poj 2676 Sudoku
Description
Sudoku is a very simple task. A square table with 9 rows and 9 columns is divided to 9 smaller squares 3x3 as shown on the Figure. In some of the cells are written decimal digits from 1 to 9. The other cells are empty. The goal is to fill the empty cells with decimal digits from 1 to 9, one digit per cell, in such way that in each row, in each column and in each marked 3x3 subsquare, all the digits from 1 to 9 to appear. Write a program to solve a given Sudoku-task.
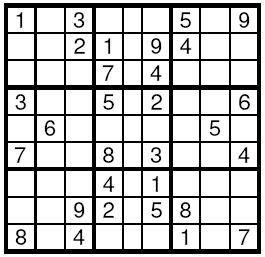
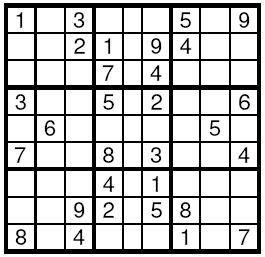
Input
The input data will start with the number of the test cases. For each test case, 9 lines follow, corresponding to the rows of the table. On each line a string of exactly 9 decimal digits is given, corresponding to the cells in this line. If a cell is empty it is represented by 0.
Output
For each test case your program should print the solution in the same format as the input data. The empty cells have to be filled according to the rules. If solutions is not unique, then the program may print any one of them.
Sample Input
1 103000509 002109400 000704000 300502006 060000050 700803004 000401000 009205800 804000107
Sample Output
143628579 572139468 986754231 391542786 468917352 725863914 237481695 619275843 854396127

1 #include <iostream> 2 #include <stdio.h> 3 #include <string.h> 4 using namespace std; 5 int num[10][10]; 6 struct node 7 { 8 int x,y; 9 }dd[85]; 10 int cnt; 11 bool mark; 12 bool judge1(int row,int col,int value)//判断一行中是否存在矛盾 13 { 14 int i; 15 for(i=0;i<9;i++) 16 { 17 if(num[row][i]==value && i!=col) 18 { 19 return false; 20 } 21 } 22 return true; 23 } 24 25 bool judge2(int row,int col,int value)//判断一列中是否存在矛盾 26 { 27 int i; 28 for(i=0;i<9;i++) 29 { 30 if(num[i][col]==value && i!=row) 31 { 32 return false; 33 } 34 } 35 return true; 36 } 37 38 bool judge3(int row,int col,int value) 39 { 40 int rr=row/3*3; 41 int cc=col/3*3; 42 int i,j; 43 for(i=rr;i<rr+3;i++) 44 { 45 for(j=cc;j<cc+3;j++) 46 { 47 if(num[i][j]==value && !(row==i && col==j)) 48 { 49 return false; 50 } 51 } 52 } 53 return true; 54 } 55 56 void dfs(int step) 57 { 58 if(step==cnt) 59 { 60 int i,j; 61 for(i=0;i<9;i++) 62 { 63 for(j=0;j<9;j++) 64 { 65 printf("%d",num[i][j]); 66 } 67 printf("\n"); 68 } 69 mark=true; 70 return; 71 } 72 if(mark) 73 { 74 return; 75 } 76 int i; 77 for(i=1;i<=9;i++) 78 { 79 num[dd[step].x][dd[step].y]=i; 80 if(!judge1(dd[step].x,dd[step].y,i) || !judge2(dd[step].x,dd[step].y,i) || !judge3(dd[step].x,dd[step].y,i)) 81 { 82 num[dd[step].x][dd[step].y]=0; 83 continue; 84 } 85 dfs(step+1); 86 if(mark) 87 { 88 return; 89 } 90 num[dd[step].x][dd[step].y]=0; 91 } 92 } 93 int main() 94 { 95 int t; 96 scanf("%d",&t); 97 while(t--) 98 { 99 char str[10]; 100 int i,j; 101 cnt=0; 102 for(i=0;i<9;i++) 103 { 104 scanf("%s",str); 105 for(j=0;j<9;j++) 106 { 107 if(str[j]=='0') 108 { 109 num[i][j]=0; 110 dd[cnt].x=i; 111 dd[cnt].y=j; 112 cnt++; 113 } 114 else 115 { 116 num[i][j]=str[j]-'0'; 117 } 118 } 119 } 120 mark=false; 121 dfs(0); 122 } 123 return 0; 124 }
数独游戏。
暴力深搜;
注意写好三个判断函数judge1(),judge2(),judge3()其他的都是简单深搜的思想