略读-策略模式
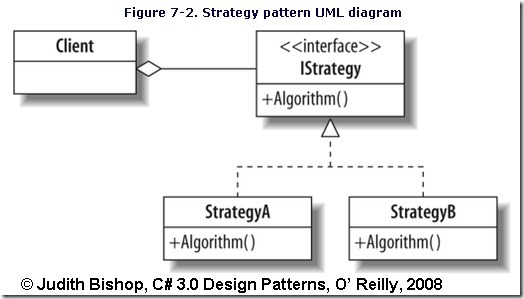
使用思路:需要动态修改类中的常变的部分
例子
简单的策略模式简单代码:
using System;
// Strategy Pattern by Judith Bishop Oct 2007
// Shows two strategies and a random switch between them
// The Context
class Context {
// Context state
public const int start = 5;
public int Counter = 5;
// Strategy aggregation
IStrategy strategy = new Strategy1();
// Algorithm invokes a strategy method
public int Algorithm() {
return strategy.Move(this);
}
// Changing strategies
public void SwitchStrategy() {
if (strategy is Strategy1)
strategy = new Strategy2();
else
strategy = new Strategy1();
}
}
// Strategy interface
interface IStrategy {
int Move (Context c);
}
// Strategy 1
class Strategy1 : IStrategy {
public int Move (Context c) {
return ++c.Counter;
}
}
// Strategy 2
class Strategy2 : IStrategy {
public int Move (Context c) {
return --c.Counter ;
}
}
// Client
static class Program {
static void Main () {
Context context = new Context();
context.SwitchStrategy();
Random r = new Random(37);
for (int i=Context.start; i<=Context.start+15; i++) {
if (r.Next(3) == 2) {
Console.Write("|| ");
context.SwitchStrategy();
}
Console.Write(context.Algorithm() +" ");
}
Console.WriteLine();
Console.ReadKey();
}
}
/* Output
4 || 5 6 7 || 6 || 7 8 9 10 || 9 8 7 6 || 7 || 6 5
*/
第一次理解此模式:
===============0816======================
一本设计模式书实在不够,所以在《C#3.0设计模式》基础上又借了《Head First设计模式》《大话设计模式》
目前的理解是:
上面的简单代码的亮点在(0817 删除让人误解的代码)
class Context 默认已经初始化了一个IStrategy,而根据需求变化对IStrategy加入了可变开关。这样就是策略模式的核心。
===============0817======================
之前说的亮点没讲清楚,比较容易让人误解。现在以客户端调用来表现该模式亮点
Context ct = new Context();
ct.Algorithm();
SwitchStrategy();
ct.Algorithm();
两次Algorithm结果不同。因为改变了策略。