Python Web-第六周-JSON and the REST Architecture(Using Python to Access Web Data)
1.JavaScript Object Notation JSON
1.JSON 官方介绍 http://www.json.org/json-zh.html
2.json1.py
import json data=''' { "name":"Chuck", "phone": { "type":"intl", "number":"+1231231234" }, "email": { "hide":"yes" } }''' info =json.loads(data) print'Name:',info["name"] print'Hide:',info["email"]["hide"]
JSON represents data as nested “lists”and “dictionaries”
JOSN数据表现为嵌套的“列表”和“字典”
而XML数据表现为树
3.josn2.py
import json input=''' [ { "id":"001", "x":"2", "name":"dch" }, { "id":"007", "x":"7", "name":"zxc" } ]''' info =json.loads(input)#deserialize print'User count:',len(info) for item in info: print'Name:',item['name'] print'Id:',item['id'] print'X:',item['x']
2.Service Oriented Approach SOA 服务导向架构
1.什么是SOA
SOA是一种架构模型,由网站服务技术等标准化组件组成,
目的是为企业、学校或提供网络服务单位建构一个具弹性、可重复使用的整合性接口,
促进内外部应用程序、用户、与部门等的沟通,提升网络服务。
3.Accessing APIs in Python
1.API Application Program Interface
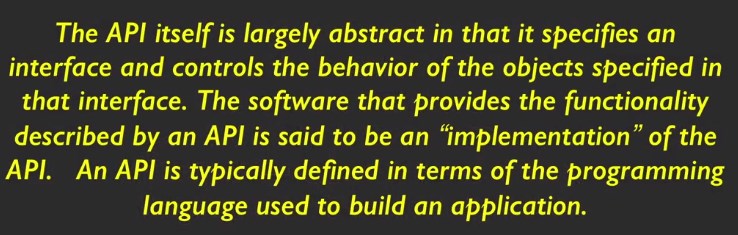
2.Web Service Technologies
3.Google Geocoding
http://maps.googleapis.com/maps/api/geocode/json?sensor=false&address=Ann+Arbor%2C+MI
{ "results":[ { "address_components":[ { "long_name":"安娜堡", "short_name":"安娜堡", "types":["locality","political"] }, { "long_name":"沃什特瑙郡", "short_name":"沃什特瑙郡", "types":["administrative_area_level_2","political"] }, { "long_name":"密歇根州", "short_name":"MI", "types":["administrative_area_level_1","political"] }, { "long_name":"美国", "short_name":"US", "types":["country","political"] } ], "formatted_address":"美国密歇根州安娜堡", "geometry":{ "bounds":{ "northeast":{ "lat":42.3239728, "lng":-83.6758069 }, "southwest":{ "lat":42.222668, "lng":-83.799572 } }, "location":{ "lat":42.2808256, "lng":-83.7430378 }, "location_type":"APPROXIMATE", "viewport":{ "northeast":{ "lat":42.3239728, "lng":-83.6758069 }, "southwest":{ "lat":42.222668, "lng":-83.799572 } } }, "place_id":"ChIJMx9D1A2wPIgR4rXIhkb5Cds", "types":["locality","political"] } ], "status":"OK" }
geojson.py
import urllib import json serviceurl ='http://maps.googleapis.com/maps/api/geocode/json?' #http://maps.googleapis.com/maps/api/geocode/json?sensor=false&address=Ann+Arbor%2C+MI whileTrue: address = raw_input('Enter location: ') if len(address)<1:break url = serviceurl + urllib.urlencode({'sensor':'false','address': address}) print'Retrieving', url # file method successd my_file=open("C:\Users\DUANCHENGHUA\Desktop\json","r") data=my_file.read() # url method failed open ''' uh = urllib.urlopen('http://maps.googleapis.com/maps/api/geocode/json?sensor=false&address=ChongQing%2CChina') data = uh.read() ''' try: js = json.loads(str(data)) except: js =None if'status'notin js or js['status']!='OK': print'==== Failure To Retrieve ====' print data continue print json.dumps(js, indent=4) lat = js["results"][0]["geometry"]["location"]["lat"] lng = js["results"][0]["geometry"]["location"]["lng"] print'lat',lat,'lng',lng location = js['results'][0]['formatted_address'] print location
用urlopen打不开,所以我是直接将该json文件下载到本地进行fileopen
4.API Security and Rate Limiting
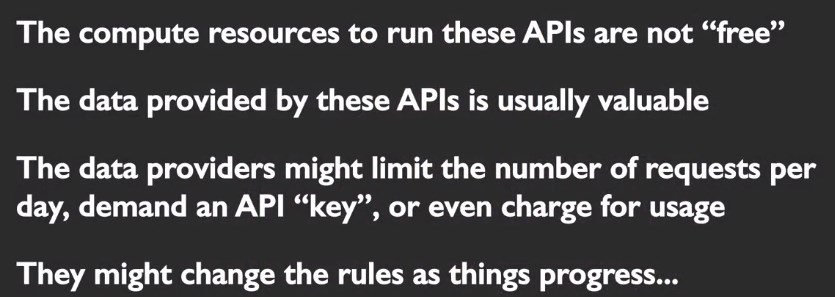
Words:
IT Information Technology、retrieve 检索
作者:Moonache
出处:http://www.cnblogs.com/moonache/
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文链接,否则保留追究法律责任的权利。