selenium4 如何支持chrome浏览器,自动下载驱动
1.驱动安装
1.1自动安装(适用于 外网可以访问的场景)
import time from selenium import webdriver from selenium.webdriver.chrome.service import Service from webdriver_manager.chrome import ChromeDriverManager option = webdriver.ChromeOptions() option.add_argument("start-maximized") driver=webdriver.Chrome(service=Service(ChromeDriverManager().install()),options=option) driver.get("https://www.baidu.com") time.sleep(3)
1.2手动安装(适用于 无法连接公网时)
import time from selenium import webdriver # 定位元素用 from selenium.webdriver.common.by import By # 模拟回车用 from selenium.webdriver.common.keys import Keys # 驱动的下载位置:https://registry.npmmirror.com/binary.html?path=chromedriver/ # 下载什么版本的驱动呢?首先查到你浏览器的版本,下载的驱动应为跟你浏览器版本一致或者最接近的版本,在上面链接中通常选择32位的,例如"chromedriver_win32.zip" # 驱动位置的写法,也和selenium的版本有很大关系 browser = webdriver.Chrome(executable_path=r"D:chromedriver.exe") # 定义下载的位置 #设置1 options = webdriver.ChromeOptions() # 更改下载路径 # 如果该路径不存在会自动创建 prefs = {"download.default_directory":'C:\Download_test','download.prompt_for_download':False} 新加一条,不加载图片 prefs = {"download.default_directory":'C:\Download_test','download.prompt_for_download':False,"profile.managed_default_content_settings.images":2} 设置2,将创建的下载部分的设置添加到option中 options.add_experimental_option('prefs',prefs) # 打开网址 # browser.get('https://cn.bing.com/') # 强制等待3秒 # 最大窗口 browser.maximize_window() time.sleep(3) browser.get('https://im.qq.com/pcqq')
2.调试模式
2.1步骤1中,正常使用selenium访问百度:调试模式会被浏览器检测到。
2.2使用代理浏览器selenium访问百度:真正的用户操作
import os import time from selenium import webdriver from selenium.webdriver.chrome.service import Service as ChromeService from webdriver_manager.chrome import ChromeDriverManager if __name__ == '__main__': # 本地谷歌浏览器地址 chrome_path = "C:\Program Files (x86)\Google\Chrome\Application\chrome.exe" # 打开代理浏览器 os.popen(rf'"{chrome_path}" --remote-debugging-port=9222 --user-data-dir="C:\selenum\AutomationProfile"') # 初始化驱动 service = ChromeService(executable_path=ChromeDriverManager().install()) # 配置 options = webdriver.ChromeOptions() # 配置代理参数 options.add_experimental_option('debuggerAddress', 'localhost:9222') # 获取浏览器实例 driver = webdriver.Chrome(service=service, options=options) # 访问百度 driver.get("https://www.baidu.com/") time.sleep(5) # 销毁实例 driver.quit()
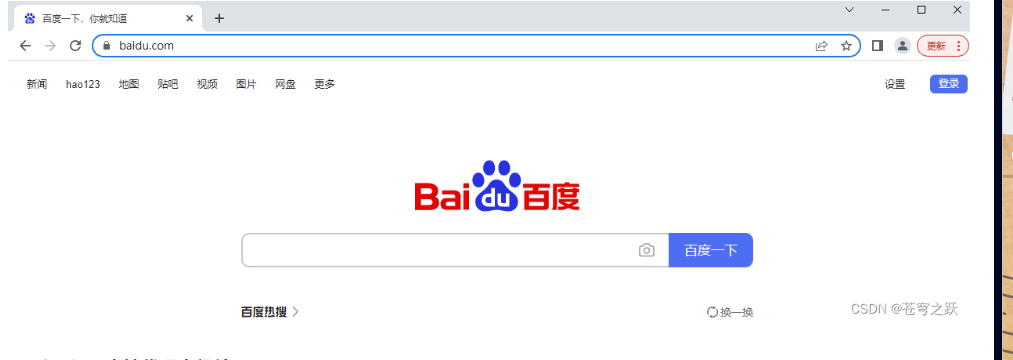
3.selenium本地代理中间件
class SeleniumMiddleware(object): def __init__(self): super().__init__() # 本地谷歌浏览器地址 chrome_path = rf"C:\Users\Lenovo\AppData\Local\Google\Chrome\Application\chrome.exe" # 打开代理浏览器 os.popen(rf'"{chrome_path}" --remote-debugging-port=9222 --user-data-dir="C:\selenum\AutomationProfile"') def process_response(self, request, response, spider): # 初始化驱动 service = ChromeService(executable_path=ChromeDriverManager().install()) # 配置代理 options = webdriver.ChromeOptions() options.add_experimental_option('debuggerAddress', '127.0.0.1:9222') # 获取浏览器实例 driver = webdriver.Chrome(service=service, options=options) # 访问网页 driver.get(request.url) # 最小化 driver.minimize_window() # 全屏 driver.maximize_window() # 指定大小 driver.set_window_rect(0, 0, 1000, 500) # 获取关键字输入框 input_element = WebDriverWait(driver, timeout=3).until(lambda d: d.find_element(By.ID, "kw")) # 输入搜索关键字 input_element.send_keys("苍穹之跃" + str(random.randint(0, 9))) # 获取【百度一下】按钮 search_button_element = WebDriverWait(driver, timeout=3).until(lambda d: d.find_element(By.ID, "su")) # 点击 search_button_element.click() # 动态加载后的网页 html = driver.page_source # 退出浏览器 driver.quit() return scrapy.http.HtmlResponse(url=request.url, body=html.encode('utf-8'), encoding='utf-8', request=request) 开启中间件: DOWNLOADER_MIDDLEWARES = { 'testproject.middlewares.SeleniumMiddleware': 543, }
4.问题:
ps:
1. ChromeDriverManager().install() doesn't work. (WebDriver manager):
https://stackoverflow.com/questions/72868256/chromedrivermanager-install-doesnt-work-webdriver-manager
Maybe your webdriver manager is outdated. 1)Uninstall 1webdriver_manager: pip uninstall webdriver_manager 2)Then install again: pip install webdriver_manager 3)And install selenium: pip install selenium
Now,it works well now