POCO C++库学习和分析 -- 内存管理 (三)
POCO C++库学习和分析 -- 内存管理 (三)
看完Poco库中的智能指针,基本上Poco中的内存管理已经快结束了。其他的部分都是些边边角角的东西,非常的简单。下面一一介绍。
4. AutoReleasePool
AutoReleasePool类的出现也同样是为了解决用户动态分配对象的释放问题,但同智能指针AutoPtr和SharedPtr通过把堆上的对象包装成栈对象,再通过引用计数在类的析构函数中实现自动删除对象的解决方案不同是,其策略为构造一个容器,用来存储动态对象的指针,在AutoReleasePool析构函数中统一释放。
这个过程和java语言中的垃圾收集机制是类似的,只不过AutoReleasePool实现的非常简单,在AutoReleasePool销毁时释放资源,而在java语言中会连续不断的定时检查并释放闲置资源。当然为了实现这个过程,AutoReleasePool对所释放的类是有要求的,释放的类必须实现release()接口。下面通过一个例子来说明问题:
#include "Poco/AutoReleasePool.h" using Poco::AutoReleasePool; class C { public: C() {} void release() { delete this; } }; int main(int argc, char** argv) { AutoReleasePool<C> pool; C* pC = new C; pool.add(pC); pC = new C; pool.add(pC); return 0; } // all C's deleted
其类图如下:
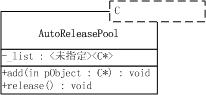
在图中可以看出,AutoReleasePool实际上就是原生指针的一个容器,在其内部定义为:
std::list<C*> ObjectList _list
AutoReleasePool<C> arp; AutoPtr<C> ptr = new C; ... arp.add(ptr.duplicate());很明显此刻AutoReleasePool和AutoPtr对对象应该共享所有权。
5. 动态工厂模板(DynamicFactory Class Template)
Poco中实现了一个动态工厂的模板,支持通过类名来创建类。其实现技术和前面的文章"Foundation库SharedLibrary模块分析"中介绍的类似。
动态工厂类DynamicFactory是抽象工厂类AbstractFactory的容器。
template <class Base> class DynamicFactory /// A factory that creates objects by class name. { .... std::map<std::string, AbstractFactory*> FactoryMap _map; }
AbstractFactory其实是模板类AbstractInstantiator<Base>的代称,是典型的工厂模式,用来实现具体类的创建,每个类工厂AbstractFactory对应一个类的创建。
template <class Base> class DynamicFactory /// A factory that creates objects by class name. { public: typedef AbstractInstantiator<Base> AbstractFactory; .... }
而一个动态工厂DynamicFactory则可负责从一个基类继承的一组继承类的创建工作。如果有多个基类,用户必须创建多个动态工厂DynamicFactory去实现类的创建。
同样为了完成通过类名来创建类的工作,DynamicFactory必须对类工厂进行注册,以便使编译器在编译时,建立类名和类的对应关系。
void registerClass(const std::string& className) /// Registers the instantiator for the given class with the DynamicFactory. /// The DynamicFactory takes ownership of the instantiator and deletes /// it when it's no longer used. /// If the class has already been registered, an ExistsException is thrown /// and the instantiator is deleted. { registerClass(className, new Instantiator<C, Base>); } void registerClass(const std::string& className, AbstractFactory* pAbstractFactory) /// Registers the instantiator for the given class with the DynamicFactory. /// The DynamicFactory takes ownership of the instantiator and deletes /// it when it's no longer used. /// If the class has already been registered, an ExistsException is thrown /// and the instantiator is deleted. { poco_check_ptr (pAbstractFactory); FastMutex::ScopedLock lock(_mutex); std::auto_ptr<AbstractFactory> ptr(pAbstractFactory); typename FactoryMap::iterator it = _map.find(className); if (it == _map.end()) _map[className] = ptr.release(); else throw ExistsException(className); }
接下来再来看DynamicFactory类中的创建工作:
template <class Base> class DynamicFactory /// A factory that creates objects by class name. { Base* createInstance(const std::string& className) const /// Creates a new instance of the class with the given name. /// The class must have been registered with registerClass. /// If the class name is unknown, a NotFoundException is thrown. { FastMutex::ScopedLock lock(_mutex); typename std::map<std::string, AbstractFactory*>::const_iterator it = _map.find(className); if (it != _map.end()) return it->second->createInstance(); else throw NotFoundException(className); } }DynamicFactory类在找个合适的类工厂后,就把任务交给了类AbstractFactory去完成。
下面是使用动态类工厂的一个例子:
#include "Poco/DynamicFactory.h" #include "Poco/SharedPtr.h" using Poco::DynamicFactory; using Poco::SharedPtr; class Base { }; class A: public Base { }; class B: public Base { }; int main(int argc, char** argv) { DynamicFactory<Base> factory; factory.registerClass<A>("A"); // creates Instantiator<A, Base> factory.registerClass<B>("B"); // creates Instantiator<B, Base> SharedPtr<Base> pA = factory.createInstance("A"); SharedPtr<Base> pB = factory.createInstance("B"); // you can unregister classes factory.unregisterClass("B"); // you can also check for the existence of a class bool haveA = factory.isClass("A"); // true bool haveB = factory.isClass("B"); // false (unregistered) bool haveC = factory.isClass("C"); // false (never registered) return 0; }
由于在Poco中用Poco::Instantiator类创建对象时使用的是类对象的默认构造函数,所以对于类创建时期望不使用默认构造函数或者对构造函数有一些特殊初始化过程要求的情况,用户必须自己实现抽象构造工厂。下面是其一个例子:
#include "Poco/DynamicFactory.h" using Poco::DynamicFactory; using Poco::AbstractInstantiator; class Base { }; class A: public Base { }; class C: public Base { public: C(int i): _i(i) {} private: int _i; }; class CInstantiator: public AbstractInstantiator<Base> { public: CInstantiator(int i): _i(i) {} Base* createInstance() const { return new C(_i); } private: int _i; }; int main(int argc, char** argv) { DynamicFactory<Base> factory; factory.registerClass<A>("A"); factory.registerClass("C", new CInstantiator(42)); return 0; }
最后给出 AbstractFactory模块的类图:
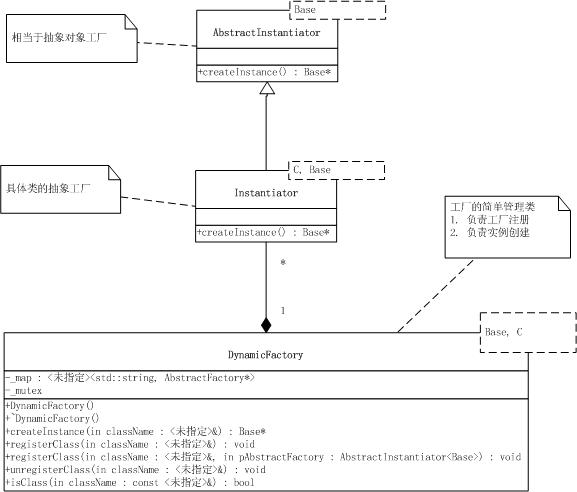
6. 内存池(Memory Pools)
同以往看过的内存池比较,Poco中内存池相当简单。既不支持对象的分配,也不对内存块大小进行分级,并且释放后的内存的合并策略也很简单。但这绝不是说它简陋,对于大多数情况,我觉得其完全够用了。同AutoReleasePool比较,两者的不同之处在于,AutoReleasePool中内存的分配是交由用户进行的,AutoReleasePool只负责释放,而MemoryPool的思想是,内存的分配和释放都由其管理。 首先来回顾一下内存池的作用:
1. 解决应用程序频繁申请和释放内存带来的执行效率问题
2. 解决内存碎片问题
下面是Poco中内存池函数调用的一些特性:
1. Poco::MemoryPool使用std::vector维护了一组固定大小的内存块指针,每个内存块大小都相等
2. 可以通过MemoryPool::get()获得一个内存块的指针,如果池中内存块不够时,一个新的内存块会被分配。但当池中内存块数目到达池定义的上限时,一个OutOfMemoryException异常会被抛出。
3. 调用MemoryPool::release(void* ptr)将把内存块释放入池中
其头文件中的定义如下:
class Foundation_API MemoryPool /// A simple pool for fixed-size memory blocks. /// /// The main purpose of this class is to speed-up /// memory allocations, as well as to reduce memory /// fragmentation in situations where the same blocks /// are allocated all over again, such as in server /// applications. /// /// All allocated blocks are retained for future use. /// A limit on the number of blocks can be specified. /// Blocks can be preallocated. { public: MemoryPool(std::size_t blockSize, int preAlloc = 0, int maxAlloc = 0); /// Creates a MemoryPool for blocks with the given blockSize. /// The number of blocks given in preAlloc are preallocated. ~MemoryPool(); void* get(); /// Returns a memory block. If there are no more blocks /// in the pool, a new block will be allocated. /// /// If maxAlloc blocks are already allocated, an /// OutOfMemoryException is thrown. void release(void* ptr); /// Releases a memory block and returns it to the pool. std::size_t blockSize() const; /// Returns the block size. int allocated() const; /// Returns the number of allocated blocks. int available() const; /// Returns the number of available blocks in the pool. private: MemoryPool(); MemoryPool(const MemoryPool&); MemoryPool& operator = (const MemoryPool&); enum { BLOCK_RESERVE = 128 }; typedef std::vector<char*> BlockVec; std::size_t _blockSize; int _maxAlloc; int _allocated; BlockVec _blocks; FastMutex _mutex; };
其中_maxAlloc是内存池可分配的最大内存块数,_blockSize是每个内存块的大小。
下面是内存池的一个例子:
#include "Poco/MemoryPool.h" #include <string> #include <iostream> using Poco::MemoryPool; int main(int argc, char** argv) { MemoryPool pool(1024); // unlimited number of 1024 byte blocks // MemoryPool pool(1024, 4, 16); // at most 16 blocks; 4 preallocated char* buffer = reinterpret_cast<char*>(pool.get()); std::cin.read(buffer, pool.blockSize()); std::streamsize n = std::cin.gcount(); std::string s(buffer, n); pool.release(buffer); std::cout << s << std::endl; return 0; }
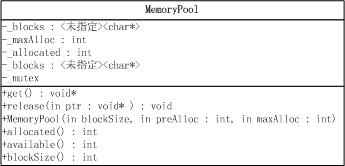
7. 单件(Singletons)
Poco中的单件可以由类模板SingletonHolder完成。其定义如下:
template <class S> class SingletonHolder /// This is a helper template class for managing /// singleton objects allocated on the heap. /// The class ensures proper deletion (including /// calling of the destructor) of singleton objects /// when the application that created them terminates. { public: SingletonHolder(): _pS(0) /// Creates the SingletonHolder. { } ~SingletonHolder() /// Destroys the SingletonHolder and the singleton /// object that it holds. { delete _pS; } S* get() /// Returns a pointer to the singleton object /// hold by the SingletonHolder. The first call /// to get will create the singleton. { FastMutex::ScopedLock lock(_m); if (!_pS) _pS = new S; return _pS; } private: S* _pS; FastMutex _m; };一眼可以望穿的代码,实在没有什么可以说的。噢,补充一下。在Poco中的Singleton模型里并没有使用DCLP(Double Checked Locking)模式。什么是DCLP。可以参考文章Double Checked Locking 模式。
其类图如下:
