SpringBoot文件上传+进度条(thymeleaf)
1. 环境搭建
新建springboot项目,添加web 和 thymeleaf 依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
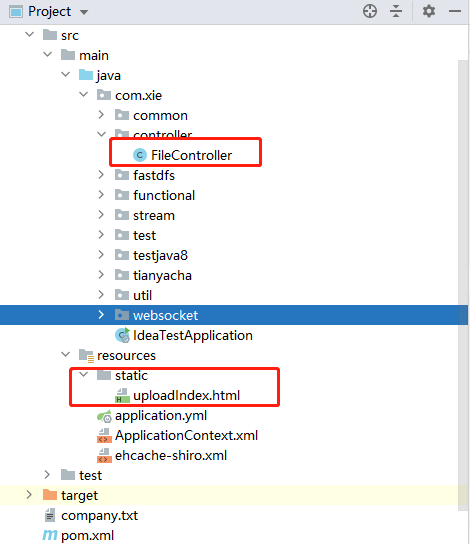
2. 接口编写
package com.xie.controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.UUID;
/**
* @Description
* @Date 2022-05-10 9:36
* @Author xie
*/
@RestController
@RequestMapping("/file")
public class FileController {
private static final String TMP_PATH = "D:/projects/tmp";
@PostMapping("/upload")
public String upload(@RequestParam("uploadFile") MultipartFile file, HttpServletRequest request, HttpServletResponse response) {
if (file.isEmpty()) {
return "文件为空";
}
// 获取当前时间
String currentDate = new SimpleDateFormat("/yyyy/MM/dd").format(new Date());
// 保存文件的目录
String folderPath = TMP_PATH + currentDate;
System.out.println("保存的路径地址:" + folderPath);
// 判断是否需要创建目录
File folderFile = new File(folderPath);
if (!folderFile.exists()) {
folderFile.mkdirs();
}
// 文件名
String fileName = file.getOriginalFilename();
fileName = String.valueOf(UUID.randomUUID()).replace("-", "") + fileName.substring(fileName.lastIndexOf("."));
try {
File destFile = new File(folderFile, fileName);
System.out.println(destFile.getAbsolutePath());
// 核心
file.transferTo(destFile);
String url = request.getScheme() + "://" + request.getServerName() + ":" + request.getServerPort() + "/img" + currentDate + fileName;
System.out.println(String.format("上传成功,图片路径:%s", url));
return "上传成功";
} catch (IOException e) {
return "上传失败";
}
}
}
3. 前端页面
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>文件上传</title>
<style type="text/css">
progress {
background-color: #56BE64;
}
progress::-webkit-progress-bar {
background: #ccc;
}
progress::-webkit-progress-value {
background: #56BE64;
}
percentage {
position: fixed;
left: 160px;
}
</style>
<script src="https://cdn.bootcss.com/jquery/3.4.1/jquery.min.js"></script>
</head>
<body>
<h1>Spring Boot file upload example</h1>
<form id="FileuploadForm" method="POST" action="/file/upload" enctype="multipart/form-data">
<input type="file" name="uploadFile" id="uploadFile"/><br/>
<br/> <input type="button" id="btnUpload" value="上传文件" onclick="upload()"/>
<div id="msg"></div>
</form>
<!--进度条部分(默认隐藏)-->
<div style="display: none;" class="progress-body">
<div>
<span style="width: 100px; display: inline-block; text-align: right">上传进度:</span>
<progress></progress>
<percentage>0%</percentage>
</div>
<div>
<span style="width: 100px; display: inline-block; text-align: right">上传速度:</span>
<span style="width: 300px;" class="progress-speed">0 M/S, 0/0M</span>
</div>
<div>
<span style="width: 100px; display: inline-block; text-align: right">上传状态:</span>
<span style="width: 300px;" class="progress-info">请选择文件并点击上传文件按钮</span>
</div>
</div>
<script>
// 上传文件
function upload() {
$("#msg").text("");
var checkFile = $("#uploadFile").val();
var msgInfo = "";
if (null == checkFile || "" == checkFile) {
$("#msg").text("文件为空,请选择文件!");
} else {
var formData = new FormData(document.getElementById("FileuploadForm"));
$.ajax({
type: "POST",
enctype: 'multipart/form-data',
url: 'file/upload',
data: formData,
cache: false,
processData: false,
contentType: false,
error: function (result) {
console.log("error");
console.log(result);
flag = false;
$("#msg").text("访问服务器错误,请重试!");
},
success: function (result) {
console.log("success");
},
xhr: function () {
var xhr = $.ajaxSettings.xhr();
if (xhr.upload) {
$("#btnUpload").attr("disabled", true);
$("#uploadFile").attr("disabled", true);
//处理进度条的事件
xhr.upload.addEventListener("progress", progressHandle, false);
//加载完成的事件
xhr.addEventListener("load", completeHandle, false);
//加载出错的事件
xhr.addEventListener("error", failedHandle, false);
//加载取消的事件
xhr.addEventListener("abort", canceledHandle, false);
//开始显示进度条
showProgress();
return xhr;
}
}
}, 'json');
}
}
var start = 0;
//显示进度条的函数
function showProgress() {
start = new Date().getTime();
$(".progress-body").css("display", "block");
}
//隐藏进度条的函数
function hideProgress() {
$("#uploadFile").val('');
$('.progress-body .progress-speed').html("0 M/S, 0/0M");
$('.progress-body percentage').html("0%");
$('.progress-body .progress-info').html("请选择文件并点击上传文件按钮");
$("#btnUpload").attr("disabled", false);
$("#uploadFile").attr("disabled", false);
//$(".progress-body").css("display", "none");
}
//进度条更新
function progressHandle(e) {
$('.progress-body progress').attr({value: e.loaded, max: e.total});
var percent = e.loaded / e.total * 100;
var time = ((new Date().getTime() - start) / 1000).toFixed(3);
if (time == 0) {
time = 1;
}
$('.progress-body .progress-speed').html(((e.loaded / 1024) / 1024 / time).toFixed(2) + "M/S, " + ((e.loaded / 1024) / 1024).toFixed(2) + "/" + ((e.total / 1024) / 1024).toFixed(2) + " MB. ");
$('.progress-body percentage').html(percent.toFixed(2) + "%");
if (percent == 100) {
$('.progress-body .progress-info').html("上传完成,后台正在处理...");
} else {
$('.progress-body .progress-info').html("文件上传中...");
}
};
//上传完成处理函数
function completeHandle(e) {
$('.progress-body .progress-info').html("上传文件完成。");
setTimeout(hideProgress, 2000);
};
//上传出错处理函数
function failedHandle(e) {
$('.progress-body .progress-info').html("上传文件出错, 服务不可用或文件过大。");
setTimeout(hideProgress, 2000);
};
//上传取消处理函数
function canceledHandle(e) {
$('.progress-body .progress-info').html("上传文件取消。");
setTimeout(hideProgress, 2000);
};
</script>
</body>
</html>
4. 测试上传
4.1 SpringBoot 默认配置的 最大文件上传大小 和 最大请求大小 都是10MB,为了能够清楚看到进度条效果配置成500MB。
spring:
http:
multipart:
max-file-size: 500MB # -1 代表不限制
max-request-size: 500MB # -1 代表不限制
thymeleaf:
cache: false
encoding: UTF-8
4.2 程序效果
访问:http://localhost:1001/uploadIndex.html(端口根据自己项目修改)
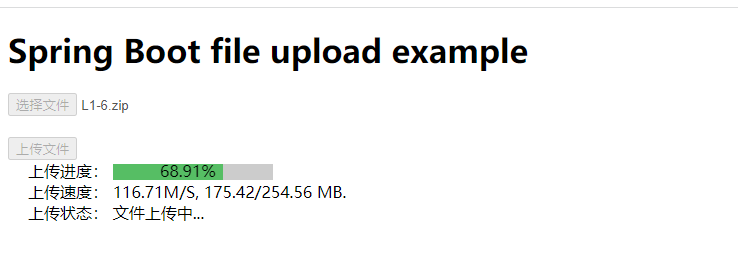
欢迎一起来学习和指导,谢谢关注!
本文来自博客园,作者:xiexie0812,转载请注明原文链接:https://www.cnblogs.com/mask-xiexie/p/16256630.html