leetcode 290. Word Pattern
Given a pattern and a string str, find if str follows the same pattern.
Here follow means a full match, such that there is a bijection between a letter in pattern and a non-empty word in str.
Examples:
pattern = "abba", str = "dog cat cat dog" should return true.
pattern = "abba", str = "dog cat cat fish" should return false.
pattern = "aaaa", str = "dog cat cat dog" should return false.
pattern = "abba", str = "dog dog dog dog" should return false.
Notes:
You may assume pattern contains only lowercase letters, and str contains lowercase letters separated by a single space.
Credits:
Special thanks to @minglotus6 for adding this problem and creating all test cases
把字符数组和字符串数组,映射到‘a’~'z'然后 判断两个映射后的字符串是否相等就行
class Solution {
public:
bool wordPattern(string pattern, string str) {
map<string, char> mp;
string t = "";
int k = 0;
string ans = "";
for (int i = 0; i < str.size(); ++i) {
if (str[i] == ' ') {
if (!mp[t]) {
mp[t] = char(k + 'a');
++k;
}
ans += mp[t];
t = "";
}
else t += str[i];
}
if (t.size()) {
if (mp[t]) ans += mp[t];
else mp[t] = char(k + 'a'), ans += mp[t];
}
map<char, char> mp2;
string w = "";
k = 0;
for (int i = 0; i < pattern.size(); ++i) {
if (!mp2[pattern[i]]) {
mp2[pattern[i]] = char(k + 'a');
++k;
}
w += mp2[pattern[i]];
}
return ans == w;
}
};
原文地址:http://www.cnblogs.com/pk28/
与有肝胆人共事,从无字句处读书。
欢迎关注公众号:
欢迎关注公众号:
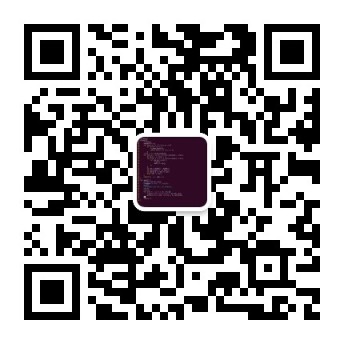