注意!!!!
knife4j需要在application.yml配置文件开启配置
若是resultful请求风格注意注解使用正确
阿里云spring初始化仓库镜像https://start.aliyun.com
一、导入maven坐标
<!--spring相关依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- 数据库驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!-- 数据库连接池 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.4</version>
</dependency>
<!--mybatisPlus-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.1</version>
</dependency>
<!-- 整合mybatis所需要的依赖-->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.3</version>
</dependency>
<!--hutools工具包-->
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.5.1</version>
</dependency>
<!--knife4j测试工具-->
<dependency>
<groupId>com.github.xiaoymin</groupId>
<artifactId>knife4j-openapi2-spring-boot-starter</artifactId>
<version>4.0.0</version>
</dependency>
<!-- validation参数校验 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
<!-- apache校验工具 -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
二、启动类配置
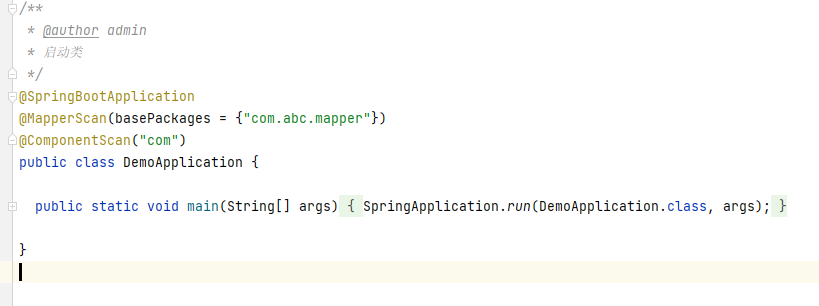
三、配置文件yml
spring:
datasource:
type: com.alibaba.druid.pool.DruidDataSource
username: root
password: 123456
url: jdbc:mysql://localhost:3306/userinfo?useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT
driver-class-name: com.mysql.cj.jdbc.Driver
mvc:
pathmatch:
matching-strategy: ANT_PATH_MATCHER
# 开启sql日志
mybatis-plus:
configuration:
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
mapper-locations: classpath:/mapper/*.xml
mybatis:
type-aliases-package: com.abc.pojo # 别名
mapper-locations: classpath:/mapper/*.xml # xml文件
四、全局异常处理
点击查看代码
自定义业务异常类
@Getter
public class BusinessException extends RuntimeException {
private int code;
private String msg;
public BusinessException() {
this(1001, "接口错误");
}
public BusinessException(String msg) {
this(1001, msg);
}
public BusinessException(int code, String msg) {
super(msg);
this.code = code;
this.msg = msg;
}
public BusinessException(ResultEnum resultEnum, String msg) {
super(msg);
this.code = resultEnum.getCode();
this.msg = msg;
}
public BusinessException(ResultEnum resultEnum) {
this.code = resultEnum.getCode();
this.msg = resultEnum.getMessage();
}
}
------------------------------------------------------------------------------
异常枚举类
public enum ResultEnum {
BODY_NOT_MATCH(400, "请求的数据格式不符!"),
SIGNATURE_NOT_MATCH(401, "请求的数字签名不匹配!"),
NOT_FOUND(404, "未找到该资源!"),
INTERNAL_SERVER_ERROR(500, "服务器内部错误!"),
SERVER_BUSY(503, "服务器正忙,请稍后再试!"),
/**
* 成功
*/
SUCCESS(1000, "成功"),
/**
* 无法找到资源错误
*/
NOT_FOUNT_RESOURCE(1001, "没有找到相关资源!"),
/**
* 请求参数有误
*/
PARAMETER_ERROR(1002, "请求参数有误!"),
/**
* 确少必要请求参数异常
*/
PARAMETER_MISSING_ERROR(1003, "确少必要请求参数!"),
/**
* 确少必要请求参数异常
*/
REQUEST_MISSING_BODY_ERROR(1004, "缺少请求体!"),
/**
* 未知错误
*/
SYSTEM_ERROR(9998, "未知的错误!"),
/**
* 系统错误
*/
UNKNOWN_ERROR(9999, "未知的错误!");
private Integer code;
private String message;
ResultEnum(Integer code, String message) {
this.code = code;
this.message = message;
}
public Integer getCode() {
return code;
}
public String getMessage() {
return message;
}
}
--------------------------------------------------------------
异常处理类
@RestControllerAdvice
public class ExceptionControllerAdvice {
/**
* 异常处理
*
* @param e
* @return
*/
@ResponseBody
@ExceptionHandler(BussinessException.class)
public BaseResult APIExceptionHandler(BussinessException e) {
return BaseResult.error(e.getMsg());
}
@ResponseBody
@ExceptionHandler(Exception.class)
public BaseResult APIExceptionHandler(Exception e) {
return BaseResult.error(ResultEnum.SYSTEM_ERROR.getCode(), e.getMessage());
}
}
---------------------------------------------------
返回统一结果类
@Data
public class Result<T> {
private int code;
private String message;
private T data;
public Result() {
}
public Result(int code, String message) {
this.code = code;
this.message = message;
}
/**
* 成功
*/
public static <T> BaseResult<T> success(String msg, T data) {
return new BaseResult<>(200, msg, data);
}
public static <T> BaseResult<T> success(T data) {
return new BaseResult<>(200, data);
}
/**
* 失败
*/
public static <T> Result<T> error(int code, String message) {
return new Result(code, message);
}
public static <T> BaseResult<T> error(String msg) {
return new BaseResult<>(HttpStatus.HTTP_BAD_REQUEST, msg);
}
}
五、knife4j配置文类
点击查看代码
@Configuration
@EnableSwagger2WebMvc
public class Knife4jConfig {
@Bean
public Docket createRestApi() {
return new Docket(DocumentationType.SWAGGER_2)
.useDefaultResponseMessages(false)
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.basePackage("com.yc.mybatisPlusDemo.controller"))
.paths(PathSelectors.any())
.build();
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.description("后台接口文档")
.version("v1.0")
.title("接口文档")
.build();
}
}
六、mapper映射请求头
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.abc.mapper.UserMapper">
</mapper>
//一对一 association 一对多 collection 多对多collection
<resultMap id="userShopMap" type="com.abc.pojo.User">
<id column="id" property="id"></id>
<result column="name" property="name"></result>
<result column="pwd" property="pwd"></result>
<!-- 一对一-->
<association property="shop" javaType="com.abc.pojo.Shop">
<id column="id" property="id"></id>
<result column="name" property="name"></result>
<result column="price" property="price"></result>
</association>
</resultMap>
七、validation 参数校验使用
单参数校验需在controller上添加@Validated 否则无效
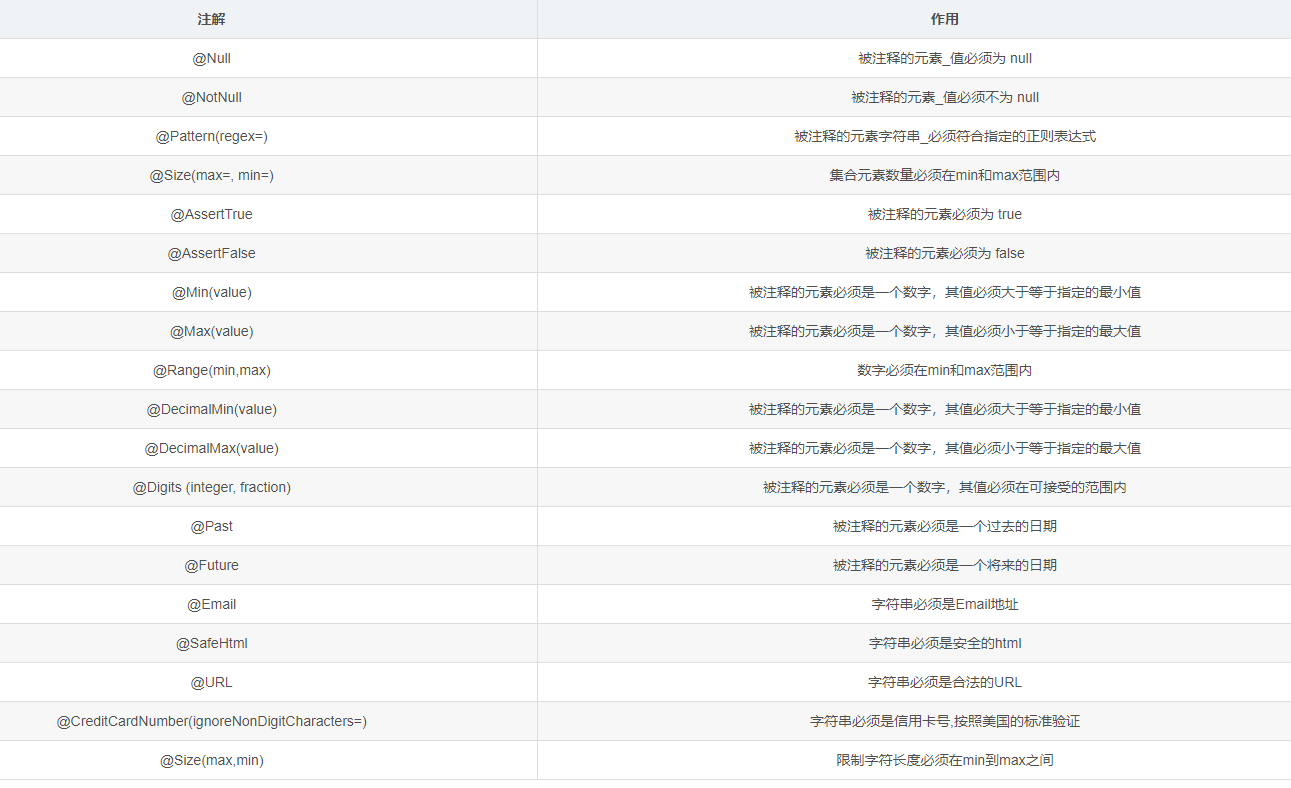