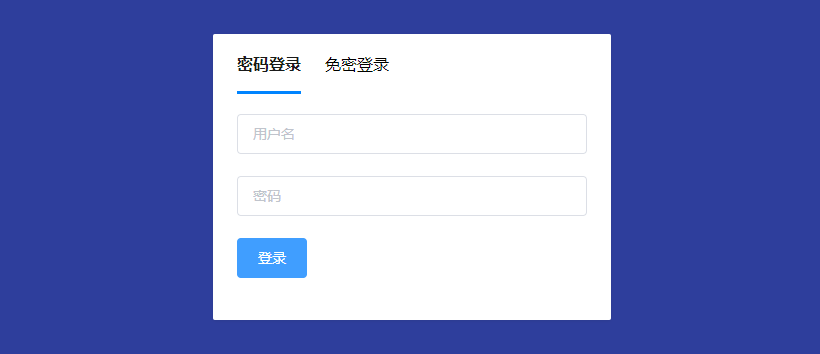
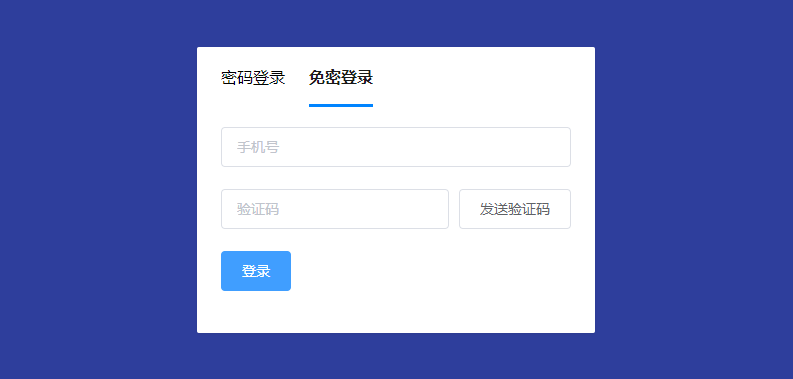
Login.vue
<template>
<div class="main">
<div class="loginBox">
<div class="tabBoxSwitch">
<ul class="tabBoxSwitchUl">
<li :class="tabSelected===index?'tab-active': ''" v-for="(txt, index) in tabList" :key="index" @click="tabSelected=index">{{ txt }}</li>
</ul>
</div>
<div v-show="tabSelected===0">
<el-form>
<el-form-item style="margin-top: 20px">
<el-input v-model="userForm.username" placeholder="用户名"></el-input>
</el-form-item>
<el-form-item>
<el-input v-model="userForm.password" placeholder="密码" show-password></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" size="smail">登录</el-button>
</el-form-item>
</el-form>
</div>
<div v-show="tabSelected===1">
<el-form>
<el-form-item style="margin-top: 20px">
<el-input v-model="smsForm.phone" placeholder="手机号"></el-input>
</el-form-item>
<el-form-item>
<el-row type="flex" justify="space-between">
<el-input v-model="smsForm.code" placeholder="验证码"></el-input>
<el-button style="margin-left: 10px">发送验证码</el-button>
</el-row>
</el-form-item>
<el-form-item>
<el-button type="primary" size="smail">登录</el-button>
</el-form-item>
</el-form>
</div>
</div>
</div>
</template>
<script>
export default {
name: "Login",
data(){
return {
tabSelected: 0,
tabList: ['密码登录', '免密登录'],
userForm: {
username: '',
password: ''
},
smsForm: {
phone: '',
code: ''
}
}
},
methods: {
}
}
</script>
<style scoped>
.main{
width: 100%;
height: 100vh; /* 可视程度的100% */
background-color: #2E3E9C;
display: flex;
flex-direction: column; /* 按列排列 */
justify-content: center; /* 纵向居中显示 */
align-items: center; /* 横向居中显示 */
}
.loginBox {
background-color: #fff;
box-shadow: 0 1px 3px rgba(26, 26, 26, 0.1);
border-radius: 2px;
width: 350px;
min-height: 200px;
padding: 0 24px 20px;
}
.tabBoxSwitch .tabBoxSwitchUl {
list-style: none;
padding: 0;
margin: 0;
}
.tabBoxSwitch .tabBoxSwitchUl li {
display: inline-block;
height: 60px;
font-size: 16px;
line-height: 60px;
margin-right: 24px;
cursor: pointer;
}
.tab-active {
position: relative;
color: #1a1a1a;
font-weight: 600;
font-synthesis: style;
}
.tab-active::before {
display: block;
position: absolute;
bottom: 0;
content: "";
width: 100%;
height: 3px;
background-color: #0084ff;
}
</style>
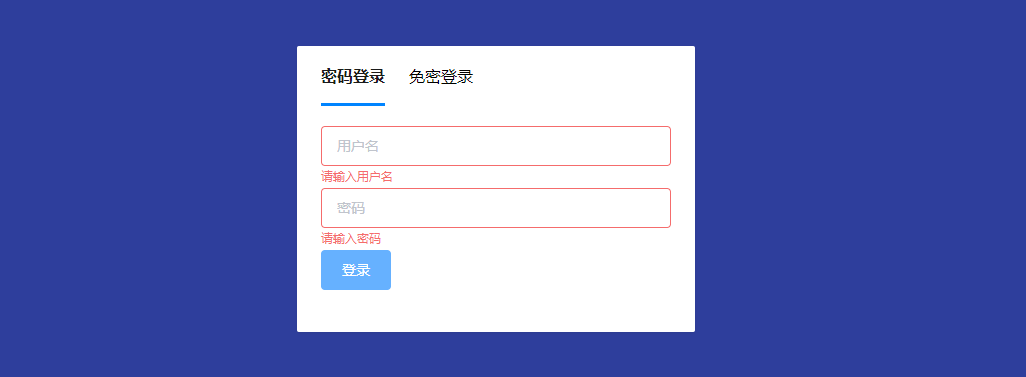
Login.vue
<template>
<div class="main">
<div class="loginBox">
<div class="tabBoxSwitch">
<ul class="tabBoxSwitchUl">
<li :class="tabSelected===index?'tab-active': ''" v-for="(txt, index) in tabList" :key="index" @click="tabSelected=index">{{ txt }}</li>
</ul>
</div>
<div v-show="tabSelected===0">
<el-form :model="userForm" :rules="userRules" ref="userForm">
<!-- rules="":校验规则 model="":要校验的数据 ref="":button提交的数据 -->
<el-form-item prop="username" style="margin-top: 20px">
<!-- prop:错误信息展示 -->
<el-input v-model="userForm.username" placeholder="用户名"></el-input>
</el-form-item>
<el-form-item prop="password">
<el-input v-model="userForm.password" placeholder="密码" show-password></el-input>
</el-form-item>
<el-form-item>
<el-button @click="submitForm('userForm')" type="primary" size="smail">登录</el-button>
</el-form-item>
</el-form>
</div>
<div v-show="tabSelected===1">
<el-form :model="smsForm" :rules="smsRules" ref="smsForm">
<el-form-item prop="phone" style="margin-top: 20px">
<el-input v-model="smsForm.phone" placeholder="手机号"></el-input>
</el-form-item>
<el-form-item prop="code">
<el-row type="flex" justify="space-between">
<el-input v-model="smsForm.code" placeholder="验证码"></el-input>
<el-button style="margin-left: 10px">发送验证码</el-button>
</el-row>
</el-form-item>
<el-form-item>
<el-button @click="submitForm('smsForm')" type="primary" size="smail">登录</el-button>
</el-form-item>
</el-form>
</div>
</div>
</div>
</template>
<script>
export default {
name: "Login",
data(){
return {
tabSelected: 0,
tabList: ['密码登录', '免密登录'],
userForm: {
username: '',
password: ''
},
userRules: {
username: [
{required: true, message: '请输入用户名', trigger: 'blur'},
],
password: [
{required: true, message: '请输入密码', trigger: 'blur'},
// required:必须的 trigger: 光标移出就会校验
]
},
smsForm: {
phone: '',
code: ''
},
smsRules: {
phone: [
{required: true, message: '请输入手机号', trigger: 'blur'},
{pattern: /^[3456789]\d{9}$/, message: '手机号格式错误', trigger: 'blur'}
// pattern: 正则
],
code: [
{required: true, message: '请输入验证码', trigger: 'blur'},
// required:必须的 trigger: 光标移出就会校验
]
},
}
},
methods: {
submitForm(forName){
// 执行验证规则
this.$refs[forName].validate((valid)=>{
if(!valid){
console.log('验证未通过');
return false;
}
console.log('验证通过');
})
}
}
}
</script>
<style scoped>
.main{
width: 100%;
height: 100vh; /* 可视程度的100% */
background-color: #2E3E9C;
display: flex;
flex-direction: column; /* 按列排列 */
justify-content: center; /* 纵向居中显示 */
align-items: center; /* 横向居中显示 */
}
.loginBox {
background-color: #fff;
box-shadow: 0 1px 3px rgba(26, 26, 26, 0.1);
border-radius: 2px;
width: 350px;
min-height: 200px;
padding: 0 24px 20px;
}
.tabBoxSwitch .tabBoxSwitchUl {
list-style: none;
padding: 0;
margin: 0;
}
.tabBoxSwitch .tabBoxSwitchUl li {
display: inline-block;
height: 60px;
font-size: 16px;
line-height: 60px;
margin-right: 24px;
cursor: pointer;
}
.tab-active {
position: relative;
color: #1a1a1a;
font-weight: 600;
font-synthesis: style;
}
.tab-active::before {
display: block;
position: absolute;
bottom: 0;
content: "";
width: 100%;
height: 3px;
background-color: #0084ff;
}
</style>
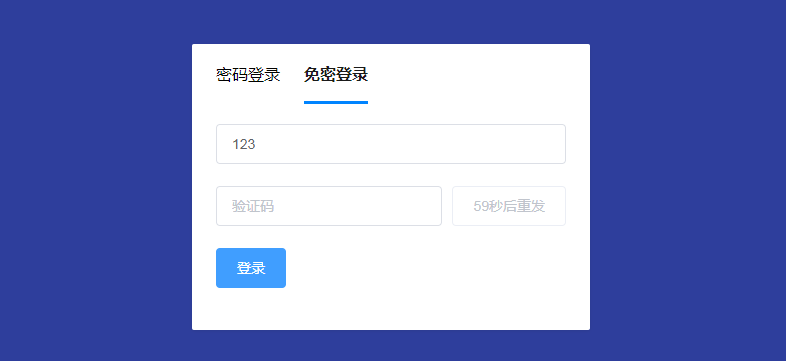
Login.vue
<template>
<div class="main">
<div class="loginBox">
<div class="tabBoxSwitch">
<ul class="tabBoxSwitchUl">
<li :class="tabSelected===index?'tab-active': ''" v-for="(txt, index) in tabList" :key="index" @click="tabSelected=index">{{ txt }}</li>
</ul>
</div>
<div v-show="tabSelected===0">
<el-form :model="userForm" :rules="userRules" ref="userForm">
<!-- rules="":校验规则 model="":要校验的数据 ref="":button提交的数据 -->
<el-form-item prop="username" style="margin-top: 20px">
<!-- prop:错误信息展示 -->
<el-input v-model="userForm.username" placeholder="用户名"></el-input>
</el-form-item>
<el-form-item prop="password">
<el-input v-model="userForm.password" placeholder="密码" show-password></el-input>
</el-form-item>
<el-form-item>
<el-button @click="submitForm('userForm')" type="primary" size="smail">登录</el-button>
</el-form-item>
</el-form>
</div>
<div v-show="tabSelected===1">
<el-form :model="smsForm" :rules="smsRules" ref="smsForm">
<el-form-item prop="phone" style="margin-top: 20px">
<el-input v-model="smsForm.phone" placeholder="手机号"></el-input>
</el-form-item>
<el-form-item prop="code">
<el-row type="flex" justify="space-between">
<el-input v-model="smsForm.code" placeholder="验证码"></el-input>
<el-button :disabled="btnSmsDisabled" @click="sendSmsCode" style="margin-left: 10px">{{ btnSmsText }}</el-button>
</el-row>
</el-form-item>
<el-form-item>
<el-button @click="submitForm('smsForm')" type="primary" size="smail">登录</el-button>
</el-form-item>
</el-form>
</div>
</div>
</div>
</template>
<script>
export default {
name: "Login",
data(){
return {
tabSelected: 0,
tabList: ['密码登录', '免密登录'],
userForm: {
username: '',
password: ''
},
userRules: {
username: [
{required: true, message: '请输入用户名', trigger: 'blur'},
],
password: [
{required: true, message: '请输入密码', trigger: 'blur'},
// required:必须的 trigger: 光标移出就会校验
]
},
smsForm: {
phone: '',
code: ''
},
smsRules: {
phone: [
{required: true, message: '请输入手机号', trigger: 'blur'},
// {pattern: /^[123456789]\d{9}$/, message: '手机号格式错误', trigger: 'blur'}
// pattern: 正则
],
code: [
{required: true, message: '请输入验证码', trigger: 'blur'},
// required:必须的 trigger: 光标移出就会校验
]
},
btnSmsDisabled: false, // 是否可点击
btnSmsText: '发送验证码'
}
},
methods: {
submitForm(forName){
// 执行验证规则
this.$refs[forName].validate((valid)=>{
if(!valid){
console.log('验证未通过');
return false;
}
console.log('验证通过');
})
},
sendSmsCode(){
// 校验单独的某个字段
this.$refs.smsForm.validateField('phone', (error)=>{
console.log(error) //请输入手机号
if (error){ return false }
// 验证通过拿到手机号,向后台发送请求 -> 发送验证码
this.btnSmsDisabled = true; // 禁用按钮
// 设置倒计时
let txt = 60;
let interval = window.setInterval(()=>{
txt -= 1;
this.btnSmsText = `${txt}秒后重发`;
if (txt < 1){
this.btnSmsText = '重新发送';
this.btnSmsDisabled = false;
window.clearInterval(interval);
}
}, 1000);
})
}
}
}
</script>
<style scoped>
.main{
width: 100%;
height: 100vh; /* 可视程度的100% */
background-color: #2E3E9C;
display: flex;
flex-direction: column; /* 按列排列 */
justify-content: center; /* 纵向居中显示 */
align-items: center; /* 横向居中显示 */
}
.loginBox {
background-color: #fff;
box-shadow: 0 1px 3px rgba(26, 26, 26, 0.1);
border-radius: 2px;
width: 350px;
min-height: 200px;
padding: 0 24px 20px;
}
.tabBoxSwitch .tabBoxSwitchUl {
list-style: none;
padding: 0;
margin: 0;
}
.tabBoxSwitch .tabBoxSwitchUl li {
display: inline-block;
height: 60px;
font-size: 16px;
line-height: 60px;
margin-right: 24px;
cursor: pointer;
}
.tab-active {
position: relative;
color: #1a1a1a;
font-weight: 600;
font-synthesis: style;
}
.tab-active::before {
display: block;
position: absolute;
bottom: 0;
content: "";
width: 100%;
height: 3px;
background-color: #0084ff;
}
</style>