholder="'请输入结束'+conditionItem.formType.name" v-model="conditionItem.value[1]" class="texts-options"></Input>
</div>
<Input
type="textarea"
:placeholder="conditionItem.formType.name"
:name="conditionItem.formType.id"
v-model="conditionItem.value"
v-if="conditionItem.formType.type == 'textarea'"
></Input>
<Select
:placeholder="'请选择'+conditionItem.formType.name"
:name="conditionItem.formType.id"
v-model="conditionItem.value"
v-if="conditionItem.formType.type == 'select'"
clearable
style="width:100%;"
>
<Option v-for="item in conditionItem.formType.options" :value="item.value" :key="item.value">{{ item.label }}</Option>
</Select>
<Select
:placeholder="'请选择'+conditionItem.formType.name"
:name="conditionItem.formType.id"
v-model="conditionItem.value"
v-if="conditionItem.formType.type == 'selects'"
multiple
style="width:100%;"
>
<Option v-for="item in conditionItem.formType.options" :value="item.value" :key="item.value">{{ item.label }}</Option>
</Select>
<Cascader
:data="conditionItem.formType.options"
:placeholder="'请选择'+conditionItem.formType.name"
:name="conditionItem.formType.id"
v-model="conditionItem.value"
v-if="conditionItem.formType.type == 'cascader'"
filterable
clearable
style="width:100%;"
></Cascader>
<DatePicker2
v-model="conditionItem.value"
:placeholder="'请选择'+conditionItem.formType.name"
:type="conditionItem.formType.type == 'times' ? 'datetimerange' :'datetime'"
:options="conditionItem.formType.type == 'times' ? optionsForRange : optionsForSingle"
split-panels
confirm
style="width:100%;"
v-if="conditionItem.formType.type == 'time' || conditionItem.formType.type == 'times'"
></DatePicker2>
</div>
</div>
</template>
3.2 父组件可传递属性 props
props: ['conditionItem']