1 控制栏
1.01 创建工具栏
/* 定义工具栏对象 */
CToolBar m_wndDemoToolBar;
//创建工具栏窗口
if (!m_wndDemoToolBar.CreateEx(this, TBSTYLE_FLAT, WS_CHILD |
WS_VISIBLE | CBRS_TOP | CBRS_GRIPPER | CBRS_TOOLTIPS |
CBRS_FLYBY | CBRS_SIZE_DYNAMIC))
{
return -1;
}
//加载工具栏资源
if (!m_wndDemoToolBar.LoadToolBar(IDR_TOOLBAR))
{
return -1;
}
//设置位图
m_Bitmap.LoadBitmap(IDB_TOOLBAR);
m_wndDemoToolBar.SetBitmap((HBITMAP)m_Bitmap);
//设置工具栏风格,工具栏可以停靠
m_wndDemoToolBar.EnableDocking(CBRS_ALIGN_ANY);
EnableDocking(CBRS_ALIGN_ANY);
DockBar(&m_wndToolBar, &m_wndDemoToolBar);
void CMainFrame::DockBar(CControlBar* pDestBar, CControlBar* pSrcBar)
{
RecalcLayout(TRUE);
//获得工具栏窗口坐标
CRect rect;
pDestBar->GetWindowRect(&rect);
rect.OffsetRect(1, 0);
//获得工具栏风格
DWORD dwStyle = pDestBar->GetBarStyle();
UINT nDockBarID = 0;
if (dwStyle & CBRS_ALIGN_TOP)
{
nDockBarID = AFX_IDW_DOCKBAR_TOP;
}
else if (dwStyle & CBRS_ALIGN_BOTTOM)
{
nDockBarID = AFX_IDW_DOCKBAR_BOTTOM;
}
else if (dwStyle & CBRS_ALIGN_LEFT)
{
nDockBarID = AFX_IDW_DOCKBAR_LEFT;
}
else if (dwStyle & CBRS_ALIGN_RIGHT)
{
nDockBarID = AFX_IDW_DOCKBAR_RIGHT;
}
//停靠工具栏
DockControlBar(pSrcBar, nDockBarID, &rect);
}
//设置工具栏扩展风格
m_wndToolBar.GetToolBarCtrl().SetExtendedStyle(TBSTYLE_EX_DRAWDDARROWS);
1.02 工具栏按钮添加文本按钮
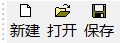
for (int n = 0; n < m_wndToolBar.GetCount(); n++)
{
//获得工具栏按钮ID
UINT nID = m_wndToolBar.GetItemID(n);
CString strText = _T("");
if (!strText.LoadString(nID))
{
continue;
}
int nIndex = strText.Find(_T('\n'));
if (nIndex < 0)
{
continue;
}
strText = strText.Right(strText.GetLength() - nIndex - 1);
//设置工具栏按钮文本
m_wndToolBar.SetButtonText(n, strText);
}
CRect rect;
m_wndToolBar.GetItemRect(0, &rect);
//设置工具栏按钮尺寸
m_wndToolBar.SetSizes(rect.Size(), CSize(16, 15));
1.03 工具栏按钮设置热点图像
//创建图像列表
if (!m_ImageList.Create(IDB_TOOLBAR, 16, 0, RGB(128, 128, 128)))
{
return -1;
}
//设置工具栏图像列表
m_wndToolBar.GetToolBarCtrl().SetHotImageList(&m_ImageList);
if (!m_wndStatusBar.Create(this) ||
!m_wndStatusBar.SetIndicators(indicators,
sizeof(indicators) / sizeof(UINT)))
{
TRACE0("Failed to create status bar\n");
return -1; // fail to create
}
1.04 目录栏禁止提示
void CMainFrame::OnEnableToolTips()
{
//获得工具栏风格
DWORD dwStyle = m_wndToolBar.GetBarStyle();
//启用或禁用工具提示
if (dwStyle & CBRS_TOOLTIPS)
{
dwStyle &= ~CBRS_TOOLTIPS;
}
else
{
dwStyle |= CBRS_TOOLTIPS;
}
//设置工具栏风格
m_wndToolBar.SetBarStyle(dwStyle);
}
ON_UPDATE_COMMAND_UI(ID_UNENABLE_MENU, OnUpdateEnableToolTips)
void CMainFrame::OnUpdateEnableToolTips(CCmdUI* pCmdUI)
{
//获得工具栏风格
DWORD dwStyle = m_wndToolBar.GetBarStyle();
if (dwStyle & CBRS_TOOLTIPS)
{
pCmdUI->SetCheck(TRUE);
}
else
{
pCmdUI->SetCheck(FALSE);
}
}
1.05 工具栏添加组合下拉框
CComboBox m_ComboBox;
//创建组合框
if (!CreateComboBox(ID_BUTTON))
{
TRACE0("Failed to create combo box\n");
return -1;
}
BOOL CMainFrame::CreateComboBox(UINT nID)
{
int nIndex = 0;
CRect rect;
//查找按钮
while (m_wndToolBar.GetItemID(nIndex) != nID)
{
nIndex++;
}
//为组合框创建一个空白区域
m_wndToolBar.SetButtonInfo(nIndex, nID, TBBS_SEPARATOR, 80);
//获得空白区域大小和位置
m_wndToolBar.GetItemRect(nIndex, &rect);
//组合框大小和位置
rect.top += 0;
rect.bottom += 200;
//在空白区域创建组合框
if (!m_ComboBox.Create(WS_CHILD | WS_VISIBLE | CBS_AUTOHSCROLL |
CBS_DROPDOWNLIST | CBS_HASSTRINGS, rect, &m_wndToolBar, nID))
{
TRACE0("Failed to create combo-box\n");
return FALSE;
}
//显示组合框
m_ComboBox.ShowWindow(SW_SHOW);
//在组合框中添加字符串
m_ComboBox.AddString(L"100%");
m_ComboBox.AddString(L"75%");
m_ComboBox.AddString(L"50%");
m_ComboBox.AddString(L"25%");
m_ComboBox.AddString(L"10%");
m_ComboBox.SetCurSel(3);
return TRUE;
}
ON_CBN_SELCHANGE(ID_BUTTON, OnSelchangeCombobox)
/* 下拉响应事件 */
void CMainFrame::OnSelchangeCombobox()
{
CString strText = _T("");
m_ComboBox.GetWindowText(strText);
AfxMessageBox(strText);
}
2 状态栏
2.01 创建状态栏窗口
//创建状态栏窗口
if (!m_wndDemoStatusBar.Create(this))
{
return -1;
}
//ID数组
UINT IDArray[2];
for (int n = 0; n < 2; n++)
{
IDArray[n] = 10000 + n;
}
//设置状态栏指示器
m_wndDemoStatusBar.SetIndicators(IDArray, sizeof(IDArray) / sizeof(UINT));
//设置窗格宽度
m_wndDemoStatusBar.SetPaneInfo(0, IDArray[0], SBPS_NORMAL, 100);
m_wndDemoStatusBar.SetPaneInfo(1, IDArray[1], SBPS_STRETCH, 0);
//设置窗格文本
m_wndDemoStatusBar.SetPaneText(0, _T("状态栏:"));
m_wndDemoStatusBar.SetPaneText(1, _T(""));
2.02 状态栏添加进度条
项目 =》添加类 =》MFC类 =》CProgressStatusBar public 继承 CStatusBar =》创建
实现OnCreate、OnSize函数
CProgressCtrl m_Progress;
int CProgressStatusBar::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CStatusBar::OnCreate(lpCreateStruct) == -1)
return -1;
//创建进度条
m_Progress.Create(WS_CHILD | WS_VISIBLE, CRect(0, 0, 0, 0), this, 1);
return 0;
}
void CProgressStatusBar::OnSize(UINT nType, int cx, int cy)
{
CStatusBar::OnSize(nType, cx, cy);
//获得指示器大小和位置
CRect rect;
GetItemRect(0, &rect);
//移动进度条窗口
m_Progress.MoveWindow(rect.left + 1, rect.top, rect.Width() - 2, rect.Height());
}
//创建状态栏窗口
if (!m_wndProgressStatusBar.Create(this))
{
return -1;
}
//ID数组
UINT IDArray[2];
for (int n = 0; n < 2; n++)
{
IDArray[n] = 10000 + n;
}
//设置状态栏指示器
m_wndProgressStatusBar.SetIndicators(IDArray, sizeof(IDArray) / sizeof(UINT));
//设置窗格宽度
m_wndProgressStatusBar.SetPaneInfo(0, IDArray[0], SBPS_NORMAL, 200);
m_wndProgressStatusBar.SetPaneInfo(1, IDArray[1], SBPS_STRETCH, 0);
//设置窗格文本
m_wndProgressStatusBar.SetPaneText(0, _T("自定义状态栏:"));
m_wndProgressStatusBar.SetPaneText(1, _T(""));
//设置进度条
m_wndProgressStatusBar.m_Progress.SetRange(0, 100);
m_wndProgressStatusBar.m_Progress.SetStep(10);
m_wndProgressStatusBar.m_Progress.SetPos(50);
2.02 禁用/起用状态栏
void CMainFrame::OnShowStatusBar()
{
if (m_wndStatusBar.GetStyle() & WS_VISIBLE)
{
//隐藏状态栏
ShowControlBar(&m_wndStatusBar, FALSE, FALSE);
}
else
{
//显示状态栏
ShowControlBar(&m_wndStatusBar, TRUE, FALSE);
}
}
void CMainFrame::OnUpdateShowStatusBar(CCmdUI* pCmdUI)
{
if (m_wndStatusBar.GetStyle() & WS_VISIBLE)
{
pCmdUI->SetCheck(TRUE);
}
else
{
pCmdUI->SetCheck(FALSE);
}
}
2.03 组合栏

//创建组合框
if (!m_ComboBox.Create(WS_CHILD | WS_VISIBLE | WS_VSCROLL |
CBS_DROPDOWN, CRect(0, 0, 100, 200), this, IDC_COMBOBOX))
{
return FALSE;
}
//创建按钮
m_Button.Create(_T("确定"), WS_CHILD | WS_VISIBLE | BS_PUSHBUTTON,
CRect(0, 0, 50, 20), this, IDC_TEST);
//创建Rebar
if (!m_wndReBar.Create(this))
{
return -1;
}
//添加工具栏、组合框、按钮添加到Rebar中
m_wndReBar.AddBar(&m_wndToolBar);
m_wndReBar.AddBar(&m_ComboBox, NULL, NULL, RBBS_NOGRIPPER | RBBS_BREAK);
m_wndReBar.AddBar(&m_Button, NULL, NULL, RBBS_NOGRIPPER);