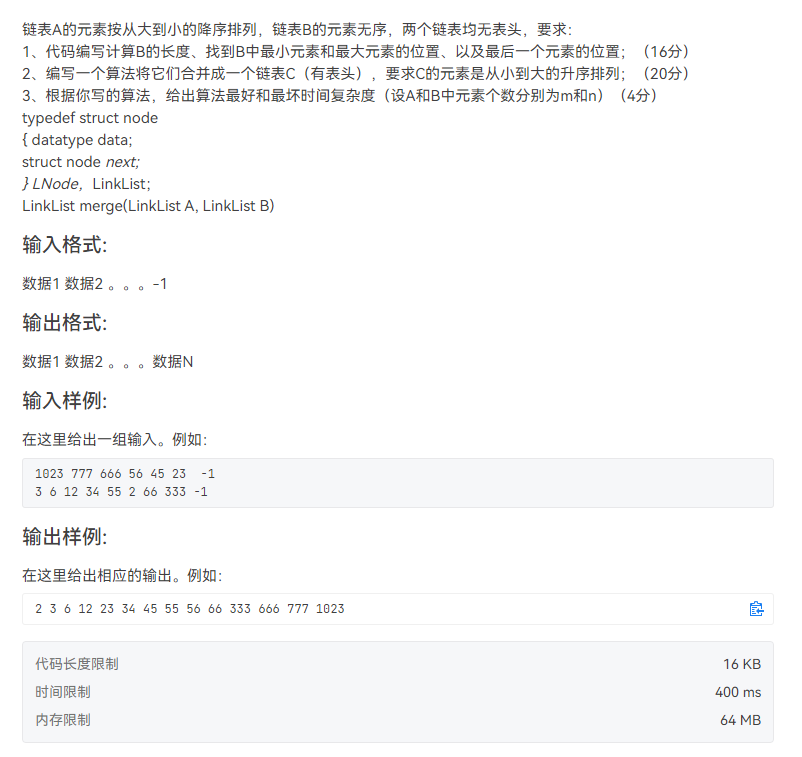
#include<stdio.h>
#include<stdlib.h>
typedef int datatype;
typedef struct LNode
{
datatype data;
LNode *next;
}LNode,*LinkList;
LNode* init_LinkList();
int CreateList(LNode* head);
void display(LinkList L);
int listLength_non(LNode* list);
LNode* getMax(LNode *list);
LNode* getMin(LNode *list);
LNode *getLast(LNode *list);
LNode* mergeList(LNode* head1, LNode* head2);
LNode* toSortList(LNode* head, LNode* tail);
LNode* sortList(LNode* head);
int main()
{
LNode *LA=init_LinkList();LNode *LB=init_LinkList();LNode *LC=init_LinkList();
CreateList(LA);
CreateList(LB);
LC=mergeList(LA,LB);
printf("链表B的长度是%d",listLength_non(LB)-1);
printf("\n最大元素的位置%d max=%d,最小元素的位置%d min=%d\n", getMax(LB),getMax(LB)->data, getMin(LB),getMin(LB)->data);
printf("最后一个元素的位置是%d值为%d\n",getLast(LB),getLast(LB)->data);
display(sortList(LC->next));
return 0;
}
LNode* init_LinkList()
{
LNode* head =(LNode*)malloc(sizeof(LNode));//head是头指针,指向头结点
head->next =NULL; //头结点的指针域为空,即首结点的地址为空
return head; //返回头指针,因为只要确定了头指针,就确定了一个链表
}
int CreateList(LNode* head)
{
LNode* move =head;//目的是让头结点一直不动,move一直指向尾结点
LNode* newnode; //指向新结点
datatype data;
while (1)
{
scanf("%d",&data);
if(data==-1)break;
else
{
newnode =(LNode*)malloc(sizeof(LNode));
newnode->data =data;
move->next =newnode;
move =newnode;
}
}
move->next =NULL;//因为move一直指向尾结点,而尾结点的指针域为空
}
LNode *getLast(LNode *list)
{
LNode *p;
p=list; //L就是头节点
while(p->next!=NULL)
p=p->next;
return p;
}
void display(LinkList L)
{
LNode *p=init_LinkList();
p=L;
while(p->next)
{
printf("%d ",p->data);
p=p->next;
}
}
int listLength_non(LNode* list)
{
int n = 0;
while(list)
{
n++;
list = list->next;
}
return n;
}
LNode* getMax(LNode *list)
{
LNode *temp=list->next;
LNode *max=temp;
while(temp!=NULL)
{
if(max->data<temp->data)max=temp;
temp=temp->next;
}
return max ;
}
LNode* getMin(LNode *list)
{
LNode *temp=list->next;
LNode *min=temp;
while(temp!=NULL)
{
if(min->data>temp->data)min=temp;
temp=temp->next;
}
return min ;
}
LNode* mergeList(LNode* head1, LNode* head2) {
LNode* dummyHead = init_LinkList();
dummyHead->data = 0;
LNode *temp = dummyHead, *temp1 = head1, *temp2 = head2;
while (temp1 != NULL && temp2 != NULL) {
if (temp1->data <= temp2->data) {
temp->next = temp1;
temp1 = temp1->next;
} else {
temp->next = temp2;
temp2 = temp2->next;
}
temp = temp->next;
}
if (temp1 != NULL) {
temp->next = temp1;
} else if (temp2 != NULL) {
temp->next = temp2;
}
return dummyHead->next;
}
LNode* toSortList(LNode* head, LNode* tail) {
if (head == NULL) {
return head;
}
if (head->next == tail) {
head->next = NULL;
return head;
}
LNode *slow = head, *fast = head;
while (fast != tail) {
slow = slow->next;
fast = fast->next;
if (fast != tail) {
fast = fast->next;
}
}
LNode* mid = slow;
return mergeList(toSortList(head, mid), toSortList(mid, tail));
}
LNode* sortList(LNode* head) {
return toSortList(head, NULL);
}
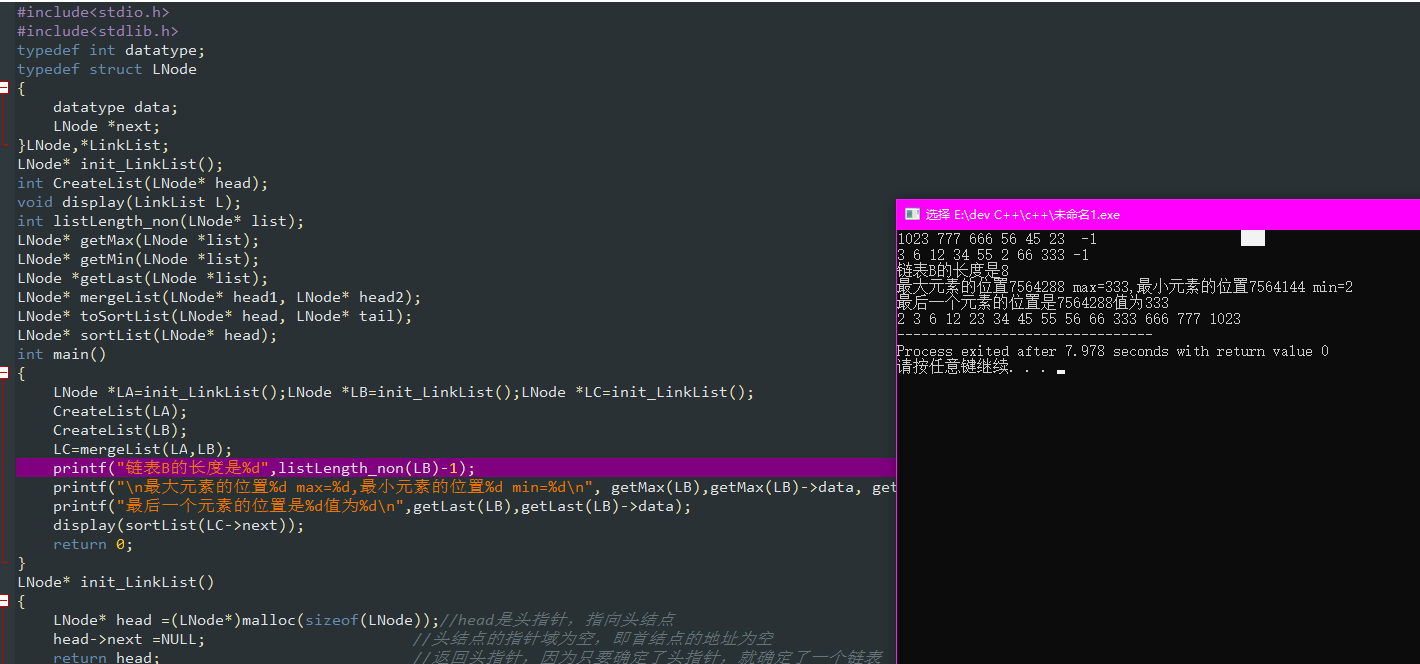