实验四 编码裁剪算法
一、实验目的和要求
1.了解二维图形裁剪的原理(点的裁剪、直线的裁剪、多边形的裁剪),利用VC+OpenGL实现直线的裁剪算法。
二、实验内容及主要步骤代码
(1) 理解直线裁剪的原理(Cohen-Surtherland算法、梁友栋算法)
(2) 利用VC+OpenGL实现直线的编码裁剪算法,在屏幕上用一个封闭矩形裁剪任意一条直线。
(3) 调试、编译、修改程序。
主要步骤代码:
(1)源代码:
oid LineGL(int x0,int y0,int x1,int y1){
glBegin(GL_LINES);
glColor3f(1.0f,0.0f,0.0f);
glVertex2f(x0,y0);
glColor3f(0.0f,1.0f,0.0f);
glVertex2f(x1,y1);
glEnd();
}
int x0,x1,y0,y1;
struct Rectangle{
float xmin,xmax,ymin,ymax;
};
Rectangle rect;
int CompCode(int x,int y,Rectangle rect){
int code = 0x00;
if(y<rect.ymin)
code = code|4;
if(y>rect.ymax)
code = code|8;
if(x>rect.xmax)
code = code|2;
if(x<rect.xmin)
code = code|1;
return code;
}
int cohensutherlandlineclip(Rectangle rect,int &x0,int &y0,int &x1,int &y1){
int accept,done;
float x,y;
accept = 0;
done = 0;
int code0,code1,codeout;
code0 = CompCode(x0,y0,rect);
code1 = CompCode(x1,y1,rect);
do{
if(!(code0|code1)){
accept = 1;
done = 1;
}else if(code0 & code1){
done = 1;
}else{
if(code0!=0)
codeout = code0;
else
codeout = code1;
if(codeout&LEFT_EDGE){
y = y0+(y1-y0)*(rect.xmin - x0)/(x1 - x0);
x = (float)rect.xmin;
}else if(codeout&RIGHT_EDGE){
y = y0+(y1-y0)*(rect.xmax - x0)/(x1 - x0);
x = (float)rect.xmax;
}else if(codeout&BOTTOM_EDGE){
x = x0+(x1-x0)*(rect.ymin - y0)/(y1 - y0);
y = (float)rect.ymin;
}else if(codeout&TOP_EDGE){
x = x0+(x1-x0)*(rect.ymax - y0)/(y1 - y0);
y = (float)rect.ymax;
}
if(codeout == code0){
x0 = x; y0 = y;
code0 = CompCode(x0,y0,rect);
}else{
x1 = x;y1 = y;
code1 = CompCode(x1,y1,rect);
}
}
}while(!done);
if(accept)
LineGL(x0,y0,x1,y1);
return accept;
}
(2)运行结果:
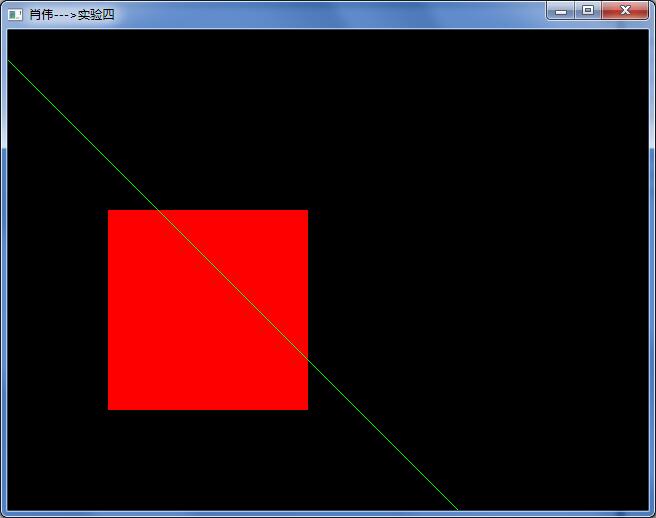
