1 from random import random
2
3 def printIntro(): # 打印程序介绍信息
4 print('这个程序模拟两个队伍A和B的排球竞技比赛')
5 print('程序运行需要A和B的能力值(以0到1之间的小数表示)')
6
7 def getInputs(): # 获得程序运行参数
8 a = eval(input('请输入队伍A的能力值(0-1):'))
9 b = eval(input('请输入队伍B的能力值(0-1):'))
10 n = eval(input('模拟比赛场次:'))
11 return a, b, n
12
13 def simOneGame(probA, probB): # 进行决赛
14 scoreA, scoreB =0, 0
15 serving = 'A'
16 while not gameOver(scoreA, scoreB):
17 if serving == 'A':
18 if random() > probA:
19 scoreB += 1
20 serving = 'B'
21 else:
22 scoreA += 1
23 else:
24 if random() > probB:
25 scoreA += 1
26 serving = 'A'
27 else:
28 scoreB += 1
29 return scoreA, scoreB
30
31 def simfirstgame(probA, probB):
32 scoreA, scoreB = 0, 0
33 for i in range(4):
34 s1, s2=0, 0
35 while not gameover(s1, s2):
36 if random()<probA:
37 s1+=1
38 elif random()<probB:
39 s2+=1
40 if s1>s2:
41 scoreA+=1
42 else:
43 scoreB+=1
44 return scoreA, scoreB
45
46 def simNGames(n, probA, probB): #进行N场比赛
47 winsA, winsB = 0, 0 # 初始化AB的胜场数
48 for i in range(n):
49 k, l=simfirstgame(probA, probB)
50 if k==1:
51 winsB+=1
52 continue
53 elif k==3:
54 winsA+=1
55 continue
56 scoreA, scoreB = simOneGame(probA, probB)
57 if scoreA > scoreB:
58 winsA += 1
59 else:
60 winsB += 1
61 return winsA, winsB
62
63 def gameOver(c, d): #比赛结束
64 return (c>=15 and c-d>=2) or (d>=15 and d-c>=2)
65 def gameover(scoreA, scoreB):
66 return (scoreA>=25 and scoreA-scoreB>=2) or (scoreB>=25 and scoreB-scoreA>=2)
67
68
69 def printSummary(n ,winA, winB): #打印比赛结果
70 print('竞技分析开始,共模拟{}场比赛'.format(n))
71 print('队伍A获胜{}场比赛,占比{:.2f}%'.format(winA, winA/n*100))
72 print('队伍B获胜{}场比赛,占比{:.2f}%'.format(winB, winB / n * 100))
73 def main():
74 printIntro()
75 probA, probB, n =getInputs()
76 winsA, winsB = simNGames(n, probA, probB)
77 printSummary(n, winsA, winsB)
78
79 main()
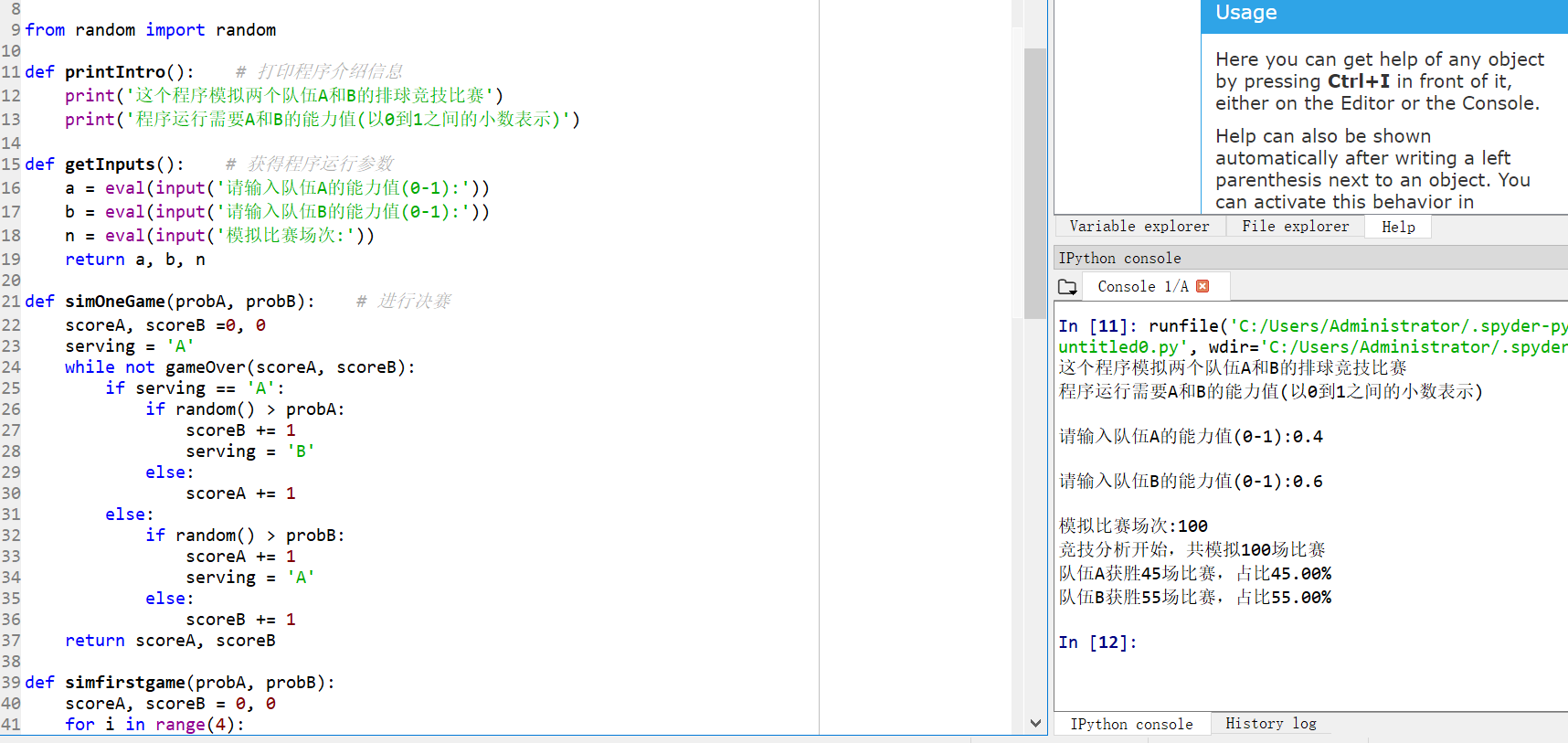