using System; using System.Collections.Generic; using System.ComponentModel; using System.Drawing; using System.Threading; using System.Windows.Forms; using System.Xml; namespace UseBackgroundWorker { public partial class Form1 : Form { private XmlDocument document = null; public Form1() { InitializeComponent(); } private void dowloadButton_Click(object sender, EventArgs e) { this.backgroundWorker1.RunWorkerAsync(); // Disable the button for the duration of the download. this.dowloadButton.Enabled = false; // Wait for the BackgroundWorker to finish the download. while (this.backgroundWorker1.IsBusy) { // Keep UI messages moving, so the form remains // responsive during the asynchronous operation. Application.DoEvents(); } // The download is done, so enable the button. this.dowloadButton.Enabled = true; } private void backgroundWorker1_DoWork(object sender, DoWorkEventArgs e) { document = new XmlDocument(); // Replace this file name with a valid file name. document.Load(@"http://www.tailspintoys.com/sample.xml"); // Uncomment the following line to // simulate a noticeable latency. //Thread.Sleep(5000); } private void backgroundWorker1_RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e) { if (e.Error == null) { MessageBox.Show(document.InnerXml, "Download Complete"); } else { MessageBox.Show( "Failed to download file", "Download failed", MessageBoxButtons.OK, MessageBoxIcon.Error); } } } }
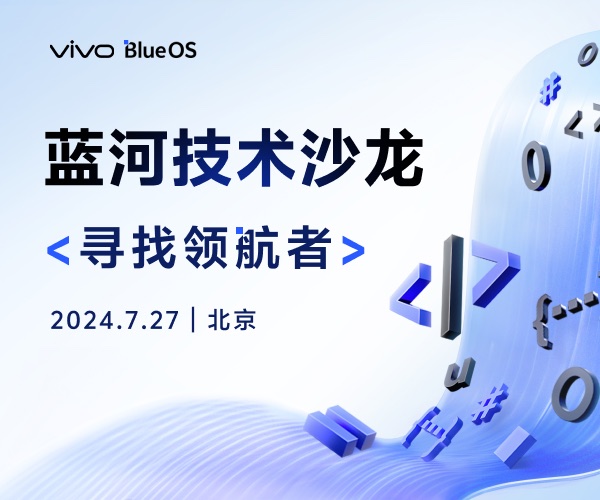