QComboBox
完整代码
from PyQt6.QtWidgets import QApplication, QWidget, QComboBox, QLabel, QHBoxLayout, QVBoxLayout
from PyQt6.QtGui import QIcon, QFont
import sys
class Window(QWidget):
def __init__(self):
super().__init__()
self.setGeometry(200, 200, 700, 400) # 设置窗口大小
self.setWindowTitle("Python QComboBox")
self.setWindowIcon(QIcon('python.png')) # 设置图片,没有的话不显示
self.create_combo()
def create_combo(self):
hbox = QHBoxLayout()
label = QLabel("Select Account Type: ")
label.setFont(QFont("Times", 15))
self.combo = QComboBox()
self.combo.addItem("Current Account")
self.combo.addItem("Deposte Account")
self.combo.addItem("Saving Account")
self.combo.currentTextChanged.connect(self.combo_changed) # 当文本改变时,调用方法
vbox = QVBoxLayout()
self.label_result = QLabel("")
self.label_result.setFont(QFont("Times", 15))
vbox.addWidget(self.label_result)
hbox.addWidget(label)
hbox.addWidget(self.combo)
vbox.addLayout(hbox)
self.setLayout(vbox)
def combo_changed(self):
item = self.combo.currentText()
self.label_result.setText(f"Your Account Type is: {item}")
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec())
程序截图
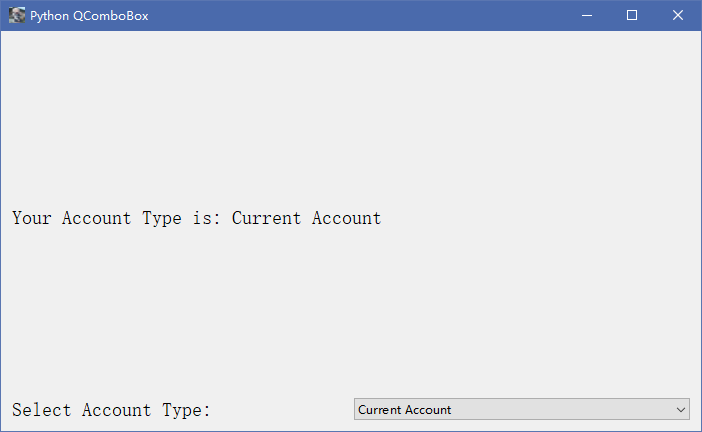
用designer设计
关键代码
self.combo.currentTextChanged.connect(self.ComboChanged) # 当前combo中,如果currentText发生改变,就传递一个signal,到ComboChanged这个slots里
完整代码
import sys
from PyQt6.QtWidgets import QApplication, QWidget, QLabel, QComboBox
from PyQt6 import uic
class UI(QWidget):
def __init__(self):
super().__init__()
uic.loadUi("ComboDemo.ui", self)
self.label_result = self.findChild(QLabel, "label_result")
self.combo = self.findChild(QComboBox, "comboBox")
self.combo.currentTextChanged.connect(self.ComboChanged)
def ComboChanged(self):
item = self.combo.currentText()
self.label_result.setText("Your favourite Language: " + item)
app = QApplication(sys.argv)
window = UI()
window.show()
app.exec()
程序截图
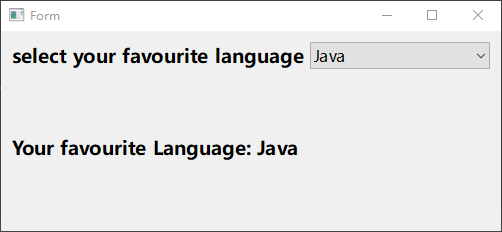