POJ 2299 Ultra-QuickSort
Ultra-QuickSort
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 32626 | Accepted: 11642 |
Description
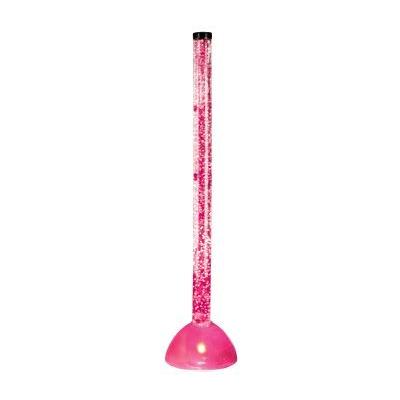
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
给一组数,求这组数的逆序数
开始直接用了线性代数里讲的方法,自己估算了一下O(n^2),感觉要跪,试着交了一下,果然跪了
百度后发现此题可以用归并排序做,每次merge的时候,当从后半部分取数时,就把当前前半部分剩余数的个数加到ans中去
这题给我最大的启示就是,每个排序算法都有其奇妙的用处,不能只会快排

1 #include<iostream> 2 3 using namespace std; 4 5 long long ans; 6 long a[500000],b[500000]; 7 8 void Merge(long low,long mid,long high) 9 { 10 long i=low,j=mid+1,k=low,end_1=mid,end_2=high; 11 while(i<=end_1&&j<=end_2) 12 { 13 b[k++]=(a[i]<=a[j]?a[i++]:(ans+=mid-i+1,a[j++])); 14 } 15 while(i<=end_1) 16 b[k++]=a[i++]; 17 while(j<=end_2) 18 b[k++]=a[j++]; 19 for(i=low;i<=high;i++) 20 a[i]=b[i]; 21 } 22 23 void Sort(long p,long q) 24 { 25 if(p==q) 26 return; 27 long mid=(p+q)/2; 28 Sort(p,mid); 29 Sort(mid+1,q); 30 Merge(p,mid,q); 31 } 32 33 int main() 34 { 35 long n; 36 37 while((cin>>n)&&n) 38 { 39 for(int i=0;i<n;i++) 40 cin>>a[i]; 41 ans=0; 42 Sort(0,n-1); 43 cout<<ans<<endl; 44 } 45 46 return 0; 47 }