leetcode 910. Smallest Range II
Given an array A of integers, for each integer A[i] we need to choose either x = -K or x = K, and add x to A[i] (only once).
After this process, we have some array B.
Return the smallest possible difference between the maximum value of B and the minimum value of B.
Example 1:
Input: A = [1], K = 0
Output: 0
Explanation: B = [1]
Example 2:
Input: A = [0,10], K = 2
Output: 6
Explanation: B = [2,8]
Example 3:
Input: A = [1,3,6], K = 3
Output: 3
Explanation: B = [4,6,3]
Note:
1 <= A.length <= 10000
0 <= A[i] <= 10000
0 <= K <= 10000
题目大意:每个元素可以选择+k
或者-k
,使得最后最大值-
最小值最小。
思路,贪心,先排序,然后结果肯定是选个位置pos,pos之前的元素都+k
之后的元素都-k
,那么我们就枚举这个位置,然后进行判断,首先pos这个位置一定是把数组分成两个递增的序列,那么我们枚举4个值就可以了。代码如下:
class Solution {
public:
int smallestRangeII(vector<int>& A, int K) {
sort(A.begin(), A.end());
int n = A.size();
if (n == 1) return 0;
int ans = A[n-1] - A[0];
for (int i = 0; i < n; ++i) {
int big = max(A[n-1]-K, A[i]+K);
int small = min(A[0]+K, A[i+1]-K);
ans = min(ans,big-small);
}
return ans;
}
};
原文地址:http://www.cnblogs.com/pk28/
与有肝胆人共事,从无字句处读书。
欢迎关注公众号:
欢迎关注公众号:
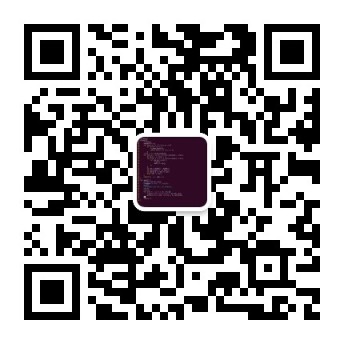