POJ 2559 Largest Rectangle in a Histogram (单调栈)
题目:
Largest Rectangle in a Histogram
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 18299 | Accepted: 5881 |
Description
A histogram is a polygon composed of a sequence of rectangles aligned at a common base line. The rectangles have equal widths but may have different heights. For example, the figure on the left shows the histogram that consists of rectangles with the heights 2, 1, 4, 5, 1, 3, 3, measured in units where 1 is the width of the rectangles:
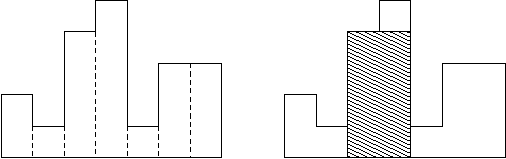
Usually, histograms are used to represent discrete distributions, e.g., the frequencies of characters in texts. Note that the order of the rectangles, i.e., their heights, is important. Calculate the area of the largest rectangle in a histogram that is aligned at the common base line, too. The figure on the right shows the largest aligned rectangle for the depicted histogram.
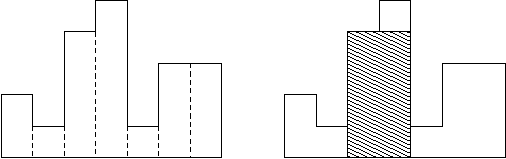
Usually, histograms are used to represent discrete distributions, e.g., the frequencies of characters in texts. Note that the order of the rectangles, i.e., their heights, is important. Calculate the area of the largest rectangle in a histogram that is aligned at the common base line, too. The figure on the right shows the largest aligned rectangle for the depicted histogram.
Input
The input contains several test cases. Each test case describes a histogram and starts with an integer n, denoting the number of rectangles it is composed of. You may assume that 1<=n<=100000. Then follow n integersh1,...,hn, where 0<=hi<=1000000000. These numbers denote the heights of the rectangles of the histogram in left-to-right order. The width of each rectangle is 1. A zero follows the input for the last test case.
Output
For each test case output on a single line the area of the largest rectangle in the specified histogram. Remember that this rectangle must be aligned at the common base line.
Sample Input
7 2 1 4 5 1 3 3 4 1000 1000 1000 1000 0
Sample Output
8 4000
Hint
Huge input, scanf is recommended.
解题思路:
这道题是说,我现在有一个横轴,然后每个轴上矩形的高度不同,求出这些连着的矩形构成的最大面积是多少?
这道题用到了一个叫做单调栈的东西,虽然先前并不是很懂单调栈,希望通过这个题,能够对单调栈有一个更加深刻的理解和认识。
就是说,栈中的元素按照某种方式的排序下,必须是单调的,但凡有新的元素入栈后,打破了原有栈的单调性,我们就必须弹出此刻栈顶栈的元素,直到当前的这个栈满足单调性。
代码:
1 # include <cstdio> 2 # include <iostream> 3 using namespace std; 4 # define MAX 123456 5 typedef long long LL; 6 struct node 7 { 8 int height; 9 int cnt; 10 }stack[MAX]; 11 int top; 12 int main(void) 13 { 14 int n; 15 int h; 16 LL ans = 0, tot = 0, tmp = 0; 17 while ( scanf("%d",&n)!=EOF ) 18 { 19 if ( n==0 ) break; 20 ans = 0; 21 for ( int i = 0;i < n;i++ ) 22 { 23 scanf("%d",&h); 24 tmp = 0; 25 while ( top > 0 && stack[top-1].height >= h ) 26 { 27 tot = stack[top-1].height*(stack[top-1].cnt+tmp); 28 ans = max(ans,tot); 29 tmp += stack[top-1].cnt; 30 top--; 31 } 32 stack[top].height = h; 33 stack[top].cnt = tmp+1; 34 top++; 35 } 36 tmp = 0; 37 while ( top > 0 ) 38 { 39 tot = stack[top-1].height*(stack[top-1].cnt+tmp); 40 ans = max(ans,tot); 41 tmp += stack[top-1].cnt; 42 top--; 43 } 44 printf("%lld\n",ans); 45 } 46 return 0; 47 }