在做这个程序的时候发现,功能需求说明书里面的进程和应用程序信息是有关联的,所以,我就把这两个功能点合并起来做了。同样的,效果图先上:
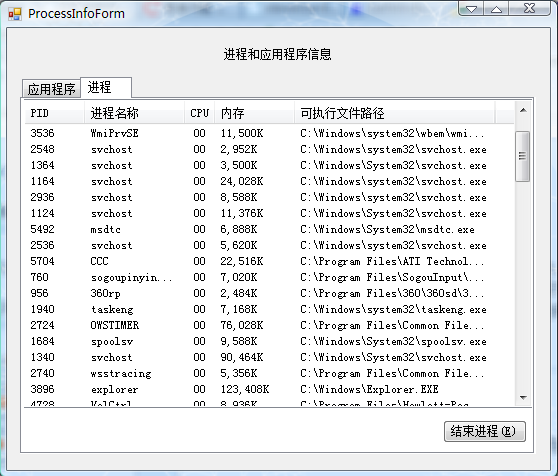
如图所示,在应用程序这个功能点上,我们需要做的是,获取当前正在运行的所有可见的应用程序的标题和这个应用程序的当前状态;在进程的功能点上,我们需要获取系统中正在运行的所有进程,需要获取的数据包括:进程ID,进程名称,进程的CPU使用率,进程占用的内存大小,进程的可执行文件路径。
根据以往的做法,需要有一个类,命名为:ProcessInfo,类图如下:
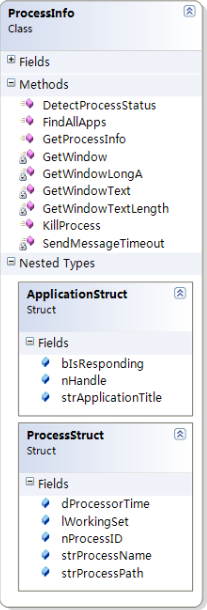
需要说明的一点是,这个类我没有采用以往那种一大堆属性的做法,尝试着用结构体的做法,在这个类里面构造了两个结构体,分别是进程信息和应用程序信息的结构体,而且,在获取数据方面,没有采用以往的WMI的方法来获取,转而采用了.NET FRAMEWORK自带的Process这个类和Windows API来获取信息。在这个功能点上,我借鉴了园里lemony朋友的《用C#开发较完整的Windows任务管理器》 在此表示感谢。
由于调试的时间比较少,代码上可能会出现些BUG,还望各位见谅哈。
OK,开始上代码,首先是ProcessInfo这个类的代码

ProcessInfo
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Management;
using System.Diagnostics;
using System.Runtime.InteropServices;
using System.Collections;

namespace ComputerInfo


{
public class ProcessInfo

{
//利用WMI的方法获取进程信息(本程序没用到)
//#region 进程ID
//int nProcessID = 0;

//public int ProcessID
//{
// get { return nProcessID; }
// set { nProcessID = value; }
//}
//#endregion

//#region 进程名称
//string strProcessName = string.Empty;

//public string ProcessName
//{
// get { return strProcessName; }
// set { strProcessName = value; }
//}
//#endregion

//#region 处理时间
//double dProcessorTime = 0.0;

//public double ProcessorTime
//{
// get { return dProcessorTime; }
// set { dProcessorTime = value; }
//}
//#endregion

//#region 使用内存
//long lWorkingSet = 0;

//public long WorkingSet
//{
// get { return lWorkingSet; }
// set { lWorkingSet = value; }
//}
//#endregion

//#region 进程路径
//string strProcessPath = string.Empty;

//public string ProcessPath
//{
// get { return strProcessPath; }
// set { strProcessPath = value; }
//}
//#endregion

//public void GetProcessInfo()
//{
// ProcessStruct ps;

// //实例化一个ManagementClass类,并将Win32_Process作为参数传递进去,
// //这样就可以查询Win32_Process这个类里面的一些信息了。
// ManagementClass mClass = new ManagementClass("Win32_Process");

// //获取Win32_Processor这个类的所有实例
// ManagementObjectCollection moCollection = mClass.GetInstances();

// //对Win32_Processor这个类进行遍历
// foreach (ManagementObject mObject in moCollection)
// {

// ps.nProcessID= mObject["Handle"].ToString(); //获取PID
// ps.strProcessName=mObject["Caption"].ToString(); //获取进程名称
// ps.dProcessorTime=mObject["Caption"].ToString(); //获取进程时间
// ps.lWorkingSet=mObject["WorkingSetSize"].ToString(); //获取进程时间
// ps.strProcessPath=mObject["ExecutablePath"].ToString(); //获取进程可执行路径

// lProcessInfo.Add(ps);
// }
//}


API声明#region API声明
[DllImport("User32")]
private extern static int GetWindow(int hWnd, int wCmd);

[DllImport("User32")]
private extern static int GetWindowLongA(int hWnd, int wIndx);

[DllImport("user32.dll")]
private static extern bool GetWindowText(int hWnd, StringBuilder title, int maxBufSize);

[DllImport("user32", CharSet = CharSet.Auto)]
private extern static int GetWindowTextLength(IntPtr hWnd);

[DllImport("user32.dll", EntryPoint = "SendMessageTimeout")]
private static extern int SendMessageTimeout(int hwnd, int msg, int wParam, int lParam, int fuFlags, int uTimeout, ref int lpdwResult);
#endregion


常量声明#region 常量声明
private const int GW_HWNDFIRST = 0;
private const int GW_HWNDNEXT = 2;
private const int GWL_STYLE = (-16);
private const int WS_VISIBLE = 268435456;
private const int WS_BORDER = 8388608;
private const int WS_POPUP = -2147483648;
private const int WS_CLIPSIBLINGS = 67108864;
private const int WS_THICKFRAME = 262144;
private const int WS_SYSMENU = 524288;
private const int HWND_BROADCAST = 0xffff;
private const int WM_NULL = 0x0;
private const int SMTO_ABORTIFHUNG = 0x2;
#endregion


进程信息结构体#region 进程信息结构体
public struct ProcessStruct

{
public int nProcessID;
public string strProcessName;
//public string strProcessTitle;
public double dProcessorTime;
public long lWorkingSet;
public string strProcessPath;
//public bool bIsResponding;
}
#endregion


应用程序信息结构体#region 应用程序信息结构体
public struct ApplicationStruct

{
public int nHandle;
public string strApplicationTitle;
public bool bIsResponding;
}
#endregion


获取所有进程信息#region 获取所有进程信息

/**//// <summary>
/// 获取所有进程信息
/// </summary>
/// <returns>进程的List<ProcessStruct></returns>
public List<ProcessStruct> GetProcessInfo()

{
List<ProcessStruct> lProcessInfo = new List<ProcessStruct>();
Process[] processes = Process.GetProcesses();
ProcessStruct ps;

foreach (Process process in processes)

{
ps = new ProcessStruct();
try

{
ps.nProcessID = process.Id;
ps.strProcessName = process.ProcessName;
//ps.strProcessTitle = process.MainWindowTitle; //没用了,已经用下面的获取应用程序标题取代了
ps.dProcessorTime = process.TotalProcessorTime.Milliseconds;
ps.lWorkingSet = process.WorkingSet64;
ps.strProcessPath = process.MainModule.FileName;
//ps.bIsResponding = process.Responding;
lProcessInfo.Add(ps);
}

catch
{ }
}
return lProcessInfo;
}
#endregion


结束指定进程#region 结束指定进程

/**//// <summary>
/// 结束指定进程
/// </summary>
/// <param name="nPID">进程ID</param>
/// <returns>是否正常结束进程</returns>
public bool KillProcess(int nPID)

{
try

{
Process process = Process.GetProcessById(nPID);
process.Kill();
return true;
}
catch

{
return false;
}
}
#endregion


查找所有应用程序标题#region 查找所有应用程序标题

/**//// <summary>
/// 查找所有应用程序标题和相应状态
/// </summary>
/// <returns>应用程序标题Hashtable</returns>
public List<ApplicationStruct> FindAllApps(int Handle)

{
List<ApplicationStruct> lApplication = new List<ApplicationStruct>();
ApplicationStruct applicationStruct;
int hwCurr;
hwCurr = GetWindow(Handle, GW_HWNDFIRST);

while (hwCurr > 0)

{
int IsTask = (WS_CLIPSIBLINGS | WS_THICKFRAME |WS_VISIBLE);
int lngStyle = GetWindowLongA(hwCurr, GWL_STYLE);
bool TaskWindow = ((lngStyle & IsTask) == IsTask);
if (TaskWindow)

{
int length = GetWindowTextLength(new IntPtr(hwCurr));
StringBuilder sb = new StringBuilder(2 * length + 1);
GetWindowText(hwCurr, sb, sb.Capacity);
string strTitle = sb.ToString();
if (!string.IsNullOrEmpty(strTitle))

{
bool bStatus = DetectProcessStatus(hwCurr);

applicationStruct.strApplicationTitle=strTitle;
applicationStruct.bIsResponding=bStatus;
applicationStruct.nHandle=hwCurr;

lApplication.Add(applicationStruct);
}
}
hwCurr = GetWindow(hwCurr, GW_HWNDNEXT);
}

return lApplication;
}
#endregion


检测应用程序的状态(正在运行,未响应)#region 检测应用程序的状态(正在运行,未响应)
int nResult;

/**//// <summary>
/// 检测应用程序的状态(正在运行,未响应)
/// </summary>
/// <param name="hwnd">需要检测应用程序的句柄</param>
/// <returns>是否正在运行</returns>
public bool DetectProcessStatus(int hwnd)

{
int nIsRunning = SendMessageTimeout(hwnd, WM_NULL, 0, 0, SMTO_ABORTIFHUNG, 5000, ref nResult);
if (nIsRunning != 0)

{
return true;
}
else

{
return false;
}
}
#endregion
}
}

跟以往一样,添加一个窗体,命名为:ProcessInfoForm,界面的布局如效果图所示,代码如下:

ProcessInfo Form
public partial class ProcessInfoForm : Form

{
public ProcessInfoForm()

{
InitializeComponent();
}
ComputerInfo.ProcessInfo processInfo = new ComputerInfo.ProcessInfo();
ComputerInfo.CPUInfo cpuInfo = new ComputerInfo.CPUInfo();

DateTime lastSysTime; //最后刷新时间, 用于计算进程 CPU 利用率
List<ComputerInfo.ProcessInfo.ProcessStruct> pInfo;
private void timer1_Tick(object sender, EventArgs e)

{

更新进程列表#region 更新进程列表
int oldWorkingSet = 0; //记录进程旧的内存大小
int oldTimePercent = 0; //记录进程旧的CPU百分比
int newTimePercent = 0;
int lvProcessCount = lvProcess.Items.Count;
TimeSpan ts = (TimeSpan)(DateTime.Now - lastSysTime);
double sysTimeSpan = ts.TotalMilliseconds;
Hashtable htProcess = new Hashtable(); //进程哈希表
pInfo = processInfo.GetProcessInfo();

for (int i = 0; i < pInfo.Count; i++)

{
htProcess.Add(pInfo[i].nProcessID.ToString(), pInfo[i].nProcessID);
ListViewItem item = lvProcessCount > 0 ? lvProcess.FindItemWithText(pInfo[i].nProcessID.ToString(), false, 0, false) : null;

更新进程#region 更新进程
if (item != null) //找到节点则更新

{

计算cpu占用率#region 计算cpu占用率
double processorTimeSpan = (double)Math.Abs(pInfo[i].dProcessorTime - (double)item.Tag);
if (sysTimeSpan != 0)

{
processorTimeSpan = processorTimeSpan / sysTimeSpan;
newTimePercent = (int)(processorTimeSpan * 100 / cpuInfo.CPUCount);
if (newTimePercent == 100)

{
newTimePercent = 99;
}
}
else

{
newTimePercent = 0;
}
#endregion
//缓冲,没有改变的数值不更新,避免界面闪烁
oldTimePercent = int.Parse(item.SubItems[2].Text);
if (newTimePercent != oldTimePercent)

{
item.SubItems[2].Text = string.Format("{0:00}", newTimePercent);
}
oldWorkingSet = int.Parse(item.SubItems[3].Text.Replace(",", "").Replace("K", ""));
if (pInfo[i].lWorkingSet != oldWorkingSet)

{
item.SubItems[3].Text = (pInfo[i].lWorkingSet / 1024).ToString("0,0") + "K";
}
item.Tag = pInfo[i].dProcessorTime;
}
else //否则添加节点

{
item = new ListViewItem(pInfo[i].nProcessID.ToString());
item.SubItems.Add(pInfo[i].strProcessName);
item.SubItems.Add(string.Format("{0:00}", 0));
item.SubItems.Add((pInfo[i].lWorkingSet / 1024).ToString("0,0") + "K");
item.SubItems.Add(pInfo[i].strProcessPath);
item.Tag = pInfo[i].dProcessorTime;
lvProcess.Items.Add(item); //add
}
#endregion
}


删除过时的进程#region 删除过时的进程
//删除过时的进程
if (lvProcess.Items.Count != htProcess.Count)

{
foreach (ListViewItem item in lvProcess.Items)

{
if (!htProcess.ContainsKey(item.Text))

{
lvProcess.Items.Remove(item);
}
}
}
#endregion



lastSysTime = DateTime.Now;
#endregion
}


private void ProcessInfoForm_Load(object sender, EventArgs e)

{
cpuInfo.GetCPUInfo();
}

List<ComputerInfo.ProcessInfo.ApplicationStruct> applicationInfo;
private void tmrApp_Tick(object sender, EventArgs e)

{
Hashtable htApp = new Hashtable();
applicationInfo = processInfo.FindAllApps(this.Handle.ToInt32());
int lvApplicationCount = lvApplication.Items.Count;
string oldTitle = string.Empty;
string oldStatus = string.Empty;
for (int i = 0; i < applicationInfo.Count; i++)

{
string strStatus = (applicationInfo[i].bIsResponding) ? "正在运行" : "未响应";
if (!htApp.ContainsKey(applicationInfo[i].strApplicationTitle))

{
htApp.Add(applicationInfo[i].strApplicationTitle, applicationInfo[i].nHandle);
}

ListViewItem item = lvApplicationCount > 0 ? lvApplication.FindItemWithText(applicationInfo[i].strApplicationTitle, false, 0, false) : null;
if (item != null) //找到节点则更新

{
//缓冲,没有改变的数值不更新,避免界面闪烁
oldTitle = applicationInfo[i].strApplicationTitle;
if (oldTitle != applicationInfo[i].strApplicationTitle)

{
item.Text = applicationInfo[i].strApplicationTitle;
}
oldStatus = strStatus;
if (oldStatus != strStatus)

{
item.SubItems[1].Text = strStatus;
}
item.Tag = applicationInfo[i].nHandle;
}
else //否则添加节点

{
item = new ListViewItem(applicationInfo[i].strApplicationTitle);
item.SubItems.Add(strStatus);

item.Tag = applicationInfo[i].nHandle;
lvApplication.Items.Add(item); //add
}
}

删除过时的应用程序#region 删除过时的应用程序
if (lvApplication.Items.Count != htApp.Count)

{
foreach (ListViewItem tempitem in lvApplication.Items)

{
if (!htApp.ContainsKey(tempitem.Text))

{
lvApplication.Items.Remove(tempitem);
}
}
}
#endregion
}

private void btnEndProcess_Click(object sender, EventArgs e)

{
if (lvProcess.SelectedItems.Count > 0)

{
ListViewItem item = lvProcess.SelectedItems[0];
int pid = int.Parse(item.Text);
string pName = item.SubItems[1].Text;
DialogResult dr = MessageBox.Show(string.Format("确定要结束进程 {0} 吗?", pName),
"警告", MessageBoxButtons.OKCancel, MessageBoxIcon.Warning);
if (dr == DialogResult.OK)

{
processInfo.KillProcess(pid);
}
}
}
}
OK,这样就完成了这两个小功能,代码上有点乱七八糟的感觉,哈哈,还没去整理,等最后整合所有的小程序再整理吧,毕竟这只是的DEMO。哈哈
未完,待续~~~