Disconnect Path in a Binary Matrix by at Most One Flip
Disconnect Path in a Binary Matrix by at Most One Flip
You are given a 0-indexed $m \times n$ binary matrix grid . You can move from a cell (row, col) to any of the cells (row + 1, col) or (row, col + 1) that has the value $1$. The matrix is disconnected if there is no path from (0, 0) to (m - 1, n - 1) .
You can flip the value of at most one (possibly none) cell. You cannot flip the cells (0, 0) and (m - 1, n - 1) .
Return true if it is possible to make the matrix disconnect or false otherwise.
Note that flipping a cell changes its value from $0$ to $1$ or from $1$ to $0$.
Example 1:
Input: grid = [[1,1,1],[1,0,0],[1,1,1]] Output: true Explanation: We can change the cell shown in the diagram above. There is no path from (0, 0) to (2, 2) in the resulting grid.
Example 2:
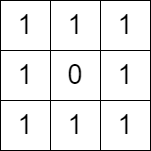
Input: grid = [[1,1,1],[1,0,1],[1,1,1]] Output: false Explanation: It is not possible to change at most one cell such that there is not path from (0, 0) to (2, 2).
Constraints:
- $m \ \mathrm{==} \ \text{grid.length}$
- $n \ \mathrm{==} \ \text{grid}[i]\text{.length}$
- $1 \leq m, n \leq 1000$
- $1 \leq m \times n \leq {10}^5$
- $\text{grid}[i][j]$ is either $0$ or $1$.
- $\text{grid}[0][0] \ \mathrm{==} \ \text{grid}[m - 1][n - 1] \ \mathrm{==} \ 1$
解题思路
考虑从$(0,0)$到$(n-1,m-1)$的所有路径所构成的轮廓。如下图(无法使得图不连通的情况),彩色代表该格子可以走,黑色的部分是轮廓。
如果通过修改一个格子使得图不连通,那么本质就是存在唯一一个格子使得除去这个格子后整个轮廓变得不连通。因此可以先dfs找出轮廓的其中一个部分。注意,在dfs的过程中要保证向下和向右搜索的顺序是不变的,例如每次先向下搜再向左搜,这样才能保证搜出的路径是轮廓的一部分。然后第一次dfs搜出的这条路径全部改成$0$(当然如果不存在路径那么图本身就不连通了),再dfs(一样是搜索轮廓,搜的是另外一部分轮廓)一次。如果第二次dfs依然存在$(0,0)$到$(n-1,m-1)$的路径,说明无法通过修改一个格子使得轮廓不连通(参考上图)。
AC代码如下:
class Solution { public: bool isPossibleToCutPath(vector<vector<int>>& grid) { int n = grid.size(), m = grid[0].size(); vector<vector<bool>> vis(n, vector<bool>(m)); function<bool(int, int)> dfs = [&](int x, int y) { if (x >= n || y >= m || !grid[x][y] || vis[x][y]) return false; if (x == n - 1 && y == m - 1) return true; vis[x][y] = true; grid[x][y] = 0; if (dfs(x + 1, y) || dfs(x, y + 1)) return true; grid[x][y] = 1; return false; }; if (!dfs(0, 0)) return true; grid[0][0] = grid[n - 1][m - 1] = 1; vis = vector<vector<bool>>(n, vector<bool>(m)); return !dfs(0, 0); } };
参考资料
c++ 30行超简单两次dfs:https://leetcode.cn/problems/disconnect-path-in-a-binary-matrix-by-at-most-one-flip/solution/c-32xing-chao-jian-dan-liang-ci-dfs-by-x-amcj/
本文来自博客园,作者:onlyblues,转载请注明原文链接:https://www.cnblogs.com/onlyblues/p/17093753.html