java-7311练习(下)
java练习,仅供参考!
欢迎同学们交流讨论。
JDK 1.8 API帮助文档
JDK 1.6 API中文文档
第一次小组作业:模拟双色球彩票
第一次小组作业(一) 控制台版

游戏规则:
• 双色球为红球和蓝球;
• 用户从1-33中自选6个数字(不能重复)代表红球;从1-16中自选1个数字代表蓝球;
• 上图为中奖规则,如一等奖为中6个红球及蓝球,二等奖为仅中6个红球……
• 请自拟六个奖项对应的奖品。

package GroupFirst; import java.util.Scanner; /** * 第一次小组作业:模拟双色球彩票 * •游戏规则 * •双色球为红球和蓝球 * •用户从1-33中自选6个数字(不重复)代表红球;从1-16中自选1个数字代表蓝球 * •上图为中奖规则,如一等奖为中6个红球及蓝球,二等奖为仅中6个红球…… * •请自拟六个奖项对应的奖品 */ public class Balllottery { private int[] betRedBall = new int[6];//存放选择的6个红球 private int betBlueBall; //存放选择的1个蓝球 private Scanner scan = null; //扫描器对象 private int[] winningRedBall = {1,2,3,4,5,6};//红球中奖号码 private int winningBlueBall = 7; //蓝球中奖号码 public static void main(String[] args) { Balllottery lottery = new Balllottery(); //从1-33中自选6个数字(不重复)代表红球 lottery.seletRedBall();//lottery.seletRedBall(); System.out.println("--------红球选择完成-----------"); //从1-16中自选1个数字代表蓝球 lottery.seletBlueBall(); System.out.println("--------蓝球选择完成-----------"); //投注并开奖; int level = lottery.lotteryBetting(); //显示奖品; lottery.showResults(level); } public void showResults(int level) { System.out.println("---------------------------"); System.out.print("您的投注为:"); for (int i = 0; i < betRedBall.length; i++) System.out.printf("%-3d",betRedBall[i]); System.out.print(", " + betBlueBall + "\n"); System.out.print("开奖号码为:"); for (int i = 0; i < winningRedBall.length; i++) System.out.printf("%-3d",winningRedBall[i]); System.out.print(", " + winningBlueBall + "\n\n"); //根据中奖等级分配奖品 switch (level) { case 0: System.out.println("抱歉,您没中奖!"); break; case 1: System.out.println("一等奖,恭喜您获得自行车一辆!"); break; case 2: System.out.println("二等奖,恭喜您获得保温杯一个!"); break; case 3: System.out.println("三等奖,恭喜您获得新书包一个!"); break; case 4: System.out.println("四等奖,恭喜您获得记事本一个!"); break; case 5: System.out.println("五等奖,恭喜您获得签字笔一个!"); break; case 6: System.out.println("六等奖,恭喜您获得黑铅笔一个!"); break; } System.out.println("\n---------------------------"); } // 从1-33中自选6个数字(不重复)代表红球 public void seletRedBall() { //用一个数组来存放33个红球并赋值1-33号 int[] redBall = new int[33]; for (int i = 0; i < redBall.length; i++) redBall[i] = i + 1; // 1--33 // used表示已经出现过的红球 ; boolean数组默认初始为false boolean[] used = new boolean[redBall.length]; int count = 0; //统计下注红球个数 // 输入6个不重复的红球号码,并存放到bet数组 //Scanner scan = null; scan = new Scanner(System.in); System.out.println("请输入6个红球号码(1-33):"); while (scan.hasNext()) { int num = scan.nextInt(); // 获得输入 // 如果这个号码是1-33号,那么就重新选择 if (num < 1 || num > 33) { System.out.println("没有" + num + "号,请选1-33号。您还需要选择" + (6-count) +"个红球!"); continue; } // 如果这个号码没有被选过,那么就放到bet数组 if (!used[num]) { betRedBall[count++] = num; used[num] = true; System.out.println("已选" + num + "号!您还需要选择" + (6-count) +"个红球!"); } else { System.out.println(num + "号已选过,您还需要选择" + (6-count) +"个红球!"); } // 选完6个红球则跳出循环 if (count==6) break; } } // 从1-16中自选1个数字代表蓝球 public void seletBlueBall() { // 输入1个蓝球号码 //Scanner scan = null; scan = new Scanner(System.in); System.out.print("请输入1个蓝球号码(1-16):"); while (scan.hasNextLine()) { int num = scan.nextInt(); // 获得输入 // // 如果这个号码是1-16号,那么就重新选择 if (num < 1 || num > 16) { System.out.println("没有" + num + "号,请选1-16号!"); continue; } else { betBlueBall = num; System.out.println("已选" + num + "号!"); break; } } } // 投注并开奖 public int lotteryBetting() { int correctRedCount = 0; // 猜中的红球个数 boolean correctBlueCount = false; // 是否猜中篮球 //遍历选择的红球;对比开奖结果 算出中奖的红球个数 for (int i = 0; i < betRedBall.length; i++) { for (int j = 0; j < winningRedBall.length; j++) { if (betRedBall[i] == winningRedBall[j]) { correctRedCount++; continue; } } } // 判断是否猜中蓝球 if (betBlueBall == winningBlueBall) correctBlueCount = true; /** 没中奖 返回 0 * 一等奖 中 6+1 * 二等奖 中 6+0 * 三等奖 中 5+1 * 四等奖 中 5+0 中 4+1 * 五等奖 中 4+0 中 3+1 * 六等奖 中 2+1 中 0+1 中 1+1 */ System.out.println("Debug:" + correctRedCount + "," + correctBlueCount); if (correctRedCount == 6) { if (correctBlueCount) return 1; else return 2; } if (correctRedCount == 5) { if (correctBlueCount) return 3; else return 4; } if (correctRedCount == 4) { if (correctBlueCount) return 4; else return 5; } if (correctBlueCount) { if (correctRedCount == 3) return 5; //else if (correctRedCount == 2) return 6; //else if (correctRedCount == 1) return 6; else return 6; } return 0; } }
运行结果:
第一次小组作业(二) 界面版
-------------------------2016-11-23 更新
整体概况:
-
JPanel面板的的建立与更新
窗口所有的组件都是绘制在JPanel上的,主要的变化来自于球的变化;(鼠标单击事件)获取的坐标定位到具体的球,才方便操作球的变化;每一次的变化都要更新面板内容。 -
开奖号码的绑定与设置
开奖号码显示在一个JLabel标签中,这样JFrame窗体直接通过JLabel就获取开奖号码;开奖号码按钮可以设置开奖号码,而不(方便)用通过ControlBall控制球类获取。 -
单击球的变化与清空选择
我是定义了(红蓝灰)3种类型的球,根据她们的状态来响应不同的颜色或号码;清空选择按钮是分别执行了模拟单个点击已选球的操作。 -
优化与改进
(1) 所有的绘制在JPanel上, 故绘制的坐标也是基于JPanel;而JPanel面板又被添加到JFrame窗体上,又因鼠标坐标却是基于JFrame窗体的;这两套坐标不一致,但却要用鼠标的坐标去定位球的坐标,球的坐标相对固定,而JPanel的零点相对JFrame窗体的零点却可能不一致,比如窗体边框发生变化时;两套坐标的对应是个问题,我这里只是用到当前状态的相对差距(9,30),如果窗体发生变化,可能就会不能对应坐标,这样也就会产生 选球“失灵”的情况。
(2) 设置开奖号码我这里只做了范围判断,并没有做重复判断,算是个小Bug。
(3) 这里用的的是鼠标响应时间,加上代码可能效率不高,这样不可避免的有延时;其实这里的所有的球可以换作按钮,这样通过组件操作必然方便准确,这样只需重点考虑按钮美观的问题。
运行演示: 我这里已经打包可直接运行(需要jdk环境), 点击下载 .Jar 。
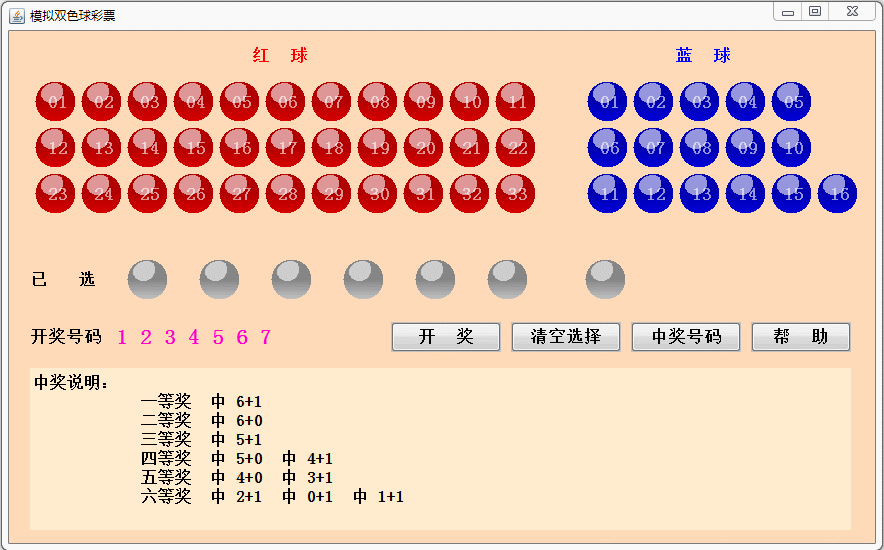
目录结构: 共享的源码只有5个类,有兴趣调试的可以参考此目录。

源码共享: 代码比较长,不在这里贴码了,有兴趣的同学可以点击下载 .rar。
week7 Cylinder(二)
2个文件 cylinder_1.dat, cylinder_0.dat都放在项目根目录的resource包下,内容如下:

7.1 Cylider.java
- package week7;
-
- import java.text.DecimalFormat;
-
- /**
- * 7.1 创建 Cylinder类,以存储标签、半度;
- * 方法包括获得及设置这些成员变量,计算直径、周长面积及体积。
- */
- public class Cylinder
- {
- private String lable; //存储标签
- private double radius; //圆柱半径
- private double height; //圆柱的高
- public Cylinder(String lable, double radius, double height)
- {
- this.lable = lable;
- this.radius = radius;
- this.height = height;
- }
-
- public String getLable()
- {
- return lable;
- }
-
- public boolean setLable(String lable)
- {
- boolean flag = true;
-
- if (lable.isEmpty()) flag = false;
- else this.lable = lable.trim();
- //String.trim()截去字符串开头和末尾的空白
- return flag;
- }
-
- public double getRadius()
- {
- return radius;
- }
-
- public void setRadius(double radius)
- {
- this.radius = radius;
- }
-
- public double getHeight()
- {
- return height;
- }
-
- public void setHeight(double height)
- {
- this.height = height;
- }
-
- //返回圆柱底面直径
- public double diameter()
- {
- return radius * 2;
- }
-
- //返回圆柱底面周长
- public double circumference()
- {
- return diameter() * Math.PI;
- }
-
- //返回 表面积 = 圆柱底面积×2 + 底面周长×高
- public double area()
- {
- return Math.PI * radius * radius * 2
- + circumference() * height;
- }
-
- //返回 圆柱底体积
- public double volumn()
- {
- return Math.PI * radius * radius * height;
- }
-
- @Override
- public String toString()
- {
- String output = null;
- DecimalFormat df = new DecimalFormat("#,##0.0##");
- output = lable
- + " is a cylinder with radius = " + df.format(radius)
- + " units and height = " + df.format(height)
- + " units, "
- + "\nwhich has diameter = " + df.format(diameter())
- + " units, circumference = " + df.format(circumference())
- + " units, "
- + "\narea = " + df.format(area())
- + " square units, and volume = " + df.format(volumn())
- + " cubic units.\n";
- return output;
- }
-
- public static void main(String[] args)
- {
- Cylinder c1 = new Cylinder("Small Example", 4.0, 10.0);
- Cylinder c2 = new Cylinder("Medium Example", 22.1, 30.6);
- Cylinder c3 = new Cylinder("Large Example", 100.0, 200.0);
- c1.setLable("");
- System.out.println(c1);
- System.out.println(c2);
- System.out.println(c3);
- }
- }
-
7.2 CylinderList.java
- package week7;
-
- import java.text.DecimalFormat;
- import java.util.ArrayList;
-
- /**
- * 7.2 CylinderList类
- */
- public class CylinderList
- {
- private String listName;
- private ArrayList<Cylinder> cList;
-
- CylinderList(String listName, ArrayList<Cylinder> cList)
- {
- this.listName = listName;
- this.cList = cList;
- }
-
- //返回一个代表几何名字的字符串
- public String getName()
- {
- return listName;
- }
-
- //返回代表集合中Cylinder对象的个数
- public int numberOfCylinders()
- {
- return cList.size();
- }
-
- //返回 所有的Cylinder对象的 高 的和
- public double totalHeight()
- {
- double totalHeight = 0;
- for (Cylinder cylinder : cList)
- {
- totalHeight += cylinder.getHeight();
- }
- return totalHeight;
- }
-
- //返回 所有的Cylinder对象的 圆柱底面直径 的和
- public double totalDiameter()
- {
- double totalDiameter = 0;
- for (Cylinder cylinder : cList)
- {
- totalDiameter += cylinder.diameter();
- }
- return totalDiameter;
- }
-
- //返回 所有的Cylinder对象的 面积 之和
- public double totalArea()
- {
- double totalArea = 0;
- for (Cylinder cylinder : cList)
- {
- totalArea += cylinder.area();
- }
- return totalArea;
- }
-
- //返回 所有的Cylinder对象的 体积 之和
- public double totalVolume()
- {
- double totalVolume = 0;
- for (Cylinder cylinder : cList)
- {
- totalVolume += cylinder.volumn();
- }
- return totalVolume;
- }
-
- //返回 所有的Cylinder对象 面积 的 平均值
- public double averageArea()
- {
- double averageArea = 0;
- if (cList.size()>0)
- {
- averageArea = totalArea()/cList.size();
- }
- return averageArea;
- }
-
- //返回 所有的Cylinder对象 体积 的 平均值
- public double averageVolume()
- {
- double averageVolume = 0;
- if (cList.size()>0)
- {
- averageVolume = totalVolume()/cList.size();
- }
- return averageVolume;
- }
-
- //返回 集合的名字及集合中每一个对象的toString方法
- public String toString()
- {
- String output = "\n" + listName + "\n\n";
- for (Cylinder cylinder : cList)
- {
- output += (cylinder.toString() + "\n");
- }
- return output;
- }
-
- //返回 集合的名字及Cylinder对象个数,
- //总面积,总体积,平均面积及平均体积
- public String summaryInfo()
- {
- String output = null;
- DecimalFormat df = new DecimalFormat("#,##0.0##");
- output = "-----" + listName + " Summary-----"
- + "\nNimber of Cylinders: " + numberOfCylinders()
- + "\nTotal Area: " + df.format(totalArea())
- + "\nTotal Volume: " + df.format(totalVolume())
- + "\nTotal Height: " + df.format(totalHeight())
- + "\nTotal Diameter: " + df.format(totalDiameter())
- + "\nAverage Area: " + df.format(averageArea())
- + "\nAverage Volume: " + df.format(averageVolume());
- return output;
- }
- }
7.3 CylinderListApp 测试类
- package week7;
-
- import java.io.File;
- import java.io.FileNotFoundException;
- import java.util.ArrayList;
- import java.util.Scanner;
-
- /**
- * 7.3 CylinderListApp 测试类
- * (a) 打开用户输入的文件并读取第一行作为集合的名字;之后读取其他行,
- * 依次生成Cylinder对象,最后生成CylinderList对象。
- * (b) 输出CylinderList对象(调用toString方法),之后空一行,
- * (c) 输出CylinderList对象的汇总信息(调用summaryInfo方法)
- * 注意:
- * 1)如果输入文件名后出现错误称找不到文件,此时可输出绝对路径
- * 2)读取文件第一行作为集合名称后,使用以scanFile.hasNext()
- * 为条件的while循环反复读入三行,然后创建Cylinder对象
- * 3)输出结果必须与下面测试输出的结果完全一致
- */
- public class CyliderListApp
- {
- public static void main(String[] args) throws FileNotFoundException
- {
- String lable;
- double radius;
- double height;
- Scanner scan0 = new Scanner(System.in);
- Scanner scan1 = null;
- Scanner inputStream = null;
-
- ArrayList<Cylinder> cList = new ArrayList<>(10);
- CylinderList cylinderList = null;//new CylinderList(listName, cList)
-
- System.out.print("Enter file name: ");
- String fileName = scan0.nextLine(); // cylinder_0.dat
- scan0.close();
-
- File file = new File("resource/" + fileName);
- if(file.exists())
- {
- inputStream = new Scanner(file);
-
- String listName = inputStream.nextLine();
- while (inputStream.hasNextLine())
- {
- String line = inputStream.nextLine();
- //使用逗号分隔line,例:Small Example, 4.0, 10.0
- scan1 = new Scanner(line);
- scan1.useDelimiter(",");
- if (scan1.hasNext())
- {
- lable = scan1.next();
- radius = Double.parseDouble(scan1.next());
- height = Double.parseDouble(scan1.next());
- //创建Cylinder对象并加入ArrayList<Cylinder>中
- cList.add(new Cylinder(lable, radius, height));
- }
- scan1.close(); //就近原则,以免出现空指针
- }
- inputStream.close();
- //初始化CylinderList对象
- cylinderList = new CylinderList(listName, cList);
- System.out.print(cylinderList.toString());
- System.out.println(cylinderList.summaryInfo());
- }
- else
- {
- System.out.println(file.getAbsolutePath());
- }
- }
- }
-
运行结果:


week8 Cylinder(三)
-------------------------2016-11-27更新
8.1 Cylider.java
导入使用的是7.1的Cylinder 类
8.2 CylinderList.java
参照 7.2 ;我这里贴出增加的部分;
- package week8;
-
- import java.io.File;
- import java.io.FileNotFoundException;
- import java.text.DecimalFormat;
- import java.util.ArrayList;
- import java.util.Scanner;
-
- import week7.Cylinder;
-
- /**
- * 8.2 同7.2 CylinderList类,只是增加了4个方法
- */
- public class CylinderList
- {
- private String listName = null;
- private Scanner scan = null;
- private Scanner inputStream = null;
- private ArrayList<Cylinder> cList = null;
-
- /**
- *
- * @param listName
- * @param cList
- */
- CylinderList(String listName, ArrayList<Cylinder> cList)
- {
- this.listName = listName;
- this.cList = cList;
- }
- /***************************以下为新增方法***********************************/
- /**
- * 接收一个代表文件名字的字符串参数,读入文件内容将其存储到集合名字变量及 ArrayList类型的集合变量中;
- * 利用集合名字及集合变量生成 CylinderList对象;最后返回该 CylinderList对象;
- * @param fileName
- * @return
- * @throws FileNotFoundException
- */
- public CylinderList readFile(String fileName) throws FileNotFoundException
- {
- CylinderList cylinderList = null;
- String lable;
- double radius;
- double height;
- String nameList = null;
-
- //读文件并赋值
- File file = new File(fileName);
- if(file.exists())
- {
- inputStream = new Scanner(file);
-
- nameList = inputStream.nextLine();
- while (inputStream.hasNextLine())
- {
- String line = inputStream.nextLine();
- //使用逗号分隔line,例:Small Example, 4.0, 10.0
- scan = new Scanner(line);
- scan.useDelimiter(",");
- if (scan.hasNext())
- {
- lable = scan.next();
- radius = Double.parseDouble(scan.next());
- height = Double.parseDouble(scan.next());
- //创建Cylinder对象并加入ArrayList<Cylinder>中
- cList.add(new Cylinder(lable, radius, height));
- }
- }
- cylinderList = new CylinderList(nameList, cList);
- }
- else
- {
- System.out.println(file.getAbsolutePath());
- System.out.println(fileName + " not exists!");
- }
-
- return cylinderList;
- }
-
- /**
- * 添加一个Cylinder对象到CylinderList对象中
- * @param label
- * @param radius
- * @param height
- */
- public void addCylinder(String label, double radius, double height)
- {
- cList.add(new Cylinder(label, radius, height));
- }
-
- /**
- * 接收一个代表 Cylinder的 label值的字符串,如果在 CylinderList对象中找到了该对象,则返回该对象并删除之;
- * 否则返回 null;
- * @param label
- * @return
- */
- public Cylinder deleteCylinder(String label)
- {
- Cylinder cylinder = null;
- for (int i = 0; i < cList.size(); i++)
- {
- //如果找到 先赋值 后 删除
- if (cList.get(i).getLable().equalsIgnoreCase(label) == true)
- {
- cylinder = cList.get(i);
- cList.remove(i);
- }
- }
- return cylinder;
- }
-
- /**
- * 参数接收收一个代表 Cylinder的 label值的字符串,如果在 CylinderList对象中找到了该对象,
- * 则返回该对象;否则返回 null;
- * @param label
- * @return
- */
- public Cylinder findCylinder(String label)
- {
- Cylinder cylinder = null;
- for (int i = 0; i < cList.size(); i++)
- {
- //如果找到 赋值
- if (cList.get(i).getLable().equalsIgnoreCase(label) == true)
- {
- cylinder = cList.get(i);
- }
- }
- return cylinder;
- }
- /**
- * String类中两个方法的比较:
- * equals:将此字符串与指定的对象比较。当且仅当该参数不为 null,
- * 并且是与此对象表示相同字符序列的 String 对象时,结果才为 true。
- * equalsIgnoreCase:将此 String 与另一个 String 比较,不考虑大小写。如果两个字符串的长度相同,
- * 并且其中的相应字符都相等(忽略大小写),则认为这两个字符串是相等的。
- */
- /***************************以上为新增方法*********************************/
-
- ....
- }
8.3 CylinderListMenuApp.java
- package week8;
-
- import java.io.IOException;
- import java.util.ArrayList;
- import java.util.Scanner;
-
- import week7.Cylinder;
-
- /**
- * 8.3 CylinderListMenuApp
- * 包含 main 方法,呈现有 7 个选项的菜单
- * (1) 读入文件内容并创建 CylinderList对象
- * (2) 打印输出 CylinderList对象
- * (3) 打印输出 CylinderList对象的汇总信息
- * (4) 增加一个 CylinderList对象至 CylinderList对象中
- * (5) 从 CylinderList对象中删除一个 Cylinder对象
- * (6) 在 CylinderList对象中找到一个 Cylinder对象
- * (7) 退出程序
- * 设计:
- * main方法中将先输出带有描述的 7个选项信息。
- * 一旦用户输入了一个选项编号,则对应 的方法将被调用。
- * 之后再次呈现选项信息,提示用户进行选择。
- */
- public class CylinderListMenuApp
- {
- /**
- * 显示菜单信息
- */
- private static void showMenu()
- {
- //输出带有描述的 7个选项信息
- System.out.println("Cylinder List System Menu");
- System.out.println("R - ReadFile and Create Cylinder List");
- System.out.println("P - Print Cylinder List");
- System.out.println("S - Print Summary");
- System.out.println("A - Add Cylinder");
- System.out.println("D - Delete Cylinder");
- System.out.println("F - Find Cylinder");
- System.out.println("Q - Quit");
- }
-
- public static void main(String[] args) throws IOException
- {
- //创建一个 Cylinder集合
- ArrayList<Cylinder> clist = new ArrayList<>();
-
- String sName = "***no list name assigned***";
- CylinderList cylinderList = new CylinderList(sName, clist);
-
-
- Scanner scan = new Scanner(System.in);
- char key; //输入的key值
-
- showMenu(); //显示提示菜单
- while (true)
- {
- //提示用户进行选择
- System.out.print("\nEnter Code [R, P, S, A, D, F or Q]: ");
-
- key = scan.nextLine().charAt(0);
- switch (key)
- {
- case 'r'://resource/cylinder_1.dat
- case 'R'://ReadFile and Create Cylinder List
- System.out.print("\tFile name: ");
- //读文件转化为CylinderList对象后重新赋值
- cylinderList = cylinderList.readFile(scan.nextLine());
- System.out.println("\tFile read in and Cylinder List created");
- break;
- case 'p':
- case 'P'://Print Cylinder List
- System.out.println(cylinderList);
- break;
- case 's':
- case 'S'://Print Summary
- System.out.println(cylinderList.summaryInfo());
- break;
- case 'a':
- case 'A'://Add Cylinder
- System.out.print("\tLabel: ");
- String labelA = scan.nextLine();
- System.out.print("\tRadius: ");
- double radius = Double.parseDouble(scan.nextLine());
- System.out.print("\tHeight: ");
- double height =Double.parseDouble(scan.nextLine());
- cylinderList.addCylinder(labelA, radius, height);
- System.out.println("\t*** Cylinder added ***");
- break;
- case 'd':
- case 'D'://Delete Cylinder
- System.out.print("\tLabel: ");
- String labelD = scan.nextLine();
- if(cylinderList.deleteCylinder(labelD) == null)
- System.out.println("\t\"" + labelD + "\" not found");
- else
- System.out.println("\t\"" + labelD +"\" deleted");
- break;
- case 'f':
- case 'F'://Find Cylinder
- System.out.print("\tLabel: ");
- String labelF = scan.nextLine();
- if(cylinderList.findCylinder(labelF) == null)
- System.out.println("\t\"" + labelF + "\" not found");
- else
- System.out.println(cylinderList.findCylinder(labelF));
- break;
- case 'q':
- case 'Q'://Quit
- return;
- default:
- System.out.println("\t*** invalid code ***");
- }
- }
- }
- }
运行演示:
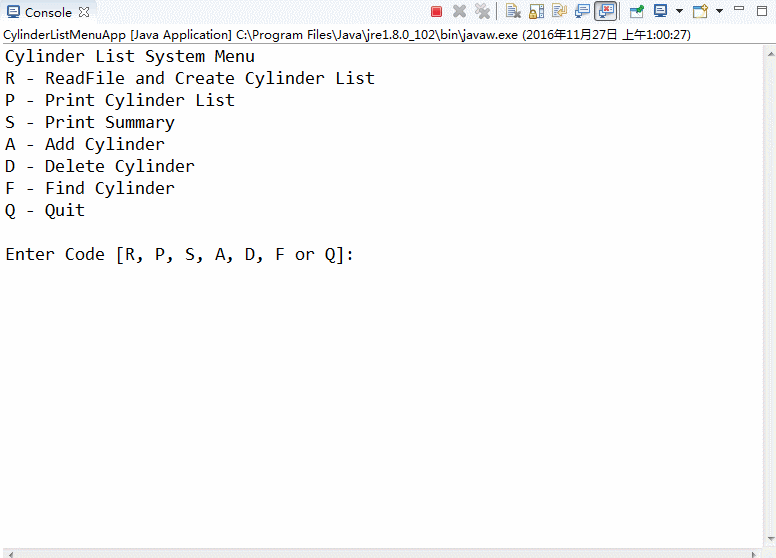
week10 继承特性的练习: 货物
-------------------------2016-12-04更新
-
谨记:重载增加了一个额外的方法,而覆盖取代了方法定义
-
静态方法不能使用隐含(或明确的把this作为其调用对象) 的实例变量(或非静态方法)
-
10.1 InventoryItem.java
- package week10;
-
- /**
- * 10.1 InventoryItem.java
- * 货物类:所有物品类的基类
- */
- public class InventoryItem
- {
- protected String name;
- protected double price;
- private static double taxRate = 0;
-
- public static void main(String[] args)
- {
- InventoryItem.setTaxRate(0.08);
- InventoryItem item1 = new InventoryItem("Birdseed", 7.99);
- InventoryItem item2 = new InventoryItem("Picture", 10.99);
-
- System.out.println(item1);
- System.out.println(item2);
- }
-
- /**
- * 初始化
- * @param name
- * @param price
- */
- public InventoryItem(String name, double price)
- {
- this.name = name;
- this.price = price;
- }
-
- @Override
- public String toString()
- {
- return name + ": $" + calculateCost();
- }
-
- /**
- * 货物含税的价格
- * @return
- */
- public double calculateCost()
- {
- return this.price * (1 + taxRate);
- }
-
- public String getName()
- {
- return name;
- }
-
- /**
- * 设置税率;
- * 静态方法不能使用隐含(或明确的把this作为其调用对象) 的实例变量(或非静态方法)
- * @param taxRateIn
- */
- public static void setTaxRate(double taxRateIn)
- {
- taxRate = taxRateIn;
- }
- }
运行结果:
10.2 ElectronicsItem.java
- package week10;
-
- /**
- * 10.2 ElectronicsItem.java
- * 电子货物类:InventoryItem的派生类
- */
- public class ElectronicsItem extends InventoryItem
- {
- protected double weight; //电子货物重量
- public static final double SHIPPING_COST = 1.5;//每磅的货运费用
-
- public ElectronicsItem(String name, double price, double weight)
- {
- super(name, price);
- this.weight = weight;
- }
-
- @Override
- public double calculateCost()
- {
- return super.calculateCost() + weight * SHIPPING_COST;
- }
-
- public static void main(String[] args)
- {
- InventoryItem.setTaxRate(0.08);
- ElectronicsItem eItem = new ElectronicsItem("Monitor", 100, 10.0);
- System.out.println(eItem);
- }
- }
-
运行结果:
10.3 OnlineTextItem.java
- package week10;
-
- /**
- * 10.3 OnlineTextItem.java
- * 在线文本商品类:InventoryItem的派生类
- * 该类代表用户可购买的在线文本商品(如电子书或者电子杂志);
- * 因为它只是概念级的、 代表物品的类,因此可以设置为抽象类;
- */
- public abstract class OnlineTextItem extends InventoryItem
- {
-
- public OnlineTextItem(String name, double price)
- {
- super(name, price);
- // TODO Auto-generated constructor stub
- }
-
- @Override
- public double calculateCost()
- {
- return price;
- }
-
- }
-
10.4 OnlineArticle.java
- package week10;
-
- /**
- * 10.4 OnlineArticle.java
- * 电子类文本物品:OnlineTextItem的派生类
- */
- public class OnlineArticle extends OnlineTextItem
- {
- private int wordCount; //记录字数
-
- public OnlineArticle(String name, double price)
- {
- super(name, price);
- this.wordCount = 0;
- }
-
- @Override
- public String toString()
- {
- return name + ": $"+ price
- + " " + this.wordCount;
- }
-
- public void setWordCount(int wordCount)
- {
- this.wordCount = wordCount;
- }
-
- }
-
10.5 OnlineBook.java
- package week10;
-
- /**
- * 10.5 OnlineBook.java
- * 电子书:OnlineTextItem的派生类
- */
- public class OnlineBook extends OnlineTextItem
- {
- protected String author; //电子书作者
-
- public OnlineBook(String name, double price)
- {
- super(name, price);
- author = "Author Not Listed";
- }
-
- public void setAuthor(String author)
- {
- this.author = author;
- }
-
- @Override
- public String toString()
- {
- return name + " - "
- + author +": $" + price;
- }
-
- public static void main(String[] args)
- {
- OnlineBook book = new OnlineBook("A Novel Novel", 9.99);
- System.out.println(book);
-
- book.setAuthor("Jane Lane");
- System.out.println(book);
- }
-
- }
-
运行结果:
10.6 InventoryApp.java
- package week10;
-
- /**
- * 10.6 InventoryApp.java
- * 测试类:
- * (1) 设置税率为 0.05
- * (2) 初始化并输出 4个对象(item1、item2、item3、item4)
- */
- public class InventoryApp
- {
- public static void main(String[] args)
- {
- InventoryItem.setTaxRate(0.05);
-
- InventoryItem item1 = new InventoryItem("pen", 25);
- ElectronicsItem item2 = new ElectronicsItem("apple phone", 1000, 1.8);
- OnlineArticle item3 = new OnlineArticle("Java", 8.5);
- OnlineBook item4 = new OnlineBook("Head first Java", 40);
-
- item3.setWordCount(700);
- item4.setAuthor("Kathy&Bert");
-
- System.out.println(item1);
- System.out.println(item2);
- System.out.println(item3);
- System.out.println(item4);
-
- System.out.println("All inventory:\n\n" + myItems);
- System.out.println("Total: " + myItems.calculateTotal(2));
- }
- }
-
运行结果:
week11 继承的多态特性:货物列表
-------------------------2016-12-05更新
任务:在week10的基础上完成该实验任务。生成2个新的类,并体会继承的多态特性。

11.7 ItemsList.java
- package week11;
-
- import week10.ElectronicsItem;
- import week10.InventoryItem;
-
- /**
- * 11.7 ItemsList.java
- * 存放InventoryItem对象的数组
- */
- public class ItemsList
- {
- private InventoryItem[] inventory;
- private int count;
-
- public ItemsList()
- {
- inventory = new InventoryItem[20];
- count = 0;
- }
-
- /**
- * 增加一个item
- * @param itemIn
- */
- public void addItem(InventoryItem itemIn)
- {
- this.inventory[count++] = itemIn;
- }
-
- /**
- * 返回代表数组各元素价格的总和
- * @param electronicsSurcharge,代表征收ElectronicsItem的附加费
- * @return
- */
- public double calculateTotal(double electronicsSurcharge)
- {
- double totalCost = 0;
-
- /** 需要遍历 inventory数组的每一个元素,将价格 (cost)累加到和上。
- * 如果元素为 ElectronicsItem类的引用变量 ,则激活calculateCost方法,
- * 增加该的 方法的electronicsSurcharge
- */
- for (int i = 0; i < count; i++)
- {
- if (inventory[i] instanceof ElectronicsItem)
- {
- totalCost += inventory[i].calculateCost() + electronicsSurcharge;
- }
- else
- {
- totalCost += inventory[i].calculateCost();
- }
-
- }
- return totalCost;
- }
-
- @Override
- public String toString()
- {
- String output = "";
- for (int i = 0; i < count; i++)
- {
- output += inventory[i].toString();
- output += "\n";
- }
- return output;
- }
- }
11.8 ItemsListApp.java
- package week11;
-
- import week10.ElectronicsItem;
- import week10.InventoryItem;
- import week10.OnlineArticle;
- import week10.OnlineBook;
-
- /**
- * 11.8 ItemsListApp.java 测试类
- */
- public class ItemListApp
- {
- public static void main(String[] args)
- {
- // a)初始化名为myItems的ItemsList对象
- ItemsList myItems = new ItemsList();
-
- // b)通过InventoryItem的setTaxRate方法设置税率为 0.05
- InventoryItem.setTaxRate(0.05);
-
- // c)初始化以下4个货物并将其增加到myItems中
- ElectronicsItem electItem = new ElectronicsItem("笔记本", 1234.56, 10);
- InventoryItem item = new InventoryItem("机油", 9.8);
- OnlineBook book = new OnlineBook("疯狂Java讲义", 12.3);
- book.setAuthor("李刚");
- OnlineArticle article = new OnlineArticle("如何学好Java", 3.4);
- article.setWordCount(700);
-
- myItems.addItem(electItem);
- myItems.addItem(item);
- myItems.addItem(book);
- myItems.addItem(article);
-
- System.out.println("All inventory:\n\n" + myItems);
-
- System.out.println("Total: " + myItems.calculateTotal(1.215));
- }
-
- }
运行结果:
week12 继承与多态:行程(一)
-------------------------2016-12-20更新
小变动:
-
参考13周的截图(修改)写的 toString 方法
-
参考13周的截图,我将类Business的静态常量AWARDMILESFACTOR初始化为2
重难点:
-
泛型接口 Comparable<T> 的使用
-
java.util.Arrays 中排序方法sort的使用
说 明:
题目所给文本(各类机票数据示例.txt)的票据信息我用表格形式展示如下:

12.1 旅程类:Itinerary
- package week12;
-
- /**
- * 12.1 旅程类
- */
- public class Itinerary
- {
- private String formAirport;
- private String toAirport;
- private String depDateTime;
- private String arrDateTime;
- private int miles;
-
- public Itinerary
- (String formAirport,String toAirport,String depDateTime,String arrDateTime,int miles)
- {
- this.formAirport = formAirport;
- this.toAirport = toAirport;
- this.depDateTime = depDateTime;
- this.arrDateTime = arrDateTime;
- this.miles = miles;
- }
-
- public String getDepDateTime()
- {
- return depDateTime;
- }
-
- public String getArrDateTime()
- {
- return arrDateTime;
- }
-
- public int getMiles()
- {
- return miles;
- }
-
- @Override
- public String toString()
- {
- String output = ""
- + "" + formAirport
- + "-" + toAirport
- + " (" + depDateTime
- + " - " + arrDateTime
- + ")"
- + " " + miles;
- return output;
- }
- }
12.2 飞机票(抽象)基类:AirTicket
- package week12;
-
- import java.text.DecimalFormat;
- import java.text.Format;
-
- /**
- * 12.2 飞机票(抽象)基类
- */
- public abstract class AirTicket implements Comparable<AirTicket>
- {
- private String flightNum; //航班号
- private Itinerary itinerary; //行程
- private double baseFare; //飞机票基本费用
- private double fareAdjustmentFactor; //费用调整因素
-
- public AirTicket(String flightNum,Itinerary itinerary,double baseFare,double fareAdjustmentFactor)
- {
- this.flightNum = flightNum;
- this.itinerary = itinerary;
- this.baseFare = baseFare;
- this.fareAdjustmentFactor = fareAdjustmentFactor;
- }
-
- public String getFlightNum()
- {
- return flightNum;
- }
-
- public Itinerary getItinerary()
- {
- return itinerary;
- }
-
- public double getBaseFare()
- {
- return baseFare;
- }
-
- public double getFareAdjustmentFactor()
- {
- return fareAdjustmentFactor;
- }
-
- /**
- * 自然比较方法:(忽略大小写)比较航班号
- * @return 该对象小于、等于或大于指定对象 at,分别返回负整数、零或正整数。
- */
- @Override
- public int compareTo(AirTicket at)
- {
- return flightNum.compareToIgnoreCase(at.flightNum);
- }
-
- @Override
- public String toString()
- {
- Format formater = new DecimalFormat("###,###.00");
- String output = ""
- + "\nFlight: " + flightNum
- + "\n" + itinerary
- + " (" + totalAwardMiles() + " award miles)"
- + "\nBase Fare: $" + formater.format(baseFare)
- + " Fare Adjustment Factor: " + fareAdjustmentFactor
- + "\nTotal Fare: $" + formater.format(totalFare())
- + "\t";
- return output;
- }
-
- public abstract double totalFare();
-
- public abstract double totalAwardMiles();
-
- }
12.3 飞机票子类---经济舱机票:Economy
- package week12;
-
- /**
- * 12.3 飞机票子类---经济舱机票
- */
- public class Economy extends AirTicket
- {
- private final static double AWARDMILESFACTOR = 1.5;
-
- public Economy(String flightNum, Itinerary itinerary, double baseFare, double fareAdjustmentFactor)
- {
- super(flightNum, itinerary, baseFare, fareAdjustmentFactor);
- }
-
- @Override
- public double totalFare()
- {
- return super.getBaseFare() * super.getFareAdjustmentFactor();
- }
-
- @Override
- public double totalAwardMiles()
- {
- return super.getItinerary().getMiles() * AWARDMILESFACTOR;
- }
-
- @Override
- public String toString()
- {
- return super.toString() + " (class Economy)\n "
- + "Includes Award Miles Factor: "
- + AWARDMILESFACTOR;
- }
- }
12.4 飞机票子类---商务舱机票:Business
- package week12;
-
- /**
- * 12.4 飞机票子类---商务舱机票
- */
- public class Business extends AirTicket
- {
- private final static double AWARDMILESFACTOR = 2; //这里我改为2(题目为1.5)
- private double foodAndBeverages;
- private double entertaiment;
-
- public Business(String flightNum, Itinerary itinerary, double baseFare, double fareAdjustmentFactor,
- double foodAndBeverages, double entertaiment)
- {
- super(flightNum, itinerary, baseFare, fareAdjustmentFactor);
- this.foodAndBeverages = foodAndBeverages;
- this.entertaiment = entertaiment;
- }
-
- @Override
- public double totalFare()
- {
- return super.getBaseFare() * super.getFareAdjustmentFactor()
- + foodAndBeverages + entertaiment;
- }
-
- @Override
- public double totalAwardMiles()
- {
- return super.getItinerary().getMiles() * AWARDMILESFACTOR;
- }
-
- @Override
- public String toString()
- {
- return super.toString()+ " (class Business)\n "
- + "Includes Food/Beverage: $" + foodAndBeverages
- + " Entertaiment: $" + entertaiment;
- }
-
- }
12.5 飞机票子类---不可退的机票: NonRefundable
- package week12;
-
- /**
- * 12.5 飞机票子类---不可退的机票
- */
- public class NonRefundable extends AirTicket
- {
- private double discountFactor;
-
- public NonRefundable(String flightNum, Itinerary itinerary, double baseFare, double fareAdjustmentFactor,
- double discountFactor)
- {
- super(flightNum, itinerary, baseFare, fareAdjustmentFactor);
- this.discountFactor = discountFactor;
- }
- @Override
- public double totalFare()
- {
- return super.getBaseFare() * super.getFareAdjustmentFactor()
- * discountFactor;
- }
-
- @Override
- public double totalAwardMiles()
- {
- return super.getItinerary().getMiles();
- }
-
- @Override
- public String toString()
- {
- return super.toString()+ " (class NonRefundable)\n "
- + "Includes DiscountFactor: "
- + discountFactor;
- }
- }
12.6 飞机票子类---商务舱机票子类---精英类机票: Elite
- package week12;
-
- /**
- * 12.6 飞机票子类---商务舱机票子类---精英类机票
- */
- public class Elite extends Business
- {
- private double cService;
-
- public Elite(String flightNum, Itinerary itinerary, double baseFare, double fareAdjustmentFactor,
- double foodAndBeverages, double entertaiment, double cService)
- {
- super(flightNum, itinerary, baseFare, fareAdjustmentFactor, foodAndBeverages, entertaiment);
- this.cService = cService;
- }
-
- @Override
- public double totalFare()
- {
- return super.totalFare() + cService;
- }
-
- @Override
- public double totalAwardMiles()
- {
- return super.totalAwardMiles();
- }
-
- @Override
- public String toString()
- {
- return super.toString()+ " \n "
- + "Includes: Comm Services: $" + cService;
- }
- }
12.7 测试类: AirTicketProcessor
- package week12;
-
- import java.util.Arrays;
-
- /**
- * 12.7 测试类
- */
- public class AirTicketProcessor
- {
- public static void main(String[] args)
- {
- AirTicket[] airTickets = new AirTicket[4]; //四张 飞机票
- Itinerary trip; // 临时行程对象
-
- // 初始化 题目给出的 四张票
- trip = new Itinerary("ATL", "LGA", "2015/05/01 1500", "2015/05/01 1740", 800);
- Economy economy = new Economy("DL 1867", trip, 450, 1);
- trip = new Itinerary("ATL", "LGA", "2015/05/01 1400", "2015/05/01 1640", 800);
- Business business = new Business("DL 1865", trip, 450, 2, 50, 50);
- trip = new Itinerary("ATL", "LGA", "2015/05/01 0900", "2015/05/01 1140", 800);
- Elite elite = new Elite("DL 1863", trip, 450, 2.5, 50, 50, 100);
- trip = new Itinerary("ATL", "LGA", "2015/05/01 0800", "2015/05/01 1040", 800);
- NonRefundable nonRefundable = new NonRefundable("DL 1861", trip, 450, 0.45, 0.9);
-
- // 将这4个对象添加到 airTickets
- airTickets[0] = (economy);
- airTickets[1] = (business);
- airTickets[2] = (elite);
- airTickets[3] = (nonRefundable);
-
- //输出报告
- System.out.println("----------Air Ticket Report-----------");
- for (int i = 0; i < airTickets.length; i++)
- System.out.println(airTickets[i]);
-
- Arrays.sort(airTickets); // 按航班号排序并输出报告
- System.out.println("\n----------Air Ticket Report (by Flight Number)-----------");
- for (int i = 0; i < airTickets.length; i++)
- System.out.println(airTickets[i]);
- }
- }
运行结果:

week13 继承与多态:行程(二)
-------------------------2016-12-20更新
小变动:
-
读文件并分割参数我并没有使用Scanner类
-
读文件的异常处理我没有放在 AirTicketApp 测试类中;(按照题意应在readAirTicketFile方法中throws异常,而不是直接try/catch)
重难点:
-
java.lang.Comparable<T> 接口: 强行对实现它的每个类的对象进行整体排序
-
java.util.Comparator<T> 接口: 强行对某个对象collection进行整体排序的比较函数
-
java.util.Arrays 中方法sort,copyOf的使用
说 明:
a. 有6个类我是直接使用week12的,下面是3个新写的类
b. 题目所给CSV文件(air_ticket_data.csv)我放在工程目录的resource下,如下所示:

- B,DL 1865,ATL,LGA,2015/05/01 1400,2015/05/01 1640,800,450,2.0,50.0,50.00
- E,DL 1867,ATL,LGA,2015/05/01 1500,2015/05/01 1740,800,450,1.0
- F,DL 1863,ATL,LGA,2015/05/01 0900,2015/05/01 1140,800,450,2.5,50.0,50.00,100.00
- N,DL 1861,ATL,LGA,2015/05/01 0800,2015/05/01 1040,800,450,0.45,0.90
- B,DL 1866,LGA,ATL,2015/05/01 1400,2015/05/01 1640,800,450,2.0,50.0,50.00
- E,DL 1868,LGA,ATL,2015/05/01 1500,2015/05/01 1740,800,450,1.0
- F,DL 1864,LGA,ATL,2015/05/01 0900,2015/05/01 1140,800,450,2.5,50.0,50.00,100.00
- N,DL 1862,LGA,ATL,2015/05/01 0800,2015/05/01 1040,800,450,0.45,0.90
-------------------------2016-12-23更新
13.8 功能类: AirTicketProcessor
- package week13;
-
- import java.io.BufferedReader;
- import java.io.File;
- import java.io.FileReader;
- import java.io.IOException;
- import java.util.Arrays;
-
- import week12.AirTicket;
- import week12.Business;
- import week12.Economy;
- import week12.Elite;
- import week12.Itinerary;
- import week12.NonRefundable;
-
- /**
- * 13.8 该类完成从数据文件中读取数据并声称报告的功能
- */
- public class AirTicketProcessor
- {
- private AirTicket[] Tickets;
- private String[] invalidRecords;
-
- public AirTicketProcessor()
- {
- Tickets = new AirTicket[0];
- invalidRecords = new String[0];
- }
-
- /**
- * 以行为单位读取文件,常用于读面向行的格式化文件 (注意:这个读取文件并分割参数的方法没有使用题目给出的方法)
- */
- public void readAirTicketFile(String fileName)
- {
- // 这里我设置为 工程 目录的 resource 下
- String path = "resource/" + fileName;
- File file = new File(path);
-
- BufferedReader reader = null;
- try
- {
- reader = new BufferedReader(new FileReader(file));
- String tempString = null; // 每行的字符串临时变量
- // 一次读入一行,直到读入null为文件结束
- while ((tempString = reader.readLine()) != null)
- {
- try
- {
- String[] lineArr = tempString.split(",");
- // 以 逗号 为分隔符, 并添加 票据信息
- switch (lineArr[0])
- {
- case "N":
- addAirTicket(new NonRefundable(lineArr[1],
- new Itinerary(lineArr[2], lineArr[3], lineArr[4], lineArr[5],
- Integer.parseInt(lineArr[6])),
- Integer.parseInt(lineArr[7]), Double.parseDouble(lineArr[8]),
- Double.parseDouble(lineArr[9])));
- break;
- case "E":
- addAirTicket(new Economy(lineArr[1],
- new Itinerary(lineArr[2], lineArr[3], lineArr[4], lineArr[5],
- Integer.parseInt(lineArr[6])),
- Double.parseDouble(lineArr[7]), Double.parseDouble(lineArr[8])));
- break;
- case "B":
- addAirTicket(new Business(lineArr[1],
- new Itinerary(lineArr[2], lineArr[3], lineArr[4], lineArr[5],
- Integer.parseInt(lineArr[6])),
- Double.parseDouble(lineArr[7]), Double.parseDouble(lineArr[8]),
- Double.parseDouble(lineArr[9]), Double.parseDouble(lineArr[10])));
- break;
- case "F":
- addAirTicket(new Elite(lineArr[1],
- new Itinerary(lineArr[2], lineArr[3], lineArr[4], lineArr[5],
- Integer.parseInt(lineArr[6])),
- Double.parseDouble(lineArr[7]), Double.parseDouble(lineArr[8]),
- Double.parseDouble(lineArr[9]), Double.parseDouble(lineArr[10]),
- Double.parseDouble(lineArr[11])));
- break;
- default:
- addInvaildRecord(tempString);
- break;
- }
- } catch (Exception e)
- {
- System.out.println("Line string split error!");
- }
- }
- } catch (IOException e)
- {
- System.out.println("Not find AirTicketFile!");
- // e.printStackTrace();
- } finally
- {
- if (reader != null)
- {
- try
- {
- reader.close();
- } catch (IOException e1)
- {
- }
- }
- }
- }
-
- /**
- * 将AirTicket类数组的长度增加1,之后将传入的对象放入数组中
- *
- * @param airTicket
- */
- public void addAirTicket(AirTicket airTicket)
- {
- Tickets = Arrays.copyOf(Tickets, Tickets.length + 1);
- Tickets[Tickets.length - 1] = (airTicket);
- }
-
- /**
- * 将invalidRecords数组的长度增加1,将传入的字符串放入数组中(每一行以代表机票种类的 字符开头(N, E, B和F是合法的机票种类),
- * 如果开头字母不在此范围中,则此行为不合法记录)
- *
- * @param lineStr
- */
- public void addInvaildRecord(String lineStr)
- {
- invalidRecords = Arrays.copyOf(invalidRecords, invalidRecords.length + 1);
- invalidRecords[invalidRecords.length - 1] = (lineStr);
- }
-
- /**
- * 返回 AirTickets报告
- */
- public String generateReport()
- {
- String output = "";
- for (AirTicket airTicket : Tickets)
- {
- output += airTicket + "\n";
- }
- return output;
- }
-
- /**
- * 以航班号的升序 返回AirTickets报告
- */
- public String generateReportByFlightNum()
- {
- String output = "";
-
- AirTicket[] orderT = Arrays.copyOf(Tickets, Tickets.length);
- Arrays.sort(orderT);
-
- for (AirTicket airTicket : orderT)
- {
- output += airTicket + "\n";
- }
- return output;
- }
-
- /**
- * 以行程的升序 返回AirTickets报告
- */
- public String generateReportByItinerary()
- {
- String output = "";
-
- AirTicket[] orderT = Arrays.copyOf(Tickets, Tickets.length);
- Arrays.sort(orderT, new ItineraryCompare());
-
- for (AirTicket airTicket : orderT)
- {
- output += airTicket + "\n";
- }
- return output;
- }
- }
13.9 自定义排序类: ItineraryCompare
- package week13;
-
- import java.util.Comparator;
-
- import week12.AirTicket;
-
- /**
- * 13.9 按照 Itinerary的tostring值由低到高排序
- */
- public class ItineraryCompare implements Comparator<AirTicket>
- {
- /**
- * @return 根据第一个参数小于、等于或大于第二个参数分别返回负整数、零或正整数。
- */
- @Override
- public int compare(AirTicket t1, AirTicket t2)
- {
- return t1.getItinerary().toString().compareTo(t2.getItinerary().toString());
- }
- }
13.10 测试类: AirTicketApp
- package week13;
-
- import java.util.Scanner;
-
- /**
- * 13.10 测试类
- */
- public class AirTicketApp
- {
- public static void main(String[] args)
- {
- // 1. 创建AirTicketProcessor对象
- AirTicketProcessor atp = new AirTicketProcessor();
-
- // 2. 判断命令行是否有参数(args.lengh的长度是否为0),
- // 如果没有,则输出“命令行中没有提供文件名,程序终止”
- Scanner scan = new Scanner(System.in);
-
- System.out.print("请输入文件名:"); // air_ticket_data.csv
- String fileName = scan.nextLine();
- scan.close();
- if (fileName.length() == 0)
- {
- System.out.println("命令行中没有提供文件名,程序终止");
- System.exit(0);
- }
-
- // 3.调用 AirTicketProcessor的方法读入数据文件,输出三个报告。
- atp.readAirTicketFile(fileName);
- System.out.println("----------Air Ticket Report-----------");
- System.out.println(atp.generateReport());
- System.out.println("----------Air Ticket Report (by Flight Number)-----------");
- System.out.println(atp.generateReportByFlightNum());
- System.out.println("----------Air Ticket Report (by Itinerary)-----------");
- System.out.println(atp.generateReportByItinerary());
-
- // 期间有如果没有找到文件,则抛出异常“文件没有找到,程序终止”
- // (我已经在AirTicketProcessor中捕获异常,故这里省略了。)
- // (如果按照题目意思,需要将readAirTicketFile方法中 抛出异常,在这里捕获即可。)
- }
- }
运行结果:
