#ifndef CAR_H #define CAR_H #include "engine.h" #include <iostream> #include <stdio.h> #include <string> #include <functional> using namespace std; class Car { public: Car(Engine *engine); ~Car(); public: void show_info(); public: Engine *m_Engine; }; #endif // CAR_H
#include "car.h" Car::Car(Engine *engine) { m_Engine = engine; m_Engine->m_f = std::bind(&Car::show_info, this); //绑定widget中的回调 } Car::~Car() { if (m_Engine != 0) { delete m_Engine; m_Engine = 0; } } void Car::show_info() { std::cout << "this is my first car." << std::endl; }
#ifndef ENGINE_H #define ENGINE_H #include "engine.h" #include <iostream> #include <stdio.h> #include <string> #include <functional> using namespace std; typedef std::function<void()> Func; //定义函数指针 返回值为void,参数为int型 class Engine { public: Engine(); public: Func m_f; }; #endif // ENGINE_H
#include "engine.h" Engine::Engine(){}
Engine *engine = NULL; Car *car = NULL; engine = new Engine(); car = new Car(engine);
engine->m_f();
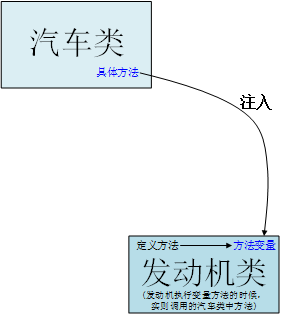
本质:将函数指针作为函数参数传递。类和类的关系是关联,一个类作为另一个类的成员。