HDU 1016 - Prime Ring Problem
Prime Ring Problem
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 37484 Accepted Submission(s): 16554
Problem Description
A ring is compose of n circles as shown in diagram. Put natural number 1, 2, ..., n into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
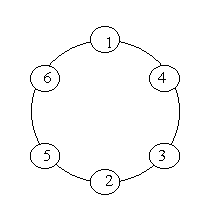
Note: the number of first circle should always be 1.
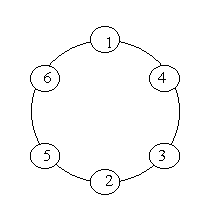
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements. Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6
8
Sample Output
Case 1: 1 4 3 2 5 6 1 6 5 2 3 4 Case 2: 1 2 3 8 5 6 7 4 1 2 5 8 3 4 7 6 1 4 7 6 5 8 3 2 1 6 7 4 3 8 5 2
解题思路:
简单的DFS即可
1 #include <iostream> 2 #include <cmath> 3 #include <string.h> 4 int n; 5 int ans[20],used[20]; 6 using namespace std; 7 bool isprime(int m) 8 { 9 for(int i=2;i<=sqrt(m*1.0);i++) 10 { 11 if(m%i==0) return 0; 12 } 13 return 1; 14 } 15 void dfs(int x) 16 { 17 if(x==n+1 && isprime(ans[1]+ans[n])) 18 { 19 for(int i=1;i<n;i++) 20 { 21 cout<<ans[i]<<' '; 22 } 23 cout<<ans[n]<<endl; 24 } 25 else 26 { 27 for(int j=2;j<=n;j++) 28 { 29 if(used[j]==0 && isprime(j+ans[x-1])) 30 { 31 ans[x]=j; 32 used[j]=1; 33 dfs(x+1); 34 used[j]=0; 35 } 36 } 37 } 38 } 39 int main() 40 { 41 int k=1; 42 while(cin>>n) 43 { 44 memset(used,0,sizeof(used)); 45 printf("Case %d:\n",k); 46 k++; 47 ans[1]=1; 48 used[1]=1; 49 dfs(2); 50 cout<<endl; 51 } 52 }
我自倾杯,君且随意