leetcode 459. Repeated Substring Pattern
Given a non-empty string check if it can be constructed by taking a substring of it and appending multiple copies of the substring together. You may assume the given string consists of lowercase English letters only and its length will not exceed 10000.
Example 1:
Input: "abab"
Output: True
Explanation: It's the substring "ab" twice.
Example 2:
Input: "aba"
Output: False
Example 3:
Input: "abcabcabcabc"
Output: True
Explanation: It's the substring "abc" four times. (And the substring "abcabc" twice.)
思路:假设子串长度为k,那么原串s的长度len为n*k(n>=2),那么s的next数组,由于对称性会在下标k+1起开始逐一递增。直到增加到len-k,如果next[len]%k==0代表s由整数个长度为k的子串构成。
class Solution {
public:
bool repeatedSubstringPattern(string s) {
int i, j;
int m = s.size();
vector<int> next(m);
for (i = 1, j = 0; i < m; ++i) {
while(j > 0 && s[i] != s[j]) j = next[j-1];
if (s[i] == s[j]) ++j;
next[i] = j;
}
int k = m - next[m-1];
return next[m-1]&&next[m-1]%k==0;
}
};
原文地址:http://www.cnblogs.com/pk28/
与有肝胆人共事,从无字句处读书。
欢迎关注公众号:
欢迎关注公众号:
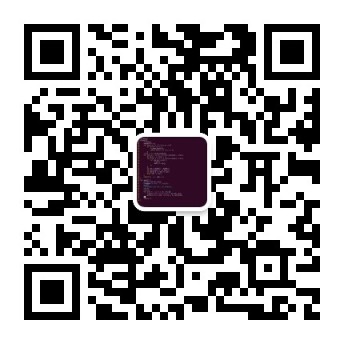