Jav程序执行Linux命令
方法一:
本地执行,也是是代码必须放在Lunix服务器上才能通过java代码执行Linux命令。
package com.xdja.dsc.common.util; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.ArrayList; import java.util.List; import org.slf4j.Logger; import org.slf4j.LoggerFactory; /** * * @ClassName: ExecuteLinuxCommandUtils * @Description: 执行Linux命令工具类 * @author niugang * @date 2018年8月30日 */ public class ExecuteLinuxCommandUtils { private static Logger logger = LoggerFactory.getLogger(ExecuteLinuxCommandUtils.class); /** * 执行命令如:<br> * cd /usr/local/openresty/nginx/conf/ngx_conf/ && tar czf /tmp/ngx.tar.gz * <br> * cp /usr/local/openresty/nginx/conf/nginx.conf /tmp <br> * @param command:执行命令 * @return * @author niugang * @date 2018年8月30日 * @throws NullPointerException */ public static String exc(String command) { String result = null; Process ps = null; BufferedReader br = null; try { if (command == null || "".equals(command)) { throw new NullPointerException("command is not null"); } List<String> comList = new ArrayList<String>(3); comList.add("/bin/sh"); comList.add("-c"); comList.add(command); logger.info("execute command is:{}", comList.toString()); String[] commandArray = comList.toArray(new String[comList.size()]); ps = Runtime.getRuntime().exec(commandArray); ps.waitFor(); br = new BufferedReader(new InputStreamReader(ps.getInputStream())); StringBuffer sb = new StringBuffer(); String line; while ((line = br.readLine()) != null) { sb.append(line).append("\n"); } result = sb.toString(); br.close(); logger.info("execute linux command result:{}", result); } catch (Exception ex) { logger.error("execute linux command error:{}", ex); } finally { if (br != null) { try { br.close(); } catch (IOException e) { e.printStackTrace(); } } } return result; } }
方法二:远程连接
pom.xml
<!--操作ssh --> <dependency> <groupId>com.jcraft</groupId> <artifactId>jsch</artifactId> <version>0.1.50</version> </dependency>
代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 | package com.xdja.dsc; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.io.OutputStream; import com.jcraft.jsch.ChannelShell; import com.jcraft.jsch.JSch; import com.jcraft.jsch.JSchException; import com.jcraft.jsch.Session; /** * * @ClassName: TestSsh * @Description: ssh测试 * @author niugang * @date 2018年8月28日 */ public class TestSsh { /** * 远程执行JSch * @param args * @throws JSchException * @throws IOException * @author niugang * @date 2018年8月30日 * @throws */ public static void main(String[] args) throws JSchException, IOException { JSch jsch = new JSch(); Session session = jsch.getSession( "root" , "11.12.115.206" , 22 ); session.setPassword( "123456" ); session.setConfig( "StrictHostKeyChecking" , "no" ); session.setConfig( "userauth.gssapi-with-mic" , "no" ); session.connect(); ChannelShell channel = (ChannelShell) session.openChannel( "shell" ); channel.connect(); InputStream inputStream = channel.getInputStream(); OutputStream outputStream = channel.getOutputStream(); String cmd2 = "cd /home/xdja \n\r" ; outputStream.write(cmd2.getBytes()); String cmd = "ll \n\r" ; outputStream.write(cmd.getBytes()); outputStream.flush(); BufferedReader in = new BufferedReader( new InputStreamReader(inputStream)); String msg = null ; while ((msg = in.readLine()) != null ) { String encoding = getEncoding(msg); System.out.println( new String(msg.getBytes(encoding), encoding)); } in.close(); } public static String getEncoding(String str) { String encode = "GB2312" ; try { if (str.equals( new String(str.getBytes(encode), encode))) { // 判断是不是GB2312 String s = encode; return s; // 是的话,返回“GB2312“,以下代码同理 } encode = "ISO-8859-1" ; if (str.equals( new String(str.getBytes(encode), encode))) { // 判断是不是ISO-8859-1 String s1 = encode; return s1; } encode = "UTF-8" ; if (str.equals( new String(str.getBytes(encode), encode))) { // 判断是不是UTF-8 String s2 = encode; return s2; } encode = "GBK" ; if (str.equals( new String(str.getBytes(encode), encode))) { // 判断是不是GBK String s3 = encode; return s3; } } catch (Exception exception3) { } return "" ; } // 如果都不是,说明输入的内容不属于常见的编码 } |
微信公众号
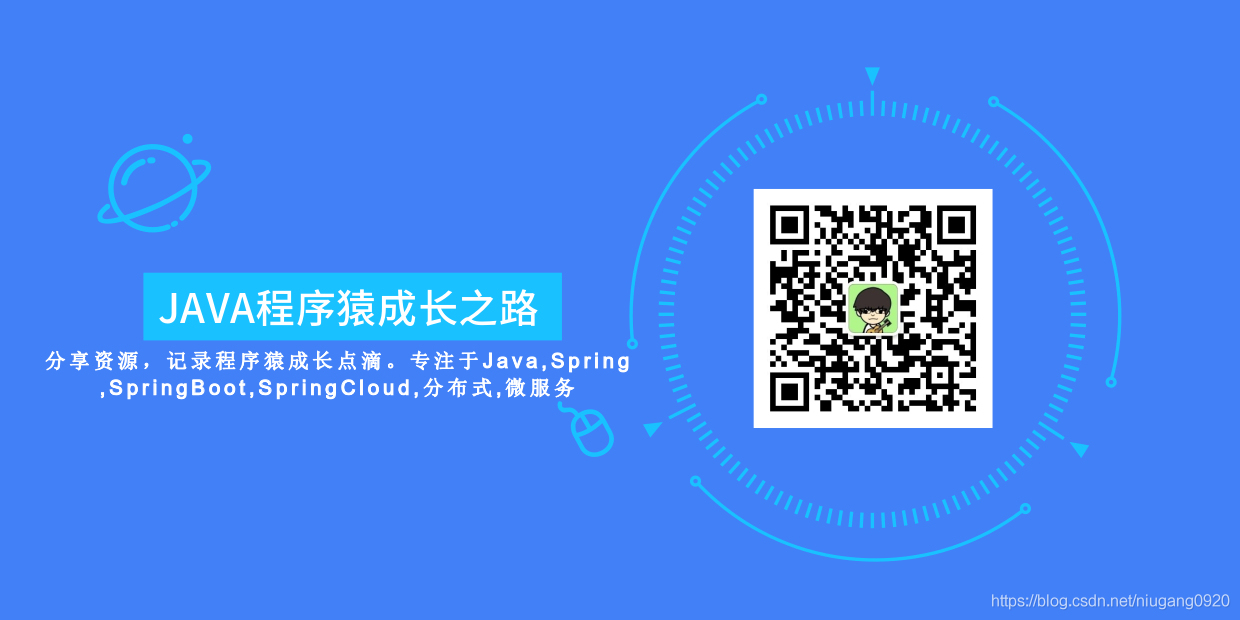
【推荐】编程新体验,更懂你的AI,立即体验豆包MarsCode编程助手
【推荐】凌霞软件回馈社区,博客园 & 1Panel & Halo 联合会员上线
【推荐】抖音旗下AI助手豆包,你的智能百科全书,全免费不限次数
【推荐】轻量又高性能的 SSH 工具 IShell:AI 加持,快人一步
· 智能桌面机器人:用.NET IoT库控制舵机并多方法播放表情
· Linux glibc自带哈希表的用例及性能测试
· 深入理解 Mybatis 分库分表执行原理
· 如何打造一个高并发系统?
· .NET Core GC压缩(compact_phase)底层原理浅谈
· 手把手教你在本地部署DeepSeek R1,搭建web-ui ,建议收藏!
· 新年开篇:在本地部署DeepSeek大模型实现联网增强的AI应用
· Janus Pro:DeepSeek 开源革新,多模态 AI 的未来
· 互联网不景气了那就玩玩嵌入式吧,用纯.NET开发并制作一个智能桌面机器人(三):用.NET IoT库
· 【非技术】说说2024年我都干了些啥