python之字典(dict)创建与使用
字典(dict) 在其他语言中被称为哈希映射(hash map)或者相关数组,它是一种大小可变的键值对集,其中的key、value都是python对象。
特别注意:
1.字典中的key不能重复,key可以是任意类型
2.字典是无序的,所以不能像数组、元组一样通过下标读取
字典创建:
1.创建空字典
word = dict()
2.创建非空字典
words = {"rice": "米", "breakfast": "早餐", "lunch": "午餐", "dinner": "晚餐", "bacon": "培根", "pasta": "意大利面", "have": "吃", "egg": "蛋"}

price = dict(rice= 3.44, bacon=8.99, egg=8.99, pasta=68.7) print(price)

price = dict(zip(["rice", "bacon", "egg", "pasta"], [3.44, 8.99, 8.99, 68.7])) print(price)

price = dict([("rice", 3.44), ("bacon", 8.99), ("egg", 9.99), ("pasta", 68.7)]) print(price)

字典读取:
1.通过key读取
print(words["egg"])

2.通过get()方法读取-----常用
使用get()方法读取时,当传入一个不存在的键时,程序不会抛出异常,若未指定返回值则返回None,否则返回所指定值
one = words.get("rice") print(one) two = words.get("apple") print(two) three = words.get("banana", "暂无该商品") print(three)

3.通过 setdefault()方法读取
setdefault()方法与get()方法类似,若给定的key存在,则返回key对应的值,若不存在,值返回给定的默认值,若未指定,值默认为None,并且字典中同时添加该键与值
four = price.setdefault("banana", 6.99) five = price.setdefault("rice") six = price.setdefault("apple") print(four) print(five) print(six)

4.遍历key
for key in words.keys(): print(key)
或
for key in words: #对比以上,如果不加任何方法说明,那么默认输出的是字典中所有键的值 print(key)
5.遍历value
for value in words.values(): print(value)
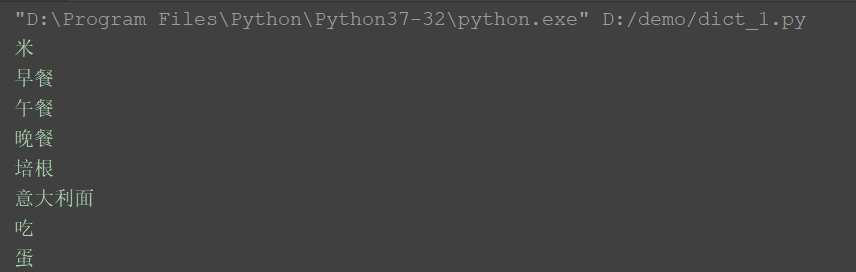
6.遍历键值对
for item in words.items(): print(item)
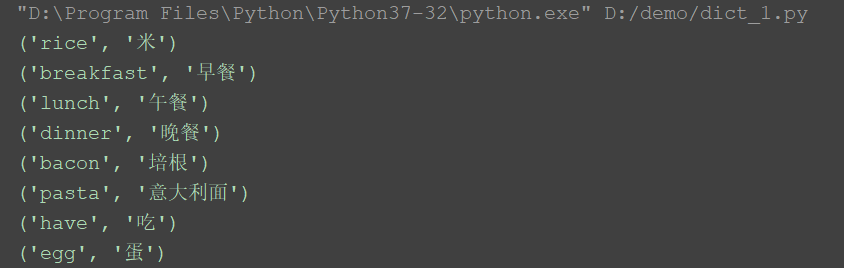
for key, value in words.items(): print({key: value})
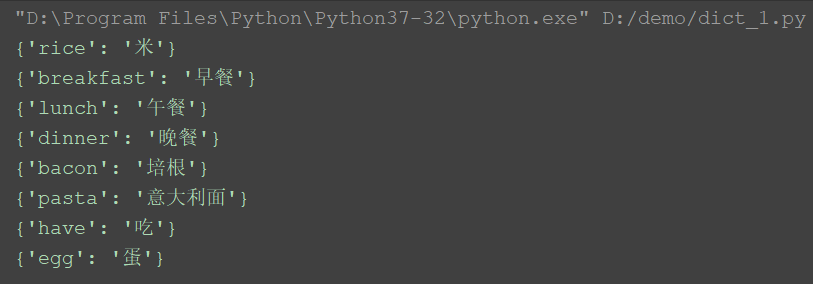
7.字典的删除
# clear()方法清除字典中所有的元素 words.clear() print(words)

# pop()方法通过传入key,删除元素 words.pop("rice") print(words)

#popitem()方法随机删除某项键值对 words.popitem() print(words)

# del()方法可通过传入的key删除某项元素,也可直接删除整个字典 del words["egg"] print(words)
del words print(words)
8.字典的修改
# 通过[key]插入元素 price["apple"] = 6.99 print(price)

# setdefault()方法与get()方法类似,若给定的key存在,则返回key对应的值, # 若不存在,值返回给定的默认值,若未指定,值默认为None,并且字典中同时添加该键与值 price.setdefault("banana", 6.99) price.setdefault("milk") print(price)

# 通过[key]修改 price["rice"] = 3.99 print(price)

# 通过update()方法修改 ---使用解包字典 price.update(**{"rice": 4.99}) # 通过update()方法修改 ---使用字典对象 price.update({"rice": 5.99}) # 通过update()方法修改 ---使用关键字参数 price.update(rice=6.99) print(price)
