WPF 笔记 八 命令
好处:从不同 的UI实现同一个功能。
例子1:为按钮绑定系统预定义Command
<Window.CommandBindings> <CommandBinding Command="ApplicationCommands.New" Executed="CommandBinding_Executed" CanExecute="CommandBinding_CanExecute"/> </Window.CommandBindings> <StackPanel HorizontalAlignment="Center" VerticalAlignment="Center"> <Button Command="ApplicationCommands.New">New</Button> </StackPanel>
注意:上面代码的等效代码,在WIndow初始化时,
private void CreateNewCommandBinding() { //后台代码创建命令绑定:1)添加绑定到窗口 //创建绑定 CommandBinding binding = new CommandBinding(ApplicationCommands.New); //添加事件处理 binding.Executed += CommandBinding_Executed; binding.CanExecute += CommandBinding_CanExecute; //注册 this.CommandBindings.Add(binding); }
后台‘cs
private void CommandBinding_Executed(object sender, ExecutedRoutedEventArgs e) { MessageBox.Show("The New Cmd was Invoked"); } private void CommandBinding_CanExecute(object sender, CanExecuteRoutedEventArgs e) { e.CanExecute = true; }
e.CanExecute = false;按钮就无效了
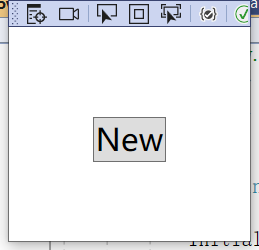
例子1.1 使用命令参数
在此处设置参数
<StackPanel HorizontalAlignment="Center" VerticalAlignment="Center"> <Button Command="ApplicationCommands.New" CommandParameter="NewPara" >New</Button> </StackPanel>
在这里获取
private void CommandBinding_Executed(object sender, ExecutedRoutedEventArgs e) { MessageBox.Show("The New Cmd was Invoked+\r\n+With Parameter :"+e.Parameter.ToString()); }
例子2:使用CanExecute,根据业务决定按钮是否可用
<Window.CommandBindings> <CommandBinding Command="ApplicationCommands.Cut" CanExecute="CutCommand_CanExecute" Executed="CutCommand_Executed" /> <CommandBinding Command="ApplicationCommands.Paste" CanExecute="PasteCommand_CanExecute" Executed="PasteCommand_Executed" /> </Window.CommandBindings> <DockPanel> <WrapPanel DockPanel.Dock="Top" Margin="3"> <Button Command="ApplicationCommands.Cut" Width="60">_Cut</Button> <Button Command="ApplicationCommands.Paste" Width="60" Margin="3,0">_Paste</Button> </WrapPanel> <TextBox AcceptsReturn="True" Name="txtEditor" /> </DockPanel>
private void CutCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e) { e.CanExecute = (txtEditor != null) && (txtEditor.SelectionLength > 0); } private void CutCommand_Executed(object sender, ExecutedRoutedEventArgs e) { txtEditor.Cut(); } private void PasteCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e) { e.CanExecute = Clipboard.ContainsText(); } private void PasteCommand_Executed(object sender, ExecutedRoutedEventArgs e) { txtEditor.Paste(); }
例子3:CommandTarget
实现和例子2 相同的功能
<DockPanel> <WrapPanel DockPanel.Dock="Top" Margin="3"> <Button Command="ApplicationCommands.Cut" CommandTarget="{Binding ElementName=txtEditor}" Width="60">_Cut</Button> <Button Command="ApplicationCommands.Paste" CommandTarget="{Binding ElementName=txtEditor}" Width="60" Margin="3,0">_Paste</Button> </WrapPanel> <TextBox AcceptsReturn="True" Name="txtEditor" /> </DockPanel>
例子4:自定义Command
xmlns:self="clr-namespace:WpfApp2" <Window.CommandBindings> <CommandBinding Command="self:CustomCommands.Exit" CanExecute="ExitCommand_CanExecute" Executed="ExitCommand_Executed" /> </Window.CommandBindings> <Grid> <Grid.RowDefinitions> <RowDefinition Height="Auto" /> <RowDefinition Height="*" /> </Grid.RowDefinitions> <Menu> <MenuItem Header="File"> <MenuItem Command="self:CustomCommands.Exit" /> </MenuItem> </Menu> <StackPanel Grid.Row="1" HorizontalAlignment="Center" VerticalAlignment="Center"> <Button Command="self:CustomCommands.Exit">Exit</Button> </StackPanel> </Grid>
后台
namespace WpfApp2 { /// <summary> /// MainWindow.xaml 的交互逻辑 /// </summary> public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } private void ExitCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e) { e.CanExecute = true; } private void ExitCommand_Executed(object sender, ExecutedRoutedEventArgs e) { Application.Current.Shutdown(); } } public static class CustomCommands { public static readonly RoutedUICommand Exit = new RoutedUICommand ( "Exit", "Exit", typeof(CustomCommands), new InputGestureCollection() { new KeyGesture(Key.F3, ModifierKeys.Alt) } ); } }
点击菜单,或者按钮,或者alt + F3都会关闭窗口。
例子5 :使用接口ICommand
接口方法的参数都是命令的参数
void Execute(object parameter);
internal class CommandBase : ICommand { public event EventHandler CanExecuteChanged; public bool CanExecute(object parameter) { return DoCanExecute?.Invoke(parameter) ==true; } public void Execute(object parameter) { DoAction?.Invoke(parameter); } public Action<object> DoAction { get; set; } public Func<object,bool> DoCanExecute { get;set; } public void RaiseCanChanged() { CanExecuteChanged?.Invoke(this,new EventArgs()); } } public class TestViewModel : INotifyPropertyChanged { public event PropertyChangedEventHandler PropertyChanged; private ICommand _command; public ICommand TestCommand { get { if( _command == null ) { _command = new CommandBase() { DoAction = (o) => { MessageBox.Show(o.ToString()); }, DoCanExecute = (o) => { return true; } }; } return _command; } set { _command = value; } } }
在窗口后台文件中
public MainWindow() { InitializeComponent(); this.DataContext = new TestViewModel(); }
<StackPanel HorizontalAlignment="Center" VerticalAlignment="Center"> <Button Command="{Binding TestCommand}" CommandParameter="hidaf" >New</Button> </StackPanel>
等待
TODO
https://blog.csdn.net/hetoby/article/details/77449762
https://www.cnblogs.com/fly-bird/p/8724915.html
https://www.cnblogs.com/gaixiaojie/archive/2010/09/01/1815015.html