A. Game With Sticks
time limit per test:1 second
memory limit per test:256 megabytes
input:standard input
output:standard output
After winning gold and silver in IOI 2014, Akshat and Malvika want to have some fun. Now they are playing a game on a grid made of nhorizontal and m vertical sticks.
An intersection point is any point on the grid which is formed by the intersection of one horizontal stick and one vertical stick.
In the grid shown below, n = 3 and m = 3. There are n + m = 6 sticks in total (horizontal sticks are shown in red and vertical sticks are shown in green). There are n·m = 9 intersection points, numbered from 1 to 9.

The rules of the game are very simple. The players move in turns. Akshat won gold, so he makes the first move. During his/her move, a player must choose any remaining intersection point and remove from the grid all sticks which pass through this point. A player will lose the game if he/she cannot make a move (i.e. there are no intersection points remaining on the grid at his/her move).
Assume that both players play optimally. Who will win the game?
The first line of input contains two space-separated integers, n and m (1 ≤ n, m ≤ 100).
Print a single line containing "Akshat" or "Malvika" (without the quotes), depending on the winner of the game.
2 2
Malvika
2 3
Malvika
3 3
Akshat
Explanation of the first sample:
The grid has four intersection points, numbered from 1 to 4.

If Akshat chooses intersection point 1, then he will remove two sticks (1 - 2 and 1 - 3). The resulting grid will look like this.
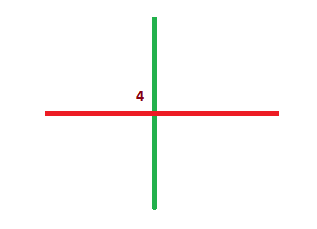
Now there is only one remaining intersection point (i.e. 4). Malvika must choose it and remove both remaining sticks. After her move the grid will be empty.
In the empty grid, Akshat cannot make any move, hence he will lose.
Since all 4 intersection points of the grid are equivalent, Akshat will lose no matter which one he picks.
题意 :给定n×m根线,如图摆放,将交点从1到n×m编号,两个人轮流走到交点上,然后将经过这个交点的某条线移走,最后没有交点可走的人输。
思路 :因为拿走一根线的话,所有别的跟这个线的交点也就没有了,所以实际上求的是min(n,m)对2取余。

1 //258A 2 #include <cstdio> 3 #include <cstring> 4 #include <iostream> 5 6 using namespace std ; 7 8 int main() 9 { 10 int n, m ; 11 while(scanf("%d %d",&n,&m) != EOF) 12 { 13 int s = min(n,m) ; 14 if(s % 2) 15 puts("Akshat") ; 16 else puts("Malvika") ; 17 } 18 return 0 ; 19 }
B. Sort the Array
time limit per test:1 second
memory limit per test:256 megabytes
input:standard input
output:standard output
Being a programmer, you like arrays a lot. For your birthday, your friends have given you an array a consisting of n distinct integers.
Unfortunately, the size of a is too small. You want a bigger array! Your friends agree to give you a bigger array, but only if you are able to answer the following question correctly: is it possible to sort the array a (in increasing order) by reversing exactly one segment of a? See definitions of segment and reversing in the notes.
The first line of the input contains an integer n (1 ≤ n ≤ 105) — the size of array a.
The second line contains n distinct space-separated integers: a[1], a[2], ..., a[n] (1 ≤ a[i] ≤ 109).
Print "yes" or "no" (without quotes), depending on the answer.
If your answer is "yes", then also print two space-separated integers denoting start and end (start must not be greater than end) indices of the segment to be reversed. If there are multiple ways of selecting these indices, print any of them.
3
3 2 1
yes
1 3
4
2 1 3 4
yes
1 2
4
3 1 2 4
no
2
1 2
yes
1 1
Sample 1. You can reverse the entire array to get [1, 2, 3], which is sorted.
Sample 3. No segment can be reversed such that the array will be sorted.
Definitions
A segment [l, r] of array a is the sequence a[l], a[l + 1], ..., a[r].
If you have an array a of size n and you reverse its segment [l, r], the array will become:
a[1], a[2], ..., a[l - 2], a[l - 1], a[r], a[r - 1], ..., a[l + 1], a[l], a[r + 1], a[r + 2], ..., a[n - 1], a[n].
题意 : 通过将数组a里的某一段逆置之后,能否将这个数组变成有序的。
思路 :既然是逆置的,例如1 2 3 6 5 4 7 8 9
那就从头开始找大于下一个数的那个数的位置,然后再接着找小于下一个数的那个数的位置,将这两者之间的逆置,如果这样是有序的了,就yes,除此之外就是不可能的情况了,比如有两段需要逆置,或者这段逆置的也不是倒着有序的等等。

1 //258B 2 #include <cstdio> 3 #include <cstring> 4 #include <string> 5 #include <iostream> 6 #include <algorithm> 7 8 using namespace std ; 9 10 int a[100010],b[100010] ; 11 12 int main() 13 { 14 int n ; 15 while(cin >> n) 16 { 17 for(int i = 0 ; i < n ; i++) 18 { 19 cin >> a[i] ; 20 b[i] = a[i] ; 21 } 22 int be = -1,en = -1 ; 23 for(int i = 0 ; i < n-1 ; i++) 24 { 25 if(a[i] > a[i+1]) 26 { 27 be = i ; 28 break ; 29 } 30 } 31 if(be == -1) {puts("yes") ; puts("1 1");continue ;} 32 int i ; 33 for(i = be ; i < n-1 ; i++) 34 { 35 if(a[i] < a[i+1]) 36 { 37 en = i ; 38 break ; 39 } 40 } 41 if(i == n-1) en = n-1 ; 42 reverse(a+be,a+en+1) ; 43 sort(b,b+n) ; 44 for(i = 0 ; i < n ; i++) 45 { 46 if(a[i] != b[i]) break ; 47 } 48 if(i == n) { 49 puts("yes") ; 50 cout << be +1 << " "<< 1 + en <<endl ; 51 } 52 else puts("no") ; 53 } 54 return 0 ; 55 }