leetcode 109. Convert Sorted List to Binary Search Tree
Given a singly linked list where elements are sorted in ascending order, convert it to a height balanced BST.
For this problem, a height-balanced binary tree is defined as a binary tree in which the depth of the two subtrees of every node never differ by more than 1.
Example:
Given the sorted linked list: [-10,-3,0,5,9],
One possible answer is: [0,-3,9,-10,null,5], which represents the following height balanced BST:
0
/ \
-3 9
/ /
-10 5
思路:类似线段树,每次去区间中间那个值作为跟节点,注意出现2个数的时候我们先取右边那个,也就是比较大的那个数。
这段代码飞快。
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode(int x) : val(x), left(NULL), right(NULL) {}
* };
*/
class Solution {
public:
vector<int> v;
TreeNode* dfs(int l, int r) {
if (l > r) return nullptr;
int mid = ((l + r)%2 == 0) ? (l+r)/2 : (l+r)/2+1;
TreeNode* root = new TreeNode(v[mid]);
//root->val = ;
root->left = dfs(l, mid-1);
root->right = dfs(mid+1, r);
return root;
}
TreeNode* sortedListToBST(ListNode* head) {
ListNode* p;
p = head;
while (p) {
v.push_back(p->val);
p = p->next;
}
int l = 0, r = v.size()-1;
return dfs(l, r);
}
};
原文地址:http://www.cnblogs.com/pk28/
与有肝胆人共事,从无字句处读书。
欢迎关注公众号:
欢迎关注公众号:
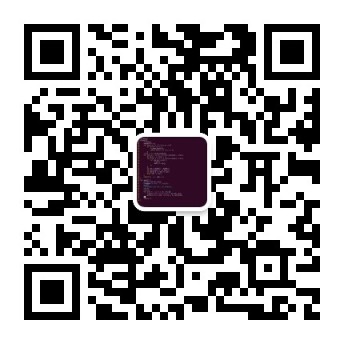