简介
ObjectMapper类(com.fasterxml.jackson.databind.ObjectMapper)是Jackson的主要类,它可以帮助我们快速的进行各个类型和Json类型的相互转换。
使用
1、引入Jackson的依赖
2、ObjectMapper的常用配置
private static ObjectMapper mapper;
public ObjectMapper getObjectMapper(){
return this.mapper;
}
static {
//创建ObjectMapper对象
mapper = new ObjectMapper();
//configure方法,配置一些需要的参数
//转换为格式化的json,显示出来的格式美化
mapper.enable(SerializationFeature.INDENT_OUTPUT);
//序列化的时候序列对象的那些属性
//JsonInclude.Include.ALWAYS --所有属性
//JsonInclude.Include.NON_DEFAULT --属性为默认值不序列化
//JsonInclude.Include.NON_EMPTY --属性为 空(“”)或者为NULL都不序列化
//JsonInclude.Include.NON_NULL --属性为NULL 不序列化
mapper.setSerializationInclusion(JsonInclude.Include.ALWAYS);
//返序列化时,遇到未知属性会不会报错
//true:遇到没有的属性就报错 false:遇到没有的属性不会管,也不会报错
mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES,false);
//如果是空对象的时候,不抛异常
mapper.configure(SerializationFeature.FAIL_ON_EMPTY_BEANS,false);
//忽略 transient 修饰的属性
mapper.configure(MapperFeature.PROPAGATE_TRANSIENT_MARKER,true);
//修改序列化后日期格式
mapper.configure(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS,false);
mapper.setDateFormat(new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"));
//处理不同的时区偏移格式
mapper.disable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS);
//mapper.registerModule(new JavaTimeModule());
}
3、ObjectMapper的常用方法
1)json字符串转对象;
将字符串转换为对象:Student sutdent = mapper.readValue(jsonString,Student.class);
将对象转换为字符串:String jsonString = mapper.writeValueAsString(student);
2)数组和对象之间转换;
将数组串转换为对象:Student sutdent = mapper.readValue(bytesArr,Student.class);
将对象转换为数组:byte[] bytesArr = mapper.writeValueAsBytes(student);
3)集合和json字符串之间转换;
将集合转换为字符串:String jsonStr = mapper.writeValueAsString(studentList);//studentList为student对象集合
将字符串转换为集合:List<Student> studentList2 = mapper.readValue(jsonStr,List.class);
4)map和json字符串之间转换;
将map转换为json字符串:String jsonStr = mapper.writeValueAsString(testMap);//testMap为Map<String,Object> testMap = new Hashmap<>()
将json字符串转换为map:Map<String,Object> testMapDes= mapper.readValue(jsonStr,Map.class);
5)日期转json字符串;
mapper.setDateFormat(new SimpleDateFormat("yyyy-MM-dd HH:mm"));
String jsonStr = mapper.writeValueAsString(student);//student对象中包含Date类型的属性
4、例
1)json字符串转对象;
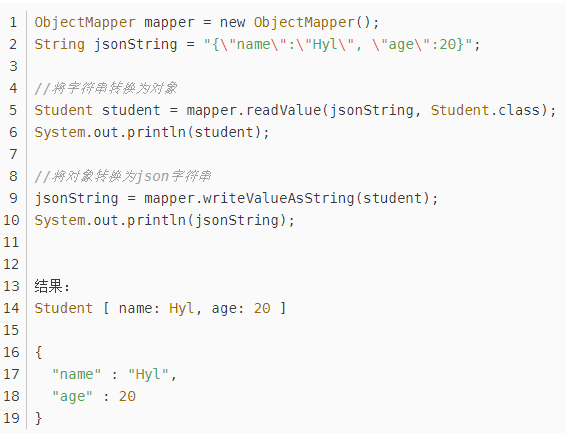
2)数组和对象之间转换;
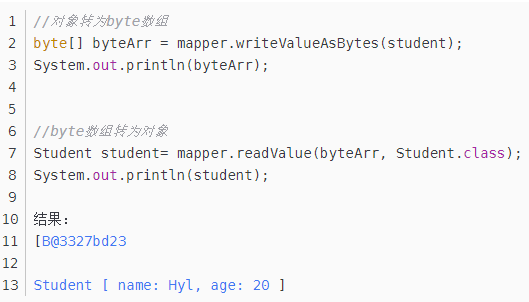
3)集合和json字符串之间转换;
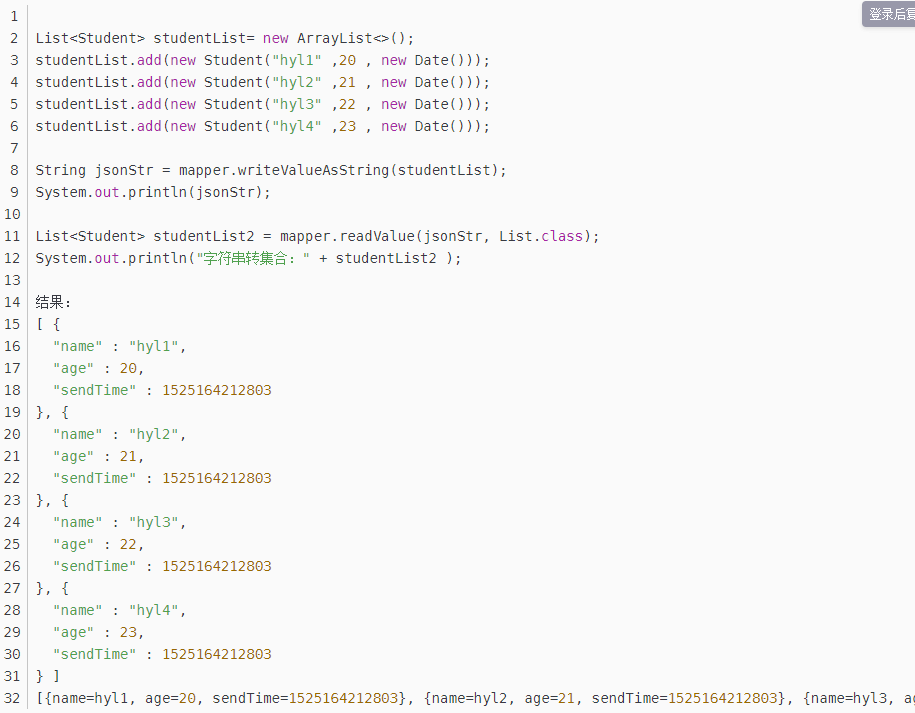
4)map和json字符串之间转换;
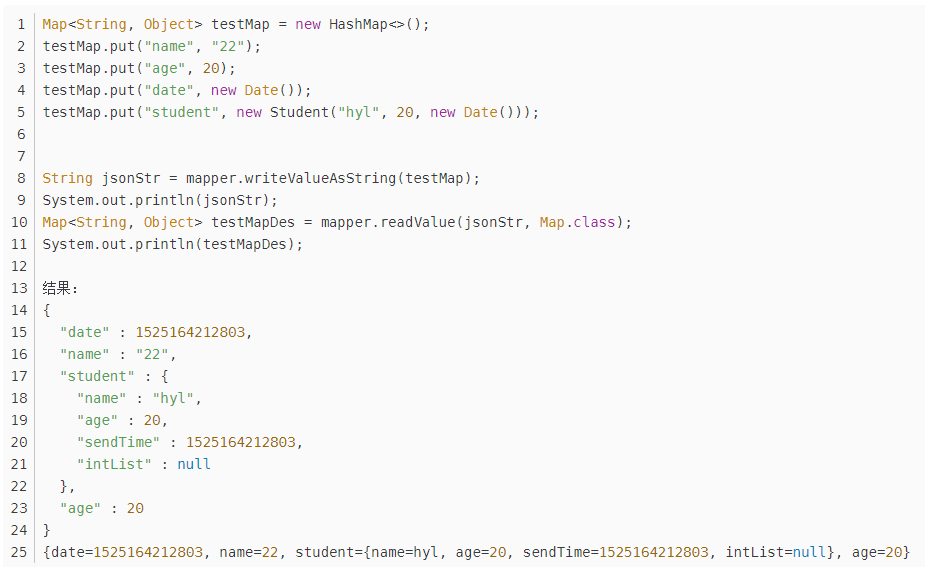
5)日期转json字符串;
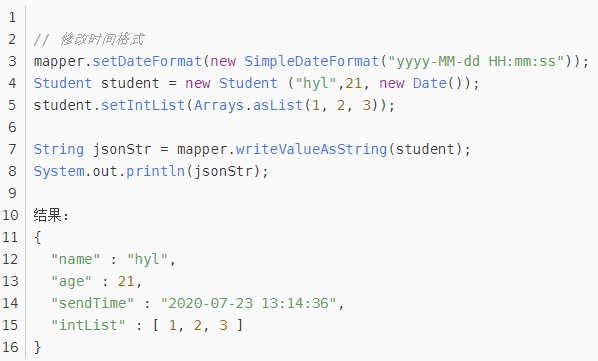
5、js中将字符串转换为json对象
摘抄自:https://blog.csdn.net/technologyleader/article/details/123358279