JUC并发编程
JUC笔记
1、什么是JUC
学习方法推荐 -->1.首先要学会使用 2.货比三家,寻找其他解决方案 3.分析源码(JDK开发手册)
源码+官方文档
JUC是 java util concurrent
面试高频问JUC~!
java.util 是Java的一个工具包~
业务:普通的线程代码 Thread
Runnable: 没有返回值、效率相比于Callable 相对较低!
2、线程和进程
进程:一个程序,QQ.EXE Music.EXE;数据+代码+pcb
一个进程可以包含多个线程,至少包含一个线程!
Java默认有几个线程?2个线程! main线程、GC线程
线程:开了一个进程Typora,写字,等待几分钟会进行自动保存(线程负责的)
对于Java而言:Thread、Runable、Callable进行开启线程的,我们之前。
提问?JAVA真的可以开启线程吗? 开不了的!
public synchronized void start() {
/**
* This method is not invoked for the main method thread or "system"
* group threads created/set up by the VM. Any new functionality added
* to this method in the future may have to also be added to the VM.
*
* A zero status value corresponds to state "NEW".
*/
if (threadStatus != 0)
throw new IllegalThreadStateException();
/* Notify the group that this thread is about to be started
* so that it can be added to the group's list of threads
* and the group's unstarted count can be decremented. */
group.add(this);
boolean started = false;
try {
start0();
started = true;
} finally {
try {
if (!started) {
group.threadStartFailed(this);
}
} catch (Throwable ignore) {
/* do nothing. If start0 threw a Throwable then
it will be passed up the call stack */
}
}
}
// 这是一个C++底层,Java是没有权限操作底层硬件的
private native void start0();
Java是没有权限去开启线程、操作硬件的,这是一个native的一个本地方法,它调用的底层的C++代码。
并发、并行
并发: 多线程操作同一个资源。
- CPU 只有一核,模拟出来多条线程,天下武功,唯快不破。那么我们就可以使用CPU快速交替,来模拟多线程。
并行: 多个人一起行走
- CPU多核,多个线程可以同时执行。 我们可以使用线程池!
public class Test1 {
public static void main(String[] args) {
//获取cpu的核数
System.out.println(Runtime.getRuntime().availableProcessors());
}
}
并发编程的本质:充分利用CPU的资源!
线程有几个状态?
线程的状态:6个状态-->
新生new、运行runnable、阻塞blocked、
等待waiting、超时等待timed-waiting、终止terminated;
public enum State {
/**
* Thread state for a thread which has not yet started.
*/
//新生
NEW,
/**
* Thread state for a runnable thread. A thread in the runnable
* state is executing in the Java virtual machine but it may
* be waiting for other resources from the operating system
* such as processor.
*/
//运行
RUNNABLE,
/**
* Thread state for a thread blocked waiting for a monitor lock.
* A thread in the blocked state is waiting for a monitor lock
* to enter a synchronized block/method or
* reenter a synchronized block/method after calling
* {@link Object#wait() Object.wait}.
*/
//阻塞
BLOCKED,
/**
* Thread state for a waiting thread.
* A thread is in the waiting state due to calling one of the
* following methods:
* <ul>
* <li>{@link Object#wait() Object.wait} with no timeout</li>
* <li>{@link #join() Thread.join} with no timeout</li>
* <li>{@link LockSupport#park() LockSupport.park}</li>
* </ul>
*
* <p>A thread in the waiting state is waiting for another thread to
* perform a particular action.
*
* For example, a thread that has called <tt>Object.wait()</tt>
* on an object is waiting for another thread to call
* <tt>Object.notify()</tt> or <tt>Object.notifyAll()</tt> on
* that object. A thread that has called <tt>Thread.join()</tt>
* is waiting for a specified thread to terminate.
*/
//等待
WAITING,
/**
* Thread state for a waiting thread with a specified waiting time.
* A thread is in the timed waiting state due to calling one of
* the following methods with a specified positive waiting time:
* <ul>
* <li>{@link #sleep Thread.sleep}</li>
* <li>{@link Object#wait(long) Object.wait} with timeout</li>
* <li>{@link #join(long) Thread.join} with timeout</li>
* <li>{@link LockSupport#parkNanos LockSupport.parkNanos}</li>
* <li>{@link LockSupport#parkUntil LockSupport.parkUntil}</li>
* </ul>
*/
//超时等待
TIMED_WAITING,
/**
* Thread state for a terminated thread.
* The thread has completed execution.
*/
//终止
TERMINATED;
}
wait/sleep的区别
1、来自不同的类
wait => Object
sleep => Thread
一般情况企业中使用休眠是:
TimeUnit.DAYS.sleep(1); // 休眠1天
TimeUnit.SECONDS.sleep(1); // 休眠1s
2、关于锁的释放
wait 会释放锁;
sleep睡觉了,不会释放锁;
3、使用的范围是不同的
wait 必须在同步代码块中;
sleep 可以在任何地方睡;
4、是否需要捕获异常
wait是不需要捕获异常;
sleep必须要捕获异常;
3、Lock锁(重点)
传统的Synchronized
/**
* 真正的多线程开发
* 线程就是一个单独的资源类,没有任何的附属操作!
*/
public class SaleTicketDemo01 {
public static void main(String[] args) {
// 多线程操作
// 并发:多线程操作同一个资源类,把资源类丢入线程
Ticket ticket = new Ticket();
// @FunctionalInterface 函数式接口 jdk1.8之后 lambda表达式
new Thread(()->{
for(int i=0;i<40;i++){
ticket.sale();
}
},"A").start();
new Thread(()->{
for(int i=0;i<40;i++){
ticket.sale();
}
},"B").start();
new Thread(()->{
for(int i=0;i<40;i++){
ticket.sale();
}
},"C").start();
}
}
// 资源类
// 属性+方法
// oop
class Ticket{
private int number=50;
// 卖票的方式
// synchronized 本质:队列,锁
public synchronized void sale(){
if(number>0){
System.out.println(Thread.currentThread().getName()+" 卖出了第"+number+" 张票,剩余:"+number+" 张票");
number--;
}
}
}
Lock接口
公平锁: 十分公平,必须先来后到~;
非公平锁: 十分不公平,可以插队;(默认为非公平锁)
public class SaleTicketDemo02 {
public static void main(String[] args) {
// 多线程操作
// 并发:多线程操作同一个资源类,把资源类丢入线程
Ticket2 ticket = new Ticket2();
new Thread(()->{for(int i=0;i<40;i++) ticket.sale(); },"A").start();
new Thread(()->{for(int i=0;i<40;i++) ticket.sale(); },"B").start();
new Thread(()->{for(int i=0;i<40;i++) ticket.sale(); },"C").start();
}
}
// lock三部曲
// 1、Lock lock=new ReentrantLock();
// 2、lock.lock() 加锁
// 3、finally=> 解锁:lock.unlock();
class Ticket2{
private int number=50;
Lock lock=new ReentrantLock();
// 卖票的方式
// 使用Lock 锁
public void sale(){
//加锁
lock.lock();
try {
//业务代码
if(number>=0){
System.out.println(Thread.currentThread().getName()+" 卖出了第"+number+" 张票,剩余:"+number+" 张票");
number--;
}
}catch (Exception e) {
e.printStackTrace();
}
finally {
// 解锁
lock.unlock();
}
}
}
Synchronized 和 Lock区别
-
1、Synchronized 内置的Java关键字,Lock是一个Java类
-
2、Synchronized 无法判断获取锁的状态,Lock可以判断是否获取到锁
-
3、Synchronized 会自动释放锁,lock必须要手动加锁和手动释放锁!可能会遇到死锁
-
4、Synchronized 线程1(获得锁->阻塞)、线程2(等待);
lock就不一定会一直等待下去,lock会有一个trylock去尝试获取锁,不会造成长久的等待。
-
5、Synchronized 是可重入锁,不可以中断的,非公平的;Lock,可重入的,可以判断锁,可以自己设置公平锁和非公平锁;
-
6、Synchronized 适合锁少量的代码同步问题,Lock适合锁大量的同步代码;
锁到底是什么? 如何判断锁的是谁?
4、生产者和消费者问题!
面试常问重点:单例模式、排序算法、生产者和消费者、死锁
Synchronized wait notify可以实现,该方法是传统版本;
我们这次使用lock版本
Synchronized版本
线程之间的通信问题:生产者和消费者问题!!!
线程的交替执行 A B 操作同一个变量
num = 0 A:num+1 B:num-1
A与B之间的交互口诀:判断等待、业务、通知
public class A {
public static void main(String[] args) {
Data data = new Data();
new Thread(()->{for(int i=0;i<10;i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
},"A").start();
new Thread(()->{for(int i=0;i<10;i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}},"B").start();
}
}
//单独的资源类,线程的耦合度降低
class Data{
// 数字 资源类
private int number = 0;
// +1
public synchronized void increment() throws InterruptedException {
if(number!=0){
// 等待操作
this.wait();
}
number++;
System.out.println(Thread.currentThread().getName()+"=>"+number);
// 通知其他线程 我+1完毕了
this.notifyAll();
}
// -1
public synchronized void decrement() throws InterruptedException {
if(number==0){
// 等待操作
this.wait();
}
number--;
System.out.println(Thread.currentThread().getName()+"=>"+number);
// 通知其他线程 我-1完毕了
this.notifyAll();
}
}
问题存在,A线程B线程,现在如果我有四个线程A B C D!
解决方案: if 改为while即可,防止虚假唤醒
这样就不存在问题了:
JUC版本的生产者和消费者问题
await、signal 替换 wait、notify
通过Lock找到Condition
public class B {
public static void main(String[] args) {
Data2 data = new Data2();
new Thread(()->{for(int i=0;i<10;i++) {
data.increment();
}
},"A").start();
new Thread(()->{for(int i=0;i<10;i++) {
data.decrement();
}},"B").start();
new Thread(()->{for(int i=0;i<10;i++) {
data.increment();
}
},"C").start();
new Thread(()->{for(int i=0;i<10;i++) {
data.decrement();
}
},"D").start();
}
}
class Data2{
//数字 资源类
private int number = 0;
//lock锁
Lock lock = new ReentrantLock();
Condition condition = lock.newCondition();
//+1
public void increment() {
lock.lock();
try{
//业务
while (number!=0){
//等待操作
condition.await();
}
number++;
System.out.println(Thread.currentThread().getName()+"=>"+number);
//通知其他线程 我+1完毕了
condition.signalAll();
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
//-1
public void decrement() {
lock.lock();
try{
//业务
while (number==0){
//等待操作
condition.await();
}
number--;
System.out.println(Thread.currentThread().getName()+"=>"+number);
//通知其他线程 我+1完毕了
condition.signalAll();
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
Condition的优势:精准的通知和唤醒的线程!
如果我们要指定通知的下一个进行顺序怎么办呢? 我们可以使用Condition来指定通知进程~
/**
* A 执行完 调用B
* B 执行完 调用C
* C 执行完 调用A
*/
public class C {
public static void main(String[] args) {
Data3 data3 = new Data3();
new Thread(()->{
for(int i=0;i<10;i++){
data3.printA();
}
},"A").start();
new Thread(()->{
for(int i=0;i<10;i++){
data3.printB();
}
},"B").start();
new Thread(()->{
for(int i=0;i<10;i++){
data3.printC();
}
},"C").start();
}
}
class Data3{
//资源类
private Lock lock=new ReentrantLock();
private Condition condition1 = lock.newCondition();
private Condition condition2 = lock.newCondition();
private Condition condition3 = lock.newCondition();
private int number = 1; //1A 2B 3C
public void printA(){
lock.lock();
try {
//业务 判断 -> 执行 -> 通知
while(number!=1){
//等待
condition1.await();
}
//操作
System.out.println(Thread.currentThread().getName()+",AAAAA");
//唤醒指定的线程
number=2;
condition2.signal(); // 唤醒2
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
public void printB(){
lock.lock();
try {
//业务 判断 -> 执行 -> 通知
while (number!=2){
condition2.await();
}
System.out.println(Thread.currentThread().getName()+",BBBBB");
//唤醒3
number=3;
condition3.signal();
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
public void printC(){
lock.lock();
try {
//业务 判断 -> 执行 -> 通知
while(number!=3){
condition3.await();
}
System.out.println(Thread.currentThread().getName()+",CCCCC");
//唤醒1
number=1;
condition1.signal();
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
5、8锁现象
如何判断锁的是谁!锁到底锁的是谁?
锁会锁住:对象、Class
深刻理解我们的锁
- 问题1:
结果是:先发短信,如何再打电话!
为什么? 如果你认为是顺序在前? 这个答案是错误的!
- 问题2:
我们再来看:我们让发短信 延迟4s
现在结果是什么呢?
结果:还是先发短信,然后再打电话!
why?
原因:并不是顺序执行!是因为synchronized 锁的对象是方法的调用!对于两个方法用的是同一个锁,谁先拿到谁先执行!另外一个则等待!
- 问题3:
如果我们添加一个普通方法,那么先执行哪一个呢?
答案是:先执行hello,然后再执行发短信!原因是hello是一个普通方法,不受synchronized锁的影响
- 问题4:
如果我们使用的是两个对象,一个调用发短信,一个调用打电话,那么整个顺序是怎么样的呢?
答案是:先打电话,后发短信。原因:在发短信方法中延迟了4s,又因为synchronized锁的是对象,但是我们这使用的是两个对象,所以每个对象都有一把锁,所以不会造成锁的等待。正常执行
- 问题5,6:
如果我们把synchronized的方法加上static变成静态方法!那么顺序又是怎么样的呢?
(1)我们先来使用一个对象调用两个方法!
答案是:先发短信,后打电话
(2)如果我们使用两个对象调用两个方法!
答案是:还是先发短信,后打电话
原因是什么呢? 为什么加了static就始终前面一个对象先执行呢!为什么后面会等待呢?
原因是:对于static静态方法来说,对于整个类Class来说只有一份,对于不同的对象使用的是同一份方法,相当于这个方法是属于这个类的,如果静态static方法使用synchronized锁定,那么这个synchronized锁会锁住整个对象!不管多少个对象,对于静态的锁都只有一把锁,谁先拿到这个锁就先执行,其他的进程都需要等待!
- 问题7:
如果我们使用一个静态同步方法、一个同步方法、一个对象调用顺序是什么?
明显答案是:先打电话,后发短信了。
因为一个锁的是Class类模板,一个锁的是对象调用者。后面那个打电话不需要等待发短信,直接运行就可以了。
- 问题8:
如果我们使用一个静态同步方法、一个同步方法、两个对象调用顺序是什么呢?
当然答案是:先打电话、后发短信!
因为两个对象,一样的原因:两把锁锁的不是同一个东西,所以后面的第二个对象不需要等待第一个对象的执行。
小结
new 出来的 this 是具体的一个对象
static Class 是唯一的一个模板
6、集合类不安全
List不安全
并发下 ArrayList不安全的
java.uil.ConcurrentModificationException 并发修改异常
// java.util.ConcurrentModificationException 并发修改异常
List<String> list = new ArrayList<>();
//并发下Array List不安全,synchranized
//解决方案
//1.List<String> list = new Vector<>();
//2.List<String> list = Collections.synchronizedList(new ArrayList<>());
//3.List<String> list = new CopyOnWriteArrayList<>();
for (int i = 1; i <= 1000; i++) {s
new Thread(()->{
list.add(UUID.randomUUID().toString().substring(0,5));
System.out.println(list);
},String.valueOf(i)).start();
}
解决方案
- 将ArrayList换成Vector
- 使用Collections.synchronizedList(new ArrayList<>());
- 使用JUC中的包:List arrayList = new CopyOnWriteArrayList<>();
- CopyOnWrite 写入时复制 COW 计算机程序设计领域的一种优化策略;
- 多线程调用的时候,list,读取的时候,固定的,写入(覆盖)
- 在写入的时候避免覆盖,造成数据问题。
- 读写分离 CopyOnWriteArrayList比vector好在不使用同步锁
Set不安全
// Set<Object> set =new HashSet<>();
// Set<Object> set = Collections.synchronizedSet(new HashSet<>());
Set<Object> set = new CopyOnWriteArraySet<>();
for (int i = 1; i <= 1000; i++) {
new Thread(()->{
set.add(UUID.randomUUID().toString().substring(0,5));
System.out.println(set);
},String.valueOf(i)).start();
}
}
HashSet底层
public HashSet() {
map = new HashMap<>();
}
//add 本质其实就是一个map的key,map的key是无法重复的,所以使用的就是map存储
//hashSet就是使用了hashmap key不能重复的原理
public boolean add(E e) {
return map.put(e, PRESENT)==null;
}
private static final Object PRESENT = new Object(): //这是不变的值
Map不安全
//一多并发 ConcurrentModificationException 并发修改异常
// Map<String, String> map = new HashMap<String, String>();
//工作中不用HashMap
//默认等价于什么? new HashMap<>(16,0.75);
// 1.Map<String, String> map = Collections.synchronizedMap(new HashMap<>());
//并发Map 研究其原理
Map<String, String> map = new ConcurrentHashMap<>();
for (int i = 0; i < 100; i++) {
new Thread(()->{
map.put(Thread.currentThread().getName(),
UUID.randomUUID().toString().substring(0,5));
System.out.println(map);
},String.valueOf(i)).start();
}
7、Callable接口
- 可有返回值
- 可抛出异常
- 方法不同,run() / call()
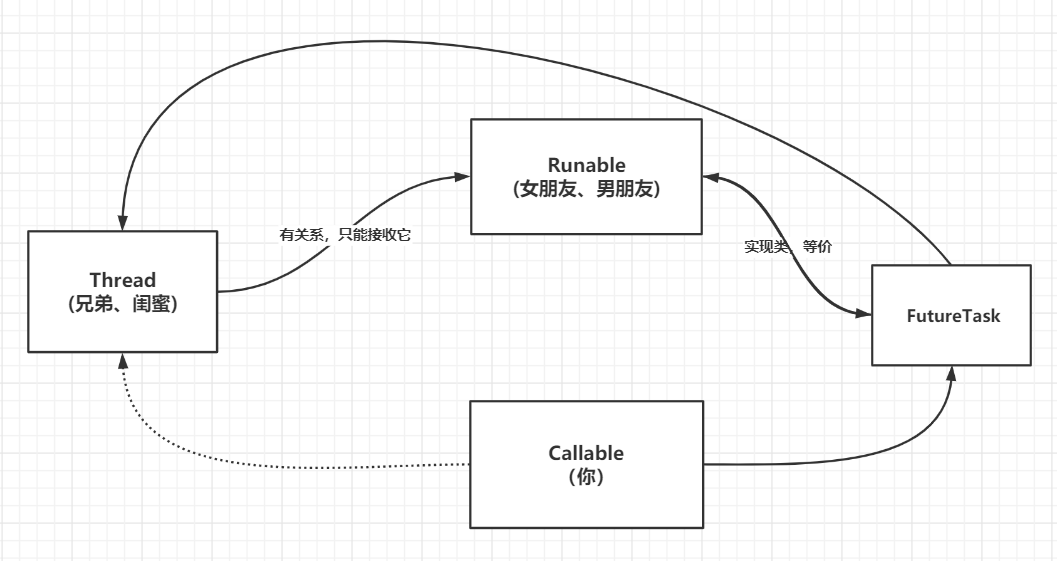
代码测试
public class CallableTest {
public static void main(String[] args) throws ExecutionException, InterruptedException {
// new Thread(new Runnable()).start();
// new Thread(new FutureTask<V>()).start();
// new Thread(new FutureTask<V>(new Callable())).start();
new Thread().start(); // 怎么启动Callable
MyThread thread = new MyThread();
FutureTask futureTask = new FutureTask(thread);
new Thread(futureTask, "A").start();
new Thread(futureTask, "B").start(); // 结果会被缓存 结果可能需要等待 会阻塞!
Integer o = (Integer)futureTask.get(); // 获取Callable的返回结果
System.out.println(o);
}
}
class MyThread implements Callable<Integer> {
@Override
public Integer call() {
System.out.println("call()");
return 1024;
}
}
8、常用辅助类(掌握)
8.1、CountDownLatch(倒数计时) 减法计数器
// 减法计数器
public class CountDownLatchDemo {
public static void main(String[] args) throws InterruptedException {
// 总数是6,有必须要执行的任务时再使用
CountDownLatch countDownLatch = new CountDownLatch(6);
for (int i = 1; i <= 6; i++) {
new Thread(() -> {
System.out.println(Thread.currentThread().getName() + "Go Out!");
countDownLatch.countDown(); // 数量-1
}, String.valueOf(i)).start();
}
countDownLatch.await(); // 等待计数器归零 在向下执行
countDownLatch.countDown(); // -1
System.out.println("close Door");
}
}
原理:
countDownLatch.countDown()
:数量-1
countDownLatch.await()
:等待计数器归零 在向下执行
await等待计数器为0,就唤醒,再继续向下运行
每次有线程调用countDown()数量-1,假设计数器变为0,countDownLatch.await()会被唤醒,继续执行
8.2、CyclicBarrier(循环壁垒) 加法计数器
// 加法计数器
public class CyclicBarrierDemo {
public static void main(String[] args) {
/**
* 集齐七个龙珠召唤神龙
*/
// 召唤龙珠的线程
CyclicBarrier cyclicBarrier = new CyclicBarrier(7, ()->{
System.out.println("召唤神龙成功!");
});
for (int i = 1; i <= 7; i++) {
final int temp = i;
// lambda能操作i吗? 不行!
new Thread(()->{
System.out.println(Thread.currentThread().getName() + "收集了" + temp + "个龙珠");
try {
cyclicBarrier.await(); // 等待,通过await()来计数 直到召唤后才执行后面的 与 CountDownLatch 有区别
} catch (InterruptedException e) {
e.printStackTrace();
} catch (BrokenBarrierException e) {
e.printStackTrace();
}
}).start();
}
}
}
8.3、Semaphore()
public class SemaphoreDemo {
public static void main(String[] args) {
// 线程数量:停车位 限流!
Semaphore semaphore = new Semaphore(3);
for (int i = 1; i <= 6; i++) {
new Thread(()->{
// acquire() 得到
try {
semaphore.acquire();
System.out.println(Thread.currentThread().getName() + "抢到车位");
TimeUnit.SECONDS.sleep(2);
System.out.println(Thread.currentThread().getName() + "离开车位");
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
semaphore.release();// release() 释放
}
}, String.valueOf(i)).start();
}
}
}
原理:
semaphore.acquire()
:获得,如果已经满了,等待,等待被释放为止!
semaphore.release()
:释放,会将当前的信号量释放+1,然后唤醒等待的线程
作用:多个共享资源互斥的使用!并发限流,控制最大的线程数,保证服务器的安全和高可用!
9、ReadWriteLock(读写锁)
/**
* 独占锁(写锁):一次只能被一个线程占有
* 共享锁(读锁):多个线程可以同时占有
* ReadWriteLock
* 读-读:可以共存
* 读-写:不能共存
* 写-写:不能共存
*/
public class ReadWriteLockDemo {
public static void main(String[] args) {
myCacheLock myCache = new myCacheLock();
// 寫入
for (int i = 1; i <= 5; i++) {
final int temp = i;
new Thread(()->{
myCache.put(temp+"", temp+"");
}, String.valueOf(i)).start();
}
// 讀取
for (int i = 1; i <= 5; i++) {
final int temp = i;
new Thread(()->{
myCache.get(temp+"");
}, String.valueOf(i)).start();
}
}
}
/**
* 自定义缓存
*/
// 加锁的
class myCacheLock {
private volatile Map<String, Object> map = new HashMap<>();
// 读写锁 更加细粒度的控制
private ReadWriteLock readWriteLock = new ReentrantReadWriteLock();
// 存, 写入的时候 只希望一个线程去写
public void put(String key, Object value) {
readWriteLock.writeLock().lock();
try {
System.out.println(Thread.currentThread().getName() + "写入" + key);
map.put(key, value);
System.out.println(Thread.currentThread().getName() + "写入完毕");
} catch (Exception e) {
e.printStackTrace();
} finally {
readWriteLock.writeLock().unlock();
}
}
// 取, 读 所有人都可以读!
public void get(String key) {
readWriteLock.readLock().lock();
try {
System.out.println(Thread.currentThread().getName() + "读取" + key);
Object o = map.get(key);
System.out.println(Thread.currentThread().getName() + "读取ok" + key);
} catch (Exception e) {
e.printStackTrace();
} finally {
readWriteLock.readLock().unlock();
}
}
}
10、阻塞队列
不得不阻塞:
阻塞队列(BlockingQueue)
使用阻塞队列的情况:多线程并发处理、线程池!
使用队列:
添加、移除
四组API
方式 | 抛出异常 | 不会抛出异常,有返回值 | 阻塞 等待 | 超时 等待 |
---|---|---|---|---|
添加 | add() | offer() | put() | offer(timenum,timeUnit) |
移除 | remove() | poll() | take() | poll(timenum,timeUnit) |
得到队首元素 | element() | peek() | - | - |
同步队列(SynchronousQueue)
没有容量,进一个必须要出一个,否者不能再次进入
put()、take()
/**
* 同步队列
* SynchronousQueue 不能连续存储
* put了一个值,必须先take出来,否则不能再次put进去值
*/
public class SynchronousQueueDemo {
public static void main(String[] args) {
// 同步队列
BlockingQueue<String> blockingQueue = new SynchronousQueue<>();
//研究一下 如果判断这是一个同步队列
//使用两个进程
// 一个进程 放进去
// 一个进程 拿出来
new Thread(()->{
try {
blockingQueue.put("1");
System.out.println(Thread.currentThread().getName() + " put 1");
blockingQueue.put("2");
System.out.println(Thread.currentThread().getName() + " put 2");
blockingQueue.put("3");
System.out.println(Thread.currentThread().getName() + " put 3");
} catch (InterruptedException e) {
e.printStackTrace();
}
}, "T1").start();
new Thread(()->{
try {
TimeUnit.SECONDS.sleep(3);
System.out.println(Thread.currentThread().getName() + " => " + blockingQueue.take());
TimeUnit.SECONDS.sleep(3);
System.out.println(Thread.currentThread().getName() + " => " + blockingQueue.take());
TimeUnit.SECONDS.sleep(3);
System.out.println(Thread.currentThread().getName() + " => " + blockingQueue.take());
} catch (InterruptedException e) {
e.printStackTrace();
}
}, "T2").start();
}
}
11、线程池(重点)
线程池:三大方法、7大参数、4种拒绝策略
池化技术
程序的运行,本质:占用系统的资源!我们需要去优化资源的使用 ===> 池化技术
线程池、JDBC的连接池、内存池、对象池 等等。。。。
资源的创建、销毁十分消耗资源
池化技术:事先准备好一些资源,如果有人要用,就来我这里拿,用完之后还给我,以此来提高效率。
线程池的好处:
1、降低资源的消耗;
2、提高响应的速度;
3、方便管理;
线程复用、可以控制最大并发数、管理线程
线程池:三大方法
- ExecutorService threadPool = Executors.newSingleThreadExecutor(); // 创建单个线程,永远只存在一个线程
- ExecutorService threadPool2 = Executors.newFixedThreadPool(5); // 创建一个固定的线程池的大小,此为5个线程
- ExecutorService threadPool3 = Executors.newCachedThreadPool(); // 可伸缩的,根据题意生成线程,遇强则强,遇弱则弱
// Executors 工具类,3大方法
public class ThreadPool {
public static void main(String[] args) {
ExecutorService threadPool = Executors.newSingleThreadExecutor(); // 单个线程
// ExecutorService threadPool = Executors.newFixedThreadPool(5); // 创建一个固定的线程池的大小
// ExecutorService threadPool = Executors.newCachedThreadPool(); // 可伸缩的
try {
for (int i = 0; i < 100; i++) {
// 使用线程池后,使用线程池创建线程
threadPool.execute(()->{
System.out.println(Thread.currentThread().getName() + " ok");
});
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 线程池用完,程序结束,关闭线程池
threadPool.shutdown();
}
}
}
七大参数
源码剖析:
//三大方法的源码剖析
public static ExecutorService newSingleThreadExecutor() {
return new FinalizableDelegatedExecutorService
(new ThreadPoolExecutor(1, 1,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>()));
}
public static ExecutorService newFixedThreadPool(int nThreads) {
return new ThreadPoolExecutor(nThreads, nThreads,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
}
public static ExecutorService newCachedThreadPool() {
return new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
new SynchronousQueue<Runnable>());
}
// 本质:三大方法都是开启 ThreadPoolExecutor 即:
//本质时开启ThreadPoolExecutor方法,它的七大参数分析
public ThreadPoolExecutor(int corePoolSize, //核心线程池大小
int maximumPoolSize, //最大的线程池大小
long keepAliveTime, //超时了没有人调用就会释放
TimeUnit unit, //超时单位
BlockingQueue<Runnable> workQueue, //阻塞队列
ThreadFactory threadFactory, //线程工厂 创建线程的 一般不用动
RejectedExecutionHandler handler //拒绝策略
) {
if (corePoolSize < 0 ||
maximumPoolSize <= 0 ||
maximumPoolSize < corePoolSize ||
keepAliveTime < 0)
throw new IllegalArgumentException();
if (workQueue == null || threadFactory == null || handler == null)
throw new NullPointerException();
this.corePoolSize = corePoolSize;
this.maximumPoolSize = maximumPoolSize;
this.workQueue = workQueue;
this.keepAliveTime = unit.toNanos(keepAliveTime);
this.threadFactory = threadFactory;
this.handler = handler;
}
阿里巴巴的Java操作手册中明确说明:对于Integer.MAX_VALUE初始值较大,所以一般情况我们要使用底层的ThreadPoolExecutor来创建线程池。
实际业务图
手动创建线程池
/**
* 四大拒绝策略
* new ThreadPoolExecutor.AbortPolicy() // 该拒绝策略为:超出最大承载,就会抛出异常
* new ThreadPoolExecutor.CallerRunsPolicy() // 该拒绝策略为:哪来的去哪里 main线程进行处理
* new ThreadPoolExecutor.DiscardPolicy() // 该拒绝策略为:队列满了,丢掉异常,不会抛出异常,丢掉任务,不理睬
* new ThreadPoolExecutor.DiscardOldestPolicy() // 该拒绝策略为:队列满了,尝试去和最早的进程竞争,不会抛出异常
*/
public class ThreadPoolDemo {
public static void main(String[] args) {
// 自定义线程池 一般情况下,工作中只用 ThreadPoolExecutor 安全!
/*最大线程如何获取? (调优)
1、CPU密集型 电脑的核数是几核就选择几;选择maximunPoolSize的大小
2、IO密集型 程序中有15个大型任务,io十分占用资源;I/O密集型就是判断我们程序中十分耗I/O的线程数量,大约是最大I/O数的一倍到两倍之间。
*/
// 获取CPU核数
System.out.println(Runtime.getRuntime().availableProcessors()); // 4
ThreadPoolExecutor threadPool = new ThreadPoolExecutor(
2,
Runtime.getRuntime().availableProcessors(),
3,
TimeUnit.SECONDS,
new LinkedBlockingDeque<>(3),
Executors.defaultThreadFactory(),
new ThreadPoolExecutor.AbortPolicy()
);
try {
// 最大承载量: Max + Deque = 5 + 3 超过则报 RejectedExecutionException
for (int i = 1; i <= 9; i++) {
threadPool.execute(()->{
System.out.println(Thread.currentThread().getName() + " ok");
});
}
} catch (Exception e) {
e.printStackTrace();
} finally {
threadPool.shutdown();
}
}
}
12、四大函数式接口(掌握)
掌握:Lambda表达式、链式编程、函数式接口、Stream流式计算
函数式接口:只有一个方法的接口
超级多FunctionInterface,特别多的函数式接口
简化编程模型,在新版本的框架底层大量应用!!
eg:foreach(消费者类的函数式接口)
Consumer,Function,Predicate,Supplier 四大原生态函数接口
@FunctionalInterface
public interface Runnable {
public abstract void run();
}
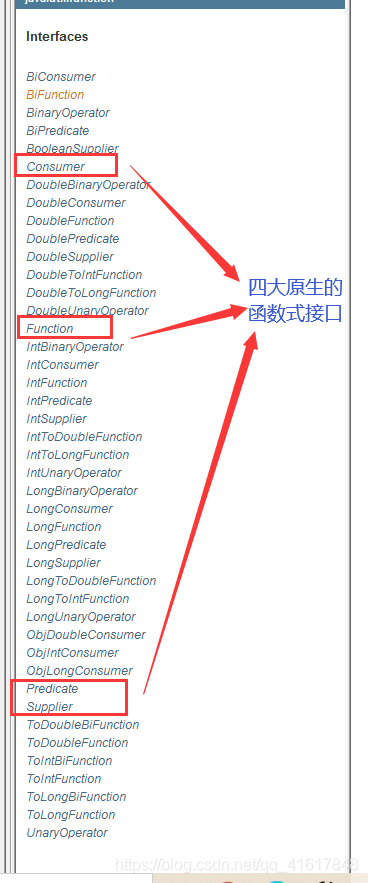
函数型接口可以使用lambda表达式
代码测试:
1.Function 函数型接口
T
- 函数输入的类型
R
- 函数结果的类型
/**
* Function 函数型接口
*有一个输入参数,一个输出参数
*只要是函数式接口,就可以使用lambda表达式简化
*/
public class FunctionDemo {
public static void main(String[] args) {
/* Function<String, String> funInterface = new Function<>() {
@Override
public String apply(String s) {
return s;
}
};*/
// Lambda 表达式简化
Function<String, String> funInterface = (str)->{return str;};
System.out.println(funInterface.apply("Hello"));
}
}
2.Predicate 断定型接口
/**
* Predicate 断定型接口
* 只有一个参数,为Boolean类型
*/
public class PredicateDemo {
public static void main(String[] args) {
//判断字符串是否为空
/* Predicate<String> predicateInterface = new Predicate<>() {
@Override
public boolean test(String s) {
return s.isEmpty();
}
};*/
//lambda简化
Predicate<String> predicateInterface = (str)->{return str.isEmpty();};
System.out.println(predicateInterface.test("hello"));
System.out.println(predicateInterface.test(""));
}
}
3.Consumer 消费型接口
/**
* Consumer 消费型接口 没有返回值!只有输入!
*/
public class ConsumerDemo {
public static void main(String[] args) {
Consumer<String> consumerInterface = (str)->{System.out.println(str);};
consumerInterface.accept("Hello!");
}
}
4.Supplier 供给型接口
/**
* Supplier 供给型接口 只返回,不输入
*/
public class SupplierDemo {
public static void main(String[] args) {
Supplier<String> supplierInterface = ()->{return "1024";};
System.out.println(supplierInterface.get());
}
}
JDK8新特性,新生代程序员必备,基本知识也不能落下(泛型、枚举、反射和注解)
13、Stream流式计算
定义:
存储+计算!
存储:集合、MySQL
计算:流式计算~ 链式编程
计算都应该交给流来操作
/**
* 题目要求:一分钟内完成此题,只能用一行代码实现!
* 现在有5个用户!筛选:
* 1、ID必须是偶数
* 2、年龄必须大于23岁
* 3、用户名转为大写字母
* 4、用户名字母倒着排序
* 5、只输出一个用户!
*/
public class StreamDemo {
public static void main(String[] args) {
User a = new User(1, "a", 21);
User b = new User(2, "b", 22);
User c = new User(3, "c", 23);
User d = new User(4, "d", 24);
User e = new User(5, "e", 25);
User f = new User(6, "f", 26);
// 集合管存储 List集合
List<User> list = Arrays.asList(a, b, c, d, e, f);
// 计算交给流
// 链式编程
list.stream()
.filter(u -> {
return u.getId() % 2 == 0;
})
.filter(u -> {
return u.getAge() > 23;
})
.map(u -> {
return u.getName().toUpperCase();
})
.sorted((u1, u2) -> {
return u2.compareTo(u1);
})
.limit(1)
.forEach(System.out::println);
}
}
14、ForkJoin
定义
ForkJoin 在JDK1.7,并行执行任务!提高效率~。在大数据量速率会更快!
大数据中:MapReduce 核心思想->把大任务拆分为小任务!
ForkJoin 特点: 工作窃取!
实现原理是:双端队列!从上面和下面都可以去拿到任务进行执行!
如何使用 ForkJoin
-
1、通过ForkJoinPool来执行
-
2、计算任务 execute(ForkJoinTask<?> task)
- 3、计算类要去继承ForkJoinTask
ForkJoin的计算类!
/**
* 计算求和的任务
*/
public class ForkJoinDemo extends RecursiveTask<Long> {
private long star;
private long end;
//临界值
private long temp = 1000000L;
public ForkJoinDemo(long star, long end) {
this.star = star;
this.end = end;
}
/**
* 计算方法
*
* @return Long
*/
@Override
protected Long compute() {
if ((end - star) < temp) {
Long sum = 0L;
for (Long i = star; i < end; i++) {
sum += i;
}
// System.out.println(sum);
return sum;
} else {
//使用forkJoin 分而治之 计算
//计算平均值
long middle = (star + end) / 2;
ForkJoinDemo forkJoinDemoTask1 = new ForkJoinDemo(star, middle);
forkJoinDemoTask1.fork(); //拆分任务,把线程任务压入线程队列
ForkJoinDemo forkJoinDemoTask2 = new ForkJoinDemo(middle, end);
forkJoinDemoTask2.fork(); //拆分任务,把线程任务压入线程队列
long taskSum = forkJoinDemoTask1.join() + forkJoinDemoTask2.join();
return taskSum;
}
}
}
测试:
public class Test {
public static void main(String[] args) throws ExecutionException, InterruptedException {
// test1(); // 24549
// test2(); // 15065
test3(); // 490
}
// 普通
public static void test1() {
long start = System.currentTimeMillis();
Long sum = 0L;
for (Long i = 1L; i <= 10_0000_0000; i++) {
sum += i;
}
long end = System.currentTimeMillis();
System.out.println("Sum = " + sum + ",时间 = " + (end - start));
}
// 使用ForkJoin
public static void test2() throws ExecutionException, InterruptedException {
long start = System.currentTimeMillis();
ForkJoinPool forkJoinPool = new ForkJoinPool();
ForkJoinTask<Long> task = new ForkJoinDemo(0L, 10_0000_0000);
ForkJoinTask<Long> submit = forkJoinPool.submit(task);
Long sum = submit.get();
long end = System.currentTimeMillis();
System.out.println("Sum = " + sum + ",时间 = " + (end - start));
}
// Stream 并行流
public static void test3() {
long start = System.currentTimeMillis();
// range() : () rangeClosed() : (]
long sum = LongStream.rangeClosed(0L, 10_0000_0000).parallel().reduce(0, Long::sum);
long end = System.currentTimeMillis();
System.out.println("Sum = " + sum + ",时间 = " + (end - start));
}
}
15、异步回调
Future 设计的初衷:对将来的某个事件结果进行建模!
其实就是前端 --> 发送ajax异步请求给后端
但是我们平时都使用CompletableFuture
异步调用:CompletableFuture
1.异步执行 2.成功回调 3.失败回调
public static void main(String[] args) throws ExecutionException, InterruptedException {
// 没有返回值的 runAsync 异步回调
CompletableFuture<Void> completableFuture = CompletableFuture.runAsync(() -> {
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "runAsync => Void");
});
System.out.println("22222");
completableFuture.get(); // 阻塞获取执行结果
System.out.println("=============================");
// 有返回值的 runAsync 异步回调 供给性参数
// ajax
CompletableFuture<Integer> completableFuture = CompletableFuture.supplyAsync(() -> {
System.out.println(Thread.currentThread().getName() + "supplyAsync => Integer");
int i = 10 / 0;
return 1024;
});
System.out.println(completableFuture.whenComplete((t, u) -> {
System.out.println("T => " + t); // 正常的返回结果
System.out.println("U => " + u); // 错误信息 java.lang.ArithmeticException: / by zero
}).exceptionally((e) -> {
System.out.println(e.getMessage());
return 233; // 可以获取到错误的返回结果
}).get());
}
}
16 、JMM
请你谈谈你对Volatile 的理解
Volatile 是 Java 虚拟机提供 轻量级的同步机制
1、保证可见性
2、不保证原子性
3、禁止指令重排
什么是JMM?
JMM:JAVA内存模型,不存在的东西,是一个概念,也是一个约定!
关于JMM的一些同步的约定:
1、线程解锁前,必须把共享变量立刻刷回主存;
2、线程加锁前,必须读取主存中的最新值到工作内存中;
3、加锁和解锁是同一把锁;
线程中分为 工作内存、主内存
8种操作:
- Read(读取):作用于主内存变量,它把一个变量的值从主内存传输到线程的工作内存中,以便随后的load动作使用;
- load(载入):作用于工作内存的变量,它把read操作从主存中变量放入工作内存中;
- Use(使用):作用于工作内存中的变量,它把工作内存中的变量传输给执行引擎,每当虚拟机遇到一个需要使用到变量的值,就会使用到这个指令;
- assign(赋值):作用于工作内存中的变量,它把一个从执行引擎中接受到的值放入工作内存的变量副本中;
- store(存储):作用于主内存中的变量,它把一个从工作内存中一个变量的值传送到主内存中,以便后续的write使用;
- write(写入):作用于主内存中的变量,它把store操作从工作内存中得到的变量的值放入主内存的变量中;
- lock(锁定):作用于主内存的变量,把一个变量标识为线程独占状态;
- unlock(解锁):作用于主内存的变量,它把一个处于锁定状态的变量释放出来,释放后的变量才可以被其他线程锁定;
JMM对这8种操作给了相应的规定:
-
不允许read和load、store和write操作之一单独出现。即使用了read必须load,使用了store必须write
-
不允许线程丢弃他最近的assign操作,即工作变量的数据改变了之后,必须告知主存
-
不允许一个线程将没有assign的数据从工作内存同步回主内存
-
一个新的变量必须在主内存中诞生,不允许工作内存直接使用一个未被初始化的变量。就是对变量实施use、
store操作之前,必须经过assign和load操作
-
一个变量同一时间只有一个线程能对其进行lock。多次lock后,必须执行相同次数的unlock才能解锁
-
如果对一个变量进行lock操作,会清空所有工作内存中此变量的值,在执行引擎使用这个变量前,必须重新
load或assign操作初始化变量的值
-
如果一个变量没有被lock,就不能对其进行unlock操作。也不能unlock一个被其他线程锁住的变量
-
对一个变量进行unlock操作之前,必须把此变量同步回主内存
所以需要A线程知道主存中的值被修改,即使用 volatile 关键词
17、Volatile
1、保证可见性
public class Test01 {
// 如果不加volatile 程序会死循环
// 加了volatile是可以保证可见性的
private volatile static int num = 0;
public static void main(String[] args) {
// main线程 和 测试线程
new Thread(()->{
while (num == 0) {}
}, "测试线程").start();
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
num = 1;
System.out.println(num);
}
}
2、不保证原子性
原子性:不可分割,线程A在执行任务的时候,不能被打扰的,也不能被分割的,要么同时成功,要么同时失败。
public class Test02 {
// volatile不保证原子性
private volatile static int number = 0;
public static void add() {
number++;
}
// 预计20w 实际 < 20w
public static void main(String[] args) {
for (int i = 0; i < 20; i++) {
new Thread(()->{
for (int j = 0; j < 10000; j++) {
add();
}
}).start();
}
while (Thread.activeCount() > 2) { // mian gc
Thread.yield();
}
System.out.println(Thread.currentThread().getName() + " " + number);
}
}
不使用Lock锁和synchronized情况下如何保证原子性?
使用原子类,解决 原子性问题
public class Test02 {
// volatile不保证原子性
// 原子类的 Integer
private volatile static AtomicInteger number = new AtomicInteger();
public static void add() {
// number++; // 不是一个原子性操作 字节码中有三个操作
number.getAndIncrement(); // AtomicInteger()+1操作 底层是CAS保证的原子性
}
// 预计20w 实际 < 20w
public static void main(String[] args) {
for (int i = 0; i < 20; i++) {
new Thread(()->{
for (int j = 0; j < 10000; j++) {
add();
}
}).start();
}
while (Thread.activeCount() > 2) { // mian gc
Thread.yield();
}
System.out.println(Thread.currentThread().getName() + " " + number);
}
}
这些类的底层都直接和操作系统挂钩!是在内存中修改值。Unsafe类是一个很特殊的存在;
3、禁止指令重排
什么是指令重排?
我们写的程序,计算机并不是按照我们自己写的那样去执行的
源代码–>编译器优化重排–>指令并行也可能会重排–>内存系统也会重排–>执行
处理器在进行指令重排的时候,会考虑数据之间的依赖性!
int x=1; //1
int y=2; //2
x=x+5; //3
y=x*x; //4
//我们期望的执行顺序是 1_2_3_4 可能执行的顺序会变成2134 1324
//可不可能是 4123? 不可能的
可能造成的影响结果:前提:a b x y这四个值 默认都是0
线程A | 线程B |
---|---|
x=a | y=b |
b=1 | a=2 |
正常的结果: x = 0; y =0;
线程A | 线程B |
---|---|
b=1 | a=2 |
x=a | y=b |
可能在线程A中会出现,先执行b=1, 然后执行x=a;
在B线程中可能会出现,先执行a=2,然后执行y=b;
那么就有可能结果如下:x=2; y=1;
volatile可以避免指令重排:
volatile中会加一道内存的屏障,这个内存屏障可以保证在这个屏障中的指令顺序。
内存屏障:CPU指令。作用:
1、保证特定的操作的执行顺序!
2、可以保证某些变量的内存可见性(利用这些特性,就可以保证volatile实现的可见性)
Q: 在哪里用这个内存屏障用得最多呢?单例模式
18、玩转单例模式
饿汉式、DCL懒汉式
饿汉式
/**
* 饿汉式单例
*/
public class Hungry {
/**
* 可能会浪费空间
*/
private byte[] data1=new byte[1024*1024];
private byte[] data2=new byte[1024*1024];
private byte[] data3=new byte[1024*1024];
private byte[] data4=new byte[1024*1024];
private Hungry(){
}
private final static Hungry hungry = new Hungry();
public static Hungry getInstance(){
return hungry;
}
}
DCL 懒汉式
//懒汉式单例模式
public class LazyMan {
private static boolean key = false;
private LazyMan(){
synchronized (LazyMan.class){
if (key==false){
key=true;
}
else{
throw new RuntimeException("不要试图使用反射破坏异常");
}
}
System.out.println(Thread.currentThread().getName()+" ok");
}
private volatile static LazyMan lazyMan;
//双重检测锁模式 简称DCL懒汉式
public static LazyMan getInstance(){
//需要加锁
if(lazyMan==null){
synchronized (LazyMan.class){
if(lazyMan==null){
lazyMan=new LazyMan();
/**
* 1、分配内存空间
* 2、执行构造方法,初始化对象
* 3、把这个对象指向这个空间
*
* 就有可能出现指令重排问题
* 比如执行的顺序是1 3 2 等
* 我们就可以添加volatile保证指令重排问题
*/
}
}
}
return lazyMan;
}
//单线程下 是ok的
//但是如果是并发的
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException, NoSuchFieldException {
//Java中有反射
// LazyMan instance = LazyMan.getInstance();
Field key = LazyMan.class.getDeclaredField("key");
key.setAccessible(true);
Constructor<LazyMan> declaredConstructor = LazyMan.class.getDeclaredConstructor(null);
declaredConstructor.setAccessible(true); //无视了私有的构造器
LazyMan lazyMan1 = declaredConstructor.newInstance();
key.set(lazyMan1,false);
LazyMan instance = declaredConstructor.newInstance();
System.out.println(instance);
System.out.println(lazyMan1);
System.out.println(instance == lazyMan1);
}
}
单例不安全,因为反射
枚举
//enum 是什么? enum本身就是一个Class 类
public enum EnumSingle {
INSTANCE;
public EnumSingle getInstance(){
return INSTANCE;
}
}
class Test{
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException {
EnumSingle instance1 = EnumSingle.INSTANCE;
Constructor<EnumSingle> declaredConstructor = EnumSingle.class.getDeclaredConstructor(String.class,int.class);
declaredConstructor.setAccessible(true);
//java.lang.NoSuchMethodException: com.ogj.single.EnumSingle.<init>()
EnumSingle instance2 = declaredConstructor.newInstance();
System.out.println(instance1);
System.out.println(instance2);
}
}
使用枚举,我们就可以防止反射破坏了。
枚举类型使用JAD最终反编译后源码:
如果我们看idea 的文件:会发现idea骗了我们,居然告诉我们是有有参构造的,我们使用jad进行反编译。
public final class EnumSingle extends Enum
{
public static EnumSingle[] values()
{
return (EnumSingle[])$VALUES.clone();
}
public static EnumSingle valueOf(String name)
{
return (EnumSingle)Enum.valueOf(com/ogj/single/EnumSingle, name);
}
private EnumSingle(String s, int i)
{
super(s, i);
}
public EnumSingle getInstance()
{
return INSTANCE;
}
public static final EnumSingle INSTANCE;
private static final EnumSingle $VALUES[];
static
{
INSTANCE = new EnumSingle("INSTANCE", 0);
$VALUES = (new EnumSingle[] {
INSTANCE
});
}
}
19、深入理解CAS
什么是CAS?
大厂必须深入研究底层!!!!修内功!操作系统、计算机网络原理、组成原理、数据结构
public class casDemo {
public static void main(String[] args) {
// CAS:compareAndSet 比较并交换
AtomicInteger atomicInteger = new AtomicInteger(2020);
//boolean compareAndSet(int expect, int update)
//期望值、更新值
//如果实际值 和 我的期望值相同,那么就更新
//如果实际值 和 我的期望值不同,那么就不更新
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
//因为期望值是2020 实际值却变成了2021 所以会修改失败
//CAS 是CPU的并发原语
atomicInteger.getAndIncrement(); // ++操作
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
}
}
Unsafa 类
总结:
CAS:比较当前工作内存中的值 和 主内存中的值,如果这个值是期望的,那么则执行操作!如果不是就一直循环,使用的是自旋锁。
缺点:
- 循环会耗时
- 一次性只能保证一个共享变量的原子性
- 它会存在ABA问题
CAS:ABA问题?(狸猫换太子)
线程1:期望值是1,要变成2;
线程2:两个操作:
- 1、期望值是1,变成3
- 2、期望是3,变成1
所以对于线程1来说,A的值还是1,所以就出现了问题,骗过了线程1
public class casDemo {
public static void main(String[] args) {
AtomicInteger atomicInteger = new AtomicInteger(2020);
// 捣乱的线程
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
System.out.println(atomicInteger.compareAndSet(2021, 2020));
System.out.println(atomicInteger.get());
// 期望的线程
System.out.println(atomicInteger.compareAndSet(2020, 5555));
System.out.println(atomicInteger.get());
}
}
20、原子引用
解决ABA问题,引入原子引用,对应的思想:乐观锁!
带版本号的原子操作
Integer 使用了对象缓存机制,默认范围是-128~127,推荐使用静态工厂方法valueOf获取对象实例,而不是new,因为valueOf使用缓存,而new一定会创建新的对象分配新的内存空间。
所以如果遇到,使用大于128的时候,使用原子引用的时候,如果超过了这个值,那么就不会进行版本上升!
public class casDemo {
public static void main(String[] args) {
// AtomicStampedReference 注意,如果泛型是一个包装类,注意对象的引用问题
// 实际业务中比较的是一个个对象
//初始值,版本号
static AtomicStampedReference<Integer> atomicStampedReference = new AtomicStampedReference<>(100, 1);
public static void main(String[] args) {
System.out.println("=============以下是ABA问题的解决===============");
new Thread(() -> {
//获取版本号1
int stamp = atomicStampedReference.getStamp();
System.out.println(Thread.currentThread().getName() + "\t 第1次版本号:" + stamp);
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
atomicStampedReference.compareAndSet(100, 101, atomicStampedReference.getStamp(), atomicStampedReference.getStamp() + 1);
System.out.println(Thread.currentThread().getName() + "\t 第2次版本号:" + atomicStampedReference.getStamp());
atomicStampedReference.compareAndSet(101, 100, atomicStampedReference.getStamp(), atomicStampedReference.getStamp() + 1);
System.out.println(Thread.currentThread().getName() + "\t 第3次版本号:" + atomicStampedReference.getStamp());
}, "t1").start();
// 与悲观锁的原理相同
new Thread(() -> {
//获取版本号1
int stamp = atomicStampedReference.getStamp();
System.out.println(Thread.currentThread().getName() + "\t 第1次版本号:" + stamp);
try {
TimeUnit.SECONDS.sleep(3);
} catch (InterruptedException e) {
e.printStackTrace();
}
boolean b = atomicStampedReference.compareAndSet(100, 111, stamp, stamp + 1);
System.out.println(Thread.currentThread().getName() + "\t修改成功否:" + b + "\t 当前最新实际版本号:" + atomicStampedReference.getStamp());
System.out.println(Thread.currentThread().getName() + "\t当前实际最新值::" + atomicStampedReference.getReference());
}, "t2").start();
}
}
21、各种锁的理解
1、公平锁、非公平锁
公平锁:非常公平;不能插队的,必须先来后到
// 源码
/**
* Creates an instance of {@code ReentrantLock}.
* This is equivalent to using {@code ReentrantLock(false)}.
*/
public ReentrantLock() {
sync = new NonfairSync();
}
非公平锁:非常不公平,允许插队的,可以改变顺序(默认都是非公平锁)。
/**
* Creates an instance of {@code ReentrantLock} with the
* given fairness policy.
*
* @param fair {@code true} if this lock should use a fair ordering policy
*/
public ReentrantLock(boolean fair) {
sync = fair ? new FairSync() : new NonfairSync();
}
2、可重入锁
1.什么是可重入锁?
可重入锁,也称递归锁,指的是同一线程外层函数获得锁之后,内层递归函数仍能能获取该锁。同一个线程中,在外层方法获取锁后,进入内层方法会自动获取锁。线程可以进入任何一个它已经拥有的锁所同步着的代码块。
ReentrantLock、Synchronized 都是典型的可重入锁。
2.可重入锁有什么作用?
可重入锁最大的作用就是可以避免死锁。
Synchronized 版
public class Demo01 {
public static void main(String[] args) {
Phone phone = new Phone();
new Thread(()->{
phone.sms();
}, "A").start();
new Thread(()->{
phone.sms();
}, "B").start();
}
}
class Phone {
public static synchronized void sms() {
System.out.println(Thread.currentThread().getName() + " sms");
call(); // 这里也有一把锁
}
private static synchronized void call() {
System.out.println(Thread.currentThread().getName() + " call");
}
}
Lock 版
public class Demo02 {
public static void main(String[] args) {
Phone2 phone = new Phone2();
new Thread(()->{
phone.sms();
}, "A").start();
new Thread(()->{
phone.sms();
}, "B").start();
}
}
class Phone2 {
static Lock lock = new ReentrantLock();
public static void sms() {
// 加锁
lock.lock(); // 细节:这个是两把锁,两个钥匙
try {
System.out.println(Thread.currentThread().getName() + " => sms");
call(); // 这里也有一把锁
} catch (Exception e) {
e.printStackTrace();
} finally {
// 释放锁
lock.unlock();
}
}
private static void call() {
lock.lock();
try {
System.out.println(Thread.currentThread().getName() + " => call");
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
- lock锁必须配对,相当于lock和 unlock 必须数量相同,否则就会死锁在里面
- 在外面加的锁,也可以在里面解锁;在里面加的锁,在外面也可以解锁
3、自旋锁
spinlock
自定义自旋锁
public class SpinLock {
// Integer 0
// Thread null
AtomicReference<Thread> atomicReference = new AtomicReference<>();
// 加锁
public void myLock() {
Thread thread = Thread.currentThread();
System.out.println(thread.currentThread().getName() + " ==> myLock");
// 自旋锁
while (!atomicReference.compareAndSet(null, thread)) {
System.out.println(Thread.currentThread().getName()+" ==> 自旋中~");
}
}
// 解锁
public void myUnLock() {
Thread thread = Thread.currentThread();
System.out.println(thread.currentThread().getName() + " ==> myUnLock");
atomicReference.compareAndSet(thread, null);
}
}
测试类
public class TestSpinLock {
public static void main(String[] args) throws InterruptedException {
// ReentrantLock reentrantLock = new ReentrantLock();
// reentrantLock.lock();
// reentrantLock.unlock();
SpinLock spinLock = new SpinLock();
// 底层使用CAS实现
new Thread(() -> {
spinLock.myLock();
try {
TimeUnit.SECONDS.sleep(3);
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
spinLock.myUnLock();
}
}, "T1").start();
TimeUnit.SECONDS.sleep(2);
new Thread(() -> {
spinLock.myLock();
try {
TimeUnit.SECONDS.sleep(5);
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
spinLock.myUnLock();
}
}, "T2").start();
/* T1.Lock() -- 2s后 --> T2.Lock()开始自旋 、T1.sleep(3)
--> T1.UnLock() --> T2.sleep(1) --> T2.UnLock()
* T1 进来是拿到锁,是期望的null,变成thread,此时T1没有自旋,而是跳出了循环,
* 这时候线程 T2 拿到锁后是 thread,进入while循环,开始自旋,
* 等待 T1 解锁变回 null,T2才能退出while循环,走出自旋
* T2线程必须等待T1线程Unlock后,才能Unlock,在这之前进行自旋等待
* */
}
}
4、死锁
解决问题
1、使用jps - l
定位进程号
2、使用jstack 进程号
找到死锁问题
面试,工作中!排查问题!
1、日志
2、堆栈信息