1 #include <iostream>
2 #include <stdlib.h>
3 #include <Eigen/Dense>
4 #include <math.h>
5 using namespace std;
6 using Eigen::MatrixXd;
7 MatrixXd extract(char str[])
8 {
9 MatrixXd p(1,2);
10 int u;
11 int i,j;
12 char a[10];
13 for(i=0;i<=49&&str[i]!='(';i++);
14 for(j=0;j<=49&&str[j]!=',';j++);
15 for(u=0;u<=10&&i!=j-1;u++)
16 {
17 i++;
18 a[u]=str[i];
19 }
20
21 p(0,0)=atof(a);
22 for(i=j;i<=49&&str[i]!=')';i++);
23 for(u=0;u<=10&&j!=i-1;u++)
24 {
25 j++;
26 a[u]=str[j];
27 }
28 p(0,1)=atof(a);
29 return p;
30 }
31 int main()
32 {
33 char name[15];
34 int n;
35 double avg,deg;
36 float pi=3.14159265;
37 char str1[20];
38 char str2[20];
39 char str3[20];
40 char str4[15];
41 char str5[15];
42 char str6[15];
43 MatrixXd rot(2,2);
44 MatrixXd p1(1,2);
45 MatrixXd p2(1,2);
46 MatrixXd p3(1,2);
47 MatrixXd p4(1,2);
48 cout<<"请输入 ";
49 cin>>name;
50 cin>>n;
51 switch(n)
52 {
53 case 1:
54 {
55 cin>>str1;
56 p1=extract(str1);
57 }
58 break;
59 case 2:
60 {
61 cin>>str1;
62 p1=extract(str1);
63 cin>>str2;
64 p2=extract(str2);
65 }
66 break;
67 case 3:
68 {
69 cin>>str1;
70 p1=extract(str1);
71 cin>>str2;
72 p2=extract(str2);
73 cin>>str3;
74 p3=extract(str3);
75 }
76 break;
77 default:cout<<"输入错误";
78
79 }
80 cout<<"请输入操作指令"<<endl;
81 cin>>str4;
82 if(str4[0]=='m'&&str4[1]=='o'&&str4[2]=='v'&&str4[3]=='e'&&str4[4]==0)
83 {
84 cin>>str5;
85 cin>>str6;
86 p4=extract(str6);
87 if(n==1)
88 {
89 p1=p1+p4;
90 cout<<"("<<p1<<")"<<endl;
91 }
92 else if(n==2)
93 {
94 p1=p1+p4;
95 p2=p2+p4;
96 cout<<"("<<p1<<")"<<"("<<p2<<")"<<endl;
97 }
98 else
99 {
100 p1=p1+p4;
101 p2=p2+p4;
102 p3=p3+p4;
103 cout<<"("<<p1<<")"<<"("<<p2<<")"<<"("<<p3<<")"<<endl;
104 }
105 }
106 else if(str4[0]=='r'&&str4[1]=='o'&&str4[2]=='t'&&str4[3]=='a'&&str4[4]=='t'&&str4[5]=='e'&&str4[6]==0)
107 {
108 cin>>str5;
109 cin>>avg;
110 deg=avg/180*pi;
111 rot(0,0)=cos(deg);
112 rot(0,1)=sin(deg);
113 rot(1,0)=-sin(deg);
114 rot(1,1)=cos(deg);
115 if(n==1)
116 {
117 p1=p1*rot;
118 cout<<"("<<p1<<")"<<endl;
119 }
120 else if(n==2)
121 {
122 p1=p1*rot;
123 p2=p2*rot;
124 cout<<"("<<p1<<")"<<"("<<p2<<")"<<endl;
125 }
126 else
127 {
128 p1=p1*rot;
129 p2=p2*rot;
130 p3=p3*rot;
131 cout<<"("<<p1<<")"<<"("<<p2<<")"<<"("<<p3<<")"<<endl;
132 }
133
134 }
135 else
136 {
137 cout<<"输入出错"<<endl;
138 }
139 return 0;
140 }

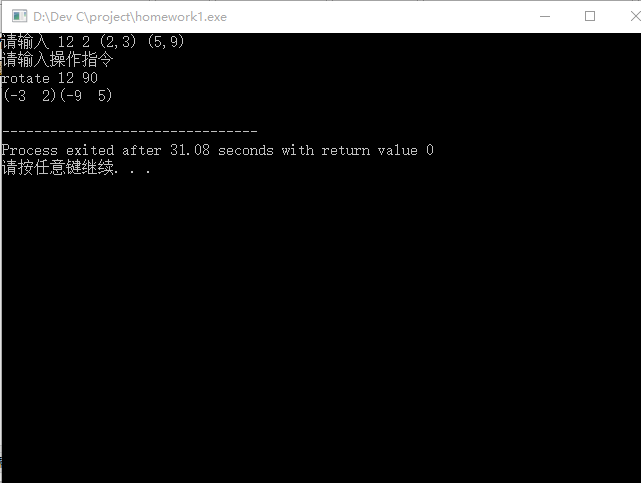
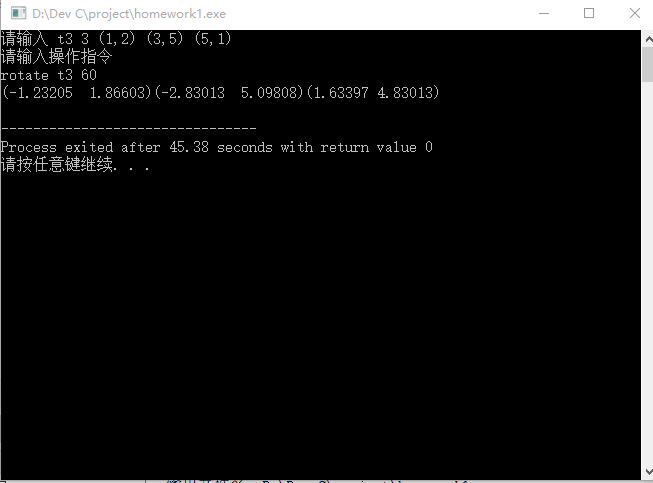