基础控件
import QtQuick 2.0
import QtQuick.Controls 2.5
import QtGraphicalEffects 1.12
Button {
id: container
// 提供对外字段属性
property string bckcolor: "#1AAD19"
property string bckHoverColor: Qt.lighter(bckcolor, 0.8)
property int backRadius: 2
// 设置字体
font.family: "Microsoft Yahei"
font.pixelSize: 20
// implicitwidth:此属性表示控件的隐式推荐宽度,即在没有明确指定width时,控件希望占据的空间大小
implicitWidth: text.contentWidth + 24
implicitHeight: text.contentHeight + 8
// contentItem用于自定义控件并用文本替换视觉前景元素的现有实现。
contentItem: Text {
id: text
text: container.text
font: container.font
color: "#fff"
horizontalAlignment: Text.AlignHCenter
verticalAlignment: Text.AlignVCenter
}
// 选中、悬浮、按下背景变化
background: Rectangle {
anchors.fill: parent
radius: backRadius
color: container.down ? bckcolor :
container.hovered ? bckHoverColor : bckcolor
layer.enabled: true
layer.effect: DropShadow {
color: bckcolor
}
}
}
RowLayout {
spacing: 20
BaseButton {
text: "Blue"
Layout.preferredHeight: 28
Layout.preferredWidth: 78
font.pixelSize: 14
backRadius: 4
bckcolor: "#4785FF"
}
BaseButton {
bckcolor: "#EC3315"
Layout.preferredHeight: 28
Layout.preferredWidth: 78
font.pixelSize: 14
backRadius: 4
text: "Red"
}
BaseButton {
text: "Yellow"
Layout.preferredHeight: 28
Layout.preferredWidth: 78
font.pixelSize: 14
backRadius: 4
bckcolor: "#ED9709"
}
}

1.02 CheckBox
// 勾选框的属性和状态改变事件
CheckBox {
text: "勾选框"
tristate: true
checkState: allChildrenChecked ? Qt.Checked :
anyChildChecked ? Qt.PartiallyChecked : Qt.Unchecked
nextCheckState: function() {
if (checkState === Qt.Checked)
return Qt.Unchecked
else
return Qt.Checked
}
onCheckStateChanged: {
console.log(checkState)
}
}
// 指定为同一个组,只能有一个能选中
ButtonGroup{
id : btnGroup
exclusive: true
}
Column {
CheckBox {
checked: true
text: qsTr("First")
ButtonGroup.group: btnGroup
}
CheckBox {
ButtonGroup.group: btnGroup
text: qsTr("Second")
}
CheckBox {
ButtonGroup.group: btnGroup
text: qsTr("Third")
}
}
// 树形结构勾选组合
Column {
ButtonGroup {
id: childGroup
exclusive: false
checkState: parentBox.checkState
}
CheckBox {
id: parentBox
text: qsTr("Parent")
checkState: childGroup.checkState
}
CheckBox {
checked: true
text: qsTr("Child 1")
leftPadding: indicator.width
ButtonGroup.group: childGroup
}
CheckBox {
text: qsTr("Child 2")
leftPadding: indicator.width
ButtonGroup.group: childGroup
}
}
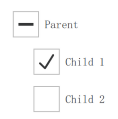
1.03 CheckBox
ComboBox{
width: 200
displayText: currentText
model: [//ListModel {
//id: model
"Banana" ,
"Apple" ,
"Coconut" //}
]
onAccepted: {
if (find(editText) === -1)
model.append({text: editText})
}
onCurrentIndexChanged: {
console.log(currentIndex)
}
onCurrentTextChanged: {
console.log(currentText)
}
}
ComboBox {
y: 200
textRole: "key"
model: ListModel {
ListElement { key: "First"; value: 123 }
ListElement { key: "Second"; value: 456 }
ListElement { key: "Third"; value: 789 }
}
}
ComboBox {
model: 10
editable: true
validator: RegExpValidator {
regExp:/[0-9A-Z]+[.][0-9]/
}
onAcceptableInputChanged: {
console.log(acceptableInput)
}
}