155. Min Stack
Design a stack that supports push, pop, top, and retrieving the minimum element in constant time.
- push(x) -- Push element x onto stack.
- pop() -- Removes the element on top of the stack.
- top() -- Get the top element.
- getMin() -- Retrieve the minimum element in the stack.
Example:
MinStack minStack = new MinStack(); minStack.push(-2); minStack.push(0); minStack.push(-3); minStack.getMin(); --> Returns -3. minStack.pop(); minStack.top(); --> Returns 0. minStack.getMin(); --> Returns -2.
思路:用两个栈,其中一个栈用来维护最小值序列,栈顶为当前序列最小值。
class MinStack { public: /** initialize your data structure here. */ stack<int> s; stack<int> ms; MinStack() { while(!s.empty()) s.pop(); while(!ms.empty()) ms.pop(); } void push(int x) { s.push(x); if (ms.empty() || x <= ms.top()) ms.push(x); } void pop() { int x = s.top(); s.pop(); if (!ms.empty() && x <= ms.top()) ms.pop(); } int top() { return s.top(); } int getMin() { return ms.top(); } }; /** * Your MinStack object will be instantiated and called as such: * MinStack obj = new MinStack(); * obj.push(x); * obj.pop(); * int param_3 = obj.top(); * int param_4 = obj.getMin(); */
挺巧妙的,之前一直没想出来怎么O(1)查最小值....
原文地址:http://www.cnblogs.com/pk28/
与有肝胆人共事,从无字句处读书。
欢迎关注公众号:
欢迎关注公众号:
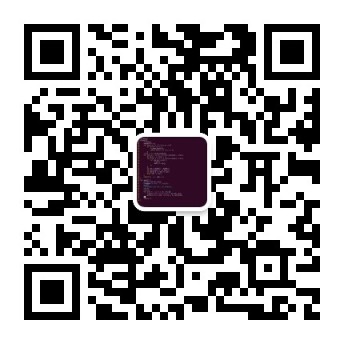