Android 登录注册-SharedPreferences-记住密码
一、概述及运行结果
注册:输入用户名、密码,验证用户名是否已存在,若存在提示 ” 用户名已存在 “,若不存在将用户名密码存储到 内部存储的 SharedPrederences 中,并重新登录。
登录:1. 检查用户名密码是否正确,若不正确提示 ” 用户名密码不正确 ” ,若正确则跳转至其他界面,并销毁登录界面。2. 检查复选框是否勾选,并改变 SharedPrederences 中复选框的状态值。
初始登录界面:用户名会显示为上次登录的用户名,若上次复选框勾选,密码自动填充且复选框状态设置为已勾选。
二、代码实现
1. 登录注册界面
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:layout_marginTop="200dp"> <TextView android:text="账号:" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:textSize="20dp" android:layout_marginLeft="70dp"/> <EditText android:id="@+id/editText_account" android:layout_width="200sp" android:layout_height="wrap_content"/> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <TextView android:text="密码:" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:textSize="20dp" android:layout_marginLeft="70dp"/> <EditText android:id="@+id/editText_password" android:inputType="textPassword" android:layout_width="200sp" android:layout_height="wrap_content"/> </LinearLayout> <CheckBox android:id="@+id/checkBox_remember_password" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="记住密码" android:layout_marginLeft="70dp"/> <Button android:id="@+id/button_login" android:layout_width="150sp" android:layout_height="wrap_content" android:text="登陆" android:layout_gravity="center" android:layout_marginTop="20dp"/> <Button android:id="@+id/button_register" android:layout_width="150sp" android:layout_height="wrap_content" android:text="注册" android:layout_gravity="center" android:layout_marginTop="10dp"/> </LinearLayout>
2. onCreate 方法,同时也是在设置初始登录界面
//控件 private EditText accountEditText ; private EditText passwordEditText; private CheckBox rememberPassCheckBox; private Button loginButton; private Button registerButton; // SharedPreferences 的文件名 final String LOGIN_SHAREDPREFERENCES_NAME = "LoginInfo";
protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_login); // 获取控件 initComponent(); // 获取上一次登录的人,将数据显示在 accountEditText 中 accountEditText.setText(getLastLoginAccount()); //如果上次勾选了复选框,则同时将密码进行显示 if(getIsRemenberPassword()){ passwordEditText.setText(getPassword(accountEditText.getText().toString())); rememberPassCheckBox.setChecked(getIsRemenberPassword()); } // 添加监听 loginButton.setOnClickListener(this); registerButton.setOnClickListener(this); } /** * 获取控件 */ private void initComponent(){ accountEditText = (EditText)findViewById(R.id.editText_account); passwordEditText = (EditText)findViewById(R.id.editText_password); rememberPassCheckBox = (CheckBox)findViewById(R.id.checkBox_remember_password); loginButton = (Button)findViewById(R.id.button_login); registerButton = (Button)findViewById(R.id.button_register); }
3. 给按钮添加监听,同时也是在设置登录注册流程(Activity 记得 实现 View.onClickListener 接口)
@Override public void onClick(View view) { int btnId = view.getId(); if(btnId == R.id.button_login){ // 检查是否成功 // 获取用户名密码 String account = accountEditText.getText().toString(); String password = passwordEditText.getText().toString(); // 检验是否登录成功 boolean isLoginSucceed = checkPassword(account,password); // 根据复选框是否被选择,改变IsRemenberPassword setIsRemenberPassword(rememberPassCheckBox.isChecked()); // 根据登录结果做出反应 loginResultAction(isLoginSucceed,account); }else if(btnId == R.id.button_register){ // 获取用户名密码 String account = accountEditText.getText().toString(); String password = passwordEditText.getText().toString(); // 获取 account 对应的密码,若为 "" 则说明之前没有该用户名 String realPassword = getPassword(account); if(realPassword.length() != 0){ Toast.makeText(this,"用户名已存在",Toast.LENGTH_SHORT).show(); }else{ Toast.makeText(this,"注册成功,请重新登录",Toast.LENGTH_SHORT).show(); // 将用户名密码存进内存 writeAccountPassword(account,password); // 注册成功后设置上一次登录用户为注册用户,并将密码框设置为 空 setLastLoginAccount(account); passwordEditText.setText(""); // 根据复选框是否被选择,改变IsRemenberPassword setIsRemenberPassword(rememberPassCheckBox.isChecked()); } } }
4. 对 SharedPreferences 的读写操作,也是上面方法中使用到的成员方法
/** * 得到上一次登录的人,若上一次没有人登录,返回 "" * @return */ private String getLastLoginAccount(){ // 获取 SharedPreferences 对象 SharedPreferences shared = getSharedPreferences(LOGIN_SHAREDPREFERENCES_NAME,MODE_PRIVATE); // 从 SharedPreferences 中读取数据 return shared.getString("last_login_account",""); } /** * 设置上一次登录的人 * @param account */ private void setLastLoginAccount(String account){ // 获取 SharedPreferences 对象 SharedPreferences shared = getSharedPreferences(LOGIN_SHAREDPREFERENCES_NAME,MODE_PRIVATE); // 获取 SharedPreferences.Editor 对象 SharedPreferences.Editor editor = shared.edit(); // 操作 editor.putString("last_login_account",account); // 提交 editor.apply(); }
/** * 得到上一次复选框是否勾选 * @return */ private boolean getIsRemenberPassword(){ // 获取 SharedPreferences 对象 SharedPreferences shared = getSharedPreferences(LOGIN_SHAREDPREFERENCES_NAME,MODE_PRIVATE); // 从 SharedPreferences 中读取数据 return shared.getBoolean("isRememberPassword",false); } /** * 设置上一次复选框是否勾选 * @param isRemenberPassword */ private void setIsRemenberPassword(boolean isRemenberPassword){ // 获取 SharedPreferences 对象 SharedPreferences shared = getSharedPreferences(LOGIN_SHAREDPREFERENCES_NAME,MODE_PRIVATE); // 获取 SharedPreferences.Editor 对象 SharedPreferences.Editor editor = shared.edit(); // 操作 editor.putBoolean("isRememberPassword",isRemenberPassword); // 提交 editor.apply(); }
/** * 根据 account 从 内存中获取密码,若没有该用户,返回 "" */ private String getPassword(String account){ // 从内部存储中获取 account 的密码 // 获取 SharedPreferences 对象 SharedPreferences shared = getSharedPreferences(LOGIN_SHAREDPREFERENCES_NAME,MODE_PRIVATE); // 从 SharedPreferences 中读取数据 return shared.getString(account,""); } /** * 将用户名密码写入内存中 */ private void writeAccountPassword(String accout,String password){ SharedPreferences shared = getSharedPreferences(LOGIN_SHAREDPREFERENCES_NAME,MODE_PRIVATE); SharedPreferences.Editor editor = shared.edit(); editor.putString(accout,password); editor.apply(); }
/** * 验证输入的用户名密码是否错误 */ private boolean checkPassword(String account,String password){ String realPassword = getPassword(account); // 对比密码 if (realPassword.length() != 0 && realPassword.equals(password)) { return true; } return false; }
/** * 根据登录/注册是否成功,做出相应操作 */ private void loginResultAction(boolean isLoginSucceed,String account){ if(isLoginSucceed){ setLastLoginAccount(account); Intent intent = new Intent(LoginActivity.this,ControlActivity.class); startActivity(intent); this.finish(); }else{ Toast.makeText(this,"用户名或密码错误",Toast.LENGTH_SHORT).show(); } }
三、SharedPreferences 存储基础知识
1. SharedPreferences 是一个轻量级的存储类,特别适合保存配置信息(例如是否记住密码,是否自动启动,用户特定信息等)
2. SharedPreferences 使用 key-value 的方式存储数据。根据 key 值获取 value 值
3. SharedPreferences 支持多种不同数据类型的存储,可以是 String,int,boolean 等,以下几种,不支持自定义类型。
4. SharedPreferences 以 xml 文件存储数据,默认放在 内部存储中,文件放在
/data/data/<package name>/shared_prefs
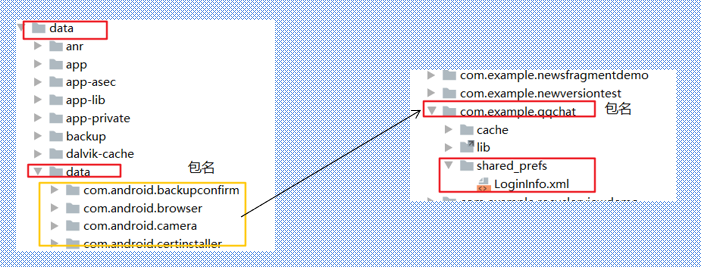
四、使用 SharedPreferences
1. 获取 SharedPreferences 对象
1. Context.getSharedPreferences(arg0,arg1); arg0 : 指定 SharedPreferences 文件的名称,如果不存在会自动创建。 arg1 : 指定操作模式,只要为 MODE_PRIVATE(默认模式)和 MODE_MULTI_PROCESS(多进程读写) 2. Activity 类中的 getPreferences(arg0); arg0 : 操作模式参数 这个方法会自动将当前活动的类名作为 SharedPreferences 的文件名 3. PreferenceManager 类中的 getDefaultSharedPreferences(arg0) 方法 arg0 : Context 参数 静态方法,自动使用当前应用的包名作为前缀来命名 SharedPreferences 文件。
2. 将数据存储到 SharedPreferences 中。
使用 SharedPreferences 存储数据时,获得 SharedPreferences 对象后,必须获得该对象的 Editor 对象,通过 Editor 对象的相关方法存储数据。
1. 获取 SharedPreferences.Editor 对象
SharedPreferences shared = getSharedPreferences("data",MODE_PRIVATE); SharedPreferences.Editor = shared.edit(); 2. 以键值对的方式存储数据 editor.putString("name","Nane"); editor.putInt("age",12); editor.putBoolean("married",false); 3. 提交数据 editor.commit(); 或 editor.apply();
程序运行以后,在 /data/data/<包名>/shared_prefs/ 目录下生辰了一个 datalxml 文件,内容如下:
<?xml version='1.0' encoding='utf-8' standalone='yes' ?> <map> <boolean name="married" value="false" /> <string name="name">Nana</string> <int name="age" value="12" /> </map>
3. 从 SharedPreferences 读取数据
直接使用 SharedPreferences 对象提供的一系列 get 方法即可,每一种 get 方法对应 Editor 中一种 put 方法。
XXX value = shared.getXXX(arg0,arg1); arg0 : Key 值 arg1 : 该 SharedPreferences 中没有这个 Key 值时返回的值 XXX 为 value 的数据类型 SharedPreferences shared = getSharedPreferences("data",MODE_PRIVATE); String name = shared.getString("name",""); int age = shared.getInt("age",0); boolean married = shared.getBoolean("married", false);
4. 从 SharedPreferences 中删除数据
需要或的 SharedPreferences.Editor 对象
1. editor.remove(arg0); arg0 : 要删除数据的 Key 值 删除一条数据 2. editor.clear(); 删除所有数据 3. 记得提修改 editor.apply; SharedPreferences shared = getSharedPreferences("data",MODE_PRIVATE); SharedPreferences.Editor editor = shared.edit();//获取编辑器 editor.remove("name");删除一条数据 editor.clear();删除所有数据 editor.apply();//提交修改