数组 指针类型 结构体 联合体 分配和访问 按地址传递 按值传递
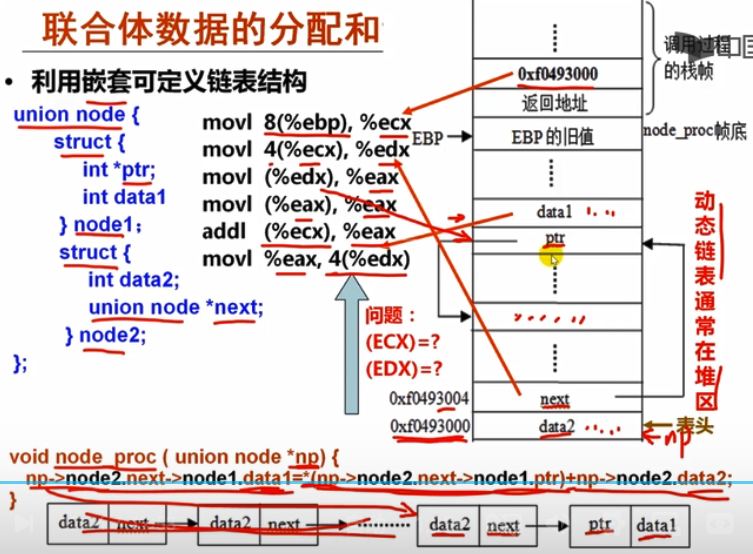
Moving scalars to or from floating point registers
movss moves a single 32 bit floating point value to or from an XMM register
movsd moves a single 64 bit floating point value
Moving packed data
The XMM registers are 128 bits
They can hold 4 floats or 2 doubles (or integers of various sizes)
Floating Point Instructions http://rayseyfarth.com/asm/pdf/ch11-floating-point.pdf 64 Bit Intel Assembly Language @2011
unsigned float2unsign(float f) { union { float f; unsigned u; } tmp_union; tmp_union.f = f; return tmp_union.u; } float unsign2float(unsigned u) { union { float f; unsigned u; } tmp_union; tmp_union.u = u; return tmp_union.f; } 1 .file "float2unsign.c" 2 .text 3 .globl float2unsign 4 .type float2unsign, @function 5 float2unsign: 6 .LFB0: 7 .cfi_startproc 8 endbr64 9 pushq %rbp 10 .cfi_def_cfa_offset 16 11 .cfi_offset 6, -16 12 movq %rsp, %rbp 13 .cfi_def_cfa_register 6 14 movss %xmm0, -20(%rbp) 15 movss -20(%rbp), %xmm0 16 movss %xmm0, -4(%rbp) 17 movl -4(%rbp), %eax 18 popq %rbp 19 .cfi_def_cfa 7, 8 20 ret 21 .cfi_endproc 22 .LFE0: 23 .size float2unsign, .-float2unsign 24 .globl unsign2float 25 .type unsign2float, @function 26 unsign2float: 27 .LFB1: 28 .cfi_startproc 29 endbr64 30 pushq %rbp 31 .cfi_def_cfa_offset 16 32 .cfi_offset 6, -16 33 movq %rsp, %rbp 34 .cfi_def_cfa_register 6 35 movl %edi, -20(%rbp) 36 movl -20(%rbp), %eax 37 movl %eax, -4(%rbp) 38 movss -4(%rbp), %xmm0 39 popq %rbp 40 .cfi_def_cfa 7, 8 41 ret 42 .cfi_endproc 43 .LFE1: 44 .size unsign2float, .-unsign2float 45 .ident "GCC: (Ubuntu 11.2.0-19ubuntu1) 11.2.0" 46 .section .note.GNU-stack,"",@progbits 47 .section .note.gnu.property,"a" 48 .align 8 49 .long 1f - 0f 50 .long 4f - 1f 51 .long 5 52 0: 53 .string "GNU" 54 1: 55 .align 8 56 .long 0xc0000002 57 .long 3f - 2f 58 2: 59 .long 0x3 60 3: 61 .align 8 62 4:
机器级代码不区分处理对象的数据类型
都把它当成一个01序列来处理。
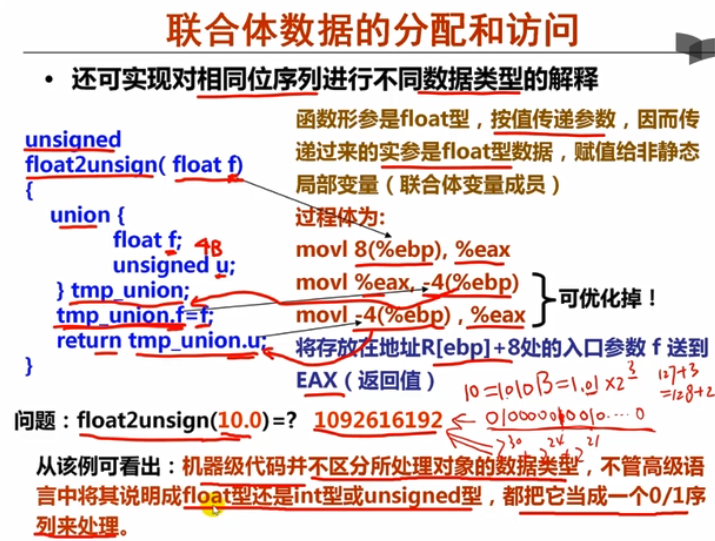
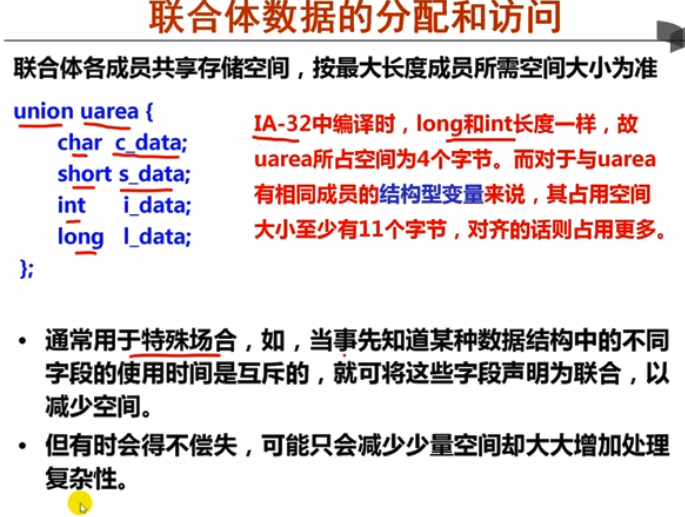
联合体:
1)当事先知道某种数据结构中的不同字段的使用时间是互斥的,
就可将这些字段声明为联合,以减少空间
2)对相同位序列实现不同数据类型的解释: 没有转换,只是解释
3)利用嵌套可以实现链表
各成员共享存储空间,按最大长度成员所需空间大小为准。
计算机系统基础(一):程序的表示、转换与链接-模块八 第2讲 结构和联合数据类型的分配和访问(2)-网易公开课 https://open.163.com/newview/movie/free?pid=WFVPGEQSL&mid=UFVPGFBJB
movl 8(%ebp),%edx
ebp+8 总是第1个参数的地址
8(%ebp)=0x8049200 赋值给edx
leal 8(%edx),%eax
将edx+8的地址赋值给eax
0x8049200+8 在静态存储区存放的是“ZhangS”
按值传递:将结构成员都复制到栈中参数区
(%edx) 取edx中的内容,
staic 存储在静态区
short {{2 9 -1 5},{}}
short 16bit int 32bit
1byte=8bit=2个16进制位表示 例如: 1100 0010=C2
行优先存放
0200 0900 ffff 0500 IA-32小端
2 9 -1 5
0300 0800 0200 faff
3 8 2 -6
2*8byte
08049300+16byte=08049310
00 93 04 08 08 93 04 08
,
08049300=num[0]
08049308=num[1]
num=num[0]=&&num[0][0]=0x8049300
pn=&pn[0]=0x8048310
pn[0]=num[0]=0x8048300
pn[1]=num[1]=0x8048308
通过指针数组,可以实现多维数组
指针数组 多维数组
访存:访问内存
A leal (%ecx),%eax 取地址
A[0] movl (%ecx),%eax 取值
i 4个字节
.globl buf
int buf[2]={10,20}; int fun(){ int i,sum=0; for(i=0;i<2;i++){ sum+=buf[i]; } return sum; } .file "array.c" .text .globl buf .data .align 8 .type buf, @object .size buf, 8 buf: .long 10 .long 20 .text .globl fun .type fun, @function fun: .LFB0: .cfi_startproc endbr64 pushq %rbp .cfi_def_cfa_offset 16 .cfi_offset 6, -16 movq %rsp, %rbp .cfi_def_cfa_register 6 movl $0, -4(%rbp) movl $0, -8(%rbp) jmp .L2 .L3: movl -8(%rbp), %eax cltq leaq 0(,%rax,4), %rdx leaq buf(%rip), %rax movl (%rdx,%rax), %eax addl %eax, -4(%rbp) addl $1, -8(%rbp) .L2: cmpl $1, -8(%rbp) jle .L3 movl -4(%rbp), %eax popq %rbp .cfi_def_cfa 7, 8 ret .cfi_endproc .LFE0: .size fun, .-fun .ident "GCC: (Ubuntu 11.2.0-19ubuntu1) 11.2.0" .section .note.GNU-stack,"",@progbits .section .note.gnu.property,"a" .align 8 .long 1f - 0f .long 4f - 1f .long 5 0: .string "GNU" 1: .align 8 .long 0xc0000002 .long 3f - 2f 2: .long 0x3 3: .align 8 4:
int fun(){ int buf[2]={10,20}; int i,sum=0; for(i=0;i<2;i++){ sum+=buf[i]; } return sum; } 1 .file "array1.c" 2 .text 3 .globl fun 4 .type fun, @function 5 fun: 6 .LFB0: 7 .cfi_startproc 8 endbr64 9 pushq %rbp 10 .cfi_def_cfa_offset 16 11 .cfi_offset 6, -16 12 movq %rsp, %rbp 13 .cfi_def_cfa_register 6 14 subq $32, %rsp 15 movq %fs:40, %rax 16 movq %rax, -8(%rbp) 17 xorl %eax, %eax 18 movl $10, -16(%rbp) 19 movl $20, -12(%rbp) 20 movl $0, -20(%rbp) 21 movl $0, -24(%rbp) 22 jmp .L2 23 .L3: 24 movl -24(%rbp), %eax 25 cltq 26 movl -16(%rbp,%rax,4), %eax 27 addl %eax, -20(%rbp) 28 addl $1, -24(%rbp) 29 .L2: 30 cmpl $1, -24(%rbp) 31 jle .L3 32 movl -20(%rbp), %eax 33 movq -8(%rbp), %rdx 34 subq %fs:40, %rdx 35 je .L5 36 call __stack_chk_fail@PLT 37 .L5: 38 leave 39 .cfi_def_cfa 7, 8 40 ret 41 .cfi_endproc 42 .LFE0: 43 .size fun, .-fun 44 .ident "GCC: (Ubuntu 11.2.0-19ubuntu1) 11.2.0" 45 .section .note.GNU-stack,"",@progbits 46 .section .note.gnu.property,"a" 47 .align 8 48 .long 1f - 0f 49 .long 4f - 1f 50 .long 5 51 0: 52 .string "GNU" 53 1: 54 .align 8 55 .long 0xc0000002 56 .long 3f - 2f 57 2: 58 .long 0x3 59 3: 60 .align 8 61 4:
取地址,而不是值:用lea,而不是mov
mov 传送 4字节 l
分配在静态区,在可执行目标文件的数据段中分配了空间
分配在栈中,通过ebp定位 局部变量、自动变量
IA-32小端,所以 首地址 0A, 低位放在低位
0A 00 00 00 14 00 00 00
908 909 90A 90B 90C 90E 911 912
计算机系统基础(一):程序的表示、转换与链接-模块八 第1讲 数组和指针类型的分配和访问(1)-网易公开课 https://open.163.com/newview/movie/free?pid=WFVPGEQSL&mid=TFVPGFAJD