hibernate-validator 参数校验1
基础:
SpringBoot各种参数效验
进阶:
hibernate-validator 参数校验2(补充)
01 请求参数bean验证
接口上的Bean验证,需要在参数前加上@Valid
或Spring的 @Validated
注解,这两种注释都会导致应用标准Bean验证。
@PostMapping("/user")
public String add(@Validated @RequestBody UserAdminAddRequest userAdmin) {
userAdminService.save(userAdmin);
return "success";
}
@Data
@Accessors(chain = true)
public class UserAdminAddRequest {
@NotEmpty(message = "name 不能为空")
private String nickName;
@NotNull(message = "id 不能为空")
private Integer id;
@NotEmpty(message = "mobile 不能为空")
@Mobile // 自定义注解 校验手机号
private String mobile;
@NotNull(message = "status 不能为空")
@InEnum(value = UserStatusEnum.class, message = "用户状态枚举必须是 {value}", ignoreEmpty = true)
private Integer status;
}
以上对属性为对象的,对象里有参数校验的不生效,需要参考如下写法
02 校验嵌套属性(集合对象)的写法
参考资料地址1: VALIDATION-API包校验嵌套属性(集合对象)的写法
示例
@Data
public class VerifyDTO {
@NotNull(message = "id不能为空")
private Long id;
@NotEmpty(message = "数据不能为空")
private List<@Valid StudentDTO> list;
@NotNull
private @Valid TeacherDTO teacher;
}
@Data
public class StudentDTO {
@NotBlank(message = "姓名不能为空")
private String name;
@NotNull(message = "年龄不能为空")
private Integer age;
}
@Data
public class TeacherDTO {
@NotBlank(message = "教师姓名不能为空")
private String name;
}
需要给属性为对象的加入如图注解
测试
参数
{
"id": 1,
"list": [{
"age": null,
"name": "java"
}],
"teacher": {
"name": "tom"
}
}
controller方法
@PostMapping("/save")
public String save(@Validated @RequestBody VerifyDTO dto) {
System.out.println(dto);
return "success";
}
结果
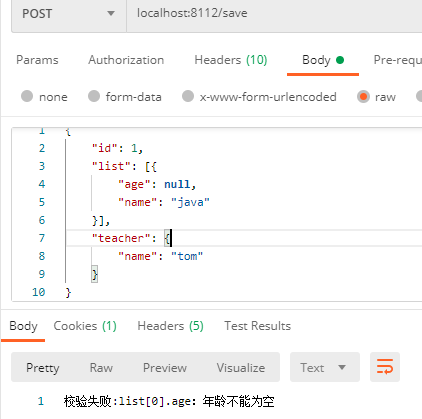
03 约束注解
空与非空检查
注解 | 支持Java类型 | 说明 |
---|---|---|
@Null | Object | 为null |
@NotNull | Object | 不为null |
@NotBlank | CharSequence | 不为null,且必须有一个非空格字符 |
@NotEmpty | CharSequence、Collection、Map、Array | 不为null,且不为空(length/size>0) |
Boolean值检查
注解 | 支持Java类型 | 说明 | 备注 |
---|---|---|---|
@AssertTrue | boolean、Boolean | 为true | 为null有效 |
@AssertFalse | boolean、Boolean | 为false | 为null有效 |
日期检查
注解 | 支持Java类型 | 说明 | 备注 |
---|---|---|---|
@Future | Date、Calendar、Instant、LocalDate、LocalDateTime、LocalTime、MonthDay、OffsetDateTime、OffsetTime、Year、YearMonth、ZonedDateTime、HijrahDate、JapaneseDate、MinguoDate、ThaiBuddhistDate | 验证日期为当前时间之后 | 为null有效 |
@FutureOrPresent | Date、Calendar、Instant、LocalDate、LocalDateTime、LocalTime、MonthDay、OffsetDateTime、OffsetTime、Year、YearMonth、ZonedDateTime、HijrahDate、JapaneseDate、MinguoDate、ThaiBuddhistDate | 验证日期为当前时间或之后 | 为null有效 |
@Past | Date、Calendar、Instant、LocalDate、LocalDateTime、LocalTime、MonthDay、OffsetDateTime、OffsetTime、Year、YearMonth、ZonedDateTime、HijrahDate、JapaneseDate、MinguoDate、ThaiBuddhistDate | 验证日期为当前时间之前 | 为null有效 |
@PastOrPresent | Date、Calendar、Instant、LocalDate、LocalDateTime、LocalTime、MonthDay、OffsetDateTime、OffsetTime、Year、YearMonth、ZonedDateTime、HijrahDate、JapaneseDate、MinguoDate、ThaiBuddhistDate | 验证日期为当前时间或之前 | 为null有效 |
数值检查
注解 | 支持Java类型 | 说明 | 备注 |
---|---|---|---|
@Max | BigDecimal、BigInteger,byte、short、int、long以及包装类 | 小于或等于 | 为null有效 |
@Min | BigDecimal、BigInteger,byte、short、int、long以及包装类 | 大于或等于 | 为null有效 |
@DecimalMax | BigDecimal、BigInteger、CharSequence,byte、short、int、long以及包装类 | 小于或等于 | 为null有效 |
@DecimalMin | BigDecimal、BigInteger、CharSequence,byte、short、int、long以及包装类 | 大于或等于 | 为null有效 |
@Negative | BigDecimal、BigInteger,byte、short、int、long、float、double以及包装类 | 负数 | 为null有效,0无效 |
@NegativeOrZero | BigDecimal、BigInteger,byte、short、int、long、float、double以及包装类 | 负数或零 | 为null有效 |
@Positive | BigDecimal、BigInteger,byte、short、int、long、float、double以及包装类 | 正数 | 为null有效,0无效 |
@PositiveOrZero | BigDecimal、BigInteger,byte、short、int、long、float、double以及包装类 | 正数或零 | 为null有效 |
@Digits(integer = 3, fraction = 2) | BigDecimal、BigInteger、CharSequence,byte、short、int、long以及包装类 | 整数位数和小数位数上限 | 为null有效 |
其他
注解 | 支持Java类型 | 说明 | 备注 |
---|---|---|---|
@Pattern | CharSequence | 匹配指定的正则表达式 | 为null有效 |
CharSequence | 邮箱地址 | 为null有效,默认正则 '.*' |
|
@Size | CharSequence、Collection、Map、Array | 大小范围(length/size>0) | 为null有效 |
hibernate-validator扩展约束(部分)
注解 | 支持Java类型 | 说明 |
---|---|---|
@Length | String | 字符串长度范围 |
@Range | 数值类型和String | 指定范围 |
@URL | URL地址验证 | |
@Mobile | String | 手机号格式校验 |