异常
Error和Exception
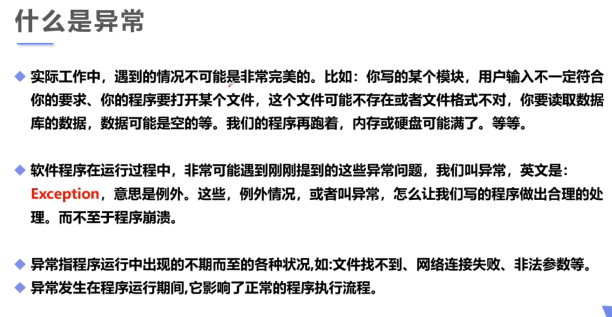
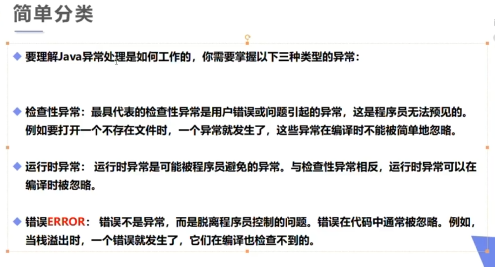
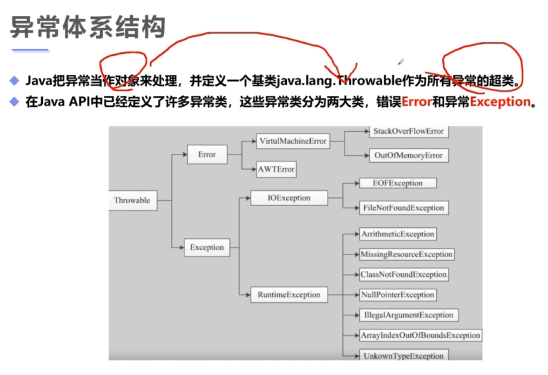
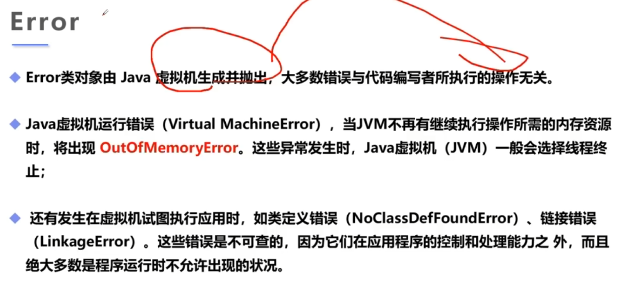
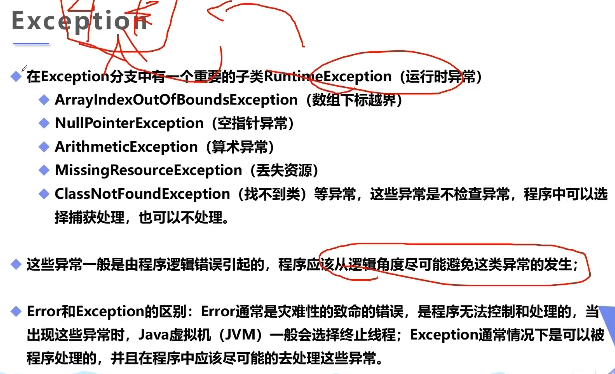
捕获和抛出异常
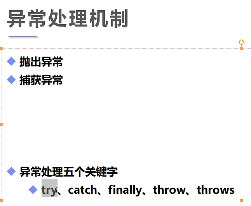
package exception;
public class Demo01 {
public static void main(String[] args) {
int a=1;
int b=0;
//Ctrl+Alt+t
try {//try监控区域
System.out.println(a/b);
}catch (ArithmeticException e){//捕获异常
System.out.println("除数不能为0");
}finally {//处理善后工作
System.out.println("finally");
}
//finally 可以不要finally ,eg:IO资源关闭
System.out.println("====================");
//多捕获要从小到大
try {//try监控区域
System.out.println(a/b);
}catch (Error e){//捕获异常
System.out.println("Error");
}catch (Exception e){
System.out.println("Exception");
}catch (Throwable e){
System.out.println("Throwable");
}
System.out.println("====================");
//多捕获要从小到大
try {//try监控区域
new Demo01().test(1,0);
} catch (ArithmeticException e){
e.printStackTrace();
}
System.out.println("====================");
}
//假设这个方法中处理不了这个异常,方法上抛出异常
public void test(int a,int b) throws ArithmeticException{
if(b==0){
throw new ArithmeticException();//主动抛出异常,一般在方法中使用
}
}
}
自定义异常及经验小结
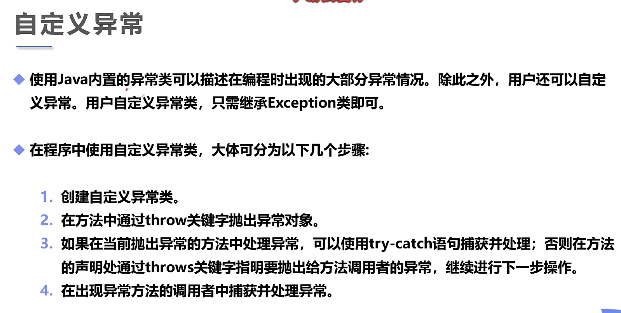
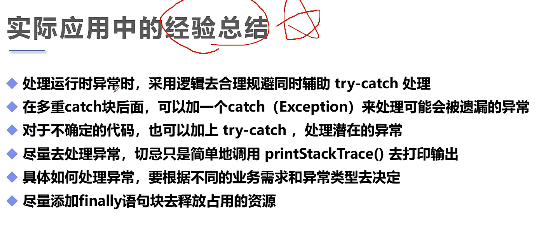
package exception;
public class MyException extends Exception{
//传递数字>10;
private int detail;
public MyException(int a){
this.detail = a;
}
//toString:异常的打印信息
@Override
public String toString() {
return "MyException{" +
"detail=" + detail +
'}';
}
}
package exception;
public class Test {
//可能存在异常的方法
static void test(int a) throws MyException {
System.out.println("传递的参数为"+a);
if(a>10){
throw new MyException(a);
}
System.out.println("OK");
}
public static void main(String[] args) {
try{
test(1);
}catch (MyException e){
System.out.println("MyException=>"+e);
}
try{
test(11);
}catch (MyException e){
System.out.println("MyException->"+e);
}
}
}