Winform ListView中使用combox填充单元格
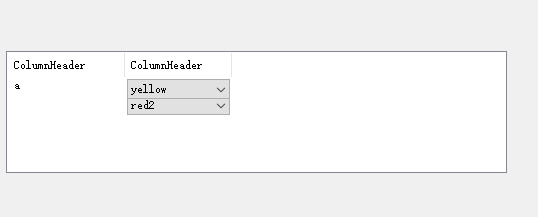
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace WindowsFormsAppTest { public partial class ListViewxc : ListView { private List<Control> controlsxc = new List<Control>(); private const int WM_PAINT = 0x000F; public ListViewxc() { InitializeComponent(); controlsxc.Clear(); this.OwnerDraw = true; this.DrawColumnHeader += new DrawListViewColumnHeaderEventHandler(this_DrawColumnHeader); this.DrawSubItem += new DrawListViewSubItemEventHandler(this_DrawSubItem); } protected override void OnPaint(PaintEventArgs e) { foreach (Control item in controlsxc) { ListViewSubItemxc subItemxc = item.Tag as ListViewSubItemxc; if (subItemxc != null) { Rectangle r = subItemxc.Bounds; ComboBox combox = item as ComboBox; if (combox != null) { if (r.Y > 0 && r.Y < this.ClientRectangle.Height) { combox.Visible = true; combox.Bounds = new Rectangle(r.X + 2, r.Y + 2, r.Width - (2 * 2), r.Height - (2 * 2)); System.Diagnostics.Debug.WriteLine(",,," + r.X + " " + r.Y + " " + r.Width + " " + r.Height); } else { combox.Visible = false; } } } } base.OnPaint(e); } protected override void WndProc(ref Message m) { if (m.Msg == WM_PAINT) { foreach (Control item in controlsxc) { ListViewSubItemxc subItemxc = item.Tag as ListViewSubItemxc; if (subItemxc != null) { Rectangle r = subItemxc.Bounds; ComboBox combox = item as ComboBox; if (combox != null) { if (r.Y > 0 && r.Y < this.ClientRectangle.Height) { combox.Visible = true; combox.Bounds = new Rectangle(r.X + 2, r.Y + 2, r.Width - (2 * 2), r.Height - (2 * 2)); System.Diagnostics.Debug.WriteLine(",,," + r.X + " " + r.Y + " " + r.Width + " " + r.Height); } else { combox.Visible = false; } } } } } base.WndProc(ref m); } private void this_DrawSubItem(object sender, DrawListViewSubItemEventArgs e) { e.DrawDefault = true; } private void this_DrawColumnHeader(object sender, DrawListViewColumnHeaderEventArgs e) { e.DrawDefault = true; } public void AddItemxc(ListViewItem item) { foreach (ListViewItem.ListViewSubItem it in item.SubItems) { ListViewSubItemxc x = it as ListViewSubItemxc; if (x != null && x._control != null) { this.Controls.Add(x._control); x._control.Tag = x; ComboBox co = x._control as ComboBox; if (co != null) { co.SelectedIndexChanged += Co_SelectedIndexChanged; controlsxc.Add(co); } } } this.Items.Add(item); } private void Co_SelectedIndexChanged(object sender, EventArgs e) { ComboBox co = sender as ComboBox; ListViewSubItemxc x = co.Tag as ListViewSubItemxc; if (x != null) { x.Text = co.SelectedText; } } } public class ListViewSubItemxc : ListViewItem.ListViewSubItem { public Control _control = null; public ListViewSubItemxc(Control con) { _control = con; } } }
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace WindowsFormsAppTest { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { ListViewItem l = new ListViewItem(); l.Text = "a"; ComboBox cb = new ComboBox(); cb.DropDownStyle = ComboBoxStyle.DropDownList; cb.Items.Add("yellow"); cb.Items.Add("red"); cb.SelectedIndex = 0; ListViewSubItemxc lxc = new ListViewSubItemxc(cb); ListViewItem l2 = new ListViewItem(); ComboBox cb2 = new ComboBox(); cb2.DropDownStyle = ComboBoxStyle.DropDownList; cb2.Items.Add("yellow2"); cb2.Items.Add("red2"); cb2.SelectedIndex = 1; ListViewSubItemxc lxc2 = new ListViewSubItemxc(cb2); l.SubItems.Add(lxc); l2.SubItems.Add(lxc2); listViewxc1.AddItemxc(l); listViewxc1.AddItemxc(l2); } } }