1120day-户别确认
1、实体类
package com.edu.empity;
public class People {
private String hubie;
private String livetype;
private String area;
private String roomnum;
private String name;
private String idcard;
private String sex;
private String nation;
private String education;
public String getHubie() {
return hubie;
}
public void setHubie(String hubie) {
this.hubie = hubie;
}
public String getLivetype() {
return livetype;
}
public void setLivetype(String livetype) {
this.livetype = livetype;
}
public String getArea() {
return area;
}
public void setArea(String area) {
this.area = area;
}
public String getRoomnum() {
return roomnum;
}
public void setRoomnum(String roomnum) {
this.roomnum = roomnum;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getIdcard() {
return idcard;
}
public void setIdcard(String idcard) {
this.idcard = idcard;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getNation() {
return nation;
}
public void setNation(String nation) {
this.nation = nation;
}
public String getEducation() {
return education;
}
public void setEducation(String education) {
this.education = education;
}
public People(String hubie, String livetype, String area, String roomnum, String name, String idcard, String sex,
String nation, String education) {
super();
this.hubie = hubie;
this.livetype = livetype;
this.area = area;
this.roomnum = roomnum;
this.name = name;
this.idcard = idcard;
this.sex = sex;
this.nation = nation;
this.education = education;
}
public People() {
// TODO 自动生成的构造函数存根
}
}
2、dao层
package com.edu.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import com.edu.dbu.DBUtil;
import com.edu.empity.People;
public class Peopledao {
public List<People> getallPeople() {
List<People> list = new ArrayList<People>();
Connection conn = null;
conn = DBUtil.getConnection();
PreparedStatement pst =null;
ResultSet rs = null;
String sql = "select * from people";
try {
pst = conn.prepareStatement(sql);
rs = pst.executeQuery();
while(rs.next()) {
People people = new People();
people.setHubie(rs.getString("hubie"));
people.setHubie(rs.getString("hubie"));
people.setHubie(rs.getString("hubie"));
people.setHubie(rs.getString("hubie"));
people.setHubie(rs.getString("hubie"));
people.setHubie(rs.getString("hubie"));
people.setHubie(rs.getString("hubie"));
}
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
return list;
}
public boolean Addpeople(People people) throws SQLException {
// TODO 自动生成的方法存根
String sql = "insert into people(hubie,livetype,area,roomnum,name,idcard,sex,nation,education)values(?,?,?,?,?,?,?,?,?)";
Connection conn = DBUtil.getConnection();
PreparedStatement pst =null;
int p =0;
try {
pst = conn.prepareStatement(sql);
pst.setString(1, people.getHubie());
pst.setString(2, people.getLivetype());
pst.setString(3, people.getArea());
pst.setString(4, people.getRoomnum());
pst.setString(5, people.getName());
pst.setString(6, people.getIdcard());
pst.setString(7, people.getSex());
pst.setString(8, people.getNation());
pst.setString(9, people.getEducation());
p = pst.executeUpdate();
pst.close();
conn.close();
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}finally {
if(p != 0) {
System.out.println("dao信息添加成功");
}
}
return true;
}
public boolean Updatepeople(People people) throws SQLException {
// TODO 自动生成的方法存根
Connection conn = DBUtil.getConnection();
String sql = "update people set hubie=? livetype=? area=? roomnum=? idcard=? sex=? nation=? education=? where name = ?";
PreparedStatement pst =null;
int p =0;
try {
pst = conn.prepareStatement(sql);
pst.setString(1, people.getHubie());
pst.setString(2, people.getLivetype());
pst.setString(3, people.getArea());
pst.setString(4, people.getRoomnum());
pst.setString(5, people.getIdcard());
pst.setString(6, people.getSex());
pst.setString(7, people.getNation());
pst.setString(8, people.getEducation());
pst.setString(9, people.getName());
p = pst.executeUpdate();
pst.close();
conn.close();
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}finally {
if(p != 0) {
System.out.println("dao信息修改成功");
}
}
return true;
}
public boolean Deletepeople(String name) throws SQLException {
// TODO 自动生成的方法存根
Connection conn = DBUtil.getConnection();
String sql = "delete from people where name = ?";
PreparedStatement pst =null;
int p =0;
try {
pst = conn.prepareStatement(sql);
pst.setString(1, name);
p = pst.executeUpdate();
pst.close();
conn.close();
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}finally {
if(p != 0) {
System.out.println("dao信息删除成功");
}
}
return true;
}
}
3、servlet
1)Add
package com.edu.servlet;
import java.io.IOException;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.edu.dao.Peopledao;
import com.edu.empity.People;
@WebServlet("/Addservlet")
public class Addservlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
String hubie = request.getParameter("hubie");
String livetype = request.getParameter("livetype");
String area = request.getParameter("area");
String roomnum = request.getParameter("roomnum");
String name = request.getParameter("name");
String idcard = request.getParameter("idcard");
String sex = request.getParameter("sex");
String nation = request.getParameter("nation");
String education = request.getParameter("education");
System.out.println(hubie+livetype+area+roomnum+name+idcard+sex+nation+education);
Peopledao dao = new Peopledao();
People people = new People(hubie,livetype,area,roomnum,name,idcard,sex,nation,education);
boolean flag = false;
try {
flag=dao.Addpeople(people);
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}finally {
if(flag) {
System.out.println("servlet信息添加成功");
}
}
request.getRequestDispatcher("showpeople.jsp").forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
2)delete
package com.edu.servlet;
import java.io.IOException;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.edu.dao.Peopledao;
@WebServlet("/Deleteservlet")
public class Deleteservlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
String name = request.getParameter("name");
Peopledao dao = new Peopledao();
try {
if(name!=null) {
dao.Deletepeople(name);
}
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
request.getRequestDispatcher("showpeople.jsp").forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
3)update
package com.edu.servlet;
import java.io.IOException;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.edu.dao.Peopledao;
import com.edu.empity.People;
@WebServlet("/Updateservlet")
public class Updateservlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
String hubie = request.getParameter("hubie");
String livetype = request.getParameter("livetype");
String area = request.getParameter("area");
String roomnum = request.getParameter("roomnum");
String name = request.getParameter("name");
String idcard = request.getParameter("idcard");
String sex = request.getParameter("sex");
String nation = request.getParameter("nation");
String education = request.getParameter("education");
People people = new People(hubie,livetype,area,roomnum,name,idcard,sex,nation,education);
Peopledao dao = new Peopledao();
try {
if(name != null) {
dao.Updatepeople(people);
}
} catch (SQLException e) {
// TODO 自动生成的 catch 块
e.printStackTrace();
}
request.getRequestDispatcher("showpeople.jsp").forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
__EOF__
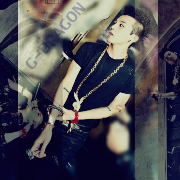
本文链接:https://www.cnblogs.com/lx06/p/14164810.html
关于博主:评论和私信会在第一时间回复。或者直接私信我。
版权声明:本博客转载请注明出处!
声援博主:如果您觉得文章对您有帮助,可以点击文章右下角【推荐】一下。您的鼓励是博主的最大动力!
【推荐】编程新体验,更懂你的AI,立即体验豆包MarsCode编程助手
【推荐】凌霞软件回馈社区,博客园 & 1Panel & Halo 联合会员上线
【推荐】抖音旗下AI助手豆包,你的智能百科全书,全免费不限次数
【推荐】博客园社区专享云产品让利特惠,阿里云新客6.5折上折
【推荐】轻量又高性能的 SSH 工具 IShell:AI 加持,快人一步
· .NET Core内存结构体系(Windows环境)底层原理浅谈
· C# 深度学习:对抗生成网络(GAN)训练头像生成模型
· .NET 适配 HarmonyOS 进展
· .NET 进程 stackoverflow异常后,还可以接收 TCP 连接请求吗?
· SQL Server统计信息更新会被阻塞或引起会话阻塞吗?
· 本地部署 DeepSeek:小白也能轻松搞定!
· 传国玉玺易主,ai.com竟然跳转到国产AI
· 自己如何在本地电脑从零搭建DeepSeek!手把手教学,快来看看! (建议收藏)
· 我们是如何解决abp身上的几个痛点
· 如何基于DeepSeek开展AI项目