leetcode 48. Rotate Image
You are given an n x n 2D matrix representing an image.
Rotate the image by 90 degrees (clockwise).
Note:
You have to rotate the image in-place, which means you have to modify the input 2D matrix directly. DO NOT allocate another 2D matrix and do the rotation.
Example 1:
Given input matrix =
[
[1,2,3],
[4,5,6],
[7,8,9]
],
rotate the input matrix in-place such that it becomes:
[
[7,4,1],
[8,5,2],
[9,6,3]
]
Example 2:
Given input matrix =
[
[ 5, 1, 9,11],
[ 2, 4, 8,10],
[13, 3, 6, 7],
[15,14,12,16]
],
rotate the input matrix in-place such that it becomes:
[
[15,13, 2, 5],
[14, 3, 4, 1],
[12, 6, 8, 9],
[16, 7,10,11]
]
模拟一下,先看四个角,每次交换两个,交换三次就可以了。对于左上角向下走一个单位找到a,左下角向右移动一个单位b,右下角向上移动c,右上角向左移动d。交换(a,b)(b,c),(c,d)....
具体见代码:
class Solution {
public:
void rotate(vector<vector<int>>& matrix) {
int n = matrix.size();
if (n == 0) return ;
int x = n/2;
int y = n;
for (int p = 0; p < x; ++p) {
for (int i = 0; i <= y - 2; ++i) {
swap(matrix[p+i][p], matrix[n-p-1][p+i]);
swap(matrix[n-p-1][p+i], matrix[n-p-1-i][n-p-1]);
swap(matrix[n-p-1-i][n-p-1], matrix[p][n-p-1-i]);
}
y -= 2;
}
}
};
原文地址:http://www.cnblogs.com/pk28/
与有肝胆人共事,从无字句处读书。
欢迎关注公众号:
欢迎关注公众号:
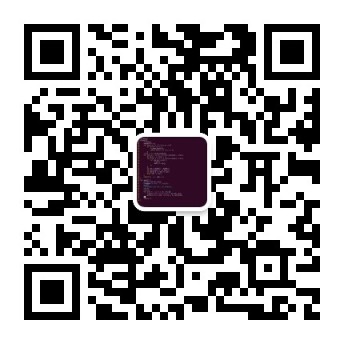