Java二维码的制作
二维码现在已经到处都是了,下面是二维码的介绍 :
二维码 ,又称 二维条码 , 二维条形码最早发明于日本,它是用某种特定的几何图形按一定规律在平面(二维方向上)分布的黑白相间的图形记录数据符号信息的,在代码编制上巧妙地利用构 成计算机内部逻辑基础的“0”、“1”比特流的概念,使用若干个与二进制相对应的几何形体来表示文字数值信息,通过图象输入设备或光电扫描设备自动识读以 实现信息自动处理。它具有条码技术的一些共性:每种码制有其特定的字符集;每个字符占有一定的宽度;具有一定的校验功能等。同时还具有对不同行的信息自动 识别功能、及处理图形旋转变化等特点。
制作流程:
1.java这边的话生成二维码有很多开发的jar包如zxing,qrcode.q前者是谷歌开发的后者则是小日本开发的,这里的话我使用zxing的开发包来弄
2.先下载zxing开发包,这里用到的只是core那个jar包
下载地址:http://download.csdn.net/download/mobiusstrip/10021893
然后将core-3.3.0.jar包放到lib目录下并添加路径;
3.代码:(http://download.csdn.net/download/mobiusstrip/10022089)

1 package com.lhj.code; 2 3 import java.awt.Graphics2D; 4 import java.awt.geom.AffineTransform; 5 import java.awt.image.BufferedImage; 6 7 import com.google.zxing.LuminanceSource; 8 9 public class BufferedImageLuminanceSource extends LuminanceSource { 10 private final BufferedImage image; 11 private final int left; 12 private final int top; 13 14 public BufferedImageLuminanceSource(BufferedImage image) { 15 this(image, 0, 0, image.getWidth(), image.getHeight()); 16 } 17 18 public BufferedImageLuminanceSource(BufferedImage image, int left, 19 int top, int width, int height) { 20 super(width, height); 21 22 int sourceWidth = image.getWidth(); 23 int sourceHeight = image.getHeight(); 24 if (left + width > sourceWidth || top + height > sourceHeight) { 25 throw new IllegalArgumentException( 26 "Crop rectangle does not fit within image data."); 27 } 28 29 for (int y = top; y < top + height; y++) { 30 for (int x = left; x < left + width; x++) { 31 if ((image.getRGB(x, y) & 0xFF000000) == 0) { 32 image.setRGB(x, y, 0xFFFFFFFF); // = white 33 } 34 } 35 } 36 37 this.image = new BufferedImage(sourceWidth, sourceHeight, 38 BufferedImage.TYPE_BYTE_GRAY); 39 this.image.getGraphics().drawImage(image, 0, 0, null); 40 this.left = left; 41 this.top = top; 42 } 43 44 45 public byte[] getRow(int y, byte[] row) { 46 if (y < 0 || y >= getHeight()) { 47 throw new IllegalArgumentException( 48 "Requested row is outside the image: " + y); 49 } 50 int width = getWidth(); 51 if (row == null || row.length < width) { 52 row = new byte[width]; 53 } 54 image.getRaster().getDataElements(left, top + y, width, 1, row); 55 return row; 56 } 57 58 59 public byte[] getMatrix() { 60 int width = getWidth(); 61 int height = getHeight(); 62 int area = width * height; 63 byte[] matrix = new byte[area]; 64 image.getRaster().getDataElements(left, top, width, height, matrix); 65 return matrix; 66 } 67 68 69 public boolean isCropSupported() { 70 return true; 71 } 72 73 74 public LuminanceSource crop(int left, int top, int width, int height) { 75 return new BufferedImageLuminanceSource(image, this.left + left, 76 this.top + top, width, height); 77 } 78 79 80 public boolean isRotateSupported() { 81 return true; 82 } 83 84 85 public LuminanceSource rotateCounterClockwise() { 86 int sourceWidth = image.getWidth(); 87 int sourceHeight = image.getHeight(); 88 AffineTransform transform = new AffineTransform(0.0, -1.0, 1.0, 89 0.0, 0.0, sourceWidth); 90 BufferedImage rotatedImage = new BufferedImage(sourceHeight, 91 sourceWidth, BufferedImage.TYPE_BYTE_GRAY); 92 Graphics2D g = rotatedImage.createGraphics(); 93 g.drawImage(image, transform, null); 94 g.dispose(); 95 int width = getWidth(); 96 return new BufferedImageLuminanceSource(rotatedImage, top, 97 sourceWidth - (left + width), getHeight(), width); 98 } 99 }

1 package com.lhj.code; 2 3 import java.awt.BasicStroke; 4 import java.awt.Graphics; 5 import java.awt.Graphics2D; 6 import java.awt.Image; 7 import java.awt.Shape; 8 import java.awt.geom.RoundRectangle2D; 9 import java.awt.image.BufferedImage; 10 import java.io.File; 11 import java.io.OutputStream; 12 import java.util.Hashtable; 13 import java.util.Random; 14 15 import javax.imageio.ImageIO; 16 17 import com.google.zxing.BarcodeFormat; 18 import com.google.zxing.BinaryBitmap; 19 import com.google.zxing.DecodeHintType; 20 import com.google.zxing.EncodeHintType; 21 import com.google.zxing.MultiFormatReader; 22 import com.google.zxing.MultiFormatWriter; 23 import com.google.zxing.Result; 24 import com.google.zxing.common.BitMatrix; 25 import com.google.zxing.common.HybridBinarizer; 26 import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel; 27 28 29 public class QRCodeUtil { 30 31 private static final String CHARSET = "utf-8"; 32 private static final String FORMAT_NAME = "JPG"; 33 // 二维码尺寸 34 private static final int QRCODE_SIZE = 300; 35 // LOGO宽度 36 private static final int WIDTH = 60; 37 // LOGO高度 38 private static final int HEIGHT = 60; 39 40 private static BufferedImage createImage(String content, String imgPath, 41 boolean needCompress) throws Exception { 42 Hashtable hints = new Hashtable(); 43 hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H); 44 hints.put(EncodeHintType.CHARACTER_SET, CHARSET); 45 hints.put(EncodeHintType.MARGIN, 1); 46 BitMatrix bitMatrix = new MultiFormatWriter().encode(content, 47 BarcodeFormat.QR_CODE, QRCODE_SIZE, QRCODE_SIZE, hints); 48 int width = bitMatrix.getWidth(); 49 int height = bitMatrix.getHeight(); 50 BufferedImage image = new BufferedImage(width, height, 51 BufferedImage.TYPE_INT_RGB); 52 for (int x = 0; x < width; x++) { 53 for (int y = 0; y < height; y++) { 54 image.setRGB(x, y, bitMatrix.get(x, y) ? 0xFF000000 55 : 0xFFFFFFFF); 56 } 57 } 58 if (imgPath == null || "".equals(imgPath)) { 59 return image; 60 } 61 // 插入图片 62 QRCodeUtil.insertImage(image, imgPath, needCompress); 63 return image; 64 } 65 66 67 private static void insertImage(BufferedImage source, String imgPath, 68 boolean needCompress) throws Exception { 69 File file = new File(imgPath); 70 if (!file.exists()) { 71 System.err.println(""+imgPath+" 该文件不存在!"); 72 return; 73 } 74 Image src = ImageIO.read(new File(imgPath)); 75 int width = src.getWidth(null); 76 int height = src.getHeight(null); 77 if (needCompress) { // 压缩LOGO 78 if (width > WIDTH) { 79 width = WIDTH; 80 } 81 if (height > HEIGHT) { 82 height = HEIGHT; 83 } 84 Image image = src.getScaledInstance(width, height, 85 Image.SCALE_SMOOTH); 86 BufferedImage tag = new BufferedImage(width, height, 87 BufferedImage.TYPE_INT_RGB); 88 Graphics g = tag.getGraphics(); 89 g.drawImage(image, 0, 0, null); // 绘制缩小后的图 90 g.dispose(); 91 src = image; 92 } 93 // 插入LOGO 94 Graphics2D graph = source.createGraphics(); 95 int x = (QRCODE_SIZE - width) / 2; 96 int y = (QRCODE_SIZE - height) / 2; 97 graph.drawImage(src, x, y, width, height, null); 98 Shape shape = new RoundRectangle2D.Float(x, y, width, width, 6, 6); 99 graph.setStroke(new BasicStroke(3f)); 100 graph.draw(shape); 101 graph.dispose(); 102 } 103 104 105 public static void encode(String content, String imgPath, String destPath, 106 boolean needCompress) throws Exception { 107 BufferedImage image = QRCodeUtil.createImage(content, imgPath, 108 needCompress); 109 mkdirs(destPath); 110 String file = new Random().nextInt(99999999)+".jpg"; 111 ImageIO.write(image, FORMAT_NAME, new File(destPath+"/"+file)); 112 } 113 114 115 public static void mkdirs(String destPath) { 116 File file =new File(destPath); 117 //当文件夹不存在时,mkdirs会自动创建多层目录,区别于mkdir.(mkdir如果父目录不存在则会抛出异常) 118 if (!file.exists() && !file.isDirectory()) { 119 file.mkdirs(); 120 } 121 } 122 123 124 public static void encode(String content, String imgPath, String destPath) 125 throws Exception { 126 QRCodeUtil.encode(content, imgPath, destPath, false); 127 } 128 129 130 public static void encode(String content, String destPath, 131 boolean needCompress) throws Exception { 132 QRCodeUtil.encode(content, null, destPath, needCompress); 133 } 134 135 136 public static void encode(String content, String destPath) throws Exception { 137 QRCodeUtil.encode(content, null, destPath, false); 138 } 139 140 141 public static void encode(String content, String imgPath, 142 OutputStream output, boolean needCompress) throws Exception { 143 BufferedImage image = QRCodeUtil.createImage(content, imgPath, 144 needCompress); 145 ImageIO.write(image, FORMAT_NAME, output); 146 } 147 148 149 public static void encode(String content, OutputStream output) 150 throws Exception { 151 QRCodeUtil.encode(content, null, output, false); 152 } 153 154 155 public static String decode(File file) throws Exception { 156 BufferedImage image; 157 image = ImageIO.read(file); 158 if (image == null) { 159 return null; 160 } 161 BufferedImageLuminanceSource source = new BufferedImageLuminanceSource( 162 image); 163 BinaryBitmap bitmap = new BinaryBitmap(new HybridBinarizer(source)); 164 Result result; 165 Hashtable hints = new Hashtable(); 166 hints.put(DecodeHintType.CHARACTER_SET, CHARSET); 167 result = new MultiFormatReader().decode(bitmap, hints); 168 String resultStr = result.getText(); 169 return resultStr; 170 } 171 172 173 public static String decode(String path) throws Exception { 174 return QRCodeUtil.decode(new File(path)); 175 } 176 177 178 }

1 package com.lhj.code; 2 3 /** 4 * 二维码生成测试类 5 * @author Cloud 6 * @data 2016-11-21 7 * QRCodeTest 8 */ 9 10 public class QRCodeTest { 11 12 public static void main(String[] args) throws Exception { 13 14 String text = "http://www.cnblogs.com/mmzs/"; 15 16 //不带logo的二维码 17 QRCodeUtil.encode(text, "", "d:/test", true); 18 //参数依次代表:内容;logo地址;二维码输出目录;是否需要压缩图片 19 //QRCodeUtil.encode(text, "d:/test");//参数依次代表:内容;二维码输出目录; 20 21 //带logo的二维码 22 QRCodeUtil.encode(text, "d:/test/1.jpg", "d:/test", true); 23 24 25 26 System.out.println("success"); 27 } 28 }
运行结果:Java学习交流QQ群:603654340
success
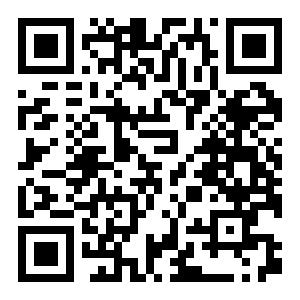
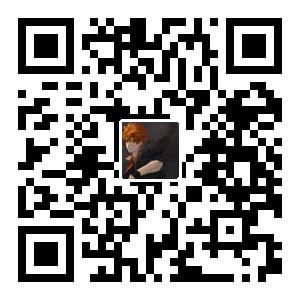
PS:引用http://blog.sina.com.cn/s/blog_5a6efa330102v1lb.html
【推荐】编程新体验,更懂你的AI,立即体验豆包MarsCode编程助手
【推荐】凌霞软件回馈社区,博客园 & 1Panel & Halo 联合会员上线
【推荐】抖音旗下AI助手豆包,你的智能百科全书,全免费不限次数
【推荐】轻量又高性能的 SSH 工具 IShell:AI 加持,快人一步
· Linux系统下SQL Server数据库镜像配置全流程详解
· 现代计算机视觉入门之:什么是视频
· 你所不知道的 C/C++ 宏知识
· 聊一聊 操作系统蓝屏 c0000102 的故障分析
· SQL Server 内存占用高分析
· 盘点!HelloGitHub 年度热门开源项目
· DeepSeek V3 两周使用总结
· 02现代计算机视觉入门之:什么是视频
· C#使用yield关键字提升迭代性能与效率
· 回顾我的软件开发经历(1)