1 ModelSerialzier + ModelViewSet 实现查询
1.1 正向查询
1.1.1 models.py
from django.db import models
from .base import BaseModel
# Create your models here.
class ColorModel(models.Model):
choice = (
('1', '红'),
('2', '橙'),
('3', '黄'),
('4', '绿'),
('5', '蓝'),
('6', '淀'),
('7', '紫'),
)
color_name = models.CharField(max_length=10,choices=choice, default='1')
is_happy = models.CharField(null=True, max_length=30)
class CateModel(BaseModel):
cate_name = models.CharField(max_length=30)
cate_img = models.ImageField(upload_to='cate')
color = models.ForeignKey(to=ColorModel, on_delete=models.CASCADE)
class Meta:
db_table = 'db_cate'
class ProductModel(BaseModel):
product_name = models.CharField(max_length=30)
product_img = models.ImageField(upload_to='product')
cate = models.ForeignKey(to=CateModel, on_delete=models.CASCADE)
class Meta:
db_table = 'db_product'
1.1.2 serializers.py
from rest_framework import serializers
from .models import *
class ColorSerializer(serializers.ModelSerializer):
class Meta:
model = ColorModel
fields = '__all__'
class CateSerializer(serializers.ModelSerializer):
color = ColorSerializer()
class Meta:
model = CateModel
fields = '__all__'
class ProductSerializer(serializers.ModelSerializer):
cate = CateSerializer()
class Meta:
model = ProductModel
fields = '__all__'
1.1.3 views.py
from django.shortcuts import render
# Create your views here.
from rest_framework.viewsets import ModelViewSet
from .serializer import *
class ProductViewSet(ModelViewSet):
queryset = ProductModel.objects.all()
serializer_class = ProductSerializer
1.1.4 urls.py
from django.urls import path, re_path
from .views import *
from rest_framework.routers import DefaultRouter
from . import views
router = DefaultRouter()
router.register(r'product', views.ProductViewSet)
urlpatterns = [
]
urlpatterns += router.urls
1.2 反向查询
1.2.1 模型名+_set 方法
1)models.py
from django.db import models
from .base import BaseModel
# Create your models here.
class ColorModel(models.Model):
choice = (
('1', '红'),
('2', '橙'),
('3', '黄'),
('4', '绿'),
('5', '蓝'),
('6', '淀'),
('7', '紫'),
)
color_name = models.CharField(max_length=10,choices=choice, default='1')
is_happy = models.CharField(null=True, max_length=30)
class CateModel(BaseModel):
cate_name = models.CharField(max_length=30)
cate_img = models.ImageField(upload_to='cate')
color = models.ForeignKey(to=ColorModel, on_delete=models.CASCADE)
class Meta:
db_table = 'db_cate'
class ProductModel(BaseModel):
product_name = models.CharField(max_length=30)
product_img = models.ImageField(upload_to='product')
cate = models.ForeignKey(to=CateModel, on_delete=models.CASCADE)
class Meta:
db_table = 'db_product'
2)serializers.py
from rest_framework import serializers
from .models import *
class ProductSerializer(serializers.ModelSerializer):
class Meta:
model = ProductModel
fields = '__all__'
class CateSerializer(serializers.ModelSerializer):
productmodel_set = ProductSerializer(many=True)
class Meta:
model = CateModel
fields = '__all__'
class ColorSerializer(serializers.ModelSerializer):
catemodel_set = CateSerializer(many=True)
class Meta:
model = ColorModel
fields = '__all__'
3)views.py
from django.shortcuts import render
# Create your views here.
from rest_framework.viewsets import ModelViewSet
from .serializer import *
class ColorViewSet(ModelViewSet):
queryset = ColorModel.objects.all()
serializer_class = ColorSerializer
4)urls.py
from django.urls import path, re_path
from .views import *
from rest_framework.routers import DefaultRouter
from . import views
router = DefaultRouter()
router.register(r'color', views.ColorViewSet)
urlpatterns = [
]
urlpatterns += router.urls
1)models.py
from django.db import models
from .base import BaseModel
# Create your models here.
class ColorModel(models.Model):
choice = (
('1', '红'),
('2', '橙'),
('3', '黄'),
('4', '绿'),
('5', '蓝'),
('6', '淀'),
('7', '紫'),
)
color_name = models.CharField(max_length=10, choices=choice, default='1')
is_happy = models.CharField(null=True, max_length=30)
class CateModel(BaseModel):
cate_name = models.CharField(max_length=30)
cate_img = models.ImageField(upload_to='cate')
color = models.ForeignKey(to=ColorModel, on_delete=models.CASCADE, related_name='cate')
class Meta:
db_table = 'db_cate'
class ProductModel(BaseModel):
product_name = models.CharField(max_length=30)
product_img = models.ImageField(upload_to='product')
cate = models.ForeignKey(to=CateModel, on_delete=models.CASCADE, related_name='product')
class Meta:
db_table = 'db_product'
2)serializers.py
from rest_framework import serializers
from .models import *
class ProductSerializer(serializers.ModelSerializer):
class Meta:
model = ProductModel
fields = '__all__'
class CateSerializer(serializers.ModelSerializer):
product = ProductSerializer(many=True)
class Meta:
model = CateModel
fields = '__all__'
class ColorSerializer(serializers.ModelSerializer):
cate = CateSerializer(many=True)
class Meta:
model = ColorModel
fields = '__all__'
3)views.py
from django.shortcuts import render
# Create your views here.
from rest_framework.viewsets import ModelViewSet
from .serializer import *
class ColorViewSet(ModelViewSet):
queryset = ColorModel.objects.all()
serializer_class = ColorSerializer
4)urls.py
from django.urls import path, re_path
from .views import *
from rest_framework.routers import DefaultRouter
from . import views
router = DefaultRouter()
router.register(r'color', views.ColorViewSet)
urlpatterns = [
]
urlpatterns += router.urls
1.2.3 反向查询注意事项
在写vue页面的时候,使用正向查询查询数据。(反向查询会在v-for里面嵌套v-for,有点复杂哦,有待研究)
1.2.4 反向查询应用场景
一个用户过来访问数据库,比如test1,我们通过用户拿到这个用户对应的blog的对象,通过blog对象拿到这个blog对象的文章分类的对象,然后按照文章分类的id做分组计算,通过跨表查询获取每个分类下的文章。
1.3 效果总览
1.3.1 正向查询
HTTP 200 OK
Allow: GET, POST, HEAD, OPTIONS
Content-Type: application/json
Vary: Accept
[
{
"id": 1,
"cate": {
"id": 4,
"color": {
"id": 7,
"color_name": "紫",
"is_happy": "unhappy"
},
"create_time": "2020-11-06T21:10:51.694838",
"update_time": "2020-11-06T21:10:51.694838",
"cate_name": "法师",
"cate_img": "http://127.0.0.1:8000/media/cate/0_5.jpg"
},
"create_time": "2020-11-06T21:11:15.934364",
"update_time": "2020-11-06T21:11:15.934364",
"product_name": "佐伊",
"product_img": "http://127.0.0.1:8000/media/product/0_5.jpg"
},
{
"id": 2,
"cate": {
"id": 1,
"color": {
"id": 1,
"color_name": "红",
"is_happy": "happy"
},
"create_time": "2020-11-06T21:10:01.463974",
"update_time": "2020-11-06T21:10:01.463974",
"cate_name": "战士",
"cate_img": "http://127.0.0.1:8000/media/cate/0_6.jpg"
},
"create_time": "2020-11-06T21:11:31.347622",
"update_time": "2020-11-06T21:11:31.347622",
"product_name": "锐雯",
"product_img": "http://127.0.0.1:8000/media/product/0_6.jpg"
},
{
"id": 3,
"cate": {
"id": 3,
"color": {
"id": 5,
"color_name": "蓝",
"is_happy": "happy"
},
"create_time": "2020-11-06T21:10:37.399083",
"update_time": "2020-11-06T21:10:37.399083",
"cate_name": "射手",
"cate_img": "http://127.0.0.1:8000/media/cate/0_2.jpg"
},
"create_time": "2020-11-06T21:11:52.642983",
"update_time": "2020-11-06T21:11:52.642983",
"product_name": "霞",
"product_img": "http://127.0.0.1:8000/media/product/0_2.jpg"
},
{
"id": 4,
"cate": {
"id": 2,
"color": {
"id": 4,
"color_name": "绿",
"is_happy": "happy"
},
"create_time": "2020-11-06T21:10:15.177589",
"update_time": "2020-11-06T21:10:15.177589",
"cate_name": "辅助",
"cate_img": "http://127.0.0.1:8000/media/cate/0_1.jpg"
},
"create_time": "2020-11-06T21:12:24.813899",
"update_time": "2020-11-06T21:12:24.813899",
"product_name": "索拉卡",
"product_img": "http://127.0.0.1:8000/media/product/0_1.jpg"
}
]
1.3.2 反向查询
Django REST frameworkmapel
Api Root Color List
Color List
GET /happy/color/
HTTP 200 OK
Allow: GET, POST, HEAD, OPTIONS
Content-Type: application/json
Vary: Accept
[
{
"id": 1,
"cate": [
{
"id": 1,
"product": [
{
"id": 2,
"create_time": "2020-11-06T21:11:31.347622",
"update_time": "2020-11-06T21:11:31.347622",
"product_name": "锐雯",
"product_img": "http://127.0.0.1:8000/media/product/0_6.jpg",
"cate": 1
}
],
"create_time": "2020-11-06T21:10:01.463974",
"update_time": "2020-11-06T21:10:01.463974",
"cate_name": "战士",
"cate_img": "http://127.0.0.1:8000/media/cate/0_6.jpg",
"color": 1
}
],
"color_name": "红",
"is_happy": "happy"
},
{
"id": 2,
"cate": [],
"color_name": "橙",
"is_happy": "unhappy"
},
{
"id": 3,
"cate": [],
"color_name": "黄",
"is_happy": "happy"
},
{
"id": 4,
"cate": [
{
"id": 2,
"product": [
{
"id": 4,
"create_time": "2020-11-06T21:12:24.813899",
"update_time": "2020-11-06T21:12:24.813899",
"product_name": "索拉卡",
"product_img": "http://127.0.0.1:8000/media/product/0_1.jpg",
"cate": 2
}
],
"create_time": "2020-11-06T21:10:15.177589",
"update_time": "2020-11-06T21:10:15.177589",
"cate_name": "辅助",
"cate_img": "http://127.0.0.1:8000/media/cate/0_1.jpg",
"color": 4
}
],
"color_name": "绿",
"is_happy": "happy"
},
{
"id": 5,
"cate": [
{
"id": 3,
"product": [
{
"id": 3,
"create_time": "2020-11-06T21:11:52.642983",
"update_time": "2020-11-06T21:11:52.642983",
"product_name": "霞",
"product_img": "http://127.0.0.1:8000/media/product/0_2.jpg",
"cate": 3
}
],
"create_time": "2020-11-06T21:10:37.399083",
"update_time": "2020-11-06T21:10:37.399083",
"cate_name": "射手",
"cate_img": "http://127.0.0.1:8000/media/cate/0_2.jpg",
"color": 5
}
],
"color_name": "蓝",
"is_happy": "happy"
},
{
"id": 6,
"cate": [],
"color_name": "靛",
"is_happy": "happy"
},
{
"id": 7,
"cate": [
{
"id": 4,
"product": [
{
"id": 1,
"create_time": "2020-11-06T21:11:15.934364",
"update_time": "2020-11-06T21:11:15.934364",
"product_name": "佐伊",
"product_img": "http://127.0.0.1:8000/media/product/0_5.jpg",
"cate": 4
}
],
"create_time": "2020-11-06T21:10:51.694838",
"update_time": "2020-11-06T21:10:51.694838",
"cate_name": "法师",
"cate_img": "http://127.0.0.1:8000/media/cate/0_5.jpg",
"color": 7
}
],
"color_name": "紫",
"is_happy": "unhappy"
}
]
2 ModelViewSet + ModelSerializer实现查询
2.1 Django端
2.1.1 models.py
from django.db import models
from .base import BaseModel
# Create your models here.
class ColorModel(models.Model):
choice = (
('1', '红'),
('2', '橙'),
('3', '黄'),
('4', '绿'),
('5', '蓝'),
('6', '淀'),
('7', '紫'),
)
color_name = models.CharField(max_length=10, choices=choice, default='1')
is_happy = models.CharField(null=True, max_length=30)
class CateModel(BaseModel):
cate_name = models.CharField(max_length=30)
cate_img = models.ImageField(upload_to='cate')
color = models.ForeignKey(to=ColorModel, on_delete=models.CASCADE, related_name='cate')
class Meta:
db_table = 'db_cate'
class ProductModel(BaseModel):
product_name = models.CharField(max_length=30)
product_img = models.ImageField(upload_to='product')
cate = models.ForeignKey(to=CateModel, on_delete=models.CASCADE, related_name='product')
class Meta:
db_table = 'db_product'
2.1.2 serializers.py
from rest_framework import serializers
from .models import *
class ColorSerializer(serializers.ModelSerializer):
class Meta:
model = ColorModel
fields = '__all__'
class CateSerializer(serializers.ModelSerializer):
color = ColorSerializer()
class Meta:
model = CateModel
fields = '__all__'
class ProductSerializer(serializers.ModelSerializer):
cate = CateSerializer()
class Meta:
model = ProductModel
fields = '__all__'
2.1.3 views.py
from django.shortcuts import render
# Create your views here.
from rest_framework.viewsets import ModelViewSet
from .serializer import *
class ProductViewSet(ModelViewSet):
queryset = ProductModel.objects.all()
serializer_class = ProductSerializer
2.1.4 urls.py
from django.urls import path, re_path
from .views import *
from rest_framework.routers import DefaultRouter
from . import views
router = DefaultRouter()
router.register(r'product', views.ProductViewSet)
urlpatterns = [
]
urlpatterns += router.urls
2.2 Vue端
2.2.1 Color.vue
<template>
<div>
<table style="margin: 0 auto">
<tr>
<th>id</th>
<th>color</th>
<th>cate</th>
<th>name</th>
<th>img</th>
</tr>
<tr v-for="(co, item) in product_list" :key="item">
<td>{{co.id}}</td>
<td>{{co.product_name}}</td>
<td>{{co.cate.cate_name}}</td>
<td>{{co.cate.color.color_name}}</td>
<td><img :src="co.product_img" style="width:100px"></td>
</tr>
</table>
<p>
输入商品的id:<input type="text" v-model="find_id" >
</p>
<p>
<button @click="findColor">查询结果显示</button>{{find_list}}
</p>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
base_url: 'http://127.0.0.1:8000',
product_list: [],
find_id:'',
find_list:[]
}
},
methods: {
getColor(){
axios(
{
url:this.base_url + '/happy/product/'
}
).then(res=>{
this.product_list = res.data
console.log(res.data)
})
},
findColor(){
// let p = {'id':this.find_id}
// aixos封装时,用字典传递
axios({
// url:this.base_url + '/happy/product/'+p.id,
// aixos封装时,取字典中的值
url:this.base_url + '/happy/product/'+this.find_id,
}).then(res=>{
console.log(res.data)
this.find_list = res.data
})
},
// 根据名称查询的时候,直接调用这个接口
// http://127.0.0.1:8000/happy/product/?color_name='紫'&color_name='红'
},
created() {
this.getColor()
}
}
</script>
<style scoped>
</style>
2.2.2 index.js
import Vue from 'vue'
import Router from 'vue-router'
import HelloWorld from '@/components/HelloWorld'
import Color from '@/components/Color'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'HelloWorld',
component: HelloWorld
},
{
path: '/color',
name: 'Color',
component: Color
}
]
})
2.3 实现效果
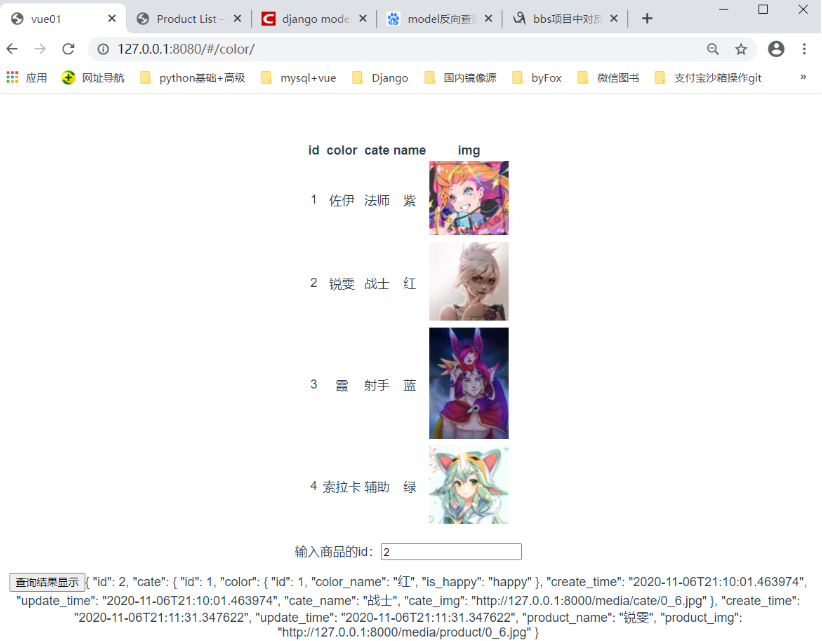
3 ModelViewSet + ModelSerializer实现删除
- ModelViewSet 实现的是物理删除,如果需要逻辑删除来写业务逻辑接口,需要继承APIView
3.1 Django端
3.1.1 models.py
from django.db import models
from .base import BaseModel
# Create your models here.
class ColorModel(models.Model):
choice = (
('1', '红'),
('2', '橙'),
('3', '黄'),
('4', '绿'),
('5', '蓝'),
('6', '淀'),
('7', '紫'),
)
color_name = models.CharField(max_length=10, choices=choice, default='1')
is_happy = models.CharField(null=True, max_length=30)
class CateModel(BaseModel):
cate_name = models.CharField(max_length=30)
cate_img = models.ImageField(upload_to='cate')
color = models.ForeignKey(to=ColorModel, on_delete=models.CASCADE, related_name='cate')
class Meta:
db_table = 'db_cate'
class ProductModel(BaseModel):
product_name = models.CharField(max_length=30)
product_img = models.ImageField(upload_to='product')
cate = models.ForeignKey(to=CateModel, on_delete=models.CASCADE, related_name='product')
class Meta:
db_table = 'db_product'
3.1.2 serializers.py
from rest_framework import serializers
from .models import *
class ColorSerializer(serializers.ModelSerializer):
class Meta:
model = ColorModel
fields = '__all__'
class CateSerializer(serializers.ModelSerializer):
color = ColorSerializer()
class Meta:
model = CateModel
fields = '__all__'
class ProductSerializer(serializers.ModelSerializer):
cate = CateSerializer()
class Meta:
model = ProductModel
fields = '__all__'
3.1.3 views.py
from django.shortcuts import render
# Create your views here.
from rest_framework.viewsets import ModelViewSet
from .serializer import *
class ProductViewSet(ModelViewSet):
queryset = ProductModel.objects.all()
serializer_class = ProductSerializer
3.1.4 urls.py
from django.urls import path, re_path
from .views import *
from rest_framework.routers import DefaultRouter
from . import views
router = DefaultRouter()
router.register(r'product', views.ProductViewSet)
urlpatterns = [
]
urlpatterns += router.urls
3.2 Vue端
3.2.1 Color.vue
<template>
<div>
<table style="margin: 0 auto">
<tr>
<th>id</th>
<th>color</th>
<th>cate</th>
<th>name</th>
<th>img</th>
<th>操作</th>
</tr>
<tr v-for="(co, item) in product_list" :key="item">
<td>{{co.id}}</td>
<td>{{co.product_name}}</td>
<td>{{co.cate.cate_name}}</td>
<td>{{co.cate.color.color_name}}</td>
<td><img :src="co.product_img" style="width:100px"></td>
<td>
<button @click="delColor(co.id)">删除</button>
</td>
</tr>
</table>
<p>
输入商品的id:<input type="text" v-model="find_id" >
</p>
<p>
<button @click="findColor">查询结果显示</button>{{find_list}}
</p>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
base_url: 'http://127.0.0.1:8000',
product_list: [],
find_id:'',
find_list:[]
}
},
methods: {
getColor(){
axios(
{
url:this.base_url + '/happy/product/'
}
).then(res=>{
this.product_list = res.data
console.log(res.data)
})
},
findColor(){
// let p = {'id':this.find_id}
// aixos封装时,用字典传递
axios({
// url:this.base_url + '/happy/product/'+p.id,
// aixos封装时,取字典中的值
url:this.base_url + '/happy/product/'+this.find_id,
}).then(res=>{
console.log(res.data)
this.find_list = res.data
})
},
// 根据名称查询的时候,直接调用这个接口
// http://127.0.0.1:8000/happy/product/?color_name='紫'&color_name='红'
delColor(c){
axios({
url:this.base_url + '/happy/product/' + c,
method: 'delete'
}).then(res=>{
console.log(res)
this.getColor()
})
}
},
created() {
this.getColor()
}
}
</script>
<style scoped>
</style>
3.2.2 index.js
import Vue from 'vue'
import Router from 'vue-router'
import HelloWorld from '@/components/HelloWorld'
import Color from '@/components/Color'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'HelloWorld',
component: HelloWorld
},
{
path: '/color',
name: 'Color',
component: Color
}
]
})
3.3 实现效果
204 请求收到,但返回信息为空
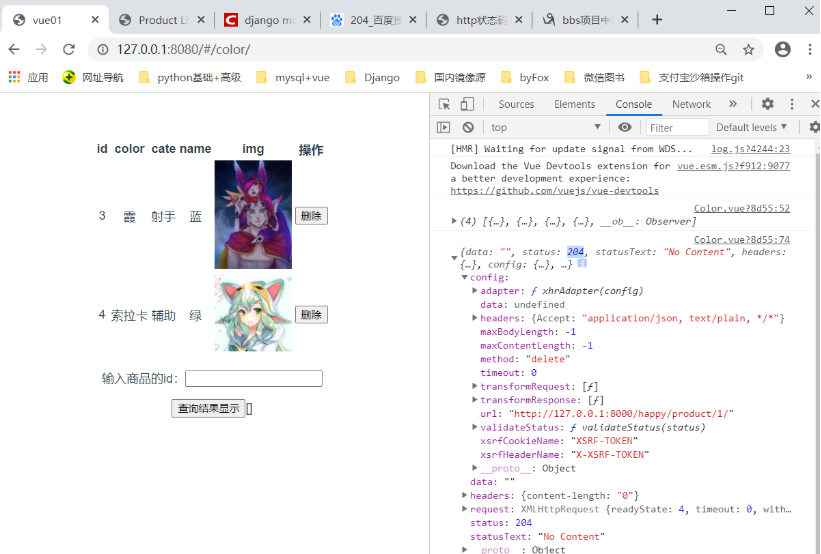