0897. Increasing Order Search Tree (M)
Increasing Order Search Tree (M)
题目
Given the root
of a binary search tree, rearrange the tree in in-order so that the leftmost node in the tree is now the root of the tree, and every node has no left child and only one right child.
Example 1:
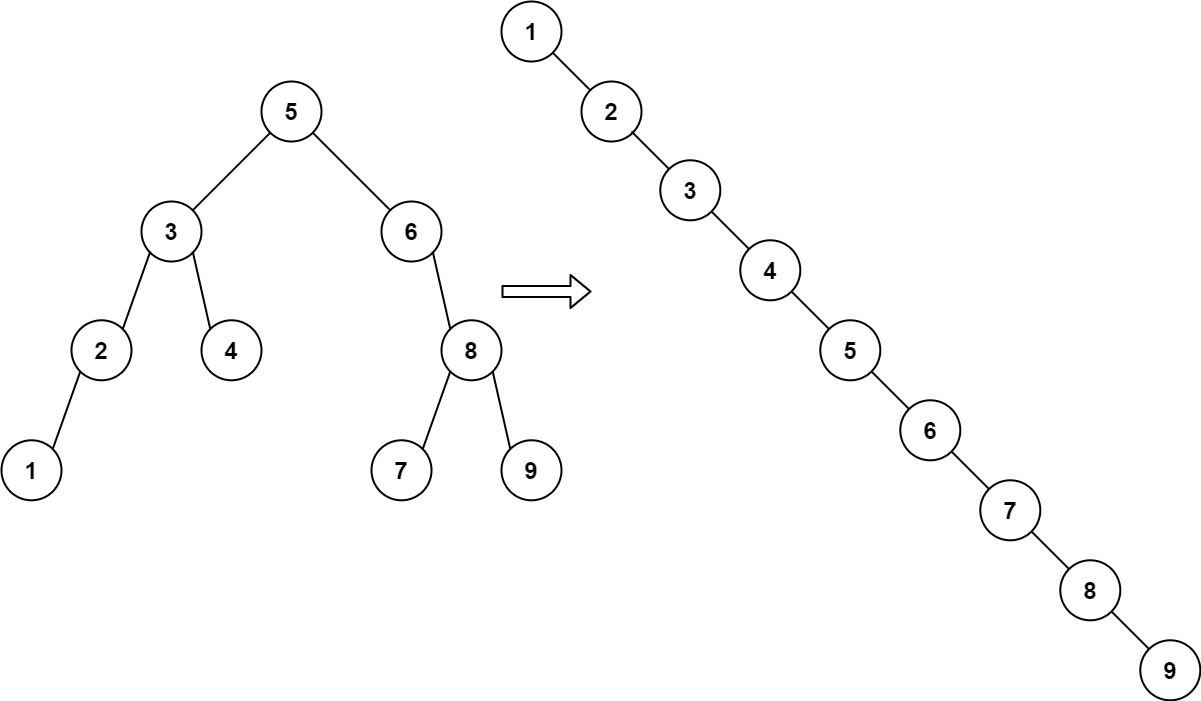
Input: root = [5,3,6,2,4,null,8,1,null,null,null,7,9]
Output: [1,null,2,null,3,null,4,null,5,null,6,null,7,null,8,null,9]
Example 2:
Input: root = [5,1,7]
Output: [1,null,5,null,7]
Constraints:
- The number of nodes in the given tree will be in the range
[1, 100]
. 0 <= Node.val <= 1000
题意
将一个BST按照顺序变成一直链。
思路
简单的可以用中序遍历实现,或者用类似Morris遍历的方法也可以。
代码实现
Java
中序遍历
class Solution {
public TreeNode increasingBST(TreeNode root) {
List<TreeNode> list = new ArrayList<>();
inorder(root, list);
TreeNode dummy = new TreeNode();
TreeNode p = dummy;
for (TreeNode node : list) {
p.right = node;
p = node;
}
return dummy.right;
}
private void inorder(TreeNode root, List<TreeNode> list) {
if (root == null) {
return;
}
inorder(root.left, list);
list.add(root);
root.left = null;
inorder(root.right, list);
}
}
Morris
class Solution {
public TreeNode increasingBST(TreeNode root) {
TreeNode newRoot = root;
while (newRoot != null && newRoot.left != null) {
newRoot = newRoot.left;
}
while (root != null) {
if (root.left != null) {
TreeNode rightMost = root.left;
while (rightMost.right != null) {
rightMost = rightMost.right;
}
rightMost.right = root;
root = root.left;
rightMost.right.left = null;
} else if (root.right != null && root.right.left != null) {
root.right = increasingBST(root.right);
root = root.right;
} else {
root = root.right;
}
}
return newRoot;
}
}